Python Programming: A Comprehensive Guide for Beginners
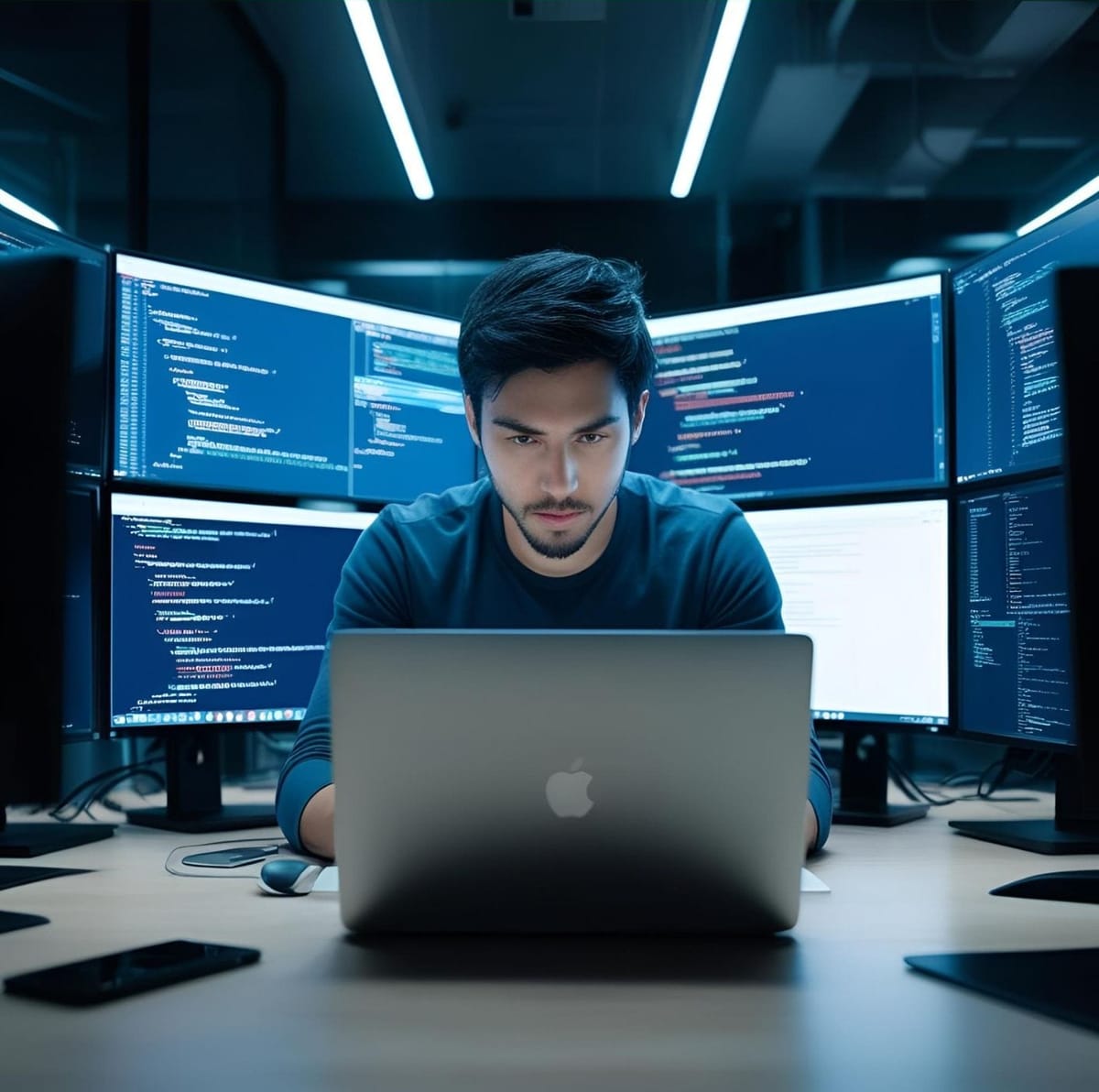
Python Programming: A Comprehensive Guide for Beginners
Table of Contents
- Introduction
- Getting Started with Python
- Python Basics
- Control Flow
- Functions
- Data Structures in Depth
- Working with Files
- Error Handling
- Modules and Packages
- Object-Oriented Programming
- Working with External Libraries
- Practical Python Projects
- Python Best Practices
- Advanced Python Concepts
- Resources for Further Learning
- Conclusion
Introduction
Python is a powerful, versatile, and beginner-friendly programming language that has gained immense popularity in recent years. Created by Guido van Rossum and first released in 1991, Python's philosophy emphasizes code readability and simplicity, making it an excellent choice for beginners.
What is Python?
Python is a high-level, interpreted programming language that supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Its syntax is designed to be readable and concise, using English keywords and requiring fewer lines of code compared to other languages.
Unlike languages such as C++ or Java that need to be compiled before execution, Python code is processed at runtime by an interpreter. This means you can write and run Python code without an intermediate compilation step, making the development process more efficient and interactive.
Why Learn Python?
There are numerous compelling reasons to learn Python:
- Easy to Learn: Python's syntax is clear and intuitive, making it accessible for beginners.
- Versatility: Python can be used for web development, data analysis, artificial intelligence, scientific computing, automation, and more.
- Community Support: Python has a vast and active community that contributes to libraries, frameworks, and provides support.
- Career Opportunities: Python skills are in high demand across various industries.
- Productivity: Python's simplicity and extensive libraries allow for rapid development.
- Cross-platform: Python runs on various operating systems like Windows, macOS, and Linux.
- Integration Capabilities: Python can easily integrate with other programming languages and technologies.
Python's Popularity and Applications
Python consistently ranks among the top programming languages in popularity indexes. Its applications span across various domains:
Domain | Applications | Popular Libraries/Frameworks |
---|---|---|
Web Development | Backend services, APIs | Django, Flask, FastAPI |
Data Science | Data analysis, visualization | NumPy, Pandas, Matplotlib |
Machine Learning | Predictive modeling, classification | TensorFlow, PyTorch, scikit-learn |
Automation | Scripting, testing | Selenium, Pytest |
Scientific Computing | Research, simulations | SciPy, SymPy |
Game Development | 2D games, game logic | Pygame |
Desktop Applications | GUI applications | Tkinter, PyQt, Kivy |
Internet of Things | Device programming | MicroPython, PyBoard |
Cybersecurity | Security tools, penetration testing | Scapy, Nmap |
Finance | Algorithmic trading, risk analysis | Quantlib, Zipline |
Who This Guide Is For
This comprehensive guide is designed for absolute beginners with no prior programming experience. However, it also serves as a thorough reference for those familiar with other programming languages who want to learn Python. By the end of this guide, you'll have a solid foundation in Python programming and be equipped to tackle more advanced topics and projects.
Getting Started with Python
Before diving into the language itself, let's set up your Python development environment.
Installing Python
Windows Installation
- Visit the official Python website at python.org.
- Download the latest Python installer for Windows.
- Run the installer. Important: Check the box that says "Add Python to PATH" before clicking "Install Now."
- Verify the installation by opening Command Prompt and typing:
This should display the Python version you installed.python --version
macOS Installation
macOS comes with Python pre-installed, but it might be an older version. To install the latest:
- The recommended approach is to use Homebrew, a package manager for macOS:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)" brew install python
- Alternatively, download the macOS installer from python.org.
- Verify the installation by opening Terminal and typing:
python3 --version
Linux Installation
Most Linux distributions come with Python pre-installed. To install the latest version:
For Ubuntu/Debian:
sudo apt update
sudo apt install python3 python3-pip
For Fedora:
sudo dnf install python3 python3-pip
Verify the installation:
python3 --version
Setting Up Your Development Environment
A proper development environment enhances productivity and makes coding more enjoyable. Here are some essential tools:
Python IDEs and Text Editors
Tool | Type | Key Features | Best For |
---|---|---|---|
Visual Studio Code | Text Editor | Free, lightweight, extensive extensions | General-purpose Python development |
PyCharm | IDE | Intelligent code completion, debugging, built-in tools | Professional Python development |
Jupyter Notebooks | Notebook Interface | Interactive cells, inline visualizations | Data analysis, experimentation |
IDLE | Simple IDE | Comes with Python, basic features | Beginners, simple scripts |
Sublime Text | Text Editor | Fast, customizable | Quick edits, lightweight projects |
Atom | Text Editor | Open-source, customizable | Collaborative development |
Spyder | IDE | Scientific computing, integration with data science libraries | Data science projects |
Thonny | IDE | Designed for beginners, simplified interface | Learning Python fundamentals |
For beginners, we recommend starting with Visual Studio Code or PyCharm Community Edition.
Installing Visual Studio Code and Python Extension
- Download and install VS Code from code.visualstudio.com.
- Open VS Code and navigate to the Extensions view (Ctrl+Shift+X).
- Search for "Python" and install the Microsoft Python extension.
- You're now ready to write Python code in VS Code!
Running Your First Python Program
Let's write the traditional "Hello, World!" program:
- Open your chosen editor.
- Create a new file named
hello.py
. - Add the following line of code:
print("Hello, World!")
- Save the file.
- Run the program:
- In VS Code: Right-click in the editor and select "Run Python File in Terminal"
- In PyCharm: Right-click in the editor and select "Run 'hello'"
- In Terminal/Command Prompt: Navigate to the directory containing your file and type
python hello.py
Congratulations! You've just run your first Python program. Now, let's dive deeper into the language.
Python Basics
Python Syntax Overview
Python's syntax is designed to be readable and concise. Here are some key characteristics:
- Indentation: Python uses indentation (whitespace) to define code blocks, rather than curly braces or keywords.
- Line Endings: Statements typically end with a newline, not semicolons.
- Comments: Use
#
for single-line comments and triple quotes ('''
or"""
) for multi-line comments. - Case Sensitivity: Python is case-sensitive (
variable
andVariable
are different).
# This is a comment
print("Hello, World!") # This is also a comment
# Python uses indentation for blocks
if True:
print("This is indented")
if True:
print("This is further indented")
"""
This is a multi-line
comment (also called a docstring
when used at the beginning of a function or class)
"""
Variables and Data Types
Variables in Python are created when you assign a value to them. Python is dynamically typed, meaning you don't need to declare variable types explicitly.
# Variable assignment
name = "John"
age = 30
height = 5.9
is_student = True
Numbers
Python supports several numeric types:
-
Integers (int): Whole numbers without a decimal point.
count = 10 negative_number = -5 big_number = 1_000_000 # Underscores for readability (Python 3.6+)
-
Floating-point numbers (float): Numbers with a decimal point.
price = 19.99 pi = 3.14159 scientific = 1.23e4 # Scientific notation: 12300.0
-
Complex numbers: Numbers with a real and imaginary part.
complex_num = 3 + 4j
Strings
Strings are sequences of characters, enclosed in either single ('
) or double ("
) quotes.
name = "Alice"
message = 'Hello, World!'
multi_line = """This is a
multi-line
string."""
