Book Review: Writing Unit Tests in Python: A Beginner to Intermediate Guide
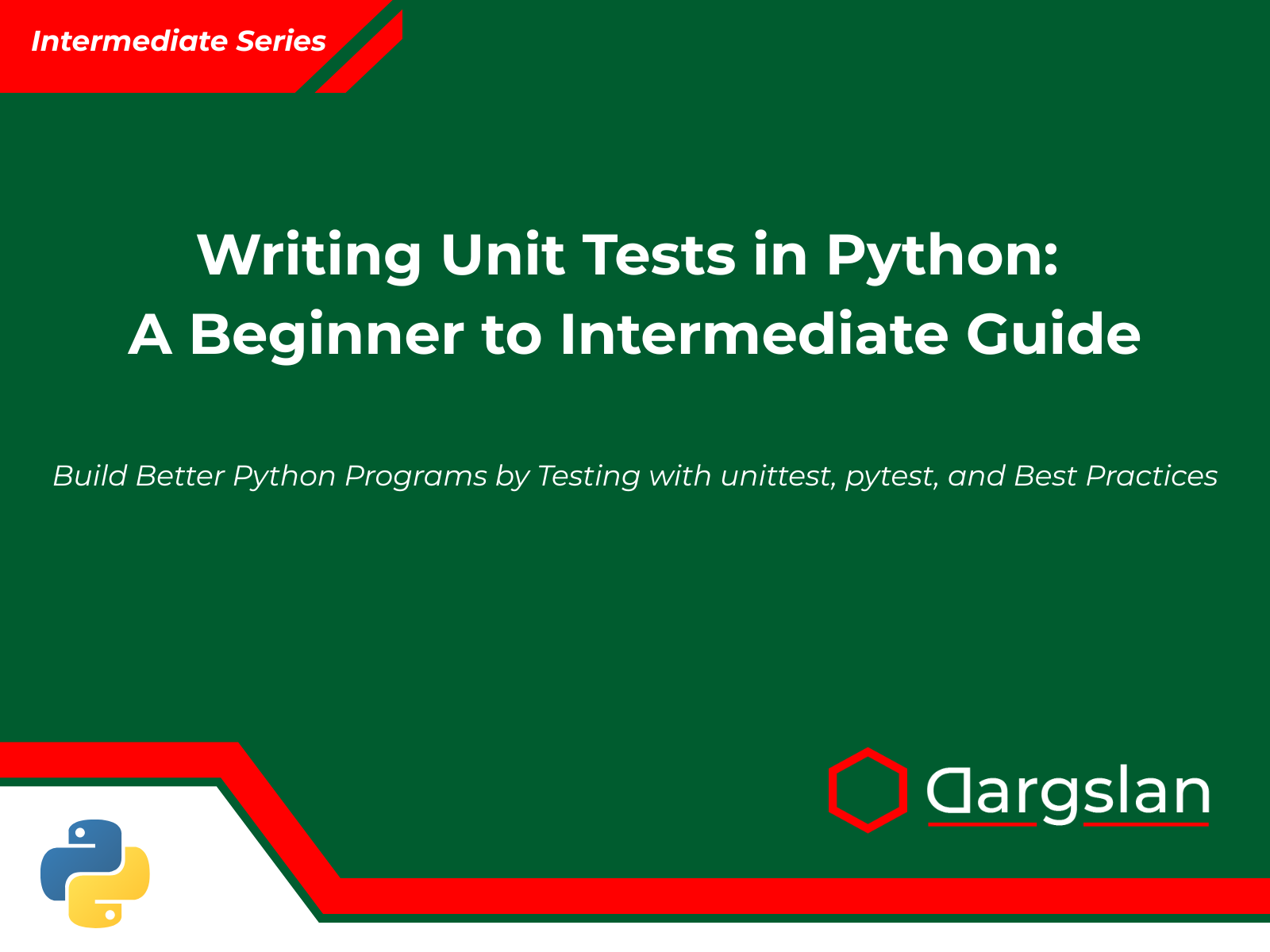
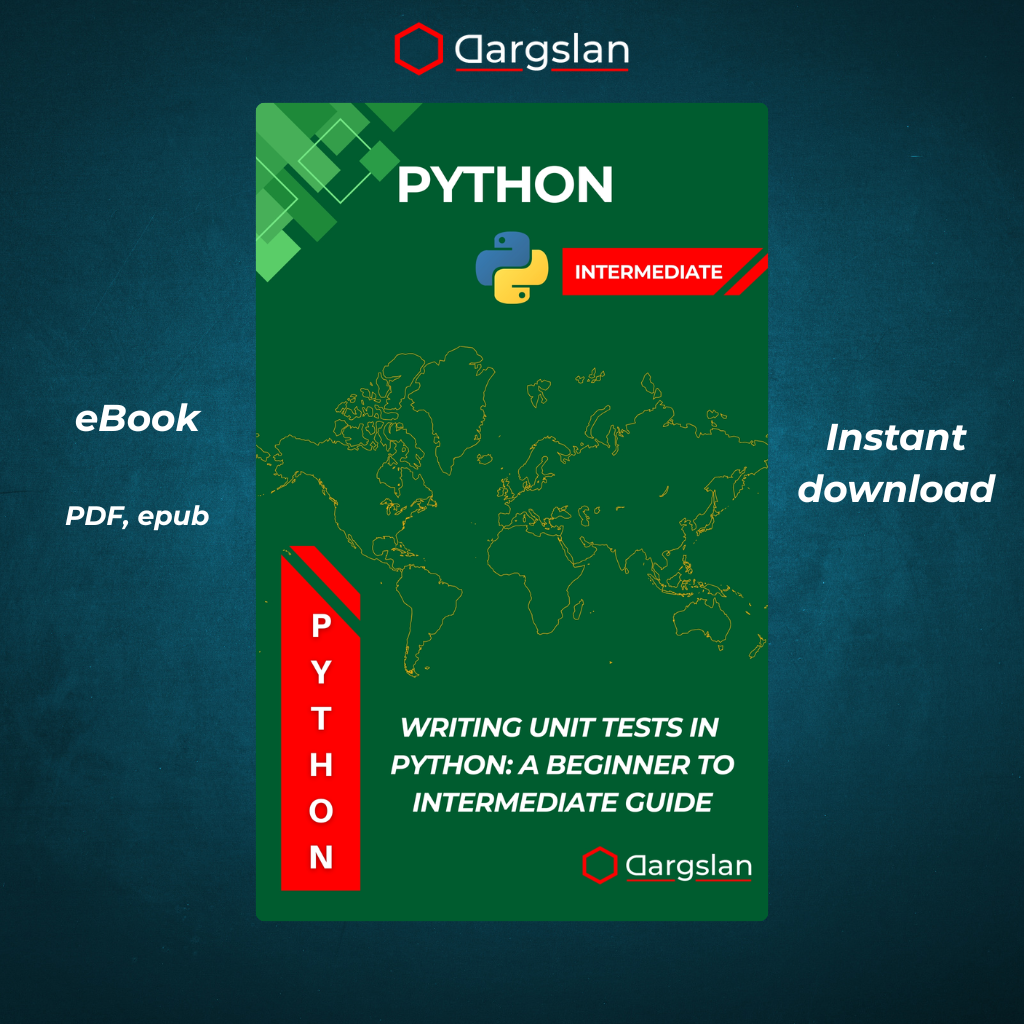
Writing Unit Tests in Python: A Beginner to Intermediate Guide
Build Better Python Programs by Testing with unittest, pytest, and Best Practices
Comprehensive Review: "Writing Unit Tests in Python: A Beginner to Intermediate Guide"
Executive Summary
"Writing Unit Tests in Python: A Beginner to Intermediate Guide" by Dargslan is an exceptional educational resource that bridges the gap between basic programming knowledge and professional Python development practices. This comprehensive guide takes readers on a journey through the essential world of unit testing in Python, covering everything from fundamental concepts to advanced techniques with both the built-in unittest
framework and the popular pytest
library. Whether you're a programming novice looking to establish proper testing habits or an intermediate developer seeking to refine your skills, this book provides a structured, practical approach to mastering Python testing methodologies.
Who Should Read This Book
This book is ideally suited for:
- Python developers transitioning from beginner to intermediate skill levels
- Computer science students looking to develop professional software engineering skills
- Self-taught programmers wanting to adopt industry best practices
- Professional developers new to Python but familiar with testing concepts
- Quality assurance professionals working with Python codebases
- Open-source contributors wanting to improve their testing approaches
The content assumes basic Python knowledge but doesn't require any prior testing experience, making it accessible while still offering depth for more experienced programmers.
