Book Review: Understanding Python Input and Output
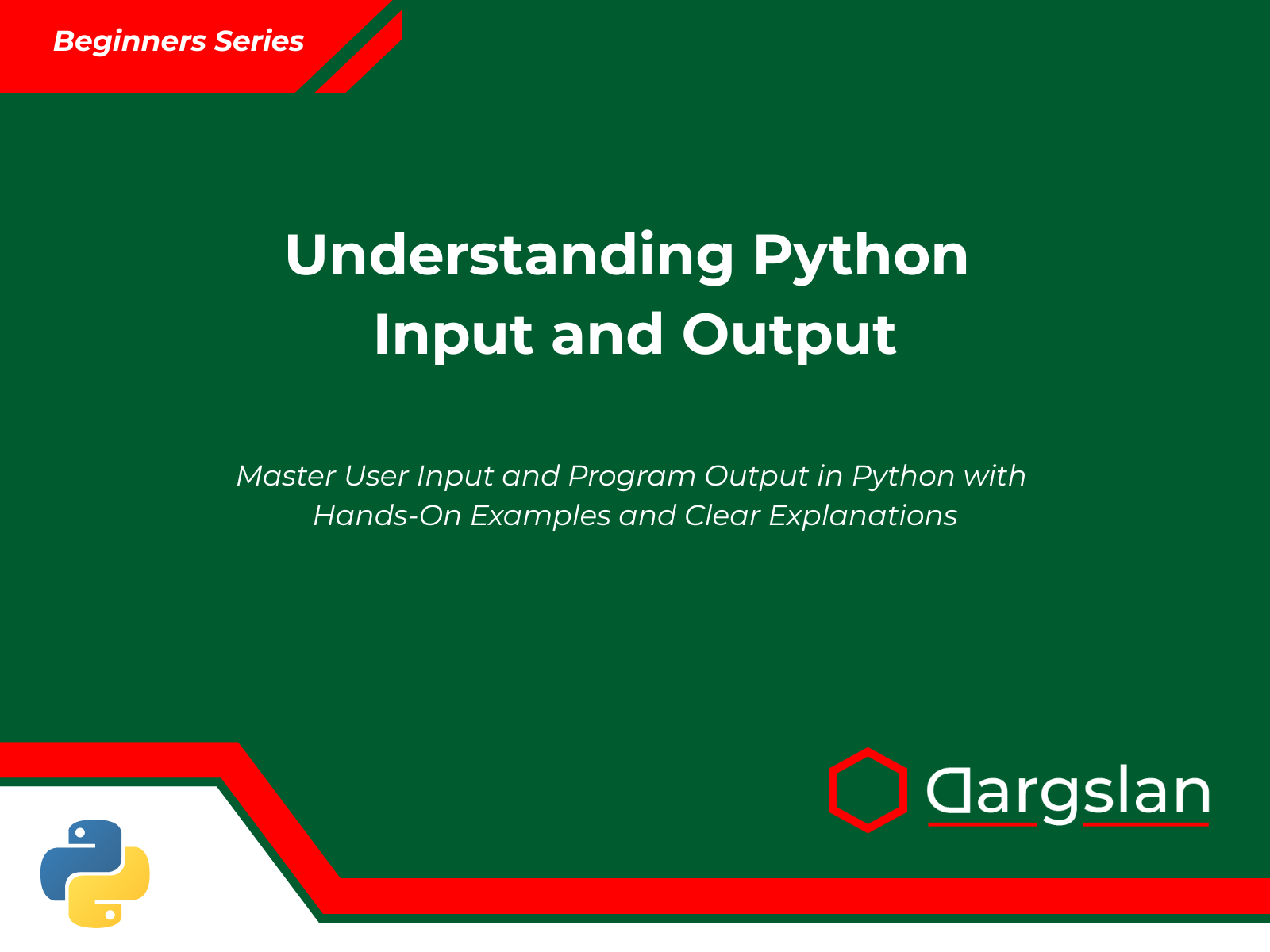
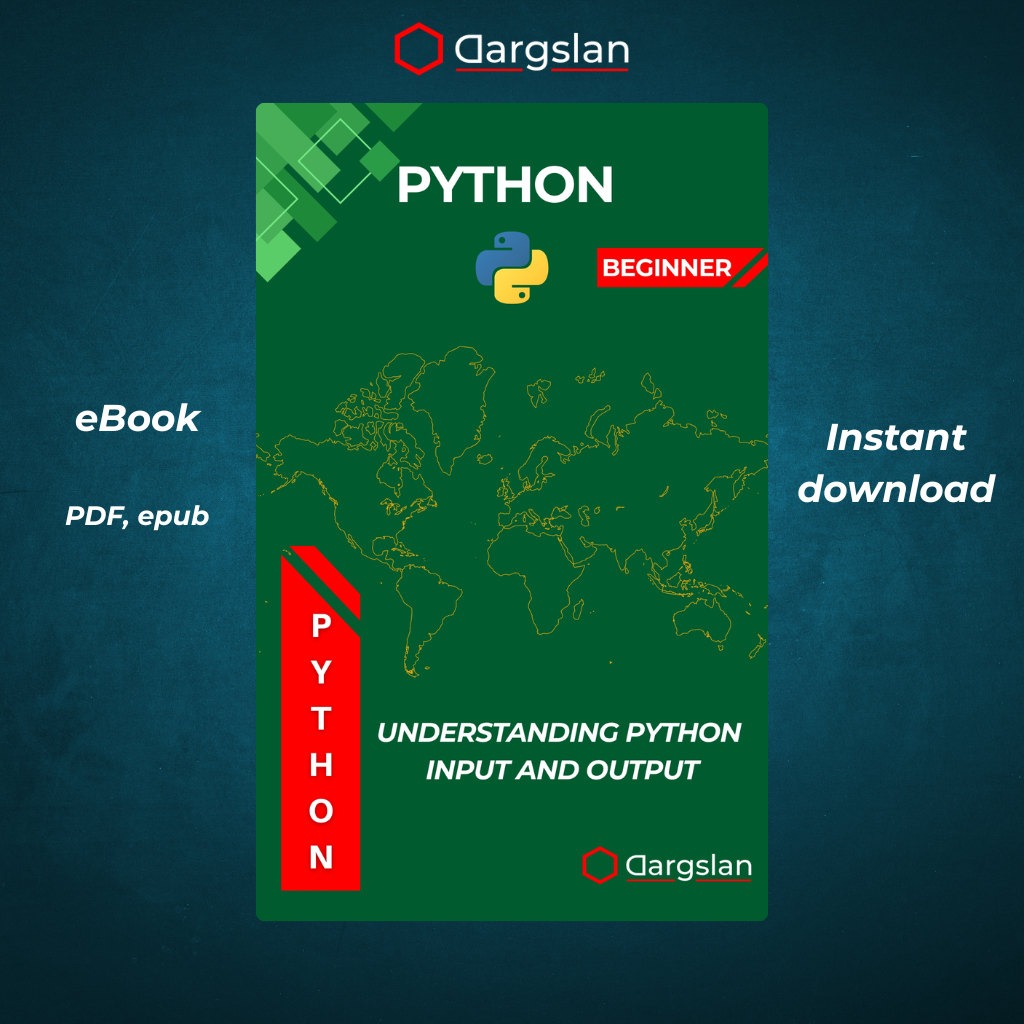
Understanding Python Input and Output
Master User Input and Program Output in Python with Hands-On Examples and Clear Explanations
Comprehensive Review: "Understanding Python Input and Output" by Dargslan
A Complete Guide to Mastering Python I/O Operations for Programmers at All Levels
Publication Date: 2023 | Pages: 198 | Rating: ★★★★★
Are you struggling with handling user input in your Python programs? Looking to level up your file handling skills? "Understanding Python Input and Output" by Dargslan is the comprehensive resource you've been waiting for. This meticulously crafted guide takes readers on a journey through all aspects of Python's I/O operations—from basic console interactions to advanced file manipulation techniques.