Common string operations:
# Concatenation
full_name = "John " + "Doe"
# String repetition
repeated = "Python " * 3 # "Python Python Python "
# Indexing (0-based)
first_char = name[0] # 'A'
# Slicing
substring = name[1:3] # 'li'
# Length
name_length = len(name) # 5
# String methods
uppercase = name.upper() # 'ALICE'
lowercase = name.lower() # 'alice'
replaced = name.replace('A', 'E') # 'Elice'
Booleans
Boolean values represent truth values: True
or False
.
is_valid = True
has_error = False
Booleans are often the result of comparison operations:
is_adult = age >= 18 # True if age is 18 or more
Lists
Lists are ordered, mutable collections of items that can be of different types.
fruits = ["apple", "banana", "orange"]
mixed_list = [1, "hello", True, 3.14]
Common list operations:
# Accessing elements (0-based indexing)
first_fruit = fruits[0] # "apple"
# Modifying elements
fruits[1] = "grape" # Changes "banana" to "grape"
# Adding elements
fruits.append("kiwi") # Adds "kiwi" to the end
fruits.insert(1, "pear") # Inserts "pear" at index 1
# Removing elements
fruits.remove("apple") # Removes "apple"
last_fruit = fruits.pop() # Removes and returns the last element
# List length
num_fruits = len(fruits)
# Checking membership
has_apple = "apple" in fruits # True if "apple" is in fruits
Tuples
Tuples are ordered, immutable collections of items.
coordinates = (10, 20)
rgb_color = (255, 0, 0)
single_item_tuple = (42,) # Note the comma
Since tuples are immutable, you cannot change their elements after creation. However, you can access elements similarly to lists:
x = coordinates[0] # 10
y = coordinates[1] # 20
Dictionaries
Dictionaries are unordered collections of key-value pairs.
person = {
"name": "John",
"age": 30,
"city": "New York"
}
Common dictionary operations:
# Accessing values
name = person["name"] # "John"
age = person.get("age") # 30 (safer method if key might not exist)
# Modifying values
person["age"] = 31
# Adding new key-value pairs
person["job"] = "Engineer"
# Removing key-value pairs
del person["city"]
job = person.pop("job") # Removes and returns the value
# Checking if a key exists
has_name = "name" in person # True
# Getting all keys and values
keys = person.keys()
values = person.values()
items = person.items() # Returns (key, value) pairs
Sets
Sets are unordered collections of unique items.
unique_numbers = {1, 2, 3, 4, 5}
fruits_set = {"apple", "banana", "orange"}
Common set operations:
# Adding elements
fruits_set.add("kiwi")
# Removing elements
fruits_set.remove("banana") # Raises error if not found
fruits_set.discard("grape") # Does not raise error if not found
# Set operations
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union = set1 | set2 # {1, 2, 3, 4, 5}
intersection = set1 & set2 # {3}
difference = set1 - set2 # {1, 2}
Operators
Python supports various types of operators for different operations:
Arithmetic Operators
Operator | Description | Example |
---|---|---|
+ |
Addition | 5 + 3 gives 8 |
- |
Subtraction | 5 - 3 gives 2 |
* |
Multiplication | 5 * 3 gives 15 |
/ |
Division (returns float) | 5 / 3 gives 1.6666... |
// |
Floor Division (returns int) | 5 // 3 gives 1 |
% |
Modulus (remainder) | 5 % 3 gives 2 |
** |
Exponentiation | 5 ** 3 gives 125 |
Comparison Operators
Operator | Description | Example |
---|---|---|
== |
Equal to | 5 == 5 gives True |
!= |
Not equal to | 5 != 3 gives True |
> |
Greater than | 5 > 3 gives True |
< |
Less than | 5 < 3 gives False |
>= |
Greater than or equal to | 5 >= 5 gives True |
<= |
Less than or equal to | 3 <= 5 gives True |
Logical Operators
Operator | Description | Example |
---|---|---|
and |
True if both operands are true | True and False gives False |
or |
True if at least one operand is true | True or False gives True |
not |
Inverts the truth value | not True gives False |
Assignment Operators
Operator | Description | Example |
---|---|---|
= |
Assigns value | x = 5 |
+= |
Add and assign | x += 3 (equivalent to x = x + 3 ) |
-= |
Subtract and assign | x -= 3 (equivalent to x = x - 3 ) |
*= |
Multiply and assign | x *= 3 (equivalent to x = x * 3 ) |
/= |
Divide and assign | x /= 3 (equivalent to x = x / 3 ) |
%= |
Modulus and assign | x %= 3 (equivalent to x = x % 3 ) |
**= |
Exponentiate and assign | x **= 3 (equivalent to x = x ** 3 ) |
Identity Operators
Operator | Description | Example |
---|---|---|
is |
True if operands are identical | x is y |
is not |
True if operands are not identical | x is not y |
Identity operators check if two variables refer to the same object in memory, not just if they have the same value.
a = [1, 2, 3]
b = [1, 2, 3]
c = a
print(a == b) # True (same values)
print(a is b) # False (different objects)
print(a is c) # True (same object)
Membership Operators
Operator | Description | Example |
---|---|---|
in |
True if value is found in the sequence | 3 in [1, 2, 3] gives True |
not in |
True if value is not found in the sequence | 4 not in [1, 2, 3] gives True |
Comments and Documentation
Comments are crucial for code readability and maintenance:
# This is a single-line comment
"""
This is a multi-line comment
or docstring that can span
multiple lines
"""
def calculate_area(radius):
"""
Calculate the area of a circle.
Args:
radius (float): The radius of the circle
Returns:
float: The area of the circle
"""
return 3.14159 * radius ** 2
Best practices for commenting:
- Use comments to explain "why" rather than "what" (the code already shows what it does).
- Keep comments up-to-date when you change code.
- Use docstrings for functions, classes, and modules to document their purpose and usage.
- Follow a consistent style guide (like Google's Python Style Guide or PEP 257).
Control Flow
Control flow refers to the order in which statements are executed in a program. Python provides several constructs to control this flow.
Conditional Statements
Conditional statements allow you to execute different code blocks based on different conditions.
if, elif, else
age = 20
if age < 13:
print("Child")
elif age < 18:
print("Teenager")
elif age < 65:
print("Adult")
else:
print("Senior")
Conditional expressions can be combined using logical operators:
if (age >= 18) and (age < 21):
print("You can vote but cannot drink in the US")
Ternary Operator (Conditional Expression)
Python also has a compact way to write simple if-else statements:
status = "Adult" if age >= 18 else "Minor"
Nested Conditions
You can nest conditional statements within each other:
if age >= 18:
if gender == "Male":
print("Mr.")
else:
print("Ms./Mrs.")
else:
print("Young person")
Loops
Loops allow you to execute a block of code multiple times.
for Loops
The for
loop in Python iterates over a sequence (like a list, tuple, dictionary, set, or string).
# Iterating through a list
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
# Iterating through a string
for char in "Python":
print(char)
# Iterating through a range
for i in range(5): # 0, 1, 2, 3, 4
print(i)
# Iterating through a range with start and stop
for i in range(2, 8): # 2, 3, 4, 5, 6, 7
print(i)
# Iterating through a range with step
for i in range(1, 10, 2): # 1, 3, 5, 7, 9
print(i)
# Iterating through a dictionary
person = {"name": "John", "age": 30, "city": "New York"}
for key in person:
print(key, person[key])
# Alternatively, using items()
for key, value in person.items():
print(key, value)
while Loops
The while
loop executes a block of code as long as a condition is true:
count = 0
while count < 5:
print(count)
count += 1
break and continue
break
: Exits the loop completelycontinue
: Skips the current iteration and continues with the next one
# Using break
for i in range(10):
if i == 5:
break # Exit the loop when i is 5
print(i) # Prints 0, 1, 2, 3, 4
# Using continue
for i in range(10):
if i % 2 == 0:
continue # Skip even numbers
print(i) # Prints 1, 3, 5, 7, 9
else Clause in Loops
Python allows an else
clause after loops, which executes when the loop completes normally (without a break
):
# Loop completes normally, else block executes
for i in range(5):
print(i)
else:
print("Loop completed successfully")
# Loop exits with break, else block does not execute
for i in range(5):
if i == 3:
break
print(i)
else:
print("This won't be printed")
List Comprehensions
List comprehensions provide a concise way to create lists based on existing sequences:
# Without list comprehension
squares = []
for i in range(10):
squares.append(i ** 2)
# With list comprehension
squares = [i ** 2 for i in range(10)]
# List comprehension with condition
even_squares = [i ** 2 for i in range(10) if i % 2 == 0]
Similar comprehensions exist for dictionaries and sets:
# Dictionary comprehension
square_dict = {i: i ** 2 for i in range(5)} # {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
# Set comprehension
square_set = {i ** 2 for i in range(5)} # {0, 1, 4, 9, 16}
Functions
Functions are blocks of reusable code that perform a specific task. They help in organizing code, making it more readable and maintainable.
Defining Functions
The basic syntax for defining a function in Python:
def function_name(parameters):
"""Docstring: A brief description of what the function does."""
# Function body - code to be executed
return value # Optional return statement
Example:
def greet(name):
"""Return a greeting message for the given name."""
return f"Hello, {name}!"
# Calling the function
message = greet("Alice")
print(message) # "Hello, Alice!"
Function Parameters and Arguments
Parameters are variables listed in the function definition. Arguments are the values passed to the function when it is called.
Positional Arguments
Arguments are matched to parameters in order:
def describe_pet(animal_type, pet_name):
print(f"I have a {animal_type} named {pet_name}.")
describe_pet("dog", "Rex") # I have a dog named Rex.
Keyword Arguments
You can specify which parameter each argument corresponds to:
describe_pet(pet_name="Rex", animal_type="dog") # I have a dog named Rex.
Default Parameters
You can set default values for parameters:
def describe_pet(pet_name, animal_type="dog"):
print(f"I have a {animal_type} named {pet_name}.")
describe_pet("Rex") # I have a dog named Rex.
describe_pet("Whiskers", "cat") # I have a cat named Whiskers.
Variable-Length Arguments
To accept an arbitrary number of arguments:
# *args - Arbitrary positional arguments (as a tuple)
def add_numbers(*numbers):
return sum(numbers)
print(add_numbers(1, 2, 3, 4)) # 10
# **kwargs - Arbitrary keyword arguments (as a dictionary)
def build_profile(first, last, **user_info):
profile = {"first_name": first, "last_name": last}
profile.update(user_info)
return profile
user = build_profile("John", "Doe", age=30, occupation="Developer")
Return Values
Functions can return values using the return
statement:
def square(number):
return number ** 2
result = square(5) # 25
A function can return multiple values (technically, it returns a single tuple, but Python allows unpacking):
def get_dimensions():
return 500, 300 # Returns a tuple (500, 300)
width, height = get_dimensions() # Unpacking the tuple
If a function doesn't explicitly return a value, it implicitly returns None
.
Variable Scope
The scope of a variable determines where in your code the variable is accessible:
- Local scope: Variables defined within a function are only accessible within that function.
- Global scope: Variables defined outside any function are accessible throughout the file.
x = 10 # Global variable
def some_function():
y = 5 # Local variable
print(x) # Can access global variable
print(y) # Can access local variable
some_function()
print(x) # Can access global variable
# print(y) # Error: y is not defined in this scope
To modify a global variable from within a function, use the global
keyword:
count = 0
def increment():
global count
count += 1
increment()
print(count) # 1
Lambda Functions
Lambda functions are small, anonymous functions defined with the lambda
keyword:
# Regular function
def square(x):
return x ** 2
# Equivalent lambda function
square = lambda x: x ** 2
# Lambda functions are often used with functions like map, filter, and sort
numbers = [1, 5, 3, 9, 2]
squared = list(map(lambda x: x ** 2, numbers)) # [1, 25, 9, 81, 4]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) # [2]
sorted_by_last_digit = sorted(numbers, key=lambda x: x % 10) # [1, 2, 3, 5, 9]
Data Structures in Depth
Let's explore Python's core data structures in more detail.
