Book Review: Elegant Code with TypeScript: A Functional Programming Approach
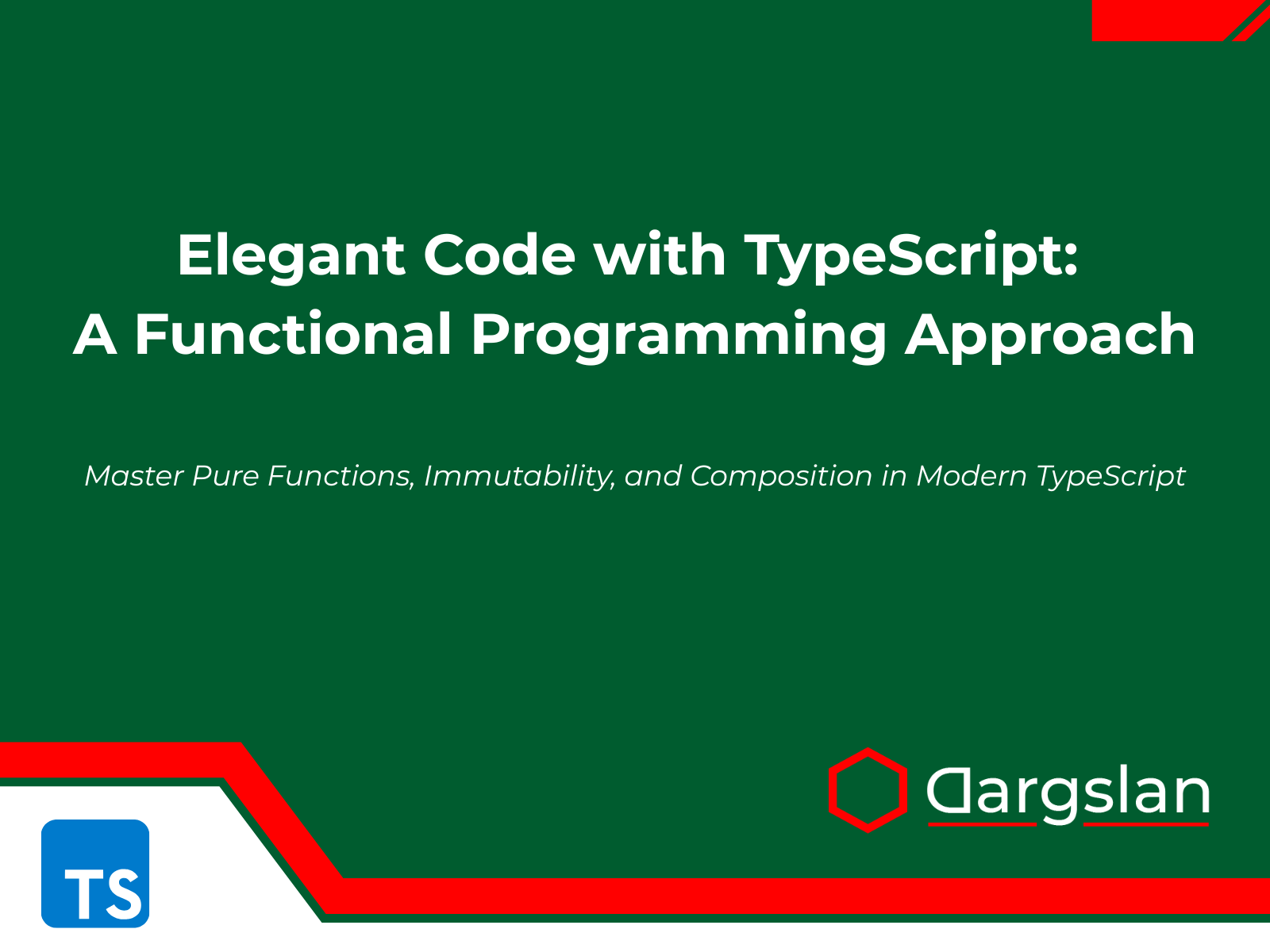
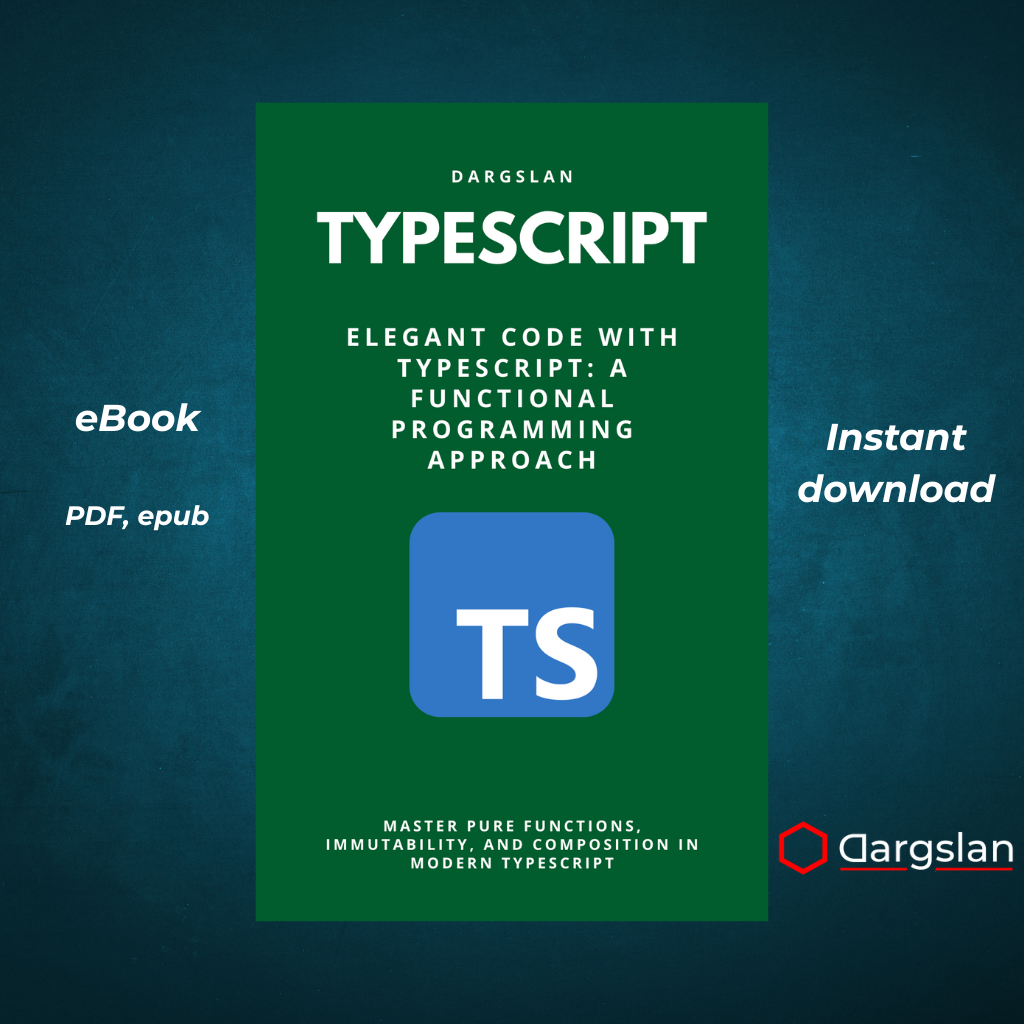
Elegant Code with TypeScript: A Functional Programming Approach
Master Pure Functions, Immutability, and Composition in Modern TypeScript
Introduction: Transforming Modern Development with Functional TypeScript
In the rapidly evolving world of software development, few combinations offer as much promise as functional programming principles applied through TypeScript's robust type system. "Elegant Code with TypeScript: A Functional Programming Approach" by Dargslan stands as a definitive guide for developers seeking to elevate their coding practices beyond mere functionality to true elegance and maintainability.
This comprehensive volume distinguishes itself in the programming literature landscape by bridging the gap between theoretical functional programming concepts and practical TypeScript implementation. As applications grow increasingly complex, the methodologies presented in this book offer a timely solution to many of the pain points experienced by modern developers.
Who Should Read This Book: Target Audience and Prerequisites
This book caters primarily to:
- Mid-level JavaScript/TypeScript developers looking to advance their coding paradigms
- Experienced developers from other languages curious about functional approaches in a JavaScript ecosystem
- Software architects seeking more maintainable and scalable application designs
- Computer science students with interest in applied functional programming
While not explicitly stated as prerequisites, readers will benefit from:
- Basic familiarity with JavaScript or TypeScript syntax
- Some experience with web or application development
- Understanding of fundamental programming concepts
What makes this book particularly accessible is its progressive approach—concepts build upon each other methodically, making it suitable even for those with limited prior exposure to functional programming principles.
