Book Review: Writing Secure Code in Node.js
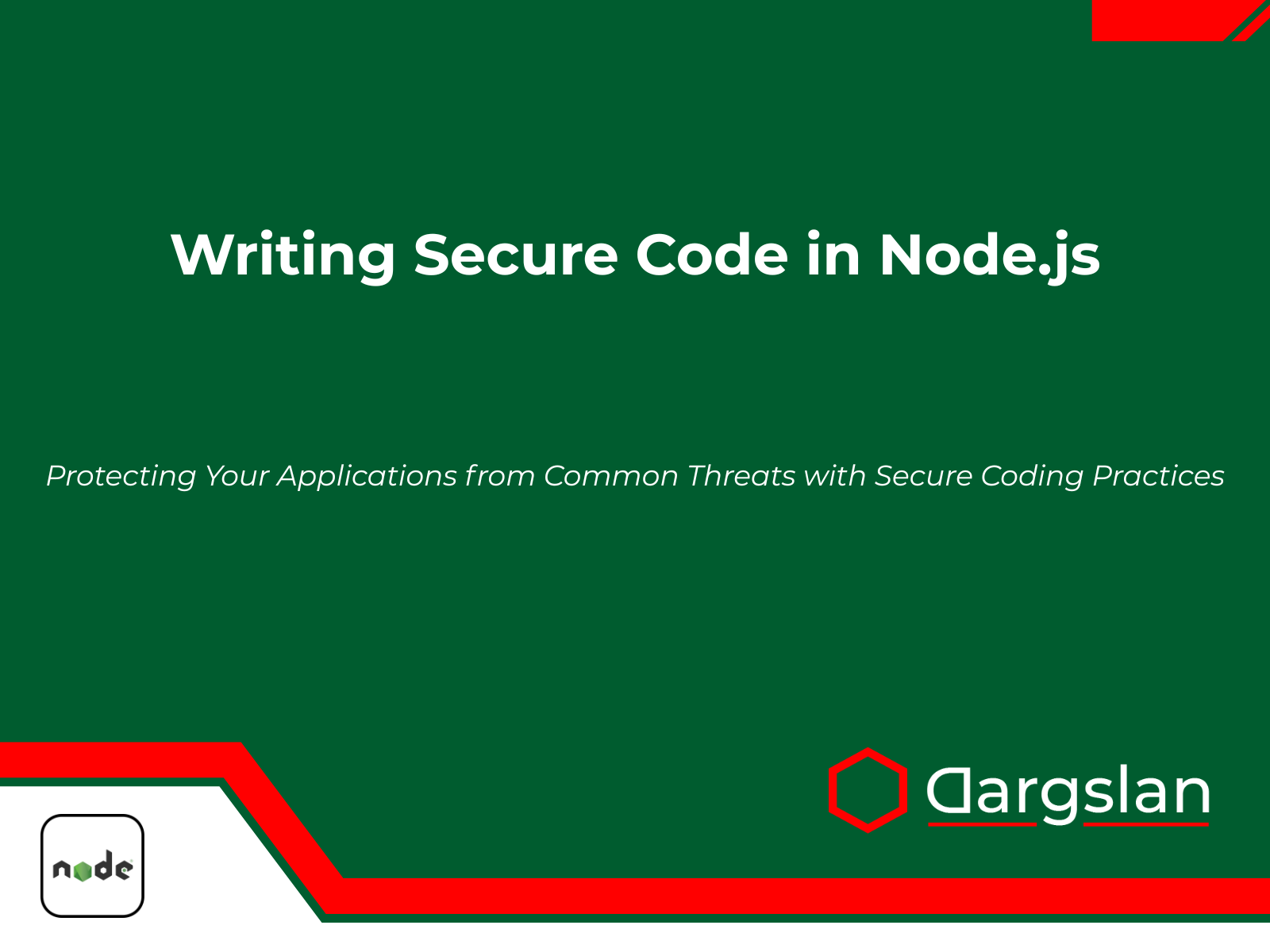
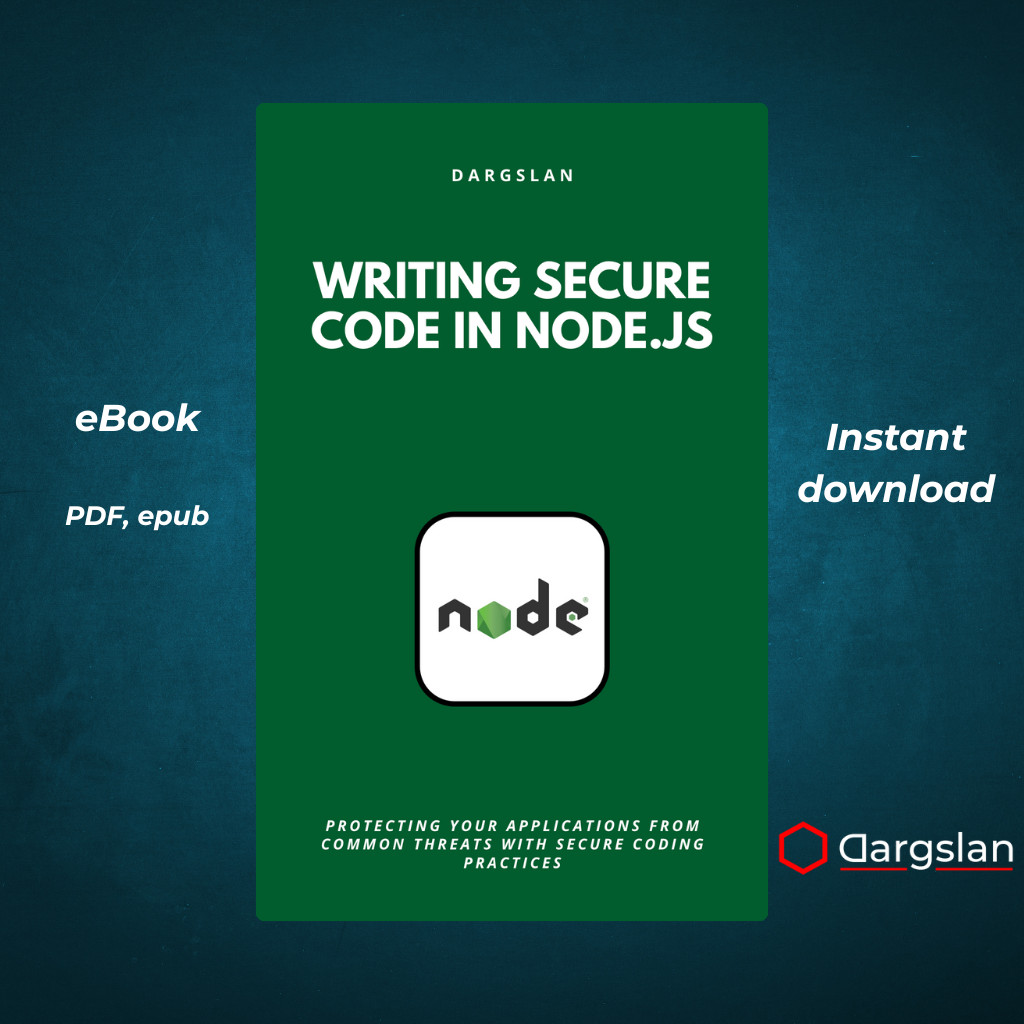
Writing Secure Code in Node.js
Protecting Your Applications from Common Threats with Secure Coding Practices
Writing Secure Code in Node.js: A Comprehensive Guide to Application Security
Book Preview and In-Depth Analysis
A practical, hands-on guide to building secure Node.js applications in an increasingly threatening digital landscape
Introduction: Why Node.js Security Matters
In today's interconnected digital ecosystem, Node.js has established itself as a cornerstone technology for modern web development. Its event-driven, non-blocking architecture has revolutionized how developers build scalable network applications. However, this popularity comes with increased security scrutiny, as Node.js applications become prime targets for malicious actors seeking to exploit vulnerabilities.
"Writing Secure Code in Node.js" arrives at a critical juncture in web development history. With cyber attacks increasing in both frequency and sophistication, the need for security-conscious development practices has never been more urgent. This comprehensive guide addresses this need by providing developers with practical strategies, techniques, and code examples to fortify their Node.js applications against common and emerging threats.
What Makes This Book Essential
This book stands out by offering more than theoretical security concepts—it delivers actionable, Node.js-specific security implementations that developers can immediately incorporate into their workflows. Whether you're building RESTful APIs, real-time applications, or microservices, the security principles outlined here will help protect your applications from vulnerabilities that could lead to data breaches, unauthorized access, or service disruptions.
The author, Dargslan, brings years of experience in secure application development to create a resource that bridges the gap between general security knowledge and Node.js-specific implementation details. This practical approach ensures readers can immediately apply what they learn to strengthen their existing and future projects.
