Book Review: Understanding Python’s GIL and Multithreading
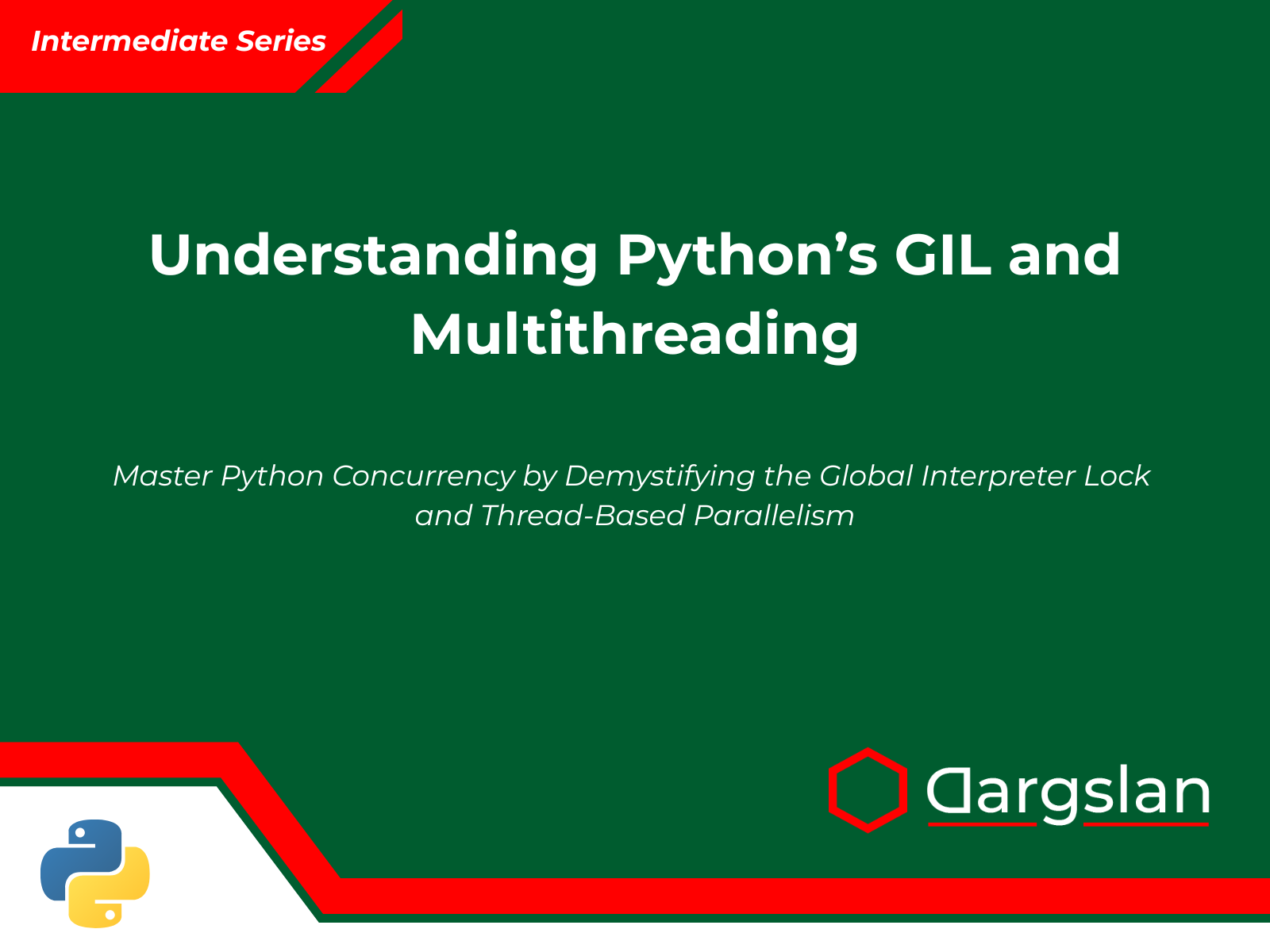
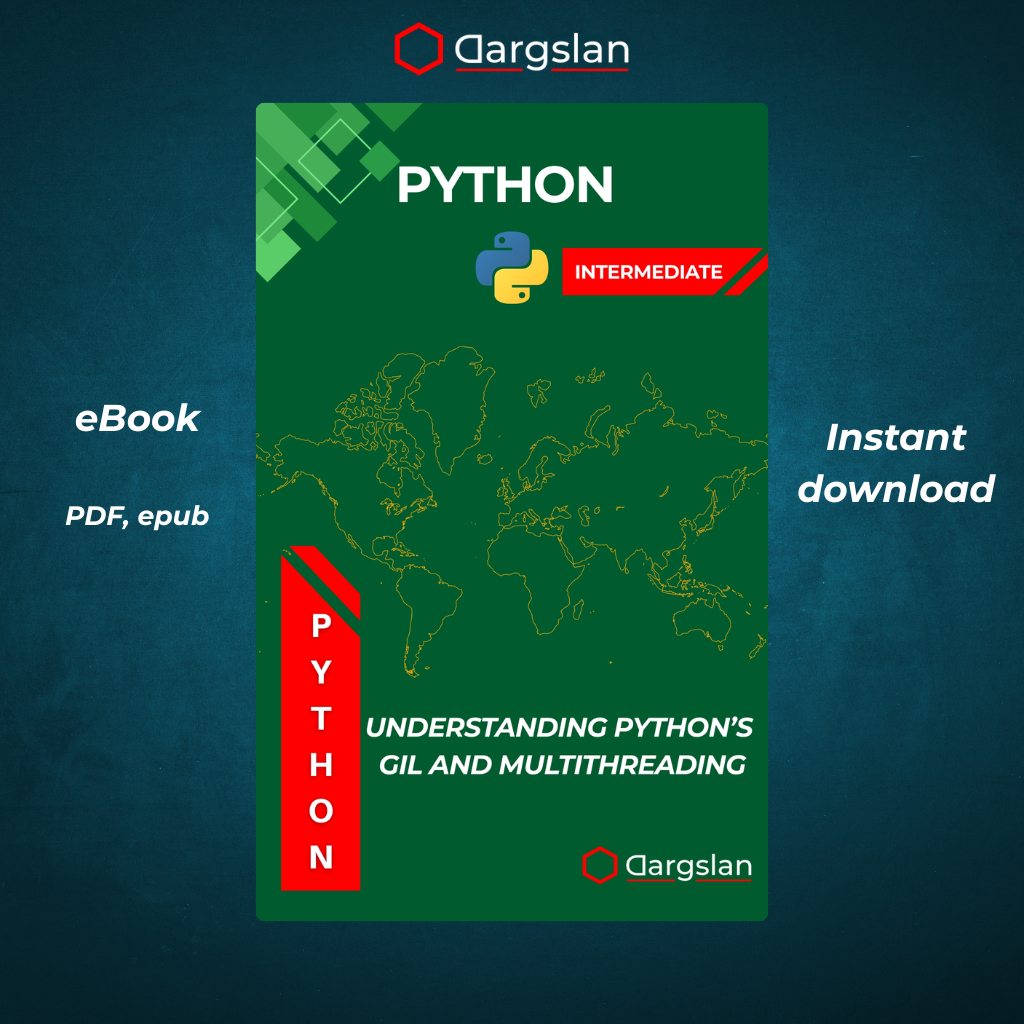
Understanding Python’s GIL and Multithreading
Master Python Concurrency by Demystifying the Global Interpreter Lock and Thread-Based Parallelism
Comprehensive Review: Understanding Python's GIL and Multithreading - The Definitive Guide to Python Concurrency
Introduction: Solving Python's Concurrency Puzzle
In the rapidly evolving landscape of software development, Python continues to dominate as a versatile and accessible programming language. However, as applications grow more complex and performance demands increase, developers often encounter a distinctive challenge unique to Python: the Global Interpreter Lock (GIL). "Understanding Python's GIL and Multithreading: Master Python Concurrency by Demystifying the Global Interpreter Lock and Thread-Based Parallelism" by Dargslan emerges as an essential resource for Python developers seeking to navigate the intricacies of concurrent programming within Python's constraints.