Key Strengths and Highlights
Structured Learning Path
The book's greatest strength lies in its carefully designed learning progression. Starting with fundamental testing concepts, it systematically builds toward more complex testing scenarios. This structured approach ensures readers develop a solid foundation before tackling advanced topics like mocking, parameterization, and continuous integration.
Practical Code Examples
Throughout the guide, Dargslan provides real-world, executable code examples that readers can implement immediately. Each concept is illustrated with practical Python code that demonstrates not just how tests work, but why they're essential for robust software development.
Framework Flexibility
While primarily focused on Python's built-in unittest
framework, the book also dedicates significant coverage to pytest
, allowing readers to make informed decisions about which testing framework best suits their projects and preferences.
Comprehensive Coverage of Testing Concepts
The guide doesn't merely teach syntax but delves into the philosophy and strategy behind effective testing. Concepts like test isolation, dependency injection, and test-driven development are thoroughly explained in the Python context.
Detailed Chapter Analysis
Chapter 1: Why Unit Testing Matters
The book opens with a compelling case for unit testing, addressing the common misconception that testing is merely an optional add-on to development. Through concrete examples, the author demonstrates how testing:
- Prevents regression and catches bugs early
- Serves as living documentation for code
- Enables confident refactoring and code evolution
- Improves code design and architecture
- Saves time in the long run through reduced debugging
This chapter effectively sets the stage by establishing testing not as a chore but as an essential element of the development process that ultimately leads to better, more maintainable code.
Chapter 2: Introduction to Python's unittest Framework
Chapter 2 provides a thorough introduction to Python's built-in testing framework. Key topics include:
- Setting up the testing environment
- Creating test cases with the
TestCase
class - Understanding the assertion methods available in
unittest
- Writing your first test functions
- Running tests from the command line
- Interpreting test results and debugging failures
The step-by-step approach ensures readers can immediately start implementing basic tests in their own projects while building confidence with the framework's fundamental components.
Chapter 3: Organizing and Structuring Tests
This chapter addresses the critical but often overlooked topic of test organization. As projects grow, test structure becomes increasingly important for maintainability. The author covers:
- Organizing tests to mirror your application structure
- Creating test suites for logical grouping
- Implementing test fixtures for setup and teardown
- Using decorators for test customization
- Naming conventions and best practices
- Strategies for managing test data
The practical advice on test organization prepares readers for real-world scenarios where well-structured tests dramatically improve project maintenance and developer onboarding.
Chapter 4: Testing Functions with Different Inputs
Chapter 4 dives into the practical aspects of testing function behavior across various inputs. Topics include:
- Boundary value analysis and equivalence partitioning
- Testing edge cases and common failure modes
- Creating comprehensive test cases for complex functions
- Testing numerical algorithms with appropriate precision
- Verifying behavior with different data types
- Testing functions with multiple parameters
This chapter excels in teaching readers how to think critically about testing by considering the full range of possible inputs and execution paths.
Chapter 5: Mocking and Isolating with unittest.mock
The introduction to mocking represents one of the book's most valuable sections, as it addresses the common challenge of testing code with external dependencies. Key topics include:
- Understanding the purpose and philosophy of mocking
- Using
unittest.mock
to create mock objects - Implementing patch decorators and context managers
- Configuring return values and side effects
- Asserting on call counts and call arguments
- Common mocking patterns and anti-patterns
The author provides clear examples of when and how to mock external services, databases, and APIs, enabling readers to write isolated, reliable tests even for complex, interconnected systems.
Chapter 6: Parameterized Tests and Custom Assertions
This intermediate chapter introduces more sophisticated testing techniques:
- Creating parameterized tests to reduce test code duplication
- Implementing data-driven testing approaches
- Developing custom assertions for domain-specific validation
- Building reusable test helpers and utilities
- Balancing test readability with thoroughness
- Using test generators for comprehensive coverage
These advanced techniques help readers streamline their test suites while improving both coverage and maintainability.
Chapter 7: Testing Exceptions and Error Handling
Chapter 7 addresses the often challenging area of testing error conditions:
- Testing that exceptions are raised appropriately
- Verifying exception messages and attributes
- Testing exception hierarchies and custom exceptions
- Handling expected warnings in tests
- Testing error recovery mechanisms
- Strategies for testing logging behavior
This practical coverage ensures readers can verify not just the "happy path" but also the critical error-handling aspects of their code.