Who Should Read This Book
This guide is meticulously crafted for:
- Node.js developers seeking to enhance their security knowledge
- Security professionals transitioning into Node.js environments
- Development team leaders responsible for establishing secure coding standards
- DevOps engineers implementing security throughout the deployment pipeline
- Students and aspiring developers wanting to build security skills from the start
While some familiarity with Node.js is assumed, the book progressively builds security concepts from foundational to advanced, making it accessible for developers at various experience levels.
Chapter-by-Chapter Analysis
Chapter 1: The Security Mindset in Node.js Development
This foundational chapter introduces the concept of "security by design" in the Node.js ecosystem. Readers will learn how to approach application development with security as a primary consideration rather than an afterthought.
Key topics include:
- Cultivating threat awareness in the development process
- Understanding the Node.js security landscape and common attack vectors
- Balancing security with performance and user experience
- Implementing defense-in-depth strategies specific to JavaScript applications
- Setting up secure development environments for Node.js
The chapter establishes a crucial mindset shift, emphasizing that security is not a feature but a continuous process that must be integrated throughout the development lifecycle.
Chapter 2: Understanding the Node.js Runtime
Security begins with comprehending how your underlying platform operates. This chapter provides a deep dive into the Node.js runtime security model.
Detailed coverage includes:
- The V8 JavaScript engine and its security implications
- Event loop vulnerabilities and how to mitigate them
- Understanding Node.js process isolation and its limitations
- Memory management and potential leaks affecting security
- The role of the global object in security considerations
- Node.js module system security (CommonJS vs. ES Modules)
Through practical examples, readers will gain insight into how the Node.js runtime can influence application security, setting the stage for more specific security implementations.
Chapter 3: Input Validation and Sanitization
The first line of defense in any secure application is proper handling of user input. This chapter explores comprehensive strategies for validating and sanitizing data in Node.js applications.
The chapter covers:
- Implementing schema validation with libraries like Joi and Yup
- Type checking and coercion security concerns in JavaScript
- Sanitizing user input to prevent XSS and injection attacks
- Creating custom validators for domain-specific data
- Balancing strict validation with user experience
- Validation strategies for different data formats (JSON, form data, query parameters)
Code examples demonstrate practical implementation of validation pipelines that protect applications without compromising usability.
Chapter 4: Authentication and Session Management
Authentication vulnerabilities remain among the most exploited security weaknesses. This chapter provides comprehensive guidance on implementing secure authentication systems.
Key aspects include:
- Password storage best practices using bcrypt and Argon2
- Implementing JWT-based authentication securely
- Multi-factor authentication implementation in Node.js
- Session management strategies and security
- Account lockout mechanisms and brute force protection
- OAuth and OpenID Connect implementation considerations
- Biometric authentication integration
Readers will learn to avoid common pitfalls in authentication systems while implementing robust solutions that protect user credentials and sessions.
Chapter 5: Authorization and Access Control
Authentication verifies identity, but authorization determines what authenticated users can access. This chapter explores implementing granular access control in Node.js applications.
Topics covered include:
- Role-based access control (RBAC) implementation
- Attribute-based access control (ABAC) for complex permissions
- JWT scope and claims for API authorization
- Implementing middleware for access control
- Database-level permission enforcement
- Principle of least privilege in Node.js contexts
- Auditing and logging access control decisions
Through practical examples, readers will learn to implement authorization systems that prevent privilege escalation and unauthorized access to sensitive resources.
Chapter 6: Secure Dependency Management
The npm ecosystem is both a strength and a security challenge for Node.js. This chapter addresses the critical issue of managing third-party dependencies securely.
In-depth coverage includes:
- Auditing dependencies for known vulnerabilities
- Strategies for keeping dependencies updated securely
- Lock files and version pinning best practices
- Creating and maintaining a dependency security policy
- Monitoring for new vulnerabilities in existing dependencies
- Evaluating the security posture of third-party packages
- Implementing dependency isolation where appropriate
This chapter provides practical workflows for managing the complex web of dependencies while minimizing security exposure.
Chapter 7: Avoiding Code Injection
Injection attacks remain among the most dangerous threats to web applications. This chapter focuses specifically on preventing various forms of injection in Node.js.
The chapter examines:
- SQL injection prevention in various Node.js database drivers
- NoSQL injection attacks and mitigations
- Command injection vulnerabilities in child processes
- Preventing prototype pollution attacks
- Regular expression injection and ReDoS attacks
- Code injection through eval() and similar functions
- Content injection vulnerabilities
Practical examples demonstrate secure coding patterns that prevent injection vulnerabilities while maintaining application functionality.
