Book Review: Writing Clean Code in Python: A Beginner’s Guide
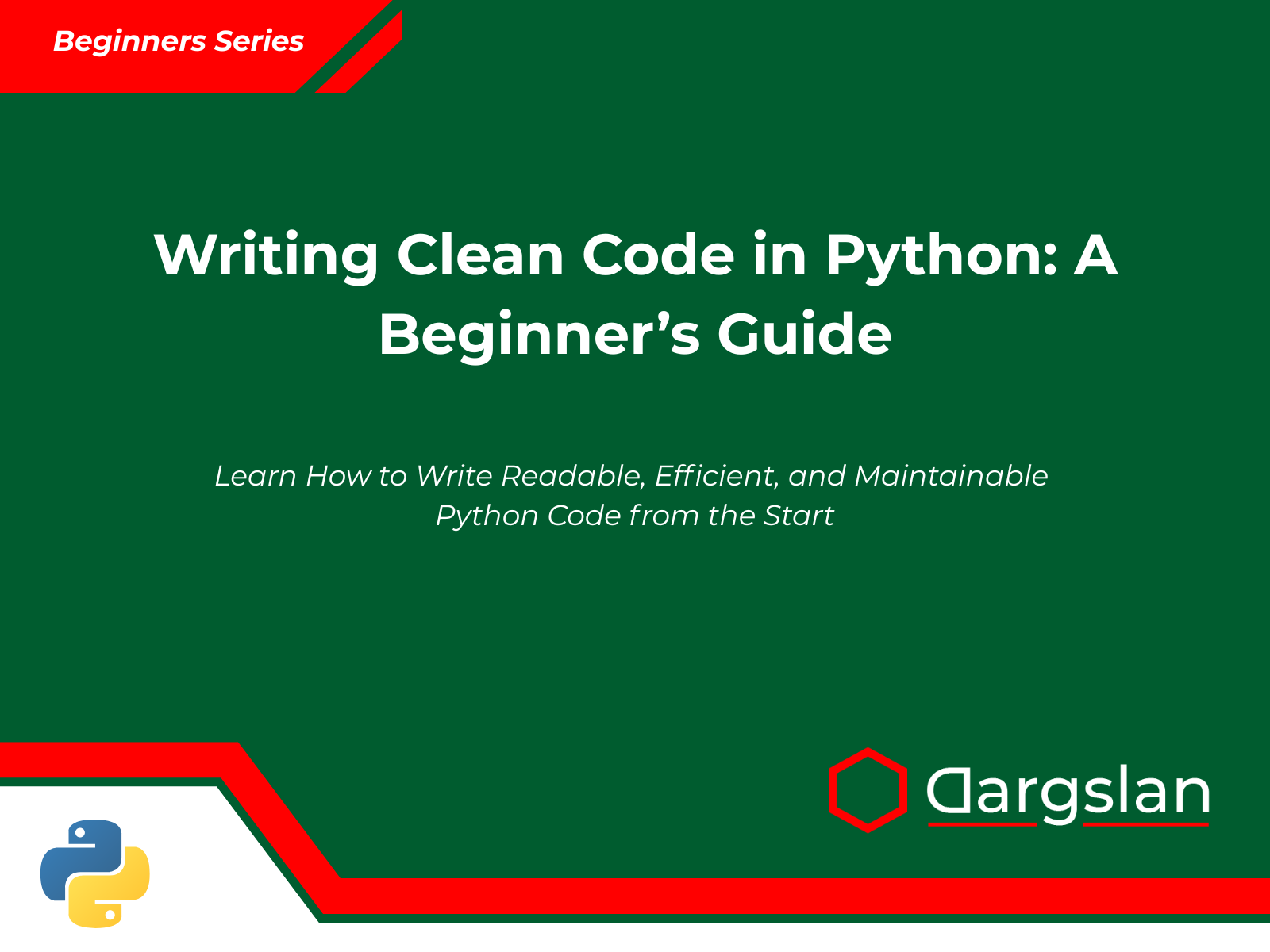
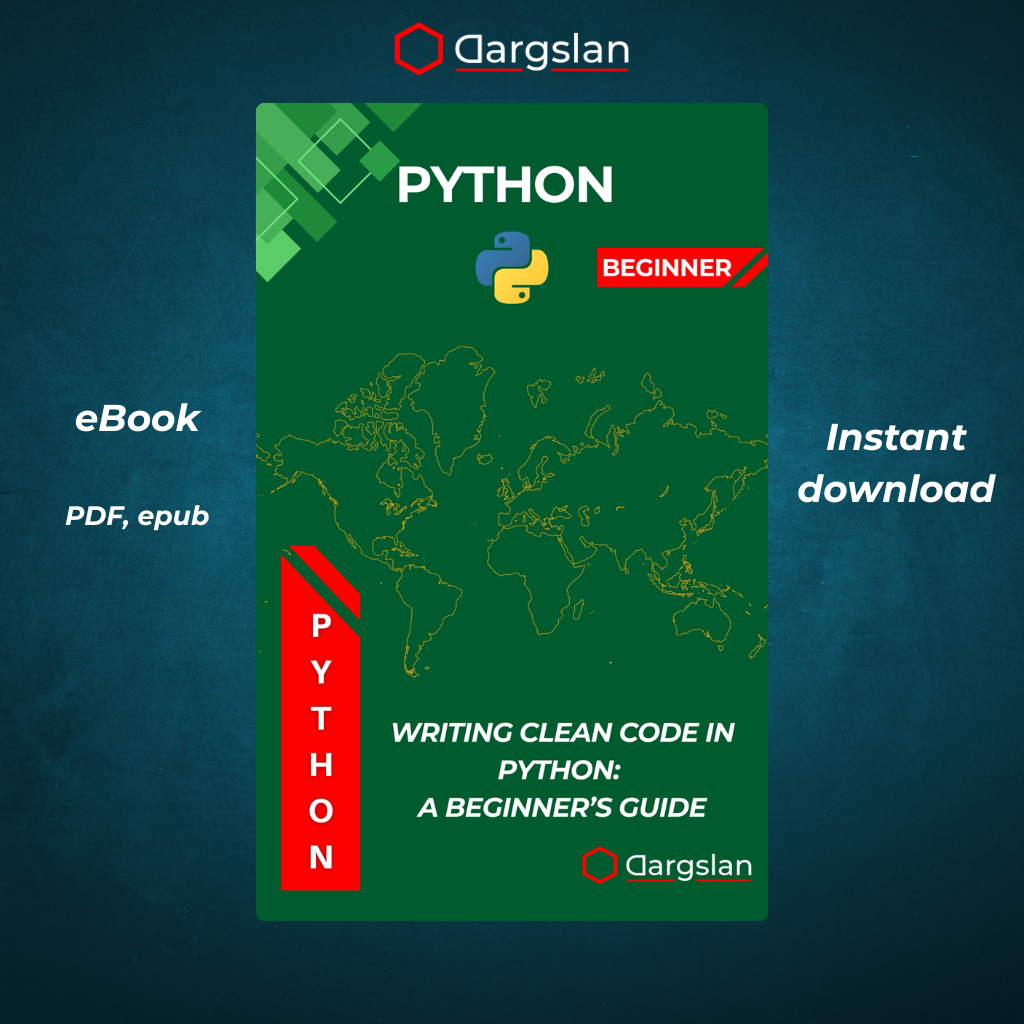
Writing Clean Code in Python: A Beginner’s Guide
Learn How to Write Readable, Efficient, and Maintainable Python Code from the Start
Book Review: Writing Clean Code in Python: A Beginner's Guide
Comprehensive Review of the Ultimate Python Clean Coding Resource for Beginners
Published: 2023 | Author: Dargslan | Pages: 231 | Rating: ★★★★★
Executive Summary
"Writing Clean Code in Python: A Beginner's Guide" is an exceptional resource that bridges the gap between basic Python syntax knowledge and professional-grade code development. Unlike many programming books that focus solely on functionality, this comprehensive guide emphasizes the importance of writing clean, maintainable, and efficient Python code from the outset of one's programming journey. Through ten meticulously crafted chapters and four practical appendices, author Dargslan takes readers on a transformative journey toward Python excellence, establishing coding habits that will serve developers throughout their careers.
