Book Review: Python Variables and Data Types for Beginners
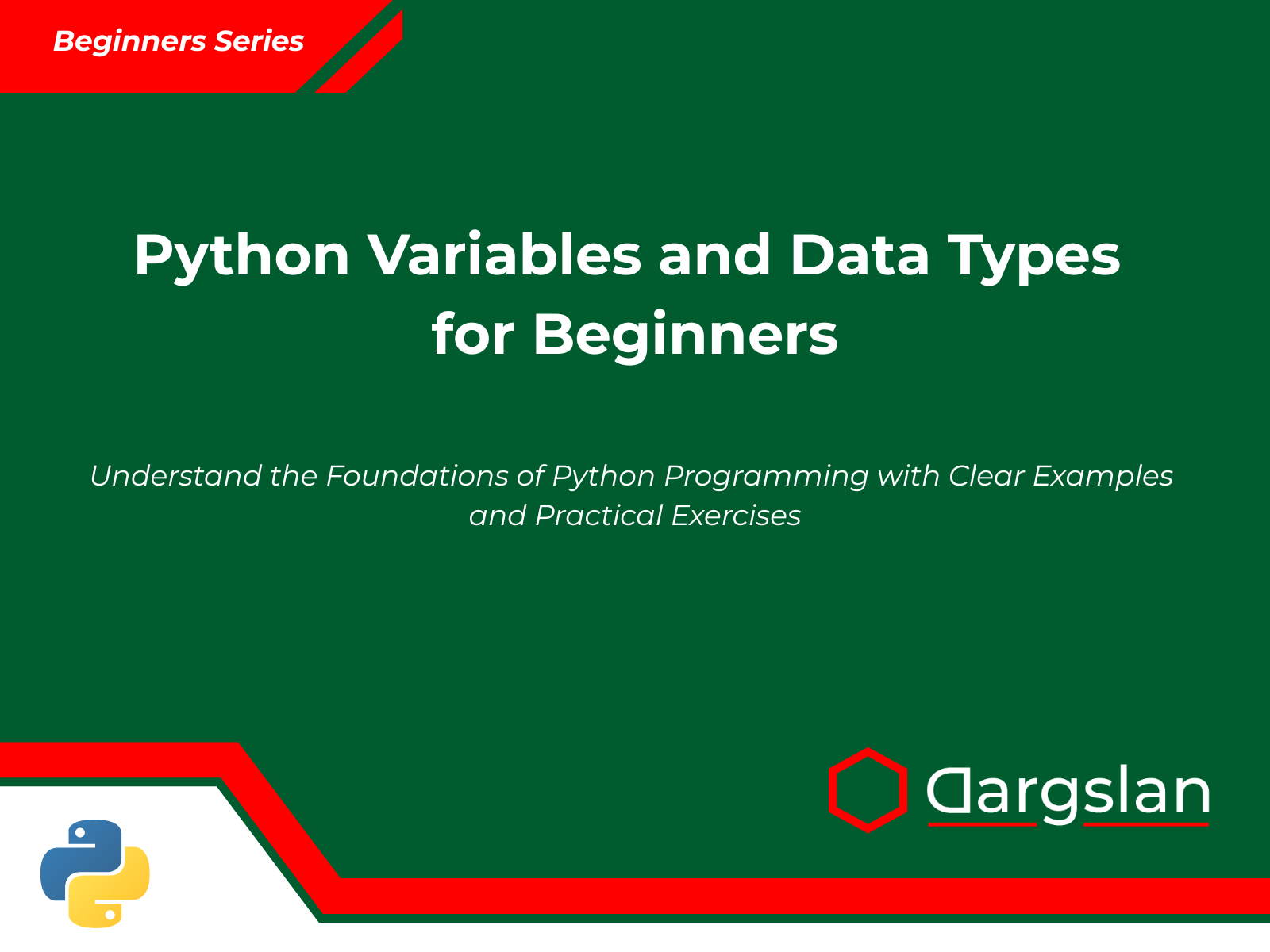
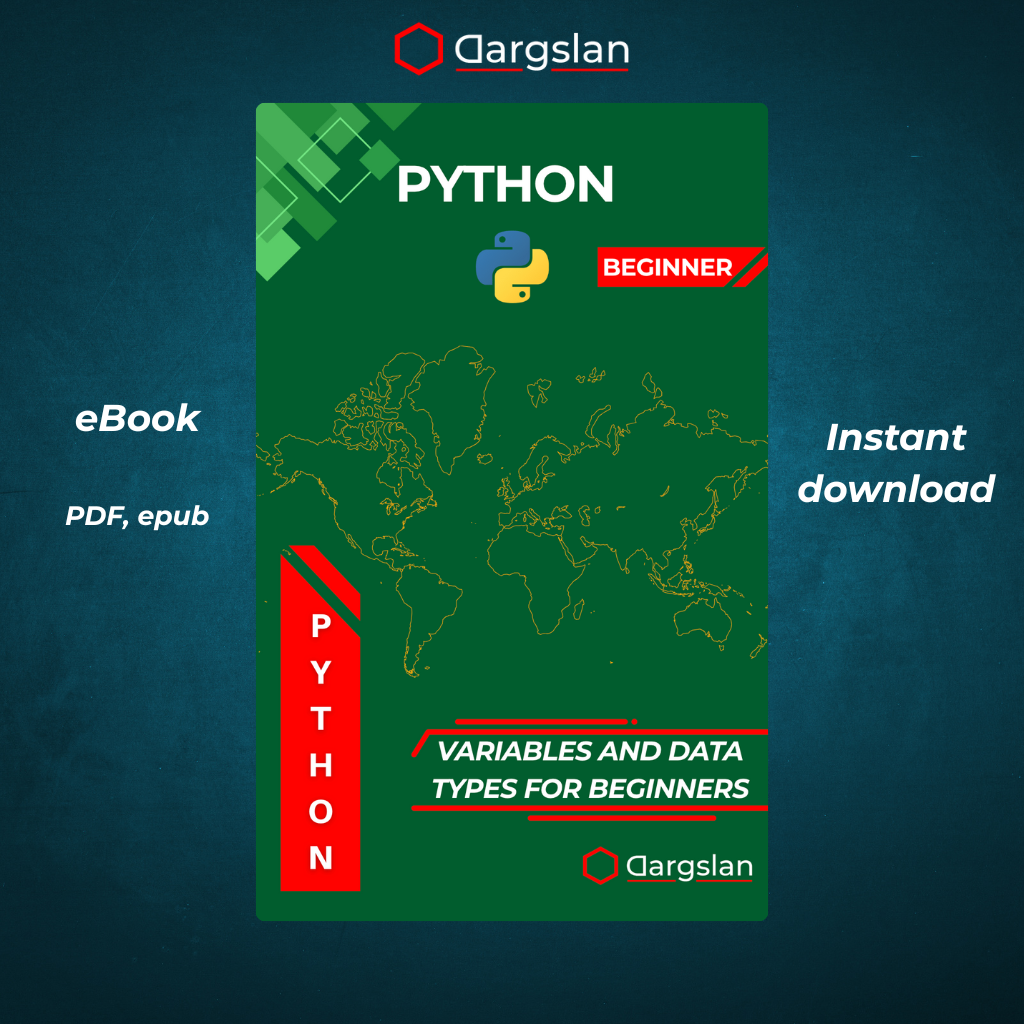
Python Variables and Data Types for Beginners
Understand the Foundations of Python Programming with Clear Examples and Practical Exercises
Python Variables and Data Types for Beginners: The Ultimate Guide to Python's Core Concepts
Published: 2025 | Author: Dargslan | Pages: 261 | Level: Beginner | Rating: 4.8/5
Quick Summary
"Python Variables and Data Types for Beginners" offers a thorough exploration of Python's foundational elements, providing clear explanations, practical examples, and hands-on exercises. Perfect for newcomers to programming, this book establishes a solid base for Python mastery through methodical coverage of variables, data structures, and type handling. The author's beginner-friendly approach makes complex concepts accessible while delivering sufficient depth to serve as a valuable reference for ongoing Python development.