Chapter 8: Secure File Handling and Uploads
File operations present unique security challenges. This chapter provides comprehensive guidance on handling files securely in Node.js applications.
Key topics include:
- Implementing secure file upload functionality
- File type verification beyond MIME types
- Preventing path traversal attacks
- Secure storage of uploaded files
- Virus scanning integration
- Secure file download implementation
- Managing file permissions securely
Readers will learn to implement file operations that protect against common exploits while providing necessary functionality.
Chapter 9: HTTPS, TLS, and Secure Communication
Secure communication is fundamental to web application security. This chapter provides detailed guidance on implementing and optimizing secure communication in Node.js.
Coverage includes:
- Setting up HTTPS servers in various Node.js frameworks
- TLS configuration best practices and common pitfalls
- Certificate management and automation
- HTTP security headers implementation
- CORS configuration for security
- Preventing man-in-the-middle attacks
- WebSocket security considerations
The chapter balances theoretical knowledge with practical implementation details for creating truly secure communication channels.
Chapter 10: Logging and Monitoring Securely
Effective security requires visibility. This chapter explores implementing logging and monitoring systems that enhance security posture without creating new vulnerabilities.
Topics covered include:
- Implementing secure application logging
- Protecting sensitive data in logs
- Log aggregation and analysis for security events
- Real-time monitoring and alerting strategies
- Intrusion detection patterns for Node.js
- Performance monitoring security implications
- Compliance considerations in logging
Practical examples demonstrate how to implement logging that serves security needs while respecting privacy and performance constraints.
Chapter 11: Secure APIs and REST Practices
APIs are the primary interface for many Node.js applications. This chapter focuses on implementing secure API design principles.
In-depth coverage includes:
- RESTful API security best practices
- API authentication and authorization patterns
- Rate limiting and throttling implementation
- Preventing API abuse and scraping
- Secure webhook implementation
- API versioning security considerations
- GraphQL specific security challenges
Through practical examples, readers will learn to design and implement APIs that resist common attack patterns.
Chapter 12: Writing Secure Middleware
Middleware is central to most Node.js frameworks. This chapter explores security considerations specific to middleware development and implementation.
Key topics include:
- Security-focused middleware development patterns
- Common middleware security pitfalls
- Authentication and authorization middleware implementation
- Implementing content security policies
- CSRF protection middleware
- Security headers middleware
- Error handling middleware for security
Practical examples demonstrate how to leverage middleware architecture to enhance application security systematically.
Chapter 13: Security Testing and Hardening
Security requires verification. This chapter provides comprehensive guidance on testing and hardening Node.js applications.
The chapter covers:
- Automated security testing frameworks for Node.js
- Static analysis tools and configuration
- Dynamic application security testing
- Penetration testing methodologies for Node.js
- Security code reviews and best practices
- Docker container security for Node.js applications
- Hardening Node.js for production environments
Readers will learn to implement multi-layered testing strategies that identify vulnerabilities before deployment.
Chapter 14: Real-World Secure Coding Case Studies
Theory meets practice in this final chapter, which analyzes real-world security incidents and provides detailed remediation strategies.
Case studies include:
- Analysis of famous Node.js security breaches
- Authentication system vulnerabilities and fixes
- API security failure analysis
- Data exposure incidents and prevention
- Performance-based attacks and mitigations
- Supply chain attack case studies
- Recovery and response strategies
Each case study connects theoretical knowledge from previous chapters to practical security incidents, reinforcing key lessons.
Appendices: Practical Resources for Ongoing Security
The book includes three valuable appendices that serve as quick reference guides:
Appendix A – Node.js Security Checklist
A comprehensive, actionable security checklist that developers can apply to any Node.js project. This appendix serves as both an audit tool and a reference for implementing security best practices.
Appendix B – Common Vulnerabilities Cheat Sheet
A quick reference guide to common Node.js vulnerabilities, including detection methods, impact assessment, and remediation strategies. This appendix helps developers quickly identify and address common security issues.
Appendix C – Tools and Resources
A curated collection of security tools, libraries, and resources specifically valuable for Node.js developers. This includes static analysis tools, security scanning services, and educational resources for continuing security education.
What Sets This Book Apart
"Writing Secure Code in Node.js" differentiates itself through several key attributes:
-
Node.js Specificity: Rather than general web security principles, the book focuses exclusively on Node.js-specific security concerns, implementation details, and best practices.