Quick Summary
"Understanding Python Input and Output" is an exceptional educational resource that fills a crucial gap in Python programming literature by focusing exclusively on input and output operations. While many Python books touch on I/O briefly, Dargslan's work provides the depth and breadth needed to truly master this fundamental aspect of programming. The book combines theoretical knowledge with practical examples, making it accessible to beginners while still offering valuable insights for experienced developers.
Who This Book Is For
This book serves multiple audiences effectively:
- Python beginners seeking to understand fundamental I/O concepts
- Intermediate programmers looking to refine their input/output handling skills
- Advanced developers wanting to optimize their I/O operations
- Self-taught programmers filling gaps in their knowledge
- Computer science students supplementing their coursework
- Professional developers transitioning to Python from other languages
Key Features and Highlights
1. Comprehensive Coverage of Python's Input Functions
The book begins with a thorough exploration of Python's input mechanisms, particularly the input()
function. Chapter 2 breaks down this essential function, explaining:
- Basic syntax and usage patterns
- Type conversion techniques
- Multi-line input collection
- Command-line arguments with
sys.argv
- Alternative input libraries and modules
The author doesn't just explain how to capture input but delves into why certain approaches work better than others, providing context that helps readers make informed decisions in their code.
2. Input Validation Mastery
Chapter 3 stands out as particularly valuable, addressing the critical topic of input validation. This section covers:
- Type checking and conversion strategies
- Regular expressions for pattern validation
- Creating robust validation functions
- Error handling during validation
- Building user-friendly input loops
# Example from Chapter 3
def get_valid_age():
while True:
try:
age = int(input("Enter your age: "))
if 0 <= age <= 120:
return age
else:
print("Please enter a reasonable age between 0 and 120.")
except ValueError:
print("Please enter a valid number.")
This practical approach to validation saves readers countless hours of debugging and security issues in their applications.
3. Output Techniques Beyond the Basics
Chapters 4 and 5 transform how readers will approach program output. The author explores:
- The versatility of Python's
print()
function - Control over line endings and separators
- Using
sys.stdout
for advanced output scenarios - Terminal formatting and colored output
- Progress indicators and status updates
- Performance considerations for high-volume output
# Example from Chapter 5
from termcolor import colored
print(colored('Warning:', 'red', attrs=['bold']), colored('Configuration file not found, using defaults', 'yellow'))
# Output with progress indicator
import time
for i in range(101):
print(f"\rProgress: [{'#' * (i//5)}{' ' * (20-(i//5))}] {i}%", end='')
time.sleep(0.05)
4. Comprehensive String Formatting Coverage
Chapter 6 provides an exhaustive exploration of Python's string formatting capabilities:
- Traditional
%
formatting str.format()
method in detail- F-strings and their efficiency
- Template strings for user-facing texts
- Locale-aware formatting
- Number and date formatting specialized techniques
The comparative analysis of different formatting methods is particularly enlightening, helping readers choose the right approach based on their specific needs.