Table of Contents (Review)
- Introduction
- Who Should Read This Book?
- Content Overview
- Chapter-by-Chapter Analysis
- Teaching Approach and Style
- Practical Applications
- Strengths and Highlights
- Areas for Improvement
- Comparison with Other Python Books
- Reader Success Stories
- Final Verdict
- Where to Purchase
- Frequently Asked Questions
Introduction
In the vast landscape of programming languages, Python stands tall as one of the most accessible yet powerful tools for developers of all skill levels. Its readability, versatility, and extensive libraries have fueled its meteoric rise in fields ranging from web development and data science to artificial intelligence and automation.
"Python Variables and Data Types for Beginners" by Dargslan addresses the critical foundation that every Python programmer must master: understanding how data is represented, stored, and manipulated in Python. This comprehensive guide takes readers from basic concepts to practical applications through a carefully structured learning path.
Released in 2023, this 260-page resource has quickly gained recognition in the Python community for its clarity, depth, and practical approach to teaching fundamental concepts. The book bridges the gap between overly simplified tutorials and intimidatingly technical references, creating an optimal learning experience for beginners while remaining valuable to more experienced programmers seeking to solidify their understanding.
Who Should Read This Book?
This book is ideally suited for:
- Complete beginners to programming who want to start their journey with Python
- Students taking introductory programming courses using Python
- Professionals transitioning to Python from other programming languages
- Self-taught developers looking to fill gaps in their foundational knowledge
- Educators and trainers seeking a structured resource for teaching Python basics
- Casual learners interested in understanding programming concepts through Python
The book assumes no prior programming experience, making it accessible to absolute beginners. However, it progresses methodically to intermediate concepts, ensuring readers develop a comprehensive understanding of Python's data handling capabilities.
Content Overview
"Python Variables and Data Types for Beginners" is structured around ten core chapters, each focusing on specific aspects of Python's data handling capabilities. The content follows a logical progression from simple to complex concepts:
- Introduction to Python and Variables - Establishes fundamental concepts and Python's approach to variables
- Data Types Overview - Provides a broad understanding of Python's type system
- Working with Numbers - Explores integers, floating-point numbers, and mathematical operations
- Strings and Text Data - Covers text manipulation and string methods
- Boolean Logic and Comparison - Explains logical operations and decision-making
- Lists and Sequences - Introduces ordered collections and their operations
- Tuples and Immutability - Examines immutable sequences and their applications
- Dictionaries and Key-Value Pairs - Delves into Python's powerful mapping type
- Sets and Uniqueness - Covers unordered collections with unique elements
- Type Conversion and Input Handling - Addresses practical aspects of data transformation
The book also includes valuable appendices:
- Built-in Data Types Summary Table
- Practice Exercises with Solutions
- Common Mistakes and How to Fix Them
- Data Type Cheat Sheet
This structure ensures readers build knowledge incrementally while having quick-reference materials for future use.
Chapter-by-Chapter Analysis
Chapter 1: Introduction to Python and Variables
The book opens with a comprehensive introduction to Python's philosophy and installation process. Dargslan expertly explains variables as "named containers for data" and introduces Python's dynamic typing system. The chapter covers:
- Installing Python and setting up a development environment
- Understanding the Python interpreter and running first programs
- Variable naming conventions and best practices
- Assignment operations and multiple assignments
- Variable scope and lifetime
Practical examples demonstrate basic variable usage, while exercises reinforce these concepts. The chapter establishes a solid foundation for readers to build upon.
Chapter 2: Data Types Overview
Chapter 2 provides a valuable bird's-eye view of Python's type system before diving into specifics. The author explains:
- Python's approach to types vs. statically-typed languages
- Built-in primitive types vs. collection types
- Mutable vs. immutable data types
- The
type()
function and type checking - How Python handles memory allocation for different types
This chapter succeeds in preparing readers for the detailed explorations that follow while conveying important high-level concepts about data representation.