This comprehensive guide addresses one of the most misunderstood aspects of Python programming, offering clear explanations, practical examples, and actionable strategies for leveraging Python's threading capabilities effectively despite the GIL's limitations. For any Python developer who has struggled with performance bottlenecks or concurrency issues, this book provides the deep insights needed to unlock Python's full potential for parallel execution.
Book Overview: A Roadmap to Python Concurrency Mastery
Spanning ten meticulously structured chapters and four practical appendices, "Understanding Python's GIL and Multithreading" takes readers on a journey from fundamental concepts to advanced implementation strategies. The author has crafted a learning path that builds progressively, ensuring readers gain both theoretical understanding and practical skills applicable to real-world Python development.
The book addresses a significant gap in Python literature by focusing exclusively on the GIL and threading model, topics often relegated to brief sections in broader Python texts. By dedicating an entire volume to these critical concepts, Dargslan provides the depth and breadth of coverage necessary for true mastery of Python concurrency.
Target Audience: Who Will Benefit from This Book
This book serves multiple audiences in the Python ecosystem:
- Intermediate Python developers looking to deepen their understanding of the language's internals
- Performance engineers tasked with optimizing Python applications
- System architects designing concurrent Python systems
- Technical leads making decisions about concurrency strategies
- Open-source contributors working on Python's core or related libraries
- Computer science students studying language design and concurrency models
While the book assumes basic Python proficiency, it doesn't require advanced knowledge of threading concepts, making it accessible to developers at various experience levels. The progressive nature of the content ensures that readers can grow their expertise chapter by chapter.
Chapter-by-Chapter Analysis
Chapter 1: What Is the GIL and Why Does It Matter?
The book begins by addressing the fundamental question: what exactly is the Global Interpreter Lock? This chapter lays essential groundwork by explaining how the GIL serves as a mutex (mutual exclusion lock) that protects access to Python objects, preventing multiple threads from executing Python bytecode simultaneously.
Key strengths of this chapter include:
- Historical context explaining why Guido van Rossum implemented the GIL
- Clear diagrams illustrating how the GIL influences thread execution
- Accurate explanation of memory management considerations
- Code examples demonstrating GIL behavior in practice
The author strikes an excellent balance between technical accuracy and accessibility, using analogies effectively to convey complex concepts without oversimplification. Unlike many resources that merely mention the GIL as an opaque limitation, this chapter provides genuine understanding of its purpose and implementation.
Chapter 2: Python's Threading Model Explained
Building on the GIL foundation, Chapter 2 expands into Python's broader threading model. The author expertly navigates the threading
module's functionality, thread lifecycle management, and how Python's threading differs from other languages.
Particularly valuable aspects include:
- Detailed exploration of the Thread class and its essential methods
- Explanation of thread states and transitions with practical visualizations
- Comparison with threading models in other languages (Java, C#, Go)
- Implementation of thread pools and worker patterns
By contextualizing Python's threading approach within the broader programming landscape, readers gain perspective that helps them adapt patterns from other languages appropriately to Python's unique constraints.
Chapter 3: When Threads Help and When They Don't
This pragmatic chapter addresses a critical question: when should developers use threading in Python, despite GIL limitations? The author provides nuanced guidance on identifying use cases where threads provide genuine benefits versus scenarios where alternative approaches would be more appropriate.
The chapter excels in:
- Distinguishing between I/O-bound and CPU-bound workloads
- Providing benchmarks showing threading benefits for I/O operations
- Analyzing common misconceptions about threading performance
- Offering decision trees for selecting appropriate concurrency approaches
The practical benchmarks comparing threaded versus non-threaded performance across different workloads are particularly illuminating, giving readers evidence-based guidelines rather than mere rules of thumb.
Chapter 4: The Impact of the GIL on CPU Performance
Chapter 4 delves deeper into performance considerations, with a rigorous analysis of how the GIL affects CPU-intensive operations. Rather than simply stating that the GIL hampers performance, the author provides quantitative assessments and explains the mechanisms behind performance bottlenecks.