Core Value Proposition: Why This Book Matters
In today's development landscape, three pressing challenges stand out:
- Growing application complexity that traditional imperative approaches struggle to manage cleanly
- Increasing demand for reliable, bug-resistant code in mission-critical applications
- Need for more declarative, readable code that future developers can easily maintain
"Elegant Code with TypeScript" directly addresses these challenges by demonstrating how functional programming principles naturally lead to more predictable, testable, and maintainable codebases. The TypeScript context adds an additional layer of safety through its strong typing system, creating a powerful synergy that the book expertly explores.
Chapter-by-Chapter Analysis: A Journey Through Functional TypeScript
Chapter 1: Introduction
The book begins by establishing the "why" behind functional programming in TypeScript. Rather than merely advocating for FP as a superior paradigm, the author presents it as a valuable tool in a developer's broader toolkit. This balanced approach helps readers understand where and when to apply functional concepts for maximum benefit.
Key concepts introduced include:
- The historical context of functional programming
- TypeScript's unique advantages for functional approaches
- The core principles that define functional programming
- How these principles translate to real-world development scenarios
Chapter 2: The Essence of Functional Programming
Chapter 2 delves deeper into the philosophical underpinnings of functional programming. By contrasting functional approaches with more familiar imperative and object-oriented paradigms, the author creates valuable mental anchors for readers transitioning to FP thinking.
Particularly noteworthy is the section on "thinking functionally," which helps readers recognize how many common programming problems are naturally suited to functional solutions. The author draws interesting parallels between mathematical functions and their programming counterparts, establishing a foundation that subsequent chapters build upon.
Chapter 3: TypeScript Essentials for FP
This chapter serves as both a TypeScript refresher and an introduction to the language features that particularly complement functional programming. The coverage of advanced type features is especially valuable:
- Algebraic data types and discriminated unions
- Generic types and type inference
- Readonly types and immutability at the type level
- Utility types that enhance functional patterns
Even experienced TypeScript developers will likely discover new type-level techniques specifically tailored for functional programming contexts.
Chapter 4: Pure Functions and Side Effects
Chapter 4 marks the transition from theory to practice, focusing on one of the most fundamental concepts in functional programming: pure functions. The author provides clear criteria for identifying pure functions and illustrates their benefits through practical examples.
The section on managing side effects stands out as particularly useful for real-world application development. Rather than unrealistically suggesting all side effects can be eliminated, the book demonstrates patterns for:
- Isolating necessary side effects
- Making side effects explicit in type signatures
- Testing code with side effects
- Using functional techniques to manage side-effecting operations
Chapter 5: Immutability and Data Transformation
The challenge of working with immutable data structures in JavaScript—a language designed around mutability—is tackled head-on in this chapter. The author presents:
- Practical techniques for immutable updates
- Performance considerations and optimizations
- Immutable data libraries and their TypeScript integrations
- Patterns for modeling state transformations functionally
The comparative analysis of different approaches to immutability is particularly valuable, helping readers make informed decisions based on their specific project requirements.
Chapter 6: Function Composition and Currying
Perhaps no chapter better exemplifies the book's "elegance" theme than this exploration of function composition. Through progressively more sophisticated examples, readers learn how to:
- Create reusable function pipelines
- Apply partial application and currying for flexible APIs
- Leverage TypeScript's type system to ensure type safety in compositions
- Build complex operations from simple, composable parts
The section connecting function composition to point-free style programming demonstrates how functional approaches can dramatically improve code readability once mastered.
Chapter 7: Higher-Order Functions
Building on previous chapters, this section explores the powerful concept of functions that operate on other functions. Practical examples demonstrate:
- Creating flexible, reusable abstractions
- Implementing common functional utilities like
map
,filter
, andreduce
- Building custom higher-order functions for domain-specific problems
- Using TypeScript's type system to ensure type safety with higher-order functions
The real-world examples showing how higher-order functions can simplify complex business logic are particularly illuminating.