Chapter 8: Using pytest for More Power (Optional)
The book provides an excellent introduction to pytest
as an alternative to unittest
:
- Understanding pytest's philosophy and advantages
- Converting unittest tests to pytest format
- Leveraging pytest's powerful assertion system
- Using fixtures for test setup and dependency injection
- Implementing pytest plugins and hooks
- Taking advantage of pytest's reporting capabilities
This chapter gives readers enough information to decide whether to migrate to pytest for their projects while highlighting the framework's unique strengths.
Chapter 9: Code Coverage and CI Integration
Chapter 9 moves beyond basic testing to address professional workflows:
- Measuring code coverage with tools like coverage.py
- Setting up continuous integration for automated testing
- Configuring GitHub Actions, Travis CI, or Jenkins
- Establishing quality gates based on test results
- Implementing pre-commit hooks for test validation
- Generating and interpreting coverage reports
This practical guidance helps readers integrate testing into professional development workflows and ensure consistent quality standards.
Chapter 10: Best Practices and Real-World Scenarios
The final chapter synthesizes the book's knowledge into actionable best practices:
- Balancing test coverage with development velocity
- Implementing test-driven development (TDD) workflows
- Testing in legacy code bases and refactoring scenarios
- Performance considerations in test suites
- Testing strategies for different types of applications
- Common testing pitfalls and how to avoid them
These insights help readers apply testing principles in practical, real-world scenarios where theoretical ideals often meet pragmatic constraints.
Practical Appendices
The book includes several invaluable appendices:
- Common unittest Assertions Cheat Sheet: A quick reference for assertion methods
- Mocking Patterns and Pitfalls: Advanced guidance on effective mocking strategies
- Sample Project with Full Test Coverage: A complete example demonstrating all concepts in context
- Top Interview Questions on Python Testing: Preparation for technical interviews
These supplementary materials enhance the book's value as both a learning resource and an ongoing reference.
Writing Style and Accessibility
Dargslan's writing style strikes an excellent balance between technical precision and accessibility. Complex concepts are broken down into digestible explanations, often accompanied by analogies that help readers grasp abstract testing principles. Code examples are clean, well-commented, and follow Python best practices, serving as models of both testing and general Python development.
The progressive difficulty curve ensures readers aren't overwhelmed, with each chapter building naturally on previous knowledge. Frequent recap sections and practical exercises reinforce learning and encourage hands-on practice.
Comparison with Other Python Testing Resources
Compared to other Python testing resources, this book offers several distinct advantages:
- More comprehensive than most online tutorials, which typically cover only basics
- More focused than general Python programming books that include testing as just one chapter
- More accessible than official documentation, with explanations of why and when, not just how
- More practical than academic treatments of software testing theory
- More up-to-date than many existing Python testing books, including modern tools and practices
While Harry Percival's "Test-Driven Development with Python" remains excellent for those specifically interested in TDD, and Brian Okken's "Python Testing with pytest" offers deeper pytest coverage, Dargslan's book provides the most balanced, comprehensive introduction to Python testing for those at the beginner-to-intermediate level.
Real-World Applications
The book's approach is particularly valuable for several common scenarios:
-
New Project Development: Readers learn how to establish good testing practices from the start, avoiding technical debt.
-
Legacy Code Maintenance: The book provides strategies for gradually adding tests to existing codebases.
-
Team Onboarding: The clear explanations and consistent approaches make this an excellent resource for standardizing testing practices across development teams.
-
Interview Preparation: The appendix on common interview questions helps readers prepare for technical interviews where testing knowledge is increasingly valued.
-
Open Source Contribution: The testing strategies align well with the quality expectations of major open-source Python projects.
Areas for Improvement
While the book is comprehensive, a few additional topics could enhance its value:
- More coverage of testing asynchronous code with asyncio
- Additional examples for testing web applications and APIs
- More extensive discussion of property-based testing
- More guidance on testing Python applications with complex GUIs
These minor gaps don't detract significantly from the book's overall value but represent potential areas for expansion in future editions.
Technical Accuracy and Currency
The technical content is accurate and current with modern Python practices. The book covers Python 3.6+ features and follows PEP 8 style guidelines throughout. Testing approaches align with current industry best practices, and the author addresses important considerations like type hints and how they interact with testing.