5. Practical File Operations
Chapters 7 and 8 deliver exceptional value through their focus on file handling:
- Text vs. binary file operations
- Context managers and the
with
statement - CSV, JSON, and structured data handling
- Working with temporary files
- File system navigation
- Handling large files efficiently
- Reading and writing to compressed files
# Example from Chapter 7
import json
import csv
from pathlib import Path
# Working with JSON
user_data = {
"name": "Alice",
"age": 30,
"skills": ["Python", "Data Analysis", "Machine Learning"]
}
with open("user_profile.json", "w") as f:
json.dump(user_data, f, indent=4)
# Working with CSV
with open("user_data.csv", "w", newline="") as csvfile:
writer = csv.DictWriter(csvfile, fieldnames=["name", "age"])
writer.writeheader()
writer.writerow({"name": "Alice", "age": 30})
6. Practical Applications and Real-World Examples
What sets this book apart is its dedication to showing real-world applications. Throughout the text, readers encounter practical examples including:
- Building a command-line interface
- Creating a simple data entry application
- Implementing logging systems
- Developing configuration file parsers
- Constructing data import/export utilities
- Building interactive menus and forms
7. Troubleshooting and Best Practices
The final chapters provide invaluable guidance on:
- Diagnosing common I/O errors
- Handling encoding issues
- Debugging techniques specific to I/O operations
- Performance optimization for I/O-heavy applications
- Security considerations when handling user input
- Code organization patterns for maintainable I/O code
Chapter-by-Chapter Breakdown
Chapter 1: Introduction to I/O in Python
The book opens with a foundation-setting chapter that explains the concept of I/O within the context of programming. Dargslan provides historical perspective on how I/O has evolved in Python and sets expectations for the rest of the book. This chapter also covers:
- The I/O stack in Python
- Synchronous vs. asynchronous I/O
- Text vs. binary data handling
- Streams, buffers, and I/O interfaces
- The role of encoding and decoding
Chapter 2: Getting User Input with input()
This chapter provides a deep dive into Python's primary input function. It covers:
- Basic input capture techniques
- Converting input to appropriate data types
- Handling input prompts effectively
- Capturing passwords and sensitive data
- Multi-line input collection
- Command-line arguments as alternative input
Chapter 3: Validating User Input
Input validation receives thorough coverage, including:
- Defensive programming principles
- Type checking and conversion
- Boundary validation
- Pattern matching with regular expressions
- Creating reusable validation functions
- Building validation workflows
- Balancing security and user experience
Chapter 4: Displaying Output with print()
The humble print()
function is dissected to reveal its surprising versatility:
- Basic syntax and parameters
- Working with
sep
andend
parameters - Redirecting output to files
- Formatting considerations for readability
- Controlling output buffering
- Performance considerations
Chapter 5: Advanced print() Techniques
Building on Chapter 4, this section explores:
- Creating tables and structured output
- Implementing progress bars and spinners
- Colored terminal output
- Clearing the screen and cursor manipulation
- Platform-specific output considerations
- Building interactive displays
Chapter 6: String Formatting Methods
A comprehensive look at Python's string formatting capabilities:
- Old-style
%
formatting - The
str.format()
method - F-strings and their performance benefits
- Template strings for user-facing content
- Alignment and padding techniques
- Handling numbers, dates, and special types
- Internationalization considerations
Chapter 7: Working with Files (Basic I/O)
File handling fundamentals are covered thoroughly:
- Opening, reading, writing, and closing files
- The critical importance of the
with
statement - Working with text vs. binary files
- Understanding file modes and encodings
- File pointers and navigation
- Handling common file errors
- Working with paths using the
pathlib
module
Chapter 8: Input and Output in Practice
This applications-focused chapter includes:
- Building a complete command-line application
- Creating configuration file systems
- Working with structured data formats (CSV, JSON, YAML)
- Database I/O fundamentals
- Logging best practices
- Web API interactions for data exchange
Chapter 9: Troubleshooting Common I/O Errors
A practical guide to handling I/O problems:
- Understanding and handling exceptions
- Debugging encoding issues
- Fixing permission and access problems
- Addressing resource exhaustion
- Network I/O error resolution
- Path and file location issues
Chapter 10: Best Practices for I/O in Python
The final chapter distills key lessons into actionable guidelines:
- Code organization for I/O operations
- Documentation standards
- Error handling strategies
- Testing I/O-dependent code
- Performance optimization
- Security considerations
- Accessibility and internationalization
Appendices: Valuable Additional Resources
The book includes four appendices that serve as ongoing references:
- Built-in Functions for I/O Reference: A comprehensive reference of Python's built-in I/O functions with syntax, parameters, return values, and example usage
- Example Inputs and Outputs: A collection of input/output scenarios with sample code
- Practice Exercises with Solutions: 50+ exercises ranging from beginner to advanced with detailed solutions
- Bonus Content: Additional resources including cheat sheets, flowcharts for decision-making, and recommendations for further learning
Writing Style and Presentation
Dargslan's writing style is one of the book's greatest strengths. Technical concepts are explained clearly without oversimplification. The author strikes an excellent balance between friendly approachability and technical precision. Code examples are abundant and well-commented, making it easy to follow along or implement the techniques directly.