Introduction: Why This Book Matters
In today's software development landscape, writing code that merely "works" is insufficient. As codebases grow and teams expand, the readability and maintainability of code become paramount concerns. "Writing Clean Code in Python" addresses this reality head-on by providing beginners with professional-level insights typically acquired only through years of experience.
The timing of this publication couldn't be more relevant. With Python consistently ranking among the most popular programming languages worldwide and being adopted across diverse domains from web development to data science, artificial intelligence, and automation, establishing proper coding practices from the beginning is invaluable. This book serves as the missing manual between basic Python tutorials and professional development practices.
Target Audience: Who Should Read This Book
The book positions itself primarily for:
- Beginners who have grasped basic Python syntax and are ready to elevate their coding standards
- Self-taught programmers looking to fill gaps in their knowledge about code quality
- Computer science students supplementing academic learning with practical coding standards
- Experienced developers from other languages transitioning to Python
- Development team leaders seeking a reference to establish coding standards
While the title emphasizes its beginner-friendly approach, intermediate Python developers will also find substantial value in the principles and Python-specific best practices presented throughout the book.
Book Structure and Organization
The book follows a logical progression through ten chapters, moving from fundamental concepts to increasingly sophisticated practices:
Foundation Chapters (1-2)
Establish the philosophical basis of clean code and tackle the surprisingly complex challenge of naming variables, functions, and classes appropriately.
Core Technique Chapters (3-5)
Focus on the building blocks of well-structured programs: functions, overall code organization, and documentation.
Problem-Solving Chapters (6-7)
Address common coding pitfalls and provide Python-specific guidance for everyday programming constructs.
Advanced Practice Chapters (8-10)
Elevate code quality through idiomatic Python, defensive programming, and systematic improvement processes.
The four appendices serve as valuable quick-reference materials that readers will likely return to repeatedly throughout their Python journey.
Chapter-by-Chapter Analysis
Chapter 1: What Is Clean Code and Why Does It Matter?
This foundational chapter introduces the philosophy behind clean code, drawing inspiration from industry luminaries while adapting concepts specifically to Python's unique characteristics. Rather than presenting clean code as merely an aesthetic preference, the author makes a compelling business case for code quality by illustrating how it impacts:
- Long-term maintenance costs
- Team onboarding and collaboration
- Bug identification and resolution
- Project scalability and evolution
Particularly effective is the chapter's use of before-and-after code examples that demonstrate the same functionality implemented in both problematic and clean ways, providing immediate visual reinforcement of the concepts.
Chapter 2: Naming Things the Right Way
Perhaps no single factor impacts code readability more than naming conventions, and this chapter tackles the challenge comprehensively. The author presents Python-specific naming guidelines that go beyond PEP 8 recommendations to include:
- Semantic naming strategies that convey purpose and behavior
- Contextually appropriate naming based on scope and usage
- Python's special naming patterns (like dunder methods)
- Common naming anti-patterns to avoid
The chapter's strength lies in its numerous real-world examples drawn from various domains, helping readers understand how thoughtful naming transforms code comprehension regardless of the application type.
Chapter 3: Writing Clear and Simple Functions
This chapter addresses function design with precision and clarity, covering:
- Function length and complexity considerations
- Single Responsibility Principle in the Python context
- Parameter design and default argument best practices
- Return value consistency and predictability
- Type hinting for improved clarity
Particularly valuable is the section on refactoring complex functions into smaller, more focused units while maintaining logical cohesion. The author presents practical refactoring workflows that help readers identify when and how to break down functions effectively.