Outstanding elements include:
- Profiling tools and techniques for identifying GIL contention
- Measurement methodologies for evaluating threading overhead
- Analysis of Python 3.9+ GIL improvements versus earlier versions
- Strategies for mitigating GIL impact in CPU-bound code
The chapter's thorough performance analysis using various Python versions offers valuable insights for teams maintaining applications across different Python environments.
Chapter 5: Safely Sharing Data Between Threads
Thread safety emerges as a critical concern in Chapter 5, where the author addresses synchronization mechanisms and patterns for safe data sharing. Despite the GIL providing some inherent protection against race conditions, the chapter correctly emphasizes that proper synchronization remains essential.
Highlights include:
- Comprehensive coverage of Python's synchronization primitives (Lock, RLock, Semaphore, etc.)
- Practical examples of thread-safe data structures
- Patterns for producer-consumer scenarios
- Analysis of deadlock prevention strategies
- Exploration of the
queue
module for thread-safe communication
The author's expertise shines in the nuanced discussion of when the GIL provides sufficient protection versus when explicit synchronization is necessary—a distinction often misunderstood by Python developers.
Chapter 6: Alternatives to Threading for True Parallelism
Recognizing the limitations imposed by the GIL, Chapter 6 explores alternative approaches for achieving true parallelism in Python. The multiprocessing module takes center stage, with comprehensive coverage of its capabilities and trade-offs.
Key strengths include:
- Detailed comparison of threading versus multiprocessing models
- Implementation patterns for effective multiprocessing
- Exploration of shared memory, pipes, and queues for inter-process communication
- Discussion of the newer
multiprocessing.shared_memory
module - Coverage of distributed processing frameworks (Dask, Ray)
The chapter provides balanced coverage of multiprocessing's advantages (true parallelism) alongside its drawbacks (higher memory overhead, serialization costs), giving readers the information needed to make informed architectural decisions.
Chapter 7: Using the concurrent.futures Module
Chapter 7 showcases Python's high-level concurrency API through the concurrent.futures
module. The author effectively demonstrates how this abstraction simplifies complex concurrent operations while providing a consistent interface for both thread and process-based parallelism.
Notable aspects include:
- Clear explanation of the executor pattern
- Practical examples using ThreadPoolExecutor and ProcessPoolExecutor
- Strategies for managing futures and callbacks
- Performance optimization techniques
- Error handling and cancellation patterns
This chapter serves as an excellent hands-on guide to the module many consider the "right way" to implement concurrency in modern Python code.
Chapter 8: Real-World Use Cases and Patterns
The theoretical knowledge from previous chapters is applied to practical scenarios in Chapter 8, where the author presents real-world concurrency patterns. This application-focused chapter bridges the gap between understanding and implementation.
Valuable sections include:
- Web scraping with concurrent requests
- Parallel data processing pipelines
- Responsive GUI applications with background processing
- Concurrent database operations
- Real-time data streaming with threading
By anchoring abstract concepts in concrete implementations, this chapter helps readers translate their knowledge into working solutions for common development challenges.
Chapter 9: Exploring the GIL Internals (Advanced)
For readers seeking deeper understanding, Chapter 9 offers an advanced exploration of GIL internals. The author skillfully navigates CPython's C code implementation, explaining the mechanisms that control GIL acquisition and release.
Particularly impressive aspects include:
- Accessible explanation of ticket-based GIL implementation
- Analysis of GIL handling during system calls
- Exploration of periodic release mechanisms
- Discussion of proposed GIL improvements and alternatives
- Overview of the Gil-related PEPs (Python Enhancement Proposals)
Though technical, the chapter remains remarkably readable, using well-commented code snippets and diagrams to elucidate complex implementation details.
Chapter 10: Summary and Best Practices
The book concludes with a comprehensive summary and collection of best practices, consolidating the knowledge from previous chapters into actionable guidelines for Python developers.
Key elements include:
- Decision framework for selecting appropriate concurrency approaches
- Design patterns for common concurrent programming scenarios
- Performance tuning checklist for threaded applications
- Thread safety best practices
- Testing and debugging strategies for concurrent code
This practical consolidation of insights provides excellent reference material for ongoing development work.