Lists in Depth
Lists are versatile and one of the most commonly used data structures in Python.
List Methods
Method | Description | Example |
---|---|---|
append(x) |
Add item to the end | fruits.append("kiwi") |
extend(iterable) |
Add all items from iterable | fruits.extend(["kiwi", "mango"]) |
insert(i, x) |
Insert item at position i | fruits.insert(1, "pear") |
remove(x) |
Remove first occurrence of item | fruits.remove("apple") |
pop([i]) |
Remove and return item at position i (default: last) | last = fruits.pop() |
clear() |
Remove all items | fruits.clear() |
index(x) |
Return index of first occurrence of item | idx = fruits.index("apple") |
count(x) |
Count occurrences of item | n = fruits.count("apple") |
sort() |
Sort items in-place | fruits.sort() |
reverse() |
Reverse items in-place | fruits.reverse() |
copy() |
Return a shallow copy | new_list = fruits.copy() |
List Slicing
Slicing allows you to access a subset of a list:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Basic slicing: list[start:stop:step]
first_three = numbers[0:3] # [0, 1, 2]
middle = numbers[3:7] # [3, 4, 5, 6]
# Omitting indices
from_beginning = numbers[:5] # [0, 1, 2, 3, 4]
to_end = numbers[5:] # [5, 6, 7, 8, 9]
all_items = numbers[:] # Creates a shallow copy
# Negative indices (count from the end)
last_three = numbers[-3:] # [7, 8, 9]
except_last_two = numbers[:-2] # [0, 1, 2, 3, 4, 5, 6, 7]
# Step
even_numbers = numbers[::2] # [0, 2, 4, 6, 8]
odd_numbers = numbers[1::2] # [1, 3, 5, 7, 9]
reversed_list = numbers[::-1] # [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
Copying Lists
There are several ways to copy a list:
original = [1, 2, 3, [4, 5]]
# Shallow copies (nested objects share references)
copy1 = original.copy()
copy2 = list(original)
copy3 = original[:]
# Deep copy (completely independent)
import copy
deep_copy = copy.deepcopy(original)
Shallow copies work fine for lists with simple values, but if your list contains mutable objects (like other lists), you might need a deep copy.
Tuples in Depth
Tuples are similar to lists but immutable (cannot be changed after creation).
# Creating tuples
empty_tuple = ()
singleton = (1,) # Note the comma
coordinates = (10, 20)
mixed = (1, "hello", True)
# Tuple packing and unpacking
point = 10, 20, 30 # Packing
x, y, z = point # Unpacking
# Swapping variables using tuple packing/unpacking
a, b = 1, 2
a, b = b, a # Now a is 2 and b is 1
Why use tuples?
- Immutability: When you want to ensure data doesn't change
- Slightly faster: Tuples are slightly more efficient than lists
- Dictionary keys: Tuples can be used as dictionary keys, lists cannot
- Return multiple values: Functions often return tuples to return multiple values
Dictionaries in Depth
Dictionaries store key-value pairs and provide fast lookup by key.
Dictionary Methods
Method | Description | Example |
---|---|---|
get(key[, default]) |
Return value for key, or default if key not found | age = person.get("age", 0) |
keys() |
Return a view of all keys | keys = person.keys() |
values() |
Return a view of all values | values = person.values() |
items() |
Return a view of all key-value pairs | items = person.items() |
update(d) |
Update with key-value pairs from d | person.update({"age": 31, "job": "Engineer"}) |
pop(key[, default]) |
Remove and return value for key | age = person.pop("age") |
popitem() |
Remove and return an arbitrary key-value pair | item = person.popitem() |
clear() |
Remove all items | person.clear() |
setdefault(key[, default]) |
Return value for key, or set and return default if key not found | name = person.setdefault("name", "Unknown") |
Dictionary Comprehension
Create dictionaries with a concise syntax:
squares = {x: x**2 for x in range(6)} # {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
# With condition
even_squares = {x: x**2 for x in range(6) if x % 2 == 0} # {0: 0, 2: 4, 4: 16}
# From another dictionary
prices = {"apple": 0.5, "banana": 0.3, "orange": 0.6}
doubled_prices = {k: v * 2 for k, v in prices.items()}
Nested Dictionaries
Dictionaries can contain other dictionaries:
users = {
"john": {
"age": 30,
"email": "john@example.com",
"location": "New York"
},
"mary": {
"age": 25,
"email": "mary@example.com",
"location": "Boston"
}
}
# Accessing nested values
print(users["john"]["email"]) # john@example.com
Sets in Depth
Sets are unordered collections of unique items, useful for membership testing and eliminating duplicates.
Set Operations
# Creating sets
fruits = {"apple", "banana", "orange"}
vegetables = {"carrot", "spinach", "broccoli"}
citrus = {"orange", "lemon", "grapefruit"}
# Union: items in either set
all_produce = fruits | vegetables # or fruits.union(vegetables)
# Intersection: items in both sets
common = fruits & citrus # or fruits.intersection(citrus)
# Difference: items in first set but not in second
non_citrus_fruits = fruits - citrus # or fruits.difference(citrus)
# Symmetric difference: items in either set but not in both
exclusive = fruits ^ citrus # or fruits.symmetric_difference(citrus)
Set Methods
Method | Description | Example |
---|---|---|
add(elem) |
Add element to the set | fruits.add("kiwi") |
remove(elem) |
Remove element, raises error if not found | fruits.remove("apple") |
discard(elem) |
Remove element if present, no error if not found | fruits.discard("grape") |
pop() |
Remove and return an arbitrary element | item = fruits.pop() |
clear() |
Remove all elements | fruits.clear() |
update(iterable) |
Add elements from iterable | fruits.update(["kiwi", "mango"]) |
union(other_set) |
Return union of sets | all_produce = fruits.union(vegetables) |
intersection(other_set) |
Return intersection of sets | common = fruits.intersection(citrus) |
difference(other_set) |
Return difference of sets | non_citrus = fruits.difference(citrus) |
symmetric_difference(other_set) |
Return symmetric difference | exclusive = fruits.symmetric_difference(citrus) |
issubset(other_set) |
Test if this set is a subset of other_set | is_subset = fruits.issubset(all_produce) |
issuperset(other_set) |
Test if this set is a superset of other_set | is_superset = all_produce.issuperset(fruits) |
isdisjoint(other_set) |
Test if sets have no elements in common | no_common = fruits.isdisjoint(vegetables) |
Set Comprehensions
# Set of squares of numbers from 0 to 5
squares = {x**2 for x in range(6)} # {0, 1, 4, 9, 16, 25}
# Set of even squares
even_squares = {x**2 for x in range(6) if x % 2 == 0} # {0, 4, 16}
Working with Files
File operations are essential for saving data, reading configuration, processing data files, and more.
Opening and Closing Files
The standard way to open files in Python is with the open()
function:
# Basic syntax
file = open("filename.txt", "mode")
# Operations on the file
file.close() # Don't forget to close!
Common file modes:
'r'
: Read (default)'w'
: Write (creates a new file or truncates an existing one)'a'
: Append (adds to the end of an existing file)'b'
: Binary mode (e.g., 'rb' for reading binary)'t'
: Text mode (default)'+'
: Read and write (e.g., 'r+')
A safer way to open files is using the with
statement, which automatically closes the file:
with open("filename.txt", "r") as file:
# Operations on the file
contents = file.read()
# File is automatically closed when the block ends
Reading from Files
There are several ways to read from a file:
# Read the entire file as a string
with open("filename.txt", "r") as file:
contents = file.read()
# Read line by line
with open("filename.txt", "r") as file:
for line in file:
print(line.strip()) # strip() removes the newline character
# Read all lines into a list
with open("filename.txt", "r") as file:
lines = file.readlines()
# Read a specific number of characters
with open("filename.txt", "r") as file:
chunk = file.read(100) # Read the first 100 characters
Writing to Files
Writing to files is straightforward:
# Write a string to a file (overwrites existing content)
with open("output.txt", "w") as file:
file.write("Hello, World!\n")
file.write("This is a new line.")
# Append to a file
with open("output.txt", "a") as file:
file.write("\nThis line is appended.")
# Write multiple lines at once
lines = ["Line 1", "Line 2", "Line 3"]
with open("output.txt", "w") as file:
file.writelines(line + "\n" for line in lines)
Working with CSV Files
CSV (Comma-Separated Values) is a popular format for tabular data. Python's csv
module makes it easy to work with CSV files:
import csv
# Reading a CSV file
with open("data.csv", "r", newline="") as file:
reader = csv.reader(file)
header = next(reader) # Skip the header row
for row in reader:
print(row) # Each row is a list
# Reading a CSV into a dictionary
with open("data.csv", "r", newline="") as file:
reader = csv.DictReader(file)
for row in reader:
print(row) # Each row is a dictionary with column names as keys
# Writing a CSV file
data = [
["Name", "Age", "City"],
["John", 30, "New York"],
["Alice", 25, "Boston"],
["Bob", 35, "Chicago"]
]
with open("output.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerows(data)
# Writing a CSV from dictionaries
data = [
{"Name": "John", "Age": 30, "City": "New York"},
{"Name": "Alice", "Age": 25, "City": "Boston"},
{"Name": "Bob", "Age": 35, "City": "Chicago"}
]
with open("output.csv", "w", newline="") as file:
fieldnames = ["Name", "Age", "City"]
writer = csv.DictWriter(file, fieldnames=fieldnames)
writer.writeheader()
writer.writerows(data)
Working with JSON Files
JSON (JavaScript Object Notation) is a lightweight data interchange format. Python's json
module provides methods to encode and decode JSON:
import json
# Python object to be serialized
data = {
"name": "John",
"age": 30,
"city": "New York",
"languages": ["Python", "JavaScript", "SQL"],
"is_employee": True,
"salary": None
}
# Writing JSON to a file
with open("data.json", "w") as file:
json.dump(data, file, indent=4) # indent for pretty formatting
# Converting Python object to JSON string
json_string = json.dumps(data, indent=4)
print(json_string)
# Reading JSON from a file
with open("data.json", "r") as file:
loaded_data = json.load(file)
# Converting JSON string to Python object
python_object = json.loads(json_string)
Error Handling
Errors are a normal part of software development. Python provides mechanisms to handle errors gracefully and prevent program crashes.
Try and Except Blocks
The basic syntax for exception handling:
try:
# Code that might raise an exception
result = 10 / 0
except:
# Code that runs if an exception occurs
print("An error occurred")
It's better to catch specific exceptions:
try:
num = int(input("Enter a number: "))
result = 10 / num
except ValueError:
print("Invalid input. Please enter a number.")
except ZeroDivisionError:
print("Cannot divide by zero.")
Handling Multiple Exceptions
You can handle multiple exceptions in different ways:
# Multiple except blocks
try:
# ...code...
except ValueError:
# Handle ValueError
except ZeroDivisionError:
# Handle ZeroDivisionError
# Grouping exceptions
try:
# ...code...
except (ValueError, ZeroDivisionError):
# Handle both ValueError and ZeroDivisionError
# Capturing the exception object
try:
# ...code...
except ValueError as e:
print(f"ValueError occurred: {e}")
The Finally Clause
The finally
block executes regardless of whether an exception occurred:
try:
file = open("file.txt", "r")
# Operations on the file
except FileNotFoundError:
print("File not found")
finally:
file.close() # This runs even if an exception occurred
Note: When using with
for file operations, you don't need finally
to close the file, as it's handled automatically.