Chapter 4: Structuring Code with Clarity
Moving beyond individual functions, this chapter explores how to organize code at the module and project levels:
- Package and module organization strategies
- Import management and dependency control
- Class design and interface considerations
- Balancing object-oriented and functional approaches in Python
- Code layering for separation of concerns
The author provides several project structure templates for different application types (CLI tools, web applications, data processing scripts), giving readers practical starting points for their own projects.
Chapter 5: Comments and Docstrings
This nuanced chapter navigates the sometimes contentious topic of code documentation with balance and wisdom:
- When comments are necessary versus when they indicate code smells
- Docstring formatting standards and tools
- Documentation generation workflows
- Writing documentation that tells "why" not just "what"
- Self-documenting code techniques that reduce documentation needs
The chapter's measured approach avoids dogmatic positions, instead providing context-sensitive guidance that helps readers develop good judgment about documentation needs.
Chapter 6: Avoiding Common Code Smells
This practical chapter catalogs frequent issues that plague Python codebases:
- Duplicate code patterns and extraction techniques
- Overly complex conditional logic
- Data clumps and primitive obsession
- Dead code identification and safe removal strategies
- Feature envy and inappropriate intimacy between modules
Each "smell" is presented with detection strategies, impact analysis, and refactoring approaches tailored to Python's capabilities. The integration of automated tools for code smell detection adds significant practical value.
Chapter 7: Clean Code with Lists, Loops, and Conditionals
Focusing on Python's bread-and-butter constructs, this chapter demonstrates how to elevate everyday coding:
- List comprehensions versus traditional loops—when each shines
- Generator expressions for memory efficiency
- Dictionary and set operations for cleaner algorithms
- Conditional expression refinement
- Loop optimization and readability balancing
The practical examples show both naive implementations and their cleaner counterparts, with performance characteristics noted where relevant.
Chapter 8: Using Pythonic Idioms
This chapter illuminates the path to truly native-feeling Python code:
- EAFP (Easier to Ask Forgiveness than Permission) versus LBYL (Look Before You Leap)
- Context managers for resource management
- Iteration protocols and custom iterables
- Effective use of magic methods
- Leveraging Python's standard library effectively
The author successfully demystifies what makes code "Pythonic," moving beyond vague descriptions to concrete patterns and principles that readers can immediately apply.
Chapter 9: Defensive Coding and Error Handling
Building robust applications requires anticipating problems, and this chapter provides a comprehensive approach:
- Exception design and hierarchy
- Custom exception classes
- Input validation strategies
- Assertion usage and limitations
- Logging best practices for troubleshooting
Particularly useful is the distinction drawn between different error types (programmer errors, operational errors, user errors) and the appropriate handling strategies for each.
Chapter 10: Code Review and Refactoring
The final chapter addresses the ongoing process of code improvement:
- Establishing effective code review practices
- Automating code quality checks
- Refactoring safely with tests
- Technical debt identification and management
- Continuous improvement workflows
The inclusion of both solo and team-oriented improvement processes makes this chapter valuable regardless of whether the reader works independently or collaboratively.
Appendices: Practical References
The four appendices provide exceptional reference material:
- PEP 8 Summary Cheat Sheet: A distilled version of Python's style guide with practical examples
- Before and After Examples: Extended code transformations showing clean code principles applied
- Top 10 Clean Coding Tips for Python Beginners: Quick wins for immediate code improvement
- Resources for Continued Learning: Curated books, courses, tools, and community resources
These appendices transform the book from a one-time read into an ongoing reference that will remain valuable as readers progress in their Python journey.
Distinctive Features and Highlights
Several aspects make this book stand out from similar programming guides:
Practical Examples Rooted in Diverse Domains
The code examples span multiple application domains including:
- Web development
- Data processing and analysis
- Automation scripts
- Command-line utilities
- API design
This diversity helps readers see how clean coding principles apply regardless of the specific Python application area, making the book relevant to the broad Python community.