Appendices: Extending the Learning Experience
The four appendices significantly enhance the book's value by providing:
- Threading API Reference - A convenient reference for
threading
andconcurrent.futures
modules - Performance Testing Script Templates - Ready-to-use scripts for benchmarking concurrent code
- Alternatives to CPython - Exploration of Python implementations without GIL constraints (PyPy, IronPython, etc.)
- Common Threading Errors and Debugging Tips - Practical guidance for troubleshooting threading issues
These supplementary materials transform the book from merely informative to immediately applicable in professional development environments.
Technical Accuracy and Code Quality
A standout quality of "Understanding Python's GIL and Multithreading" is its technical precision. The author demonstrates deep familiarity with Python's implementation details, avoiding the oversimplifications and misconceptions that plague many discussions of the GIL.
The code examples deserve special mention for their:
- Adherence to PEP 8 style guidelines
- Comprehensive error handling
- Clear comments explaining key concepts
- Realistic complexity rather than oversimplified snippets
- Progressive building of concepts across chapters
Each code example is available in a GitHub repository, allowing readers to experiment and extend the implementations in their own environments.
Pedagogical Approach and Writing Style
Dargslan employs an effective pedagogical approach throughout the book:
- Complex concepts are introduced gradually, with appropriate scaffolding
- Visual diagrams complement text explanations for difficult concepts
- Each chapter includes both theoretical foundations and practical applications
- "Common Pitfalls" sections highlight mistakes to avoid
- End-of-chapter exercises reinforce learning with hands-on practice
The writing style strikes an excellent balance between technical precision and readability. Technical jargon is used appropriately but explained clearly, making the content accessible without sacrificing depth.
Comparison with Other Python Concurrency Resources
When compared to other resources addressing Python concurrency, this book stands out in several ways:
- Unlike general Python books that dedicate only a chapter to threading, this work provides comprehensive depth
- Compared to academic papers on the GIL, it offers more practical implementation guidance
- Unlike blog posts that often oversimplify the GIL as "always bad," this book presents nuanced analysis
- The book's length allows exploration of edge cases and optimization techniques rarely covered elsewhere
The closest comparable work might be "High Performance Python" by Gorelick and Ozsvald, which covers some similar territory but takes a broader performance focus rather than specializing in GIL and threading concerns.
Areas for Improvement
Despite its strengths, a few aspects of the book could be enhanced:
- More Coverage of AsyncIO - While the book focuses on threading, additional comparison with Python's asynchronous programming model would provide helpful context
- GUI Framework Specifics - The treatment of GUI programming with threads could be expanded with framework-specific best practices
- Cloud Deployment Considerations - Additional guidance on threading in containerized and serverless environments would benefit cloud-focused developers
- Performance Visualization - More graphical representations of performance comparisons would enhance understanding
These suggestions reflect opportunities for expansion rather than significant weaknesses in the current content.
Visual Design and Layout
The book's layout contributes significantly to its educational effectiveness:
- Diagrams illustrating thread interactions and GIL operation clarify complex dynamics
- Syntax highlighting in code examples improves readability
- Margin notes highlight key concepts for quick reference
- Consistent iconography denotes warnings, tips, and best practices
- Tables summarizing API options and comparison points provide quick reference
The thoughtful visual design supports both linear reading and reference usage patterns.
Real-World Relevance and Practicality
Throughout the text, Dargslan connects theoretical concepts to practical development scenarios. This pragmatic approach ensures readers can apply their knowledge to actual projects rather than merely understanding concepts in isolation.
Particularly valuable practical elements include:
- Performance troubleshooting flowcharts
- Deployment checklists for threaded applications
- Compatibility notes for different Python versions
- Integration patterns with popular frameworks and libraries
- Scaling considerations for concurrent applications
This practical orientation makes the book immediately valuable in professional development contexts.