The Else Clause
The else
block executes if no exceptions were raised:
try:
num = int(input("Enter a number: "))
except ValueError:
print("That's not a valid number!")
else:
print(f"You entered: {num}")
# More operations with num
Raising Exceptions
You can raise exceptions explicitly using the raise
statement:
def divide(a, b):
if b == 0:
raise ZeroDivisionError("Cannot divide by zero")
return a / b
try:
result = divide(10, 0)
except ZeroDivisionError as e:
print(e) # "Cannot divide by zero"
Creating Custom Exceptions
You can define your own exception classes by inheriting from existing exception classes:
class InsufficientFundsError(Exception):
"""Raised when a withdrawal would result in a negative balance."""
def __init__(self, balance, amount):
self.balance = balance
self.amount = amount
self.deficit = amount - balance
message = f"Cannot withdraw ${amount}. Balance is ${balance}, resulting in a deficit of ${self.deficit}."
super().__init__(message)
def withdraw(balance, amount):
if amount > balance:
raise InsufficientFundsError(balance, amount)
return balance - amount
try:
new_balance = withdraw(100, 150)
except InsufficientFundsError as e:
print(e)
print(f"You need ${e.deficit} more to complete this withdrawal.")
Modules and Packages
Modules and packages help organize and reuse code across different programs.
Importing Modules
A module is a file containing Python code. To use a module, you import it:
# Import the entire module
import math
print(math.sqrt(16)) # 4.0
# Import specific items from a module
from math import sqrt, pi
print(sqrt(16)) # 4.0
print(pi) # 3.141592653589793
# Import with an alias
import numpy as np
arr = np.array([1, 2, 3])
# Import all items from a module (not recommended)
from math import *
print(sqrt(16)) # 4.0
Creating Your Own Modules
Any Python file can be a module. For example, if you have a file named mymodule.py
:
# mymodule.py
def greeting(name):
return f"Hello, {name}!"
PI = 3.14159
You can import and use it in another file:
# main.py
import mymodule
print(mymodule.greeting("Alice")) # "Hello, Alice!"
print(mymodule.PI) # 3.14159
Python's Standard Library
Python comes with a comprehensive standard library. Here are some commonly used modules:
Module | Description | Example Use |
---|---|---|
math |
Mathematical functions | math.sqrt(16) |
random |
Random number generation | random.randint(1, 10) |
datetime |
Date and time manipulation | datetime.datetime.now() |
os |
Operating system interface | os.listdir(".") |
sys |
System-specific parameters and functions | sys.argv |
re |
Regular expressions | re.search(r'\d+', text) |
json |
JSON encoding and decoding | json.dumps(data) |
csv |
CSV file reading and writing | csv.reader(file) |
collections |
Specialized container datatypes | collections.Counter(items) |
itertools |
Functions for efficient iteration | itertools.permutations([1, 2, 3]) |
pathlib |
Object-oriented filesystem paths | pathlib.Path.home() |
statistics |
Mathematical statistics functions | statistics.mean([1, 2, 3]) |
urllib |
URL handling modules | urllib.request.urlopen(url) |
socket |
Low-level networking interface | socket.socket() |
email |
Email message handling | email.message.EmailMessage() |
Installing Packages with pip
Python's package manager, pip
, allows you to install third-party packages:
# Basic installation
pip install package_name
# Install a specific version
pip install package_name==1.0.0
# Upgrade a package
pip install --upgrade package_name
# Install multiple packages from a file
pip install -r requirements.txt
Common packages to know:
- NumPy: For numerical computing
- Pandas: For data analysis and manipulation
- Matplotlib: For data visualization
- Requests: For HTTP requests
- Flask/Django: For web development
- Scikit-learn: For machine learning
- TensorFlow/PyTorch: For deep learning
- Beautiful Soup: For web scraping
- SQLAlchemy: For database interactions
- Pillow: For image processing
Virtual Environments
Virtual environments allow you to create isolated Python environments for different projects, avoiding package conflicts:
# Create a virtual environment
python -m venv myenv
# Activate the virtual environment
# On Windows:
myenv\Scripts\activate
# On Unix or MacOS:
source myenv/bin/activate
# Deactivate when done
deactivate
Using virtualenv
(an alternative to venv
):
# Install virtualenv
pip install virtualenv
# Create a virtual environment
virtualenv myenv
# Activate as above
Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of "objects," which can contain data and code. Python is a multi-paradigm language that supports OOP.
Classes and Objects
A class is a blueprint for creating objects:
class Dog:
# Class attribute (shared by all instances)
species = "Canis familiaris"
# Initializer (constructor)
def __init__(self, name, age):
# Instance attributes (unique to each instance)
self.name = name
self.age = age
# Instance method
def bark(self):
return f"{self.name} says Woof!"
def __str__(self):
return f"{self.name}, {self.age} years old"
# Creating instances of the class
buddy = Dog("Buddy", 9)
miles = Dog("Miles", 4)
# Accessing attributes
print(buddy.name) # "Buddy"
print(buddy.species) # "Canis familiaris"
# Calling methods
print(buddy.bark()) # "Buddy says Woof!"
# String representation
print(buddy) # "Buddy, 9 years old"
Inheritance
Inheritance allows a class to inherit attributes and methods from another class:
class Pet:
def __init__(self, name, age):
self.name = name
self.age = age
def show(self):
print(f"I am {self.name} and I am {self.age} years old")
class Cat(Pet): # Cat inherits from Pet
def __init__(self, name, age, color):
# Call the parent class's __init__ method
super().__init__(name, age)
self.color = color
def speak(self):
return "Meow!"
# Override the parent class's method
def show(self):
print(f"I am {self.name}, a {self.color} cat and I am {self.age} years old")
class Dog(Pet): # Dog inherits from Pet
def speak(self):
return "Woof!"
# Creating instances
fluffy = Cat("Fluffy", 3, "white")
buddy = Dog("Buddy", 9)
# Calling methods
fluffy.show() # "I am Fluffy, a white cat and I am 3 years old"
print(buddy.speak()) # "Woof!"
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass:
def pet_speak(pet):
print(pet.speak())
pet_speak(fluffy) # "Meow!"
pet_speak(buddy) # "Woof!"
Encapsulation
Encapsulation is the bundling of data and methods that operate on that data:
class BankAccount:
def __init__(self, owner, balance=0):
self.owner = owner
self._balance = balance # Protected attribute (convention)
def deposit(self, amount):
if amount > 0:
self._balance += amount
return True
return False
def withdraw(self, amount):
if 0 < amount <= self._balance:
self._balance -= amount
return True
return False
def get_balance(self):
return self._balance
# Creating an account
account = BankAccount("John", 1000)
# Using methods to interact with protected attributes
account.deposit(500)
account.withdraw(200)
print(account.get_balance()) # 1300
Python doesn't have true private attributes, but a convention is to use a single underscore (_
) for protected attributes and double underscore (__
) for name mangling (which helps to avoid name conflicts in subclasses).
Special Methods (Magic Methods)
Special methods, also called magic methods, allow you to define how objects of your class behave with Python's built-in operations:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __sub__(self, other):
return Vector(self.x - other.x, self.y - other.y)
def __mul__(self, scalar):
return Vector(self.x * scalar, self.y * scalar)
def __eq__(self, other):
return self.x == other.x and self.y == other.y
def __repr__(self):
return f"Vector({self.x}, {self.y})"
# Using the special methods
v1 = Vector(2, 3)
v2 = Vector(3, 4)
v3 = v1 + v2 # Vector(5, 7)
v4 = v1 * 2 # Vector(4, 6)
print(v1 == Vector(2, 3)) # True
print(repr(v1)) # "Vector(2, 3)"
Common magic methods include:
__init__
: Constructor__str__
: String representation (for humans)__repr__
: String representation (for developers)__len__
: Length (forlen()
function)__getitem__
: Index access (forobj[key]
)__setitem__
: Index assignment (forobj[key] = value
)__eq__
,__lt__
, etc.: Comparison operators__add__
,__sub__
, etc.: Arithmetic operators
Working with External Libraries
Python's ecosystem includes thousands of libraries that extend its functionality. Let's explore some popular ones.