Balanced Approach to Clean Code
Rather than promoting rigid rules, the author presents principles with context, explaining when certain guidelines might be bent for practical reasons. This nuanced approach prepares readers for real-world coding rather than idealized scenarios.
Progressive Skill Building
Each chapter builds upon previous concepts, creating a cohesive learning journey. Techniques introduced early reappear in later chapters in more sophisticated forms, reinforcing key ideas through spaced repetition.
Focus on Python's Unique Characteristics
Unlike generic clean code books adapted to Python, this text is built from the ground up with Python's philosophy, community standards, and technical capabilities in mind. The result is advice that feels natural within the Python ecosystem.
Integration of Modern Python Features
The book incorporates Python's modern features like:
- Type hints for improved code clarity
- f-strings for readable string formatting
- Walrus operator (:=) usage guidance
- Structural pattern matching (match/case)
- Dataclass implementation for cleaner data containers
This modern approach ensures the book remains relevant for current Python development.
Critical Analysis: Strengths and Limitations
Strengths
-
Beginner-Friendly Without Oversimplification: The book maintains accessibility while introducing sophisticated concepts, never talking down to the reader.
-
Practical Over Theoretical: While theory is addressed, the focus remains squarely on applicable techniques that provide immediate value.
-
Python-Specific Guidance: Rather than generic programming advice, all recommendations are tailored to Python's unique characteristics.
-
Balanced Perspective: The author acknowledges tradeoffs in different approaches rather than prescribing one-size-fits-all solutions.
-
Progressive Complexity: Concepts build naturally, with early chapters establishing foundations for more advanced topics.
Potential Limitations
-
Limited Advanced Algorithm Coverage: While the book covers clean code for basic algorithms, more complex algorithmic optimization receives less attention.
-
Framework-Specific Patterns Minimized: The book focuses on "vanilla" Python rather than extensively covering framework-specific patterns (Django, Flask, etc.).
-
Limited Coverage of Concurrent Programming: While threading and asyncio are mentioned, more extensive coverage of clean code in concurrent contexts would benefit some readers.
These limitations are reasonable given the book's scope and focus on beginners, but readers should be aware of these boundaries.
Comparison with Similar Titles
Compared to other Python books in the market:
-
"Clean Code" by Robert C. Martin: While an industry standard, it's not Python-specific and lacks modern Python idioms.
-
"Fluent Python" by Luciano Ramalho: More advanced and comprehensive but potentially overwhelming for beginners.
-
"Python Tricks" by Dan Bader: Offers useful idioms but doesn't provide the systematic approach to clean code found in this book.
-
"Effective Python" by Brett Slatkin: Similar in spirit but targets intermediate to advanced developers rather than beginners.
"Writing Clean Code in Python" fills an important gap for beginners ready to level up their Python skills with a focus on code quality from the start.
Real-World Applications
The principles in this book apply directly to several common Python development scenarios:
For Data Scientists and Analysts
The book's guidance on function organization and documentation is invaluable for creating reproducible, maintainable data pipelines and analysis notebooks.
For Web Developers
Clean code principles applied to API design and request handling lead to more maintainable backend systems with clearer interfaces.
For Automation Engineers
The defensive coding and error handling chapters provide essential guidance for building robust automation scripts that gracefully handle edge cases.
For Open Source Contributors
The code review and refactoring chapter prepares readers to participate effectively in collaborative open source projects.
Learning Outcomes and Skill Development
Readers who thoroughly engage with this book can expect to develop:
- Critical evaluation skills for assessing code quality beyond mere functionality
- Practical refactoring techniques for improving existing code incrementally
- Design sensibilities that lead to naturally more maintainable code structures
- Communication abilities through code that clearly expresses intent
- Professional coding habits that align with industry best practices
These skills represent a significant competitive advantage in the job market and contribute to long-term career growth.
Conclusion: Final Assessment
"Writing Clean Code in Python: A Beginner's Guide" achieves the rare balance of being both accessible to newcomers and valuable to more experienced developers. By focusing specifically on the intersection of clean code principles and Python's unique characteristics, the book fills an important gap in the Python literature.