Chapter 8: Recursion and Tail Call Optimization
This chapter tackles one of the more challenging aspects of functional programming: recursive problem-solving. The author does an excellent job making these concepts approachable through:
- Step-by-step analysis of recursive functions
- Comparison of recursive vs. iterative solutions
- Practical techniques for avoiding stack overflow issues
- Working around JavaScript's lack of tail call optimization in production code
The treatment of recursion as a fundamental problem-solving technique rather than a mere syntactic curiosity sets this chapter apart from many other programming texts.
Chapter 9: Functors and Monads (Made Easy)
Perhaps the most intimidating functional programming concepts are demystified in this remarkably accessible chapter. Using concrete TypeScript examples, the author presents:
- Functors as mappable containers
- Monads as computational contexts
- Practical implementations of Option/Maybe and Either/Result types
- How these abstractions solve common programming problems
By focusing on the practical utility rather than mathematical theory, this chapter succeeds where many functional programming texts fail: making advanced concepts accessible to mainstream developers.
Chapters 10-13: Applied Functional Programming
The final four chapters form a cohesive unit focused on applying functional concepts to real-world development scenarios:
- Functional Error Handling: Beyond try/catch with robust functional patterns
- Functional Collections: Working with data transformations at scale
- Reactive Programming: Connecting functional concepts with event-driven architecture
- Building a Functional TypeScript App: Synthesizing all concepts into complete applications
These chapters transform theoretical knowledge into practical skills through comprehensive case studies and complete example applications.
Practical Applications and Industry Relevance
The book excels in demonstrating how functional TypeScript applies across various domains:
- Web Development: Front-end state management, component composition, and side-effect handling
- Backend Systems: Data processing pipelines, API composition, and error management
- Cross-platform Development: Writing consistent business logic across platforms
- Enterprise Applications: Managing complexity in large-scale systems
Particularly valuable is the focus on incremental adoption—showing how functional techniques can be gradually introduced into existing codebases rather than requiring complete rewrites.
Learning Resources and Supplementary Materials
The three appendices provide exceptional supplementary value:
- Appendix A: A curated overview of TypeScript-friendly functional programming libraries
- Appendix B: A practical cheat sheet for quick reference during development
- Appendix C: Structured learning paths for continued exploration
These resources transform the book from a one-time read into an ongoing reference for professional development.
Comparative Analysis: How This Book Stands Out
When compared to other resources in this space:
- Unlike pure functional programming texts, it remains grounded in practical TypeScript development
- Unlike general TypeScript books, it provides deep insight into the functional paradigm
- Unlike online tutorials, it offers a comprehensive, structured learning path
- Unlike academic resources, it focuses on real-world application over theory
This balanced approach makes it uniquely valuable in the current programming literature landscape.
Visual Elements and Pedagogical Approach
While I cannot comment on the specific visual elements without seeing the published book, the structure suggests a thoughtful pedagogical approach:
- Progressive complexity building from fundamentals to advanced topics
- Theoretical foundations followed immediately by practical applications
- Consistent patterns of introducing concepts, demonstrating usage, and exploring edge cases
- A strong focus on TypeScript-specific implementations rather than abstract theory
Who Would Benefit Most From This Book
This book provides exceptional value for:
- Front-end developers working with React, Vue, or Angular seeking more maintainable state management approaches
- Backend developers building Node.js services who want more robust error handling and data processing
- Full-stack developers looking to unify their approach across the technology stack
- Technical leads guiding teams toward more maintainable codebases
- Self-taught programmers looking to deepen their computer science knowledge through practical means
Conclusion: A Definitive Resource for Modern TypeScript Development
"Elegant Code with TypeScript: A Functional Programming Approach" stands as an essential resource for developers seeking to elevate their craft beyond merely functioning code to truly elegant solutions. By bridging the gap between functional programming theory and practical TypeScript development, this book equips readers with powerful tools for managing the complexity of modern applications.
What sets this work apart is its unwavering focus on practical application without sacrificing conceptual depth. Readers will finish not only with new syntax and patterns but with a fundamentally enhanced approach to problem-solving that will serve them across projects and technologies.
For developers feeling the limitations of imperative and object-oriented approaches alone, this book opens new horizons of expression, maintainability, and reliability. In the evolving JavaScript ecosystem, the functional programming skills presented here represent not merely academic interest but essential professional development for forward-thinking developers.