NumPy for Numerical Computing
NumPy provides support for large, multi-dimensional arrays and matrices, along with mathematical functions to operate on them:
import numpy as np
# Creating arrays
arr1 = np.array([1, 2, 3, 4, 5])
arr2 = np.zeros((3, 3)) # 3x3 array of zeros
arr3 = np.ones((2, 2)) # 2x2 array of ones
arr4 = np.linspace(0, 10, 5) # 5 evenly spaced values from 0 to 10
arr5 = np.random.rand(3, 3) # 3x3 array of random values
# Array operations
print(arr1 + 5) # Element-wise addition: [6, 7, 8, 9, 10]
print(arr1 * 2) # Element-wise multiplication: [2, 4, 6, 8, 10]
print(arr1 ** 2) # Element-wise power: [1, 4, 9, 16, 25]
# Matrix operations
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
print(matrix1 + matrix2) # Element-wise addition
print(matrix1 * matrix2) # Element-wise multiplication
print(np.dot(matrix1, matrix2)) # Matrix multiplication
print(matrix1.T) # Transpose
# Array indexing and slicing
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(arr[0, 1]) # 2 (element at row 0, column 1)
print(arr[:, 1]) # [2, 5, 8] (all elements in column 1)
print(arr[1:3, :]) # Rows 1 and 2, all columns
# Statistical functions
print(np.mean(arr)) # Mean of all elements
print(np.max(arr)) # Maximum value
print(np.min(arr)) # Minimum value
print(np.std(arr)) # Standard deviation
Pandas for Data Analysis
Pandas provides high-performance, easy-to-use data structures and data analysis tools:
import pandas as pd
import numpy as np
# Creating DataFrames
# From a dictionary
data = {
'Name': ['John', 'Alice', 'Bob'],
'Age': [28, 24, 32],
'City': ['New York', 'Boston', 'Chicago']
}
df1 = pd.DataFrame(data)
# From a list of dictionaries
records = [
{'Name': 'John', 'Age': 28, 'City': 'New York'},
{'Name': 'Alice', 'Age': 24, 'City': 'Boston'},
{'Name': 'Bob', 'Age': 32, 'City': 'Chicago'}
]
df2 = pd.DataFrame(records)
# From a CSV file
# df3 = pd.read_csv('data.csv')
# Basic DataFrame operations
print(df1.head()) # First 5 rows
print(df1.info()) # Summary information
print(df1.describe()) # Statistical summary
# Accessing data
print(df1['Name']) # Access a column
print(df1.loc[0]) # Access a row by label
print(df1.iloc[0]) # Access a row by position
print(df1.loc[df1['Age'] > 25]) # Filtering rows
# Data manipulation
df1['Salary'] = [50000, 60000, 70000] # Add a new column
df1.drop('Salary', axis=1, inplace=True) # Drop a column
df4 = df1.sort_values('Age') # Sort by age
df5 = df1.groupby('City').mean() # Group by city and calculate mean
# Handling missing values
df6 = pd.DataFrame({
'A': [1, 2, np.nan, 4],
'B': [5, np.nan, np.nan, 8],
'C': [9, 10, 11, 12]
})
print(df6.isnull()) # Check for missing values
print(df6.fillna(0)) # Fill missing values with 0
print(df6.dropna()) # Drop rows with any missing values
# Data export
# df1.to_csv('output.csv', index=False)
# df1.to_excel('output.xlsx', index=False)
# df1.to_json('output.json', orient='records')
Matplotlib for Data Visualization
Matplotlib is a comprehensive library for creating static, interactive, and animated visualizations:
import matplotlib.pyplot as plt
import numpy as np
# Basic line plot
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure(figsize=(8, 4))
plt.plot(x, y, label='sin(x)')
plt.title('Sine Wave')
plt.xlabel('x')
plt.ylabel('sin(x)')
plt.legend()
plt.grid(True)
# plt.savefig('sine_wave.png')
plt.show()
# Multiple plots
plt.figure(figsize=(10, 6))
plt.plot(x, np.sin(x), 'b-', label='sin(x)')
plt.plot(x, np.cos(x), 'r--', label='cos(x)')
plt.title('Sine and Cosine Waves')
plt.xlabel('x')
plt.ylabel('y')
plt.legend()
plt.grid(True)
plt.show()
# Subplots
fig, axs = plt.subplots(2, 1, figsize=(8, 8))
axs[0].plot(x, np.sin(x), 'b-')
axs[0].set_title('Sine Wave')
axs[1].plot(x, np.cos(x), 'r--')
axs[1].set_title('Cosine Wave')
for ax in axs:
ax.set_xlabel('x')
ax.set_ylabel('y')
ax.grid(True)
plt.tight_layout()
plt.show()
# Bar chart
categories = ['A', 'B', 'C', 'D', 'E']
values = [25, 40, 30, 55, 15]
plt.figure(figsize=(8, 4))
plt.bar(categories, values, color='skyblue')
plt.title('Bar Chart')
plt.xlabel('Category')
plt.ylabel('Value')
plt.show()
# Scatter plot
x = np.random.rand(50)
y = np.random.rand(50)
colors = np.random.rand(50)
sizes = 1000 * np.random.rand(50)
plt.figure(figsize=(8, 6))
plt.scatter(x, y, c=colors, s=sizes, alpha=0.6)
plt.title('Scatter Plot')
plt.xlabel('x')
plt.ylabel('y')
plt.colorbar()
plt.show()
# Histogram
data = np.random.randn(1000)
plt.figure(figsize=(8, 4))
plt.hist(data, bins=30, color='skyblue', edgecolor='black')
plt.title('Histogram')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
# Pie chart
labels = ['A', 'B', 'C', 'D', 'E']
sizes = [15, 30, 25, 10, 20]
explode = (0, 0.1, 0, 0, 0) # explode the 2nd slice
plt.figure(figsize=(8, 8))
plt.pie(sizes, explode=explode, labels=labels, autopct='%1.1f%%',
shadow=True, startangle=90)
plt.axis('equal') # Equal aspect ratio ensures that pie is drawn as a circle
plt.title('Pie Chart')
plt.show()
Requests for HTTP Requests
The Requests library simplifies making HTTP requests:
import requests
# GET request
response = requests.get('https://api.github.com/users/python')
print(response.status_code) # 200 if successful
print(response.headers['content-type'])
data = response.json() # Parse JSON response
print(data['login']) # 'python'
# GET with parameters
params = {'q': 'python', 'sort': 'stars'}
response = requests.get('https://api.github.com/search/repositories', params=params)
search_results = response.json()
for repo in search_results['items'][:5]:
print(f"{repo['name']}: {repo['html_url']}")
# POST request
data = {'username': 'user', 'password': 'pass'}
response = requests.post('https://httpbin.org/post', data=data)
print(response.json()['form']) # {'username': 'user', 'password': 'pass'}
# Custom headers
headers = {'User-Agent': 'My Python App'}
response = requests.get('https://api.github.com/users/python', headers=headers)
# Session for multiple requests
session = requests.Session()
session.headers.update({'User-Agent': 'My Python App'})
response = session.get('https://api.github.com/users/python')
# Multiple requests will now use the same session
# Timeout and error handling
try:
response = requests.get('https://api.github.com/users/python', timeout=3)
response.raise_for_status() # Raise an exception for 4XX/5XX responses
except requests.exceptions.Timeout:
print("Request timed out")
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
except requests.exceptions.RequestException as err:
print(f"An error occurred: {err}")
Practical Python Projects
Let's explore some simple projects to apply what we've learned.
Building a Simple Calculator
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Cannot divide by zero"
return x / y
def calculator():
print("Simple Calculator")
print("Operations:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
print("5. Exit")
while True:
choice = input("Enter choice (1-5): ")
if choice == '5':
print("Exiting calculator...")
break
if choice not in ('1', '2', '3', '4'):
print("Invalid input. Please try again.")
continue
try:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
except ValueError:
print("Invalid input. Please enter a number.")
continue
if choice == '1':
print(f"{num1} + {num2} = {add(num1, num2)}")
elif choice == '2':
print(f"{num1} - {num2} = {subtract(num1, num2)}")
elif choice == '3':
print(f"{num1} * {num2} = {multiply(num1, num2)}")
elif choice == '4':
result = divide(num1, num2)
print(f"{num1} / {num2} = {result}")
print() # Empty line for readability
if __name__ == "__main__":
calculator()
Creating a To-Do List Application
class TodoList:
def __init__(self):
self.tasks = []
def add_task(self, task):
self.tasks.append({"task": task, "completed": False})
print(f"Task '{task}' added successfully.")
def view_tasks(self):
if not self.tasks:
print("No tasks in the list.")
return
print("\nTasks:")
for i, task_obj in enumerate(self.tasks, 1):
status = "✓" if task_obj["completed"] else " "
print(f"{i}. [{status}] {task_obj['task']}")
def mark_completed(self, task_index):
if 1 <= task_index <= len(self.tasks):
self.tasks[task_index - 1]["completed"] = True
print(f"Task '{self.tasks[task_index - 1]['task']}' marked as completed.")
else:
print("Invalid task index.")
def delete_task(self, task_index):
if 1 <= task_index <= len(self.tasks):
deleted_task = self.tasks.pop(task_index - 1)
print(f"Task '{deleted_task['task']}' deleted successfully.")
else:
print("Invalid task index.")
def save_to_file(self, filename="todo.txt"):
with open(filename, "w") as file:
for task_obj in self.tasks:
status = "completed" if task_obj["completed"] else "pending"
file.write(f"{task_obj['task']},{status}\n")
print(f"Tasks saved to {filename}")
def load_from_file(self, filename="todo.txt"):
try:
with open(filename, "r") as file:
self.tasks = []
for line in file:
task, status = line.strip().split(",")
self.tasks.append({"task": task, "completed": status == "completed"})
print(f"Tasks loaded from {filename}")
except FileNotFoundError:
print(f"File {filename} not found.")
def todo_app():
todo_list = TodoList()
print("To-Do List Application")
while True:
print("\nOptions:")
print("1. Add Task")
print("2. View Tasks")
print("3. Mark Task as Completed")
print("4. Delete Task")
print("5. Save Tasks to File")
print("6. Load Tasks from File")
print("7. Exit")
choice = input("Enter choice (1-7): ")
if choice == '1':
task = input("Enter the task: ")
todo_list.add_task(task)
elif choice == '2':
todo_list.view_tasks()
elif choice == '3':
todo_list.view_tasks()
try:
task_index = int(input("Enter task number to mark as completed: "))
todo_list.mark_completed(task_index)
except ValueError:
print("Please enter a valid number.")
elif choice == '4':
todo_list.view_tasks()
try:
task_index = int(input("Enter task number to delete: "))
todo_list.delete_task(task_index)
except ValueError:
print("Please enter a valid number.")
elif choice == '5':
filename = input("Enter filename to save (default: todo.txt): ") or "todo.txt"
todo_list.save_to_file(filename)
elif choice == '6':
filename = input("Enter filename to load (default: todo.txt): ") or "todo.txt"
todo_list.load_from_file(filename)
elif choice == '7':
print("Exiting application...")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
todo_app()
Web Scraping Basics
Web scraping is the process of extracting data from websites. Python's requests
and BeautifulSoup
libraries make this relatively easy:
import requests
from bs4 import BeautifulSoup
def scrape_quotes():
# Send a GET request to the quotes website
url = "http://quotes.toscrape.com/"
response = requests.get(url)
# Check if the request was successful
if response.status_code != 200:
print(f"Failed to fetch the page. Status code: {response.status_code}")
return
# Parse the HTML content
soup = BeautifulSoup(response.text, 'html.parser')
# Find all quote elements
quote_elements = soup.find_all('div', class_='quote')
# Extract and print each quote with its author
for quote_elem in quote_elements:
# Get the text of the quote
text = quote_elem.find('span', class_='text').get_text()
# Get the author
author = quote_elem.find('small', class_='author').get_text()
# Get the tags
tags = [tag.get_text() for tag in quote_elem.find_all('a', class_='tag')]
# Print the information
print(f"Quote: {text}")
print(f"Author: {author}")
print(f"Tags: {', '.join(tags)}")
print("-" * 50)
if __name__ == "__main__":
scrape_quotes()
Working with APIs
APIs (Application Programming Interfaces) allow your code to interact with external services:
import requests
import json
from datetime import datetime
def weather_app():
api_key = "YOUR_API_KEY" # Replace with your actual API key
base_url = "http://api.openweathermap.org/data/2.5/weather"
city = input("Enter city name: ")
# Request parameters
params = {
"q": city,
"appid": api_key,
"units": "metric" # Use metric units (Celsius)
}
try:
# Make the API request
response = requests.get(base_url, params=params)
response.raise_for_status() # Raise an exception for 4XX/5XX responses
# Parse the JSON response
weather_data = response.json()
# Extract relevant information
temperature = weather_data["main"]["temp"]
feels_like = weather_data["main"]["feels_like"]
humidity = weather_data["main"]["humidity"]
wind_speed = weather_data["wind"]["speed"]
description = weather_data["weather"][0]["description"]
sunrise_time = datetime.fromtimestamp(weather_data["sys"]["sunrise"]).strftime('%H:%M:%S')
sunset_time = datetime.fromtimestamp(weather_data["sys"]["sunset"]).strftime('%H:%M:%S')
# Display the weather information
print(f"\nWeather in {city.title()}:")
print(f"Temperature: {temperature}°C")
print(f"Feels like: {feels_like}°C")
print(f"Humidity: {humidity}%")
print(f"Wind speed: {wind_speed} m/s")
print(f"Description: {description.capitalize()}")
print(f"Sunrise: {sunrise_time}")
print(f"Sunset: {sunset_time}")
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
if response.status_code == 404:
print(f"City '{city}' not found.")
elif response.status_code == 401:
print("Invalid API key.")
except requests.exceptions.RequestException as err:
print(f"An error occurred: {err}")
except (KeyError, json.JSONDecodeError):
print("Could not parse weather data.")
if __name__ == "__main__":
weather_app()
Python Best Practices
Writing clean, maintainable, and efficient code is just as important as making it work.