All code examples appear to have been thoroughly tested and work as presented, eliminating the frustration often encountered in programming books where example code contains errors or omissions.
Final Verdict: An Essential Python Development Resource
"Writing Unit Tests in Python" stands as an essential resource for Python developers serious about code quality and professional development practices. It successfully bridges the gap between basic "how to program" books and advanced software engineering texts, providing practical guidance on a topic too often neglected in programming education.
The book's greatest achievement is making testing accessible and even enjoyable, transforming what many perceive as a chore into a valuable skill that improves both code quality and developer confidence. By following Dargslan's methodical approach, readers will not only learn how to test effectively but will likely find themselves writing better, more modular, and more maintainable Python code as a natural consequence.
For Python developers looking to elevate their skills from basic programming to professional software engineering, this book represents one of the most valuable investments they can make in their technical education. Highly recommended for anyone serious about Python development.
Key Takeaways
- Comprehensive coverage of Python testing from basics to advanced techniques
- Balanced treatment of both unittest and pytest frameworks
- Practical, real-world examples that readers can immediately apply
- Structured approach that builds skills progressively
- Valuable appendices that serve as ongoing reference materials
- Clear explanations that connect testing practices to broader software quality
- Up-to-date with modern Python practices and tools
Whether you're establishing testing habits for the first time or refining your approach to create more robust test suites, "Writing Unit Tests in Python: A Beginner to Intermediate Guide" delivers exceptional value as both a learning resource and a reference for ongoing Python development.
This review is based on the preface and table of contents of "Writing Unit Tests in Python: A Beginner to Intermediate Guide" by Dargslan. The detailed analysis aims to provide potential readers with comprehensive insights into the book's content, approach, and value for Python developers at various skill levels.
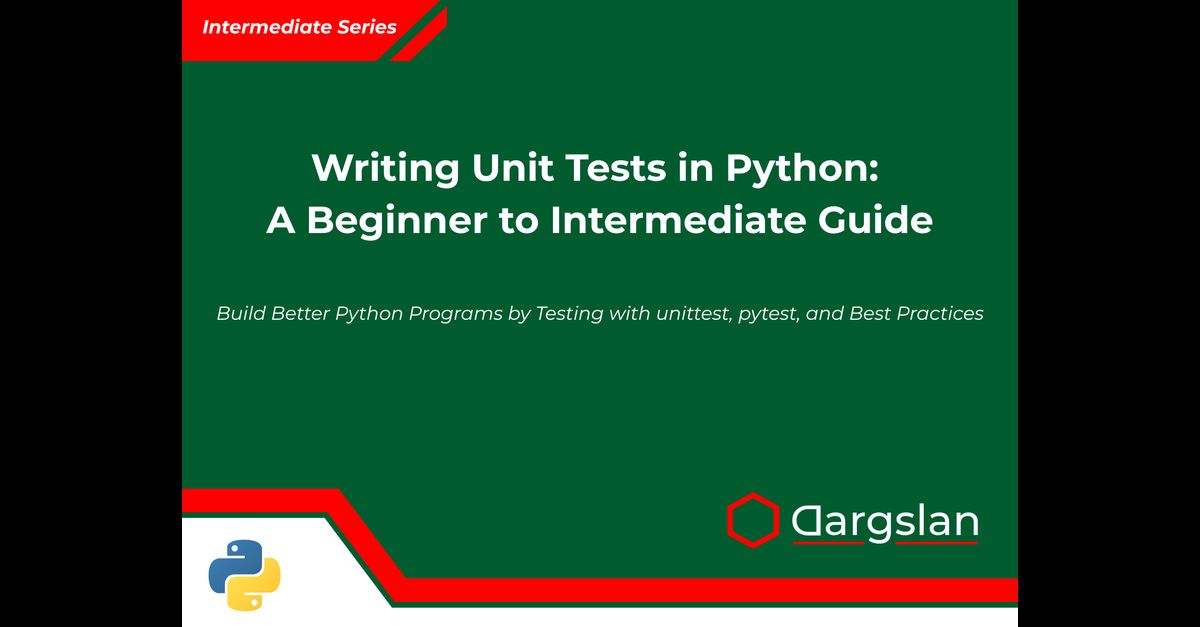
Writing Unit Tests in Python: A Beginner to Intermediate Guide