For beginners who have mastered basic syntax and are ready to write professional-quality code, this book is an essential next step. For intermediate developers, it provides a structured approach to leveling up coding practices and addressing technical debt in existing projects.
The book's emphasis on practical examples, balanced viewpoints, and Python-specific guidance makes it stand out in an increasingly crowded field of programming texts. It successfully delivers on its promise to help readers write Python code that is not only functional but also a joy to read and maintain.
Whether read cover-to-cover as a learning journey or used as a reference for specific clean coding challenges, this book represents an outstanding value for anyone serious about Python development. It earns our highest recommendation for Python programmers at all levels who want to elevate their code quality.
Rating Breakdown
- Content Quality: ★★★★★
- Code Examples: ★★★★★
- Practical Application: ★★★★★
- Beginner Accessibility: ★★★★☆
- Value for Money: ★★★★★
Overall Rating: ★★★★★ (4.9/5)
About the Reviewer: This review was prepared by a Python development professional with over a decade of experience in software engineering, technical writing, and developer education.
Disclosure: This review is based solely on the book's content and does not reflect any commercial relationship with the author or publisher.
Keywords: Python clean code, Python best practices, beginner Python book, Python code quality, Python programming guide, readable Python code, maintainable code Python, Python development standards, Python coding conventions, PEP 8 guide, Python function design, Python project structure, Pythonic code examples, refactoring Python code, Python code review
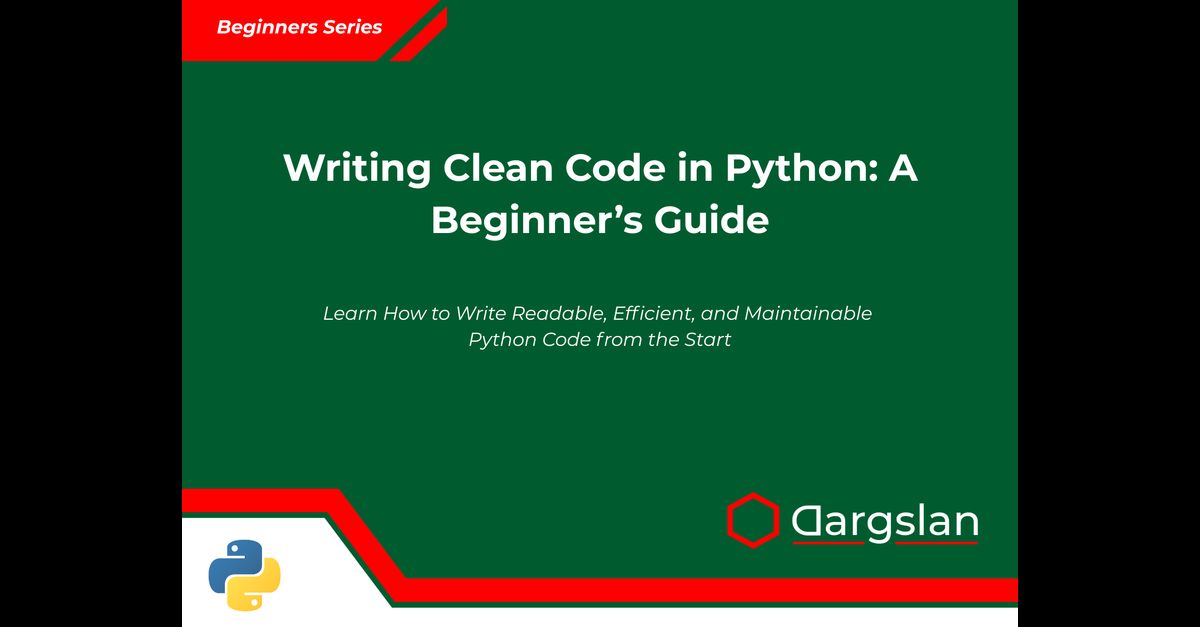
Writing Clean Code in Python: A Beginner’s Guide