-
Practical Implementation: Each concept is accompanied by real-world code examples that readers can adapt to their own projects, bridging the gap between theory and practice.
-
Full Stack Coverage: The security guidance covers the entire application stack, from the Node.js runtime through middleware to client-facing APIs.
-
Current Best Practices: The content reflects the latest security approaches for modern Node.js development, including newer features and contemporary threat models.
-
DevOps Integration: Security principles are presented in the context of modern development workflows, including CI/CD pipelines and containerized deployments.
How to Get the Most from This Book
To maximize the value of this comprehensive guide:
-
Work through the examples: Type out and experiment with the provided code examples rather than just reading them.
-
Apply principles incrementally: Start by applying security principles to new code, then gradually refactor existing codebases.
-
Use the checklists: Apply the security checklists to your projects regularly as part of your development workflow.
-
Join the community: Connect with other security-minded Node.js developers to share experiences and stay current on emerging threats.
-
Establish team practices: Use the book as a foundation for establishing security standards within your development team.
Conclusion: Security as a Continuous Journey
"Writing Secure Code in Node.js" serves as both a comprehensive learning resource and an ongoing reference for developers committed to creating secure applications. In a landscape where security threats continuously evolve, this book provides the foundational knowledge and practical skills needed to protect applications, data, and users.
Security is not a destination but a journey—one that requires vigilance, continuous learning, and adaptation. By integrating the principles from this book into your development practice, you'll not only write more secure code today but develop the security mindset needed to address the challenges of tomorrow.
For Node.js developers who recognize that security is no longer optional but essential, this book represents an invaluable investment in both professional development and application quality. Start your journey toward more secure Node.js development today, and help create a safer digital ecosystem for all.
"Writing Secure Code in Node.js: Protecting Your Applications from Common Threats with Secure Coding Practices" is the definitive guide for developers who understand that in today's threat landscape, application security is not just a feature—it's a fundamental responsibility.
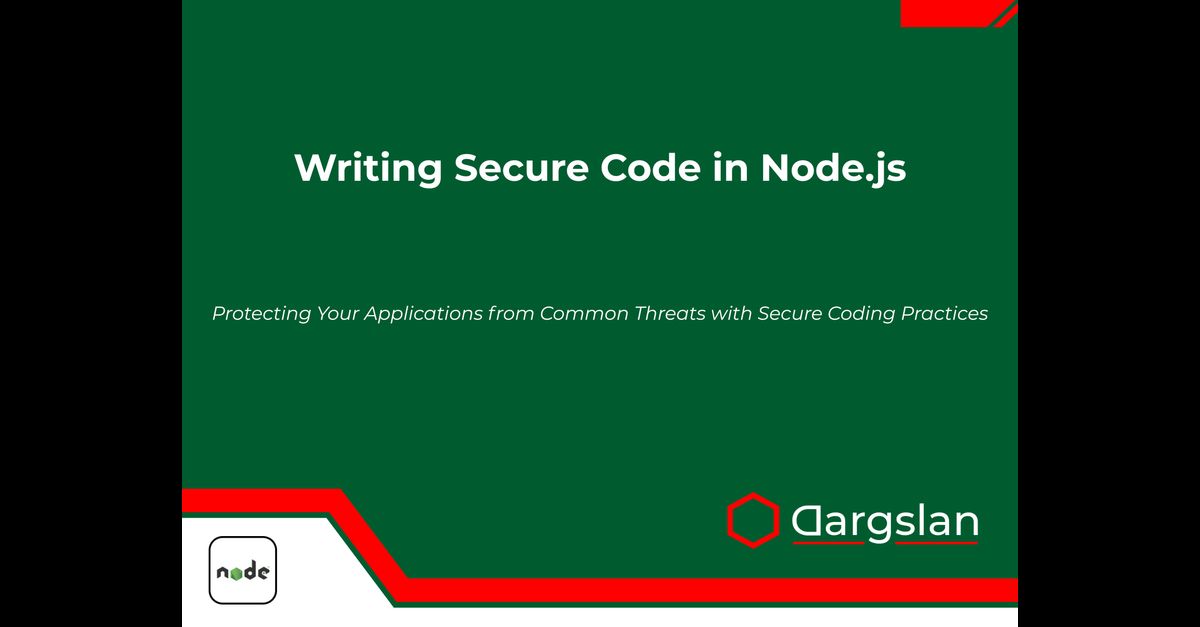
Writing Secure Code in Node.js