Keywords: TypeScript functional programming, pure functions TypeScript, immutable data TypeScript, function composition, TypeScript monads, functional error handling, TypeScript type system, reactive programming TypeScript, functional web development, JavaScript functional programming
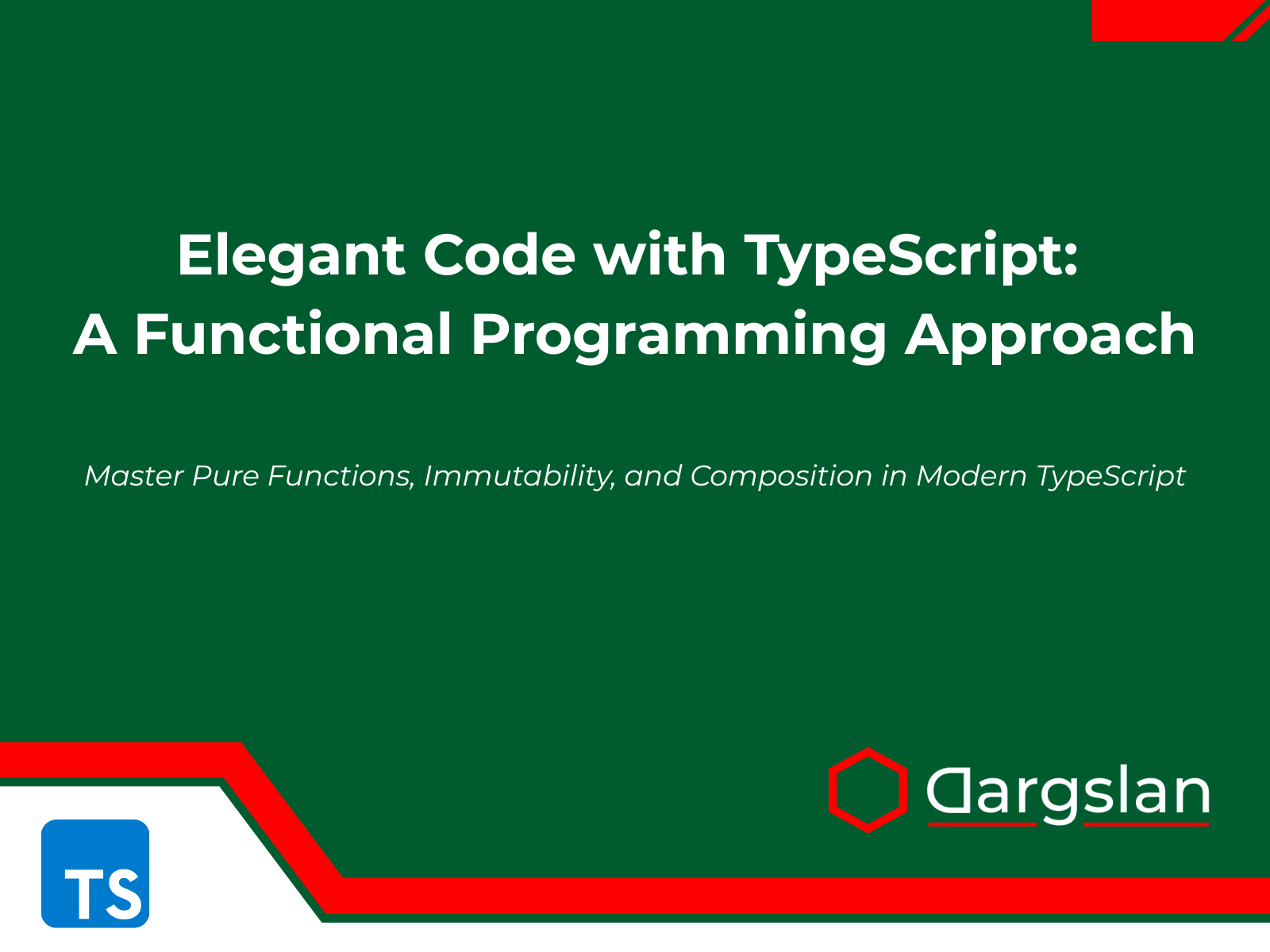
Elegant Code with TypeScript: A Functional Programming Approach
Master Pure Functions, Immutability, and Composition in Modern TypeScript