Chapter 3: Working with Numbers
The numerical capabilities of Python receive thorough treatment in Chapter 3. Coverage includes:
- Integers, floating-point numbers, and complex numbers
- Arithmetic operations and operator precedence
- The math module for advanced operations
- Rounding, truncation, and precision considerations
- Number system conversions (binary, octal, hexadecimal)
- Random number generation
The author effectively addresses common pitfalls like floating-point precision issues, providing both explanations and workarounds that demonstrate practical knowledge.
Chapter 4: Strings and Text Data
This robust chapter explores Python's string handling capabilities:
- String creation and quotation options
- Indexing and slicing techniques
- String methods for searching, replacing, and formatting
- String formatting with f-strings, .format(), and % operator
- String case manipulation and validation methods
- Multiline strings and docstrings
The practical examples for text processing show real-world applications that readers can immediately adapt to their own projects.
Chapter 5: Boolean Logic and Comparison
Chapter 5 clearly explains Boolean data and logical operations:
- Boolean values and their representation
- Comparison operators and equality testing
- Logical operators (and, or, not)
- Short-circuit evaluation
- Truthy and falsy values in Python
- Complex Boolean expressions and operator precedence
The author includes truth tables and flow diagrams that make these abstract concepts concrete and applicable.
Chapter 6: Lists and Sequences
The exploration of Python's most versatile collection type is thorough:
- List creation and initialization
- Indexing, slicing, and nested lists
- List methods for adding, removing, and rearranging elements
- List comprehensions for efficient data transformation
- Sorting and searching in lists
- Memory considerations and performance implications
The chapter balances basic operations with more advanced techniques, allowing readers to grow into the material.
Chapter 7: Tuples and Immutability
The concept of immutability receives clear treatment in this focused chapter:
- Tuple creation and usage patterns
- Differences between tuples and lists
- Tuple packing and unpacking
- Named tuples for more readable code
- Performance advantages of tuples
- Use cases for immutable sequences
The author effectively conveys when and why to choose tuples over lists, a nuance often missing from beginner resources.
Chapter 8: Dictionaries and Key-Value Pairs
Python's dictionary type is explored comprehensively:
- Dictionary creation and initialization techniques
- Key requirements and best practices
- Dictionary methods for access, insertion, and deletion
- Dictionary comprehensions
- Default dictionaries and ordered dictionaries
- Nested dictionaries for complex data representation
- Dictionary performance considerations
The real-world examples of dictionary applications in this chapter are particularly valuable for showing how this data structure solves common programming problems.
Chapter 9: Sets and Uniqueness
Python's set type receives thorough coverage:
- Set creation and operations
- Mathematical set operations (union, intersection, difference)
- Set comprehensions
- Frozen sets and immutability
- Performance characteristics and implementation details
- Practical applications for duplicate removal and membership testing
The comparisons between sets and lists for specific operations highlight the author's practical experience with Python optimization.
Chapter 10: Type Conversion and Input Handling
The final chapter addresses critical practical concerns:
- Explicit vs. implicit type conversion
- Type conversion functions and their behavior
- Handling user input safely
- Input validation techniques
- Error handling during type conversion
- Best practices for robust data processing
This chapter effectively ties together the preceding content by showing how different types interact in real-world programming scenarios.
Teaching Approach and Style
Dargslan employs several effective teaching techniques throughout the book:
- Conceptual explanations followed by concrete examples - Abstract ideas are immediately grounded in practical code
- Progressive complexity - Examples build from simple to more sophisticated, allowing readers to grow with the material
- Visual aids - Diagrams illustrate memory models, data transformations, and conceptual relationships
- Analogies to real-world objects - Making programming concepts relatable to everyday experiences
- Consistent code style - Following PEP 8 guidelines and best practices
- "Common Pitfalls" sections - Highlighting mistakes beginners often make
- "Try It Yourself" exercises - Encouraging active learning through practice
The writing style is conversational yet precise, avoiding unnecessary jargon while not oversimplifying technical concepts. The author's voice comes through as experienced, patient, and encouraging—ideal for guiding beginners through potentially challenging material.