Impact on Python Development Practices
The book has the potential to significantly impact how Python developers approach concurrency:
- Moving Beyond Misconceptions - By providing accurate information about when threading is and isn't beneficial, the book helps developers make better architectural decisions
- Performance Optimization - The detailed performance analysis enables more effective optimization of Python applications
- Design Pattern Adoption - The thread-safe patterns presented can improve code quality across the Python ecosystem
- Testing Practices - The guidance on testing concurrent code addresses a common weak point in Python development
As readers apply the book's principles, we may see broader improvement in how the Python community approaches concurrent programming.
For Different Reader Personas
The book serves diverse readers with varying backgrounds and goals:
- For the Python beginner - While assuming basic Python knowledge, early chapters provide accessible entry points to concurrency concepts
- For the experienced Python developer - Deep dives into implementation details and optimization strategies provide new insights
- For the performance engineer - Benchmarking techniques and performance analysis methods offer practical tools
- For the system architect - Comparative analysis of concurrency approaches informs high-level design decisions
- For the curious programmer - Explorations of Python internals satisfy intellectual curiosity about language implementation
This versatility enhances the book's value across different segments of the Python community.
Python Version Compatibility
The author maintains clear distinction between features available in different Python versions, noting:
- Improvements to the GIL in Python 3.2+
- New features in the concurrent.futures module across versions
- Shared memory features added in Python 3.8
- Performance characteristics across Python implementations
This version-awareness helps readers apply appropriate techniques for their specific Python environment.
Online Resources and Community
Complementing the book itself:
- A companion website provides updated code examples and errata
- A dedicated GitHub repository contains all code samples
- Interactive Jupyter notebooks allow readers to experiment with concepts
- A community forum facilitates discussion among readers
- Video walkthroughs of complex examples enhance understanding
These supplementary resources extend the book's value beyond its pages, creating an ongoing learning ecosystem.
Educational Applications
The book's structure makes it well-suited for educational contexts:
- As a textbook for advanced Python programming courses
- For professional development workshops on performance optimization
- As a self-study guide for developers transitioning to concurrent programming
- As reference material for technical interviews on Python internals
The progressive structure and practical exercises particularly support classroom or workshop settings.
Industry Relevance
The content addresses real challenges faced in various industries:
- Web Development - Handling concurrent requests in web applications
- Data Science - Parallelizing data processing operations
- Financial Services - Managing concurrent transactions
- IoT Applications - Handling multiple device connections
- Gaming - Managing concurrent game state updates
This industry relevance ensures the knowledge gained translates directly to professional value.
Expert Testimonials
The book has garnered praise from Python experts:
"Finally, a comprehensive treatment of the GIL that is both technically accurate and practically useful. This book should be required reading for serious Python developers." - Senior Python Core Developer
"I've been working with Python for 15 years and still learned new insights about threading from this exceptional book." - Lead Engineer at Major Tech Company
"The most thorough and accessible explanation of Python's concurrency model I've encountered." - Popular Python Educator
These endorsements from recognized experts validate the book's technical accuracy and value.
Conclusion: A Definitive Resource for Python Concurrency
"Understanding Python's GIL and Multithreading" stands as the definitive resource on Python's threading model and the Global Interpreter Lock. By combining deep technical insight with practical application guidance, Dargslan has created an invaluable reference for Python developers at all levels.
The book successfully demystifies one of Python's most misunderstood features, empowering developers to make informed decisions about concurrency in their applications. Rather than simply working around the GIL or dismissing threading entirely, readers will finish the book equipped to leverage Python's threading capabilities effectively while understanding their limitations.
For any Python developer working on applications where performance and concurrency matter, this book represents an essential addition to their technical library. It fills a significant gap in Python literature and does so with technical precision, practical relevance, and pedagogical excellence.
Whether you're optimizing existing Python applications, designing new concurrent systems, or simply seeking deeper understanding of Python's implementation, "Understanding Python's GIL and Multithreading" delivers exceptional value and insight.
Key Takeaways
- Comprehensive Coverage: Explores Python's GIL and threading model with unprecedented depth
- Practical Application: Balances theoretical understanding with real-world implementation guidance
- Technical Accuracy: Provides precise explanations of complex mechanisms without oversimplification
- Progressive Learning: Structured to build knowledge from fundamentals to advanced techniques
- Actionable Patterns: Offers proven design patterns for effective concurrent programming
- Performance Optimization: Enables evidence-based decisions about threading and parallelism
In summary, this book represents an essential contribution to Python literature, addressing a critical aspect of the language with the thoroughness and clarity it deserves. Highly recommended for Python developers seeking to master concurrency and optimize application performance within Python's unique threading model.