Code Style and PEP 8
PEP 8 is Python's style guide. Here are some key guidelines:
- Indentation: Use 4 spaces (not tabs)
- Line Length: Maximum of 79 characters
- Imports: One per line, grouped in the order of standard library, third-party, local application
- Whitespace:
- No extra whitespace within parentheses or brackets
- One space around operators
- No space before a comma, semicolon, or colon
- Naming Conventions:
- Functions, variables, methods:
lowercase_with_underscores
- Classes:
CamelCase
- Constants:
ALL_UPPERCASE
- Private attributes/methods:
_leading_underscore
- Functions, variables, methods:
- Comments: Start with a # and a space
- Docstrings: Use triple quotes (
"""
)
Tools like pylint
, flake8
, and black
can help you enforce PEP 8 guidelines.
Writing Clean and Readable Code
-
Be explicit:
# Bad def f(x): return x**2 # Good def square(number): return number ** 2
-
Avoid magic numbers:
# Bad if user.age < 18: ... # Good MINIMUM_AGE = 18 if user.age < MINIMUM_AGE: ...
-
Use descriptive names:
# Bad a = [] for i in range(10): a.append(i * 2) # Good doubled_numbers = [] for number in range(10): doubled_numbers.append(number * 2)
-
Keep functions small and focused:
- Each function should do one thing well
- If a function is getting too long, consider breaking it up
-
Use list/dictionary comprehensions appropriately:
# Instead of: squares = [] for i in range(10): squares.append(i ** 2) # Use: squares = [i ** 2 for i in range(10)]
-
Avoid deeply nested code:
- Extract complex conditions into helper functions
- Use early returns
Documentation
Good documentation makes your code more accessible to others (and to future you):
-
Docstrings: Add docstrings to modules, classes, and functions:
def calculate_area(radius): """ Calculate the area of a circle. Args: radius (float): The radius of the circle Returns: float: The area of the circle Raises: ValueError: If radius is negative """ if radius < 0: raise ValueError("Radius cannot be negative") return 3.14159 * radius ** 2
-
README.md: Include a README.md file in your project with:
- Project overview
- Installation instructions
- Usage examples
- API documentation
- License information
-
Comments: Use comments to explain "why" rather than "what":
# Bad comment x += 1 # Increment x # Good comment x += 1 # Adjust for zero-indexing
Testing Your Code
Testing helps ensure your code works as expected and continues to work after changes:
- Unit Tests: Test individual functions and methods
- Integration Tests: Test how different parts of your code work together
- Functional Tests: Test the system as a whole
Python's unittest
module is part of the standard library:
import unittest
# Code to test
def add(a, b):
return a + b
# Test class
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(1, 2), 3)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -2), -3)
def test_add_mixed_numbers(self):
self.assertEqual(add(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
pytest
is a popular alternative that offers a simpler syntax:
# File: test_functions.py
def test_add_positive_numbers():
from my_module import add
assert add(1, 2) == 3
def test_add_negative_numbers():
from my_module import add
assert add(-1, -2) == -3
Debugging Techniques
When your code doesn't work as expected:
-
Print statements: The simplest debugging method:
print(f"Variable x: {x}")
-
Logging: Better than print statements for production code:
import logging logging.basicConfig(level=logging.DEBUG) logging.debug(f"Variable x: {x}") logging.info("Process started") logging.warning("Resource nearly exhausted") logging.error("Failed to connect to database")
-
Python Debugger (pdb):
import pdb def complex_function(): x = 5 y = 0 pdb.set_trace() # Execution stops here for interactive debugging z = x / y # Error will occur here return z
-
IDE Debuggers: Most Python IDEs (like PyCharm, Visual Studio Code) have built-in debuggers that allow you to:
- Set breakpoints
- Step through code
- Inspect variables
- Evaluate expressions
-
Exception handling: Use try-except to identify where errors occur:
try: result = x / y except ZeroDivisionError as e: print(f"Error occurred: {e}") # Handle the error
Advanced Python Concepts
These concepts might be more complex, but understanding them can greatly enhance your Python skills.
Generators and Iterators
Iterators are objects that implement the iterator protocol, with __iter__()
and __next__()
methods:
class CountDown:
def __init__(self, start):
self.start = start
def __iter__(self):
return self
def __next__(self):
if self.start <= 0:
raise StopIteration
self.start -= 1
return self.start + 1
# Using the iterator
for i in CountDown(5):
print(i) # 5, 4, 3, 2, 1
Generators are a simpler way to create iterators using functions with the yield
statement:
def countdown(start):
while start > 0:
yield start
start -= 1
# Using the generator
for i in countdown(5):
print(i) # 5, 4, 3, 2, 1
Generator expressions are similar to list comprehensions but create generators:
# List comprehension (creates a list in memory)
squares_list = [x**2 for x in range(1000000)]
# Generator expression (creates a generator object)
squares_gen = (x**2 for x in range(1000000))
# The generator expression is more memory-efficient for large sequences
Decorators
Decorators modify the behavior of a function or class without changing its code:
def log_function_call(func):
def wrapper(*args, **kwargs):
print(f"Calling {func.__name__} with args: {args}, kwargs: {kwargs}")
result = func(*args, **kwargs)
print(f"{func.__name__} returned: {result}")
return result
return wrapper
@log_function_call
def add(a, b):
return a + b
# Equivalent to: add = log_function_call(add)
add(3, 5) # This call will be logged
Decorators with arguments:
def repeat(n):
def decorator(func):
def wrapper(*args, **kwargs):
result = None
for _ in range(n):
result = func(*args, **kwargs)
return result
return wrapper
return decorator
@repeat(3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Prints "Hello, Alice!" three times
Context Managers
Context managers allow setup and cleanup actions around a block of code. The with
statement is used to invoke them:
# Built-in context manager for files
with open("file.txt", "r") as file:
content = file.read()
# File is automatically closed when the block ends
# Creating a context manager using a class
class Timer:
def __enter__(self):
import time
self.start = time.time()
return self
def __exit__(self, exc_type, exc_val, exc_tb):
import time
self.end = time.time()
print(f"Elapsed time: {self.end - self.start:.6f} seconds")
with Timer():
# Code to be timed
sum(range(10000000))
# Creating a context manager using contextlib
from contextlib import contextmanager
@contextmanager
def timer():
import time
start = time.time()
try:
yield # This is where the with-block code executes
finally:
end = time.time()
print(f"Elapsed time: {end - start:.6f} seconds")
with timer():
# Code to be timed
sum(range(10000000))
Regular Expressions
Regular expressions are powerful tools for pattern matching and text manipulation:
import re
# Simple pattern matching
text = "The quick brown fox jumps over the lazy dog"
match = re.search(r"fox", text)
if match:
print(f"Found 'fox' at position: {match.start()}")
# Character classes
digits = re.findall(r"\d+", "There are 123 apples and 456 oranges")
print(digits) # ['123', '456']
# Splitting text
words = re.split(r"\W+", "Hello, world! How are you?")
print(words) # ['Hello', 'world', 'How', 'are', 'you', '']
# Replacing text
new_text = re.sub(r"fox", "cat", text)
print(new_text) # "The quick brown cat jumps over the lazy dog"
# Groups
pattern = r"(\w+)@(\w+)\.(\w+)"
match = re.search(pattern, "Contact me at john@example.com for details")
if match:
print(match.group(0)) # john@example.com (entire match)
print(match.group(1)) # john (first group)
print(match.group(2)) # example (second group)
print(match.group(3)) # com (third group)
# Named groups
pattern = r"(?P<username>\w+)@(?P<domain>\w+)\.(?P<tld>\w+)"
match = re.search(pattern, "Contact me at john@example.com for details")
if match:
print(match.groupdict()) # {'username': 'john', 'domain': 'example', 'tld': 'com'}
Threading and Multiprocessing
Python provides several ways to run code concurrently.
Threading is useful for I/O-bound tasks:
import threading
import time
def task(name, delay):
print(f"Task {name} started")
time.sleep(delay) # Simulate work
print(f"Task {name} completed")
# Create threads
thread1 = threading.Thread(target=task, args=("A", 2))
thread2 = threading.Thread(target=task, args=("B", 1))
# Start threads
start_time = time.time()
thread1.start()
thread2.start()
# Wait for threads to complete
thread1.join()
thread2.join()
print(f"All tasks completed in {time.time() - start_time:.2f} seconds")
Multiprocessing is better for CPU-bound tasks, as it bypasses the Global Interpreter Lock (GIL):
import multiprocessing
import time
def cpu_bound_task(number):
return sum(i * i for i in range(number))
if __name__ == "__main__":
numbers = [10000000, 20000000, 30000000, 40000000]
# Sequential execution
start_time = time.time()
results = [cpu_bound_task(number) for number in numbers]
print(f"Sequential execution took {time.time() - start_time:.2f} seconds")
# Parallel execution with multiprocessing
start_time = time.time()
with multiprocessing.Pool(processes=4) as pool:
results = pool.map(cpu_bound_task, numbers)
print(f"Parallel execution took {time.time() - start_time:.2f} seconds")
Resources for Further Learning
Websites and Tutorials
- Official Python Documentation: docs.python.org
- Real Python: realpython.com
- Python for Beginners: python.org/about/gettingstarted
- GeeksforGeeks - Python Programming Language: geeksforgeeks.org/python-programming-language
- W3Schools - Python Tutorial: w3schools.com/python
- Project Euler: projecteuler.net - Programming challenges
- Exercism.io: exercism.io/tracks/python - Coding exercises
- Programiz Python Tutorial: programiz.com/python-programming
- Tutorialspoint Python Tutorial: tutorialspoint.com/python
- PyNative: pynative.com - Python exercises and solutions
Communities and Forums
- Stack Overflow: stackoverflow.com/questions/tagged/python
- Reddit - r/Python: reddit.com/r/Python
- Reddit - r/learnpython: reddit.com/r/learnpython
- Python Discord: pythondiscord.com
- Python Forum: python-forum.io
- Python Community on DEV: dev.to/t/python
- Python.org Community: python.org/community
- PySlackers: pyslackers.com - Slack community
Conclusion
Python is a versatile, readable, and powerful programming language with applications across various domains. Its simplicity makes it an excellent choice for beginners, while its extensive ecosystem of libraries and frameworks satisfies the needs of professionals.
This guide has covered the fundamentals of Python programming, from basic syntax to advanced concepts. We've explored:
- Setting up your Python environment
- Core Python concepts like variables, data types, control flow, and functions
- Data structures including lists, tuples, dictionaries, and sets
- Working with files and handling errors
- Organizing code with modules and packages
- Object-oriented programming in Python
- Using external libraries for data analysis, visualization, HTTP requests, and more
- Building practical projects
- Best practices for writing clean, maintainable code
- Advanced concepts like generators, decorators, and regular expressions
As you continue your Python journey, remember these key principles:
- Practice regularly: Programming is a skill best learned by doing.
- Build projects: Apply what you've learned to real-world problems.
- Read other people's code: Learn from the Python community.
- Debug patiently: Debugging is a valuable skill that improves with experience.
- Stay updated: Python is evolving, so keep learning about new features.
The Python community is known for its helpfulness and inclusivity. Don't hesitate to ask questions, contribute to open-source projects, and share your knowledge with others.
Whether you're using Python for web development, data analysis, automation, scientific computing, or just for fun, the skills you've learned in this guide provide a solid foundation for your programming journey.
Happy coding!