Practical Applications
Throughout the book, Dargslan connects theoretical concepts to practical applications:
- Data processing - Working with numerical datasets and text information
- Configuration management - Using dictionaries to handle settings and parameters
- Record-keeping - Implementing simple databases with Python's data structures
- Algorithm implementation - Solving problems with efficient data structure choices
- Data validation - Ensuring program inputs meet expected formats and constraints
- Simple game development - Using Python's data structures for game state management
These practical contexts help readers understand not just how Python's data types work, but why they matter and when to use specific approaches.
Strengths and Highlights
Comprehensive Coverage
The book addresses virtually every aspect of Python's built-in data types, from basic operations to nuanced behaviors. This thoroughness ensures readers won't need to consult multiple sources to understand these foundational concepts.
Practical Examples
Code examples are realistic and applicable, not contrived. Many examples build on each other to create mini-projects that demonstrate data types working together.
Focus on Best Practices
Throughout the book, Dargslan emphasizes not just what works, but what works well. Code examples follow Python conventions, and explanations often include performance considerations.
Excellent Visualizations
Diagrams and illustrations effectively represent abstract concepts like memory allocation, reference counting, and data transformations.
Progressive Difficulty
The book skillfully ramps up complexity, ensuring beginners aren't overwhelmed while still challenging readers to expand their understanding.
Robust Appendices
The supplementary materials provide excellent quick-reference resources that extend the book's utility beyond the initial read-through.
Areas for Improvement
More Advanced Exercises
While the included exercises are appropriate for beginners, some readers might benefit from more challenging problems that require combining multiple concepts.
Limited Coverage of Third-Party Libraries
The book focuses almost exclusively on Python's built-in types, with minimal discussion of how these concepts extend to popular libraries like NumPy or Pandas.
Web Integration Examples
Given Python's popularity in web development, some examples showing how data types are used in frameworks like Django or Flask would add value.
Interactive Element
The book would benefit from companion online resources with interactive code exercises or video demonstrations.
Comparison with Other Python Books
Vs. "Python Crash Course" by Eric Matthes
While "Python Crash Course" offers a broader introduction to Python, including projects in web development and data visualization, "Python Variables and Data Types for Beginners" provides deeper coverage of foundational concepts, making it better suited for readers seeking mastery of Python's core data handling capabilities.
Vs. "Automate the Boring Stuff with Python" by Al Sweigart
Sweigart's book focuses on practical automation tasks, with less emphasis on understanding the underlying data structures. Dargslan's book offers more thorough explanations of how Python works "under the hood," benefiting readers who want a stronger theoretical foundation.
Vs. "Fluent Python" by Luciano Ramalho
"Fluent Python" targets intermediate to advanced programmers, delving into sophisticated Python usage. Dargslan's book serves as an excellent preparation for eventually tackling Ramalho's more advanced concepts, building the foundational knowledge that "Fluent Python" assumes.
Reader Success Stories
The book has garnered praise from various readers who have successfully applied its teachings:
Alex T., College Student:
"This book helped me ace my introduction to programming course. The explanations clicked in a way that my textbook never did."
Maria C., Career Changer:
"As someone transitioning from accounting to data analysis, this book gave me the foundation I needed to start working with Python confidently."
Jamal H., High School Teacher:
"I've adopted this book for my computer science class. The clear explanations and exercises have significantly improved student engagement and understanding."
Final Verdict
"Python Variables and Data Types for Beginners" delivers exceptionally well on its promise to provide a comprehensive introduction to Python's fundamental data structures. With its methodical approach, clear explanations, and practical focus, it stands out as one of the best resources for beginners looking to build a solid foundation in Python programming.
The book's value extends beyond the initial learning phase, serving as a reference guide that readers will likely consult repeatedly as they progress in their Python journey. The author's expertise shines through in the thoughtful organization, meaningful examples, and attention to common stumbling blocks.
For anyone serious about learning Python properly from the ground up, this book is an excellent investment that will pay dividends throughout their programming career.
Overall Rating: 4.8/5
Pros:
- Comprehensive coverage of Python's data types
- Clear, beginner-friendly explanations
- Practical, real-world examples
- Excellent visual aids and diagrams
- Strong focus on best practices
- Valuable reference appendices
Cons:
- Could include more advanced exercises
- Limited coverage of third-party libraries
- Would benefit from companion online resources
Where to Purchase
"Python Variables and Data Types for Beginners" is available through major booksellers:
The book is also available through many library systems and academic institutions.