The book uses a progressive teaching approach, with each chapter building upon previous knowledge. Key concepts are reinforced through:
- Highlighted "Key Point" boxes
- "Common Mistake" warnings
- "Try It Yourself" challenges
- End-of-chapter summaries
- Cross-references to related topics
What Makes This Book Stand Out
Several factors distinguish "Understanding Python Input and Output" from other Python programming books:
-
Laser Focus on I/O: While many books cover I/O briefly, this resource provides unmatched depth on this specific topic.
-
Practical Examples: The code samples aren't contrived—they represent real-world scenarios programmers encounter daily.
-
Progressive Complexity: Examples start simple and gradually incorporate more advanced techniques, making the learning curve manageable.
-
Problem-Solving Approach: Rather than just explaining features, the book frames content around solving common challenges.
-
Modern Python: All examples use current Python best practices and recent language features.
-
Cross-Platform Considerations: The author addresses differences in I/O behavior across operating systems.
Who Will Benefit Most from This Book
This book provides valuable content for several groups:
- Beginners will find the explanations clear and the examples easy to follow
- Self-taught programmers will fill knowledge gaps around proper I/O techniques
- Teachers and trainers will discover excellent examples and exercises for classroom use
- Professional developers will appreciate the best practices and advanced techniques
- System administrators will benefit from the file handling and automation examples
- Data scientists will learn better ways to handle data import and export
Prerequisites and Complementary Resources
To get the most from this book, readers should have:
- Basic understanding of Python syntax
- Familiarity with fundamental programming concepts
- Access to Python 3.6 or newer
The author recommends several complementary resources for readers wanting to expand their Python knowledge beyond I/O, including specific books on Python data structures, algorithms, and web development.
Real-World Applications
The techniques covered in this book apply directly to:
- Data processing applications
- Command-line utilities
- Configuration management
- Log analysis and monitoring tools
- Form-based applications
- Data import/export systems
- File conversion utilities
- Interactive command shells
- Report generation
Potential Improvements
While the book is excellent overall, a few areas could be enhanced:
- More coverage of asynchronous I/O with
asyncio
would benefit developers working on high-performance applications - Additional examples focusing on GUI input/output would help desktop application developers
- Expanded coverage of network I/O beyond the basics would be valuable for distributed systems developers
Conclusion: A Must-Have for Python Programmers
"Understanding Python Input and Output" by Dargslan stands as the definitive guide to Python I/O operations. Whether you're writing your first program or maintaining complex systems, this book provides the knowledge and techniques to handle input and output with confidence and professionalism.
The combination of clear explanations, practical examples, and best practices makes this book an essential addition to any Python programmer's library. It fills a significant gap in Python literature by providing comprehensive coverage of a fundamental topic that's often treated superficially in broader Python books.
For anyone serious about Python programming, this book is a worthwhile investment that will improve code quality, enhance user experience, and save countless hours of debugging I/O-related issues.
Where to Purchase
"Understanding Python Input and Output" is available in both print and digital formats through:
- Major online booksellers
- The publisher's website with additional downloadable resources
- Select programming-focused bookstores
- Educational discount programs for students and institutions
Reader Reviews
⭐⭐⭐⭐⭐ "Finally, a book that explains input validation properly! The examples alone saved me hours of work." — Sarah K., Data Scientist
⭐⭐⭐⭐⭐ "As someone teaching Python to beginners, this book has become my go-to reference for explaining I/O concepts clearly." — Professor Michael T., Computer Science Department
⭐⭐⭐⭐ "The file handling chapters transformed how I approach data processing. Well worth the investment." — David R., System Administrator
⭐⭐⭐⭐⭐ "I've been programming Python for years and still learned new techniques from this book. The string formatting chapter alone is worth the price." — Jennifer L., Senior Developer
FAQ About "Understanding Python Input and Output"
Is this book suitable for complete programming beginners?