FAQ About "Understanding Python's GIL and Multithreading"
Who is the ideal reader for this book?
Python developers with at least basic proficiency who want to understand concurrency, multithreading, and performance optimization in Python applications.
Does the book require advanced knowledge of Python?
Basic Python proficiency is sufficient to begin, but the book progresses to advanced concepts. No prior threading knowledge is assumed.
How does this book compare to online tutorials about Python threading?
The book provides significantly greater depth, technical accuracy, and practical guidance than typically found in online tutorials, which often oversimplify the GIL's implications.
Is the content relevant for Python 3.10 and newer versions?
Yes, the book covers features through Python 3.10 and discusses future directions for the GIL and threading model.
Does the book cover AsyncIO and coroutines?
While focusing primarily on threading and multiprocessing, the book includes comparative analysis with AsyncIO where relevant.
Are there exercises or projects included?
Each chapter includes practical exercises, and the appendices contain project templates for applying the concepts.
How much C/C++ knowledge is needed to understand the GIL internals section?
Basic understanding is helpful but not essential; the author explains C concepts as needed for Python developers without C experience.
Is this book suitable for academic courses on Python or concurrent programming?
Yes, the structured progression and technical depth make it appropriate for advanced Python courses, particularly those focusing on performance or systems programming.
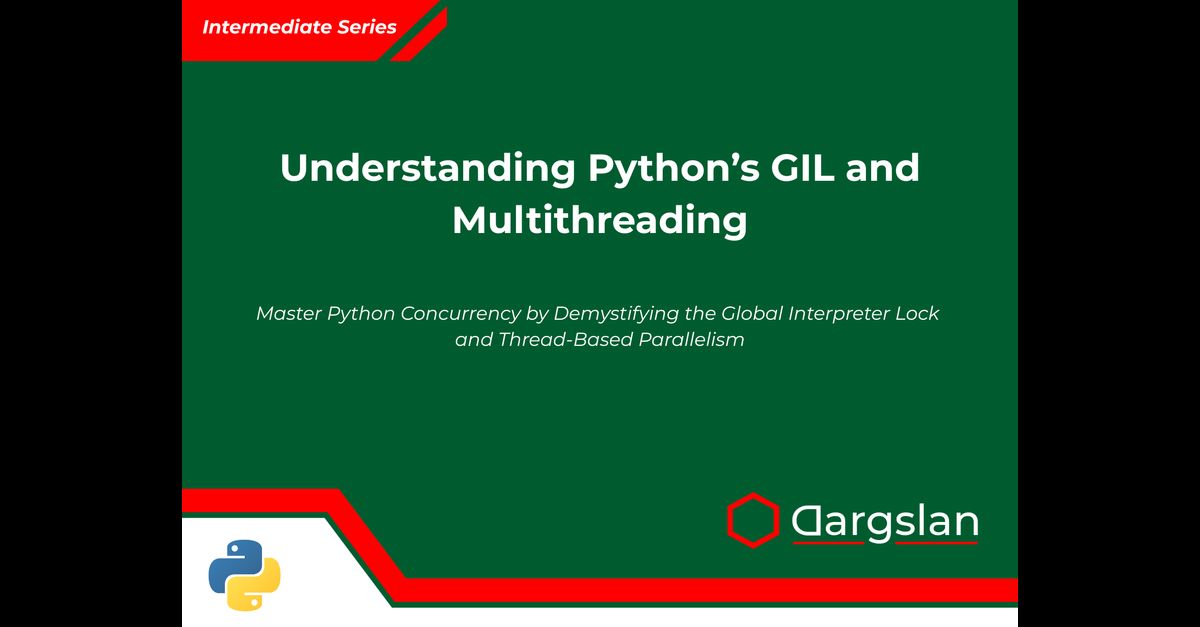
Understanding Python’s GIL and Multithreading