Books: Dargslan Python Beginners Series
-1- : Python Variables and Data Types for Beginners:
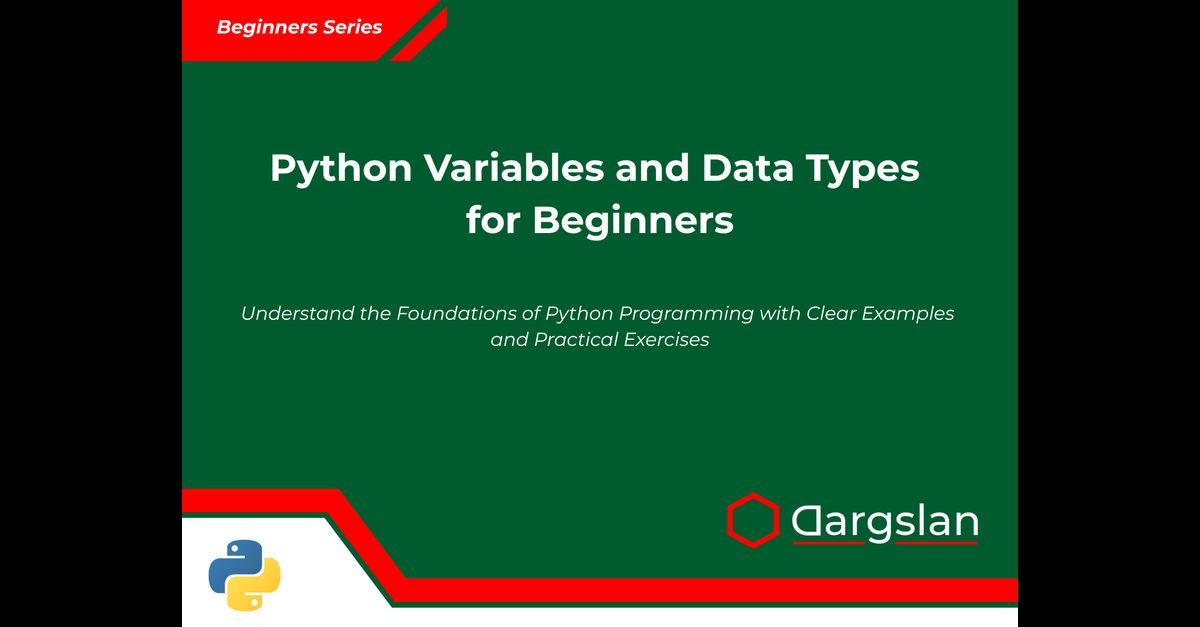
Python Variables and Data Types for Beginners
-2- : Learn Python Loops the Easy Way
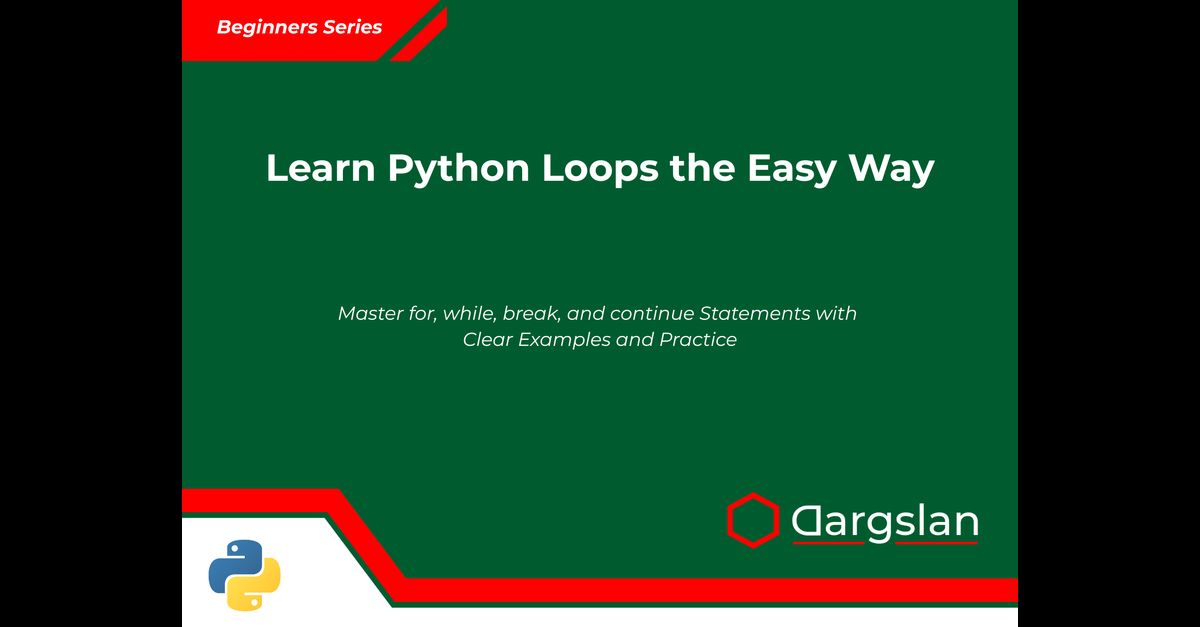
Learn Python Loops the Easy Way
-3- : Python Functions Step by Step
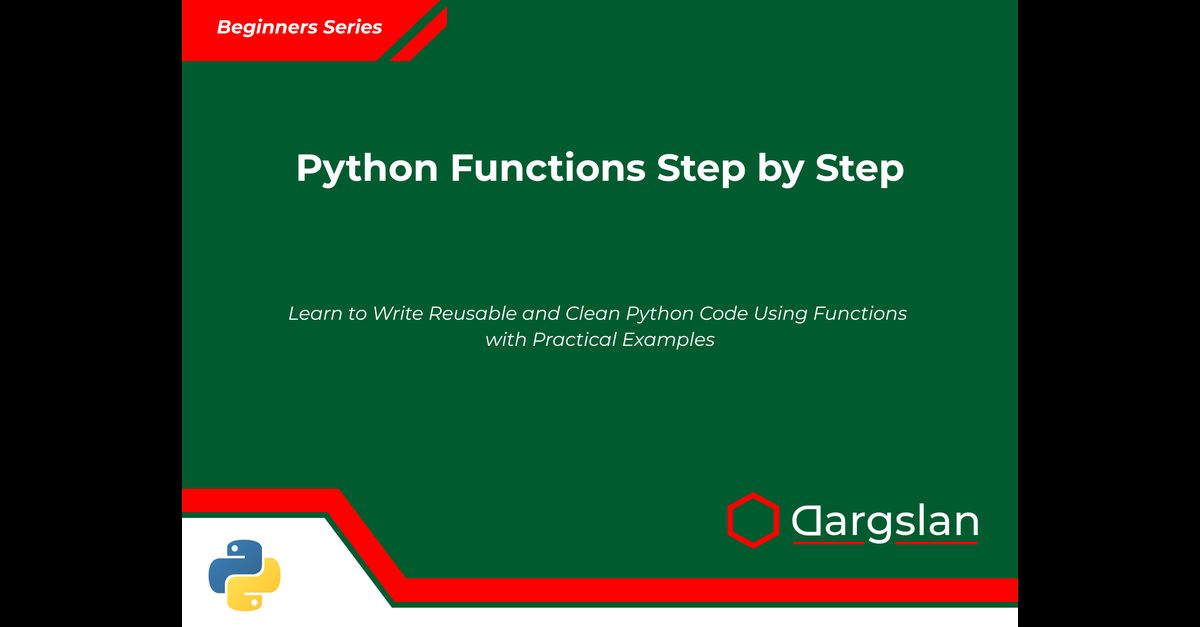
Python Functions Step by Step
-4- : Getting Started with Python Lists and Tuples
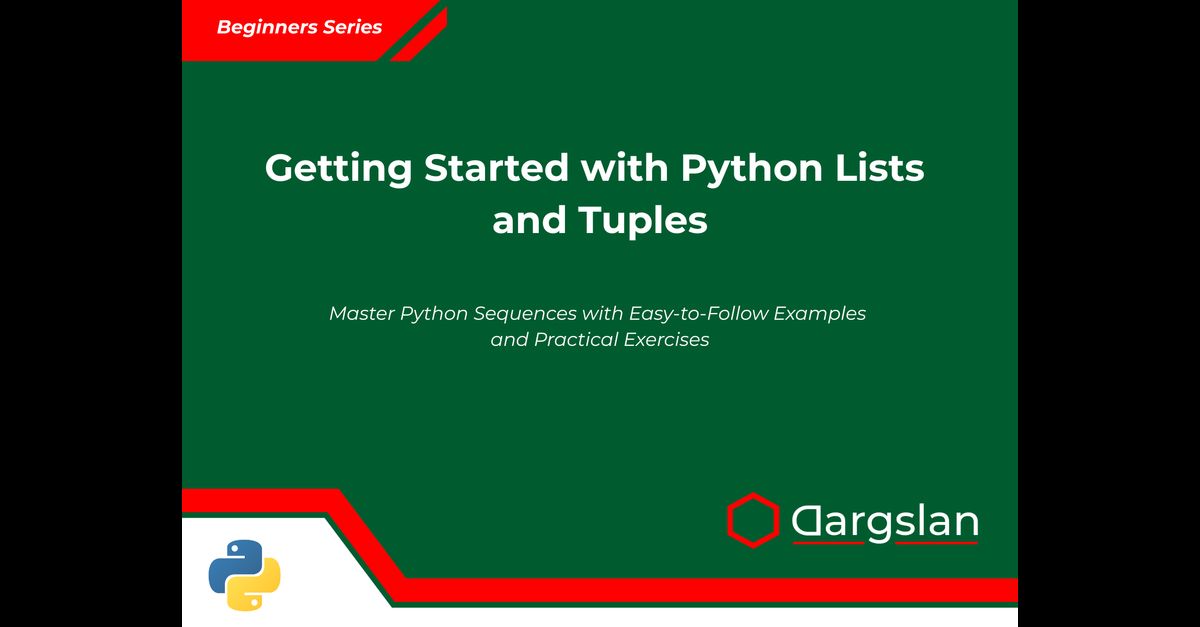
Getting Started with Python Lists and Tuples
-5- : Python Dictionaries Made Simple