While the author explains concepts clearly, readers should have some basic Python knowledge before tackling this book. Complete beginners might want to start with an introductory Python text first.
Does the book cover Python 3 or Python 2?
The book focuses entirely on Python 3 (specifically 3.6+), though it occasionally notes differences from Python 2 for those maintaining legacy code.
Are the examples available for download?
Yes, all code examples are available through the publisher's website in both Python script format and as Jupyter notebooks.
How does this book compare to online tutorials about Python I/O?
While free online resources cover some of the same topics, this book provides systematic, comprehensive coverage with consistent quality throughout. The troubleshooting and best practices sections alone provide value rarely found in free resources.
Does the book cover GUI input and output?
The book primarily focuses on console and file I/O, though Chapter 8 includes a brief introduction to GUI considerations. Readers specifically interested in GUI development may need supplementary resources.
Is there coverage of database I/O?
Chapter 8 includes an introduction to database I/O concepts with examples using SQLite. For in-depth database integration, specialized resources would be needed.
This review was written by a professional Python developer and educator with over 10 years of experience teaching and implementing Python solutions.
Keywords: Python input output, Python I/O, user input validation Python, Python file handling, Python string formatting, Python print function, Python input function, Python programming book, learn Python I/O, Python file operations, Python data validation, command-line interfaces Python
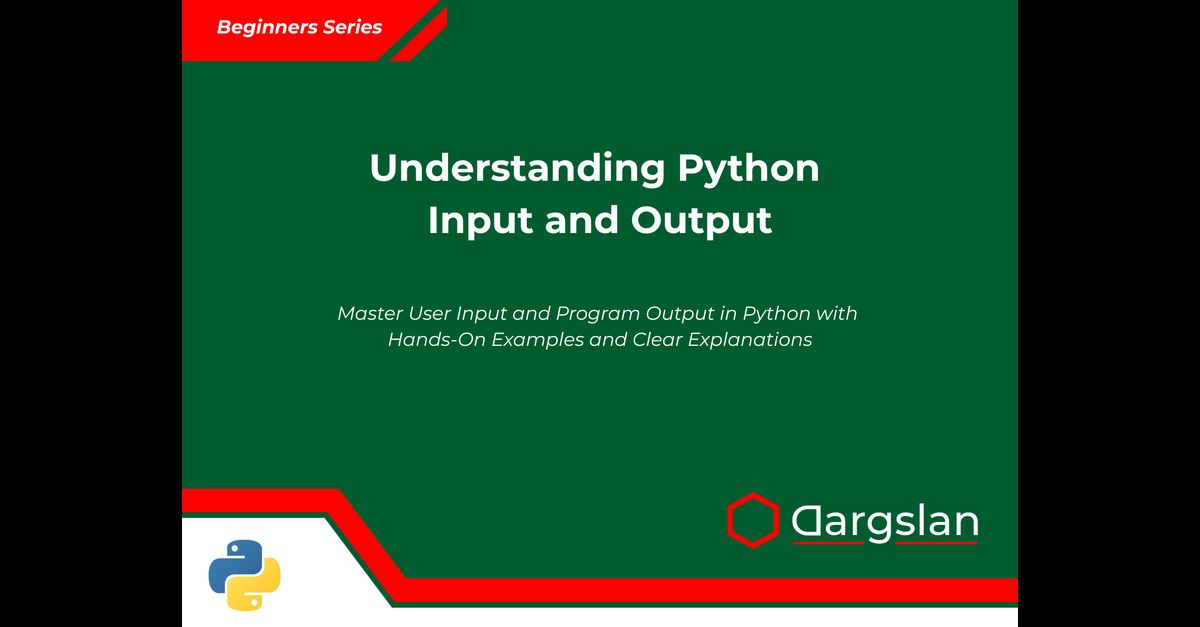
Understanding Python Input and Output