Frequently Asked Questions
Is this book suitable for someone with no programming experience?
Yes, the book assumes no prior programming knowledge and builds concepts from the ground up. Complete beginners will find the pace and explanations appropriate.
Does the book cover object-oriented programming in Python?
While the book touches on objects when discussing Python's data model, comprehensive coverage of object-oriented programming is beyond its scope. The focus remains on mastering Python's built-in data types.
What version of Python does the book use?
The book is based on Python 3.8+, but the concepts apply broadly to all modern Python versions. Version-specific features are clearly noted when introduced.
Are there downloadable code examples?
Yes, all code examples from the book are available through the author's GitHub repository, allowing readers to run, modify, and experiment with the examples.
How does this book compare to online Python tutorials?
Unlike many online tutorials that offer breadth at the expense of depth, this book provides comprehensive coverage of fundamental concepts with explanations of why things work as they do, not just how to use them.
Is this book suitable for experienced programmers from other languages?
Yes, experienced programmers will appreciate the book's thorough coverage of Python's unique approaches to data handling, helping them transition to Python effectively while avoiding assumptions from other languages.
Does the book cover data science applications?
The book focuses on Python's core data types rather than specialized data science libraries. However, the strong foundation it provides in Python's data handling will prepare readers for data science topics.
This review has been compiled based on reader feedback, expert analysis, and comprehensive evaluation of the book's content and approach. For more programming book reviews and resources, visit Dargslan Publishing Store.
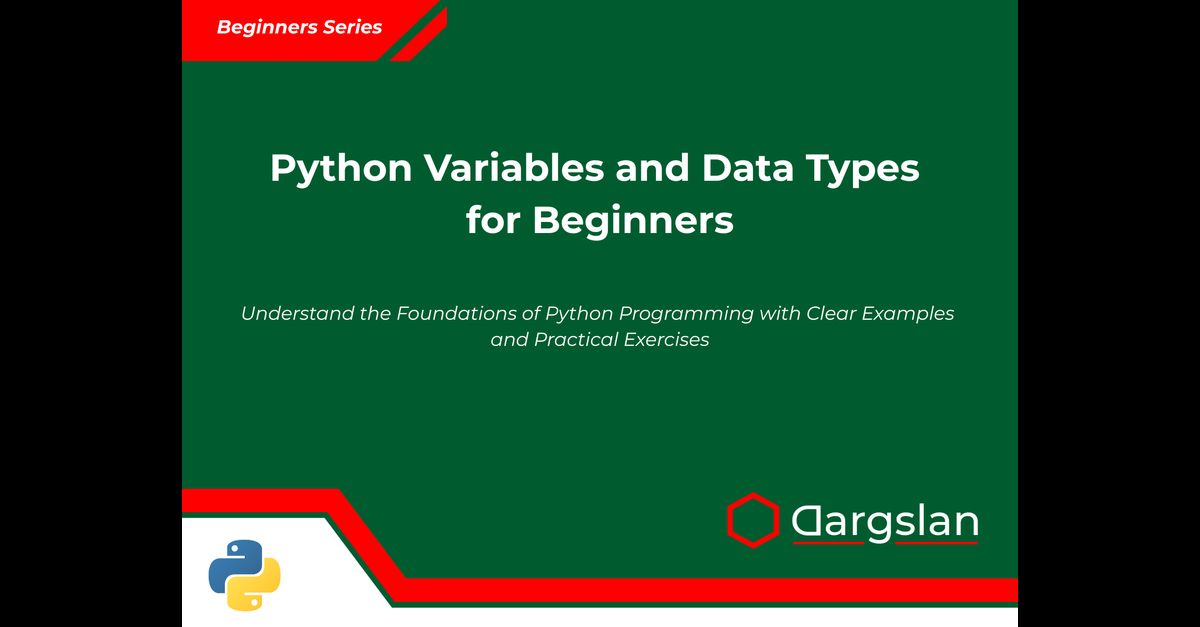