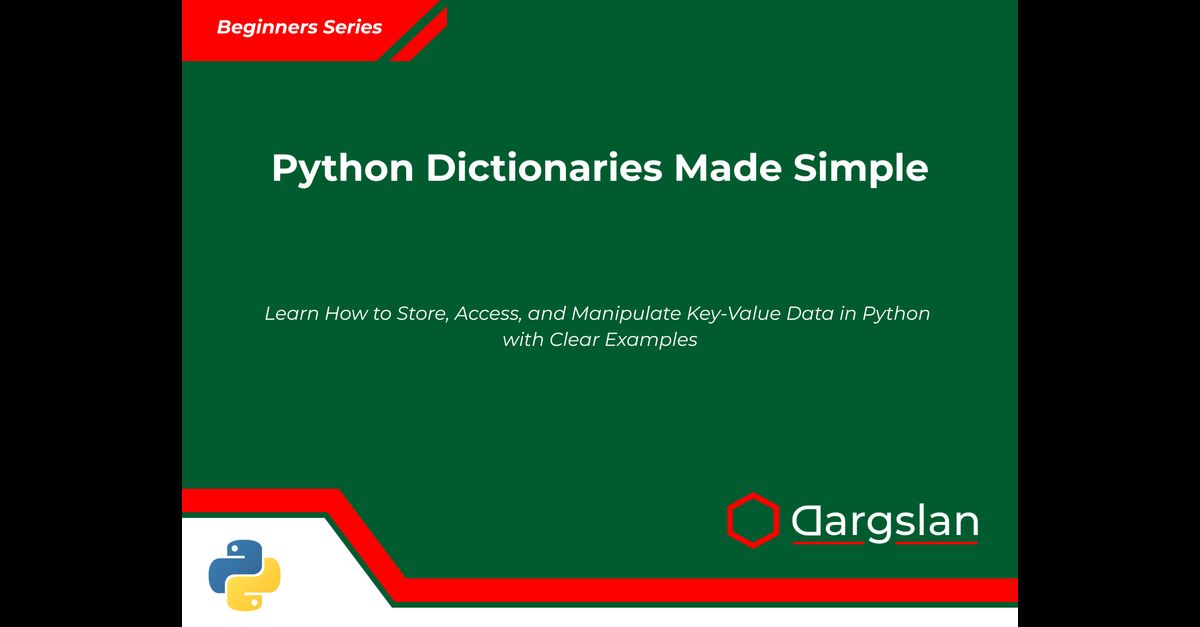
Python Dictionaries Made Simple
-6- : Mastering Conditional Statements in Python
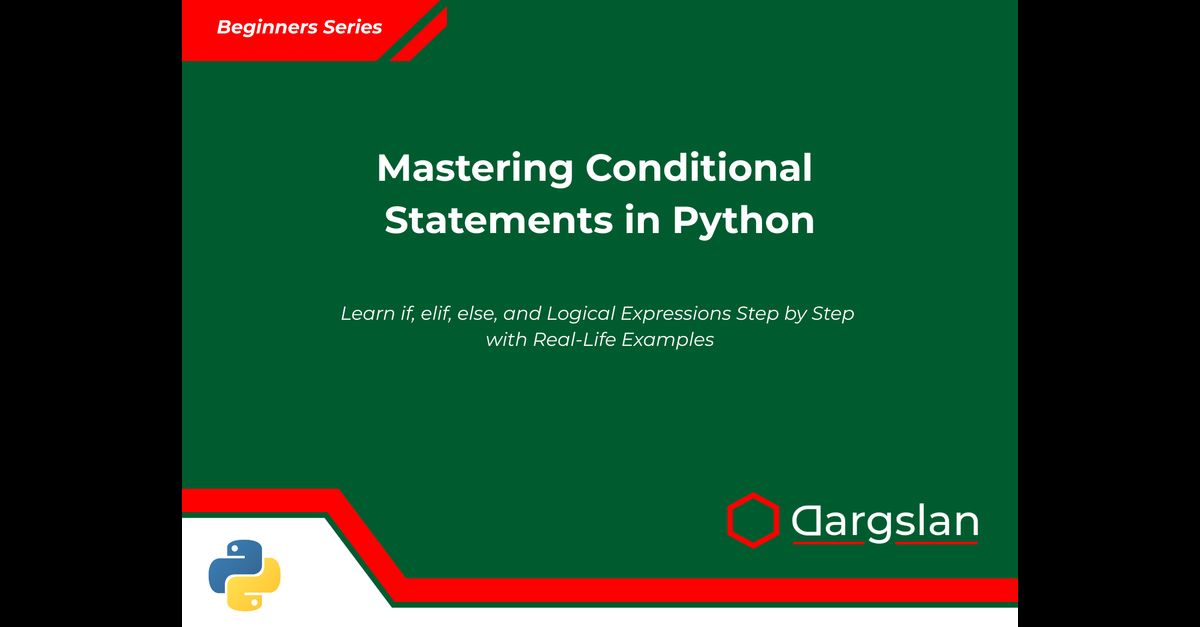
Mastering Conditional Statements in Python
-7- : File Handling in Python for Absolute Beginner
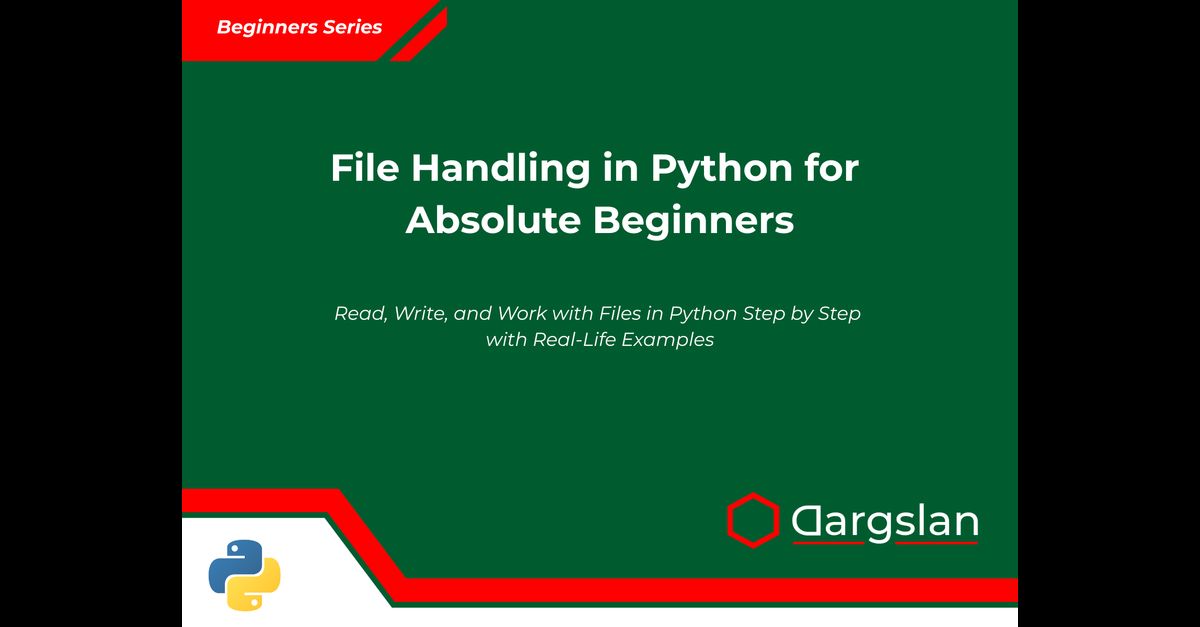
File Handling in Python for Absolute Beginner
-8- : Basic Python Projects for New Coders
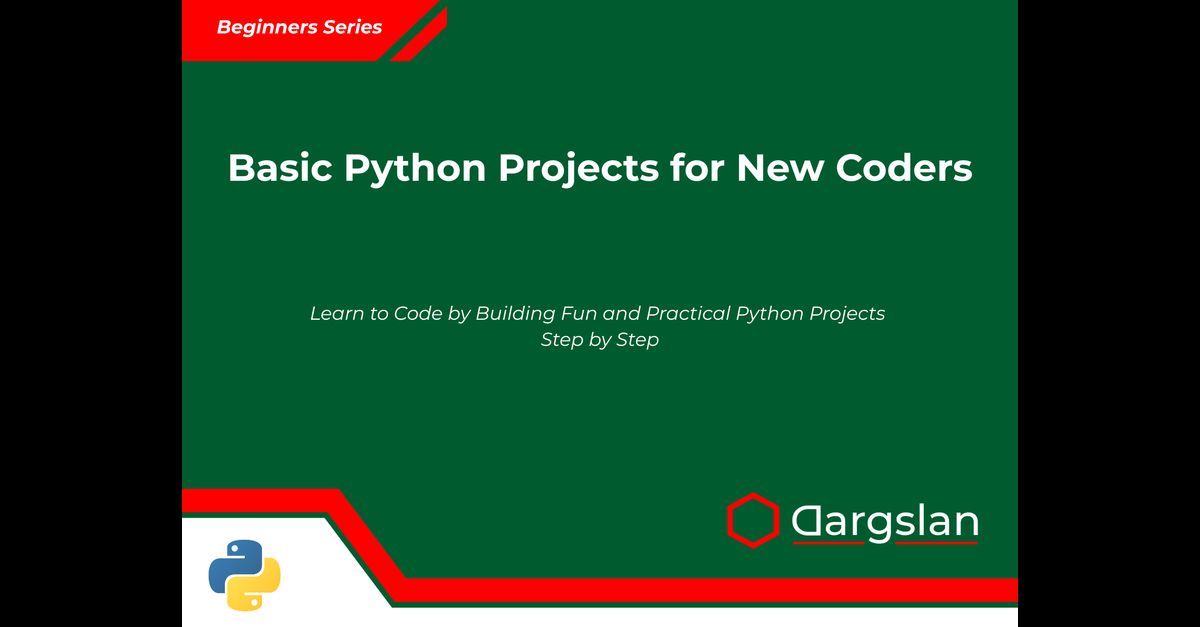
Basic Python Projects for New Coders
-9- : Python Error Handling for Beginners
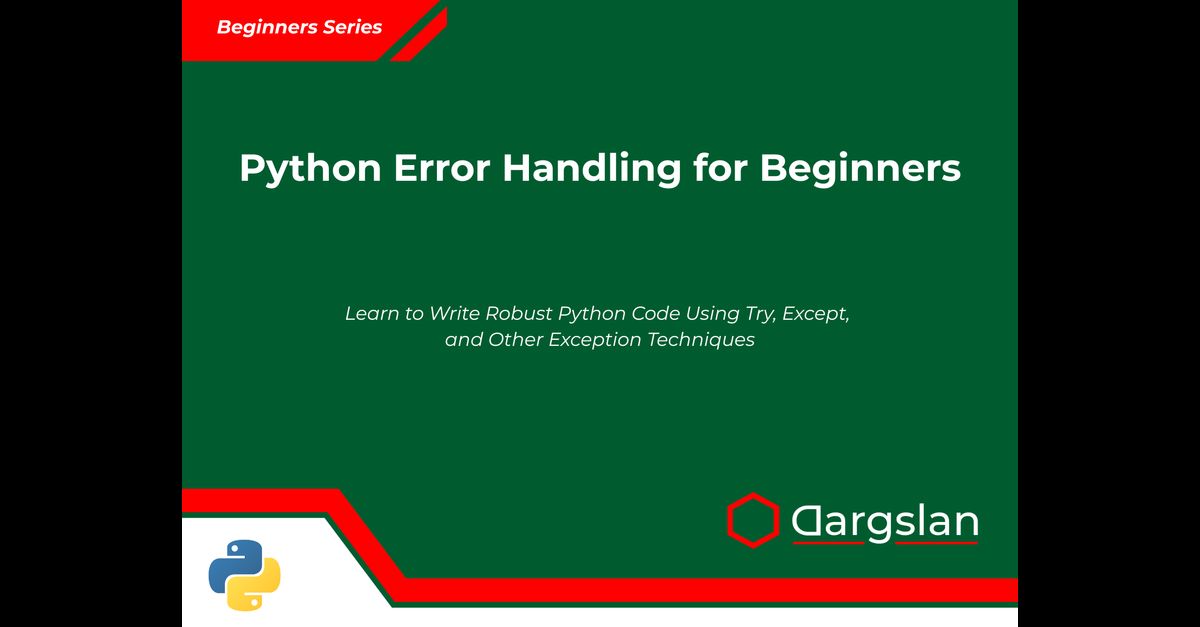
Python Error Handling for Beginners