Book Review: Python Modules and Packages: Organize Your Code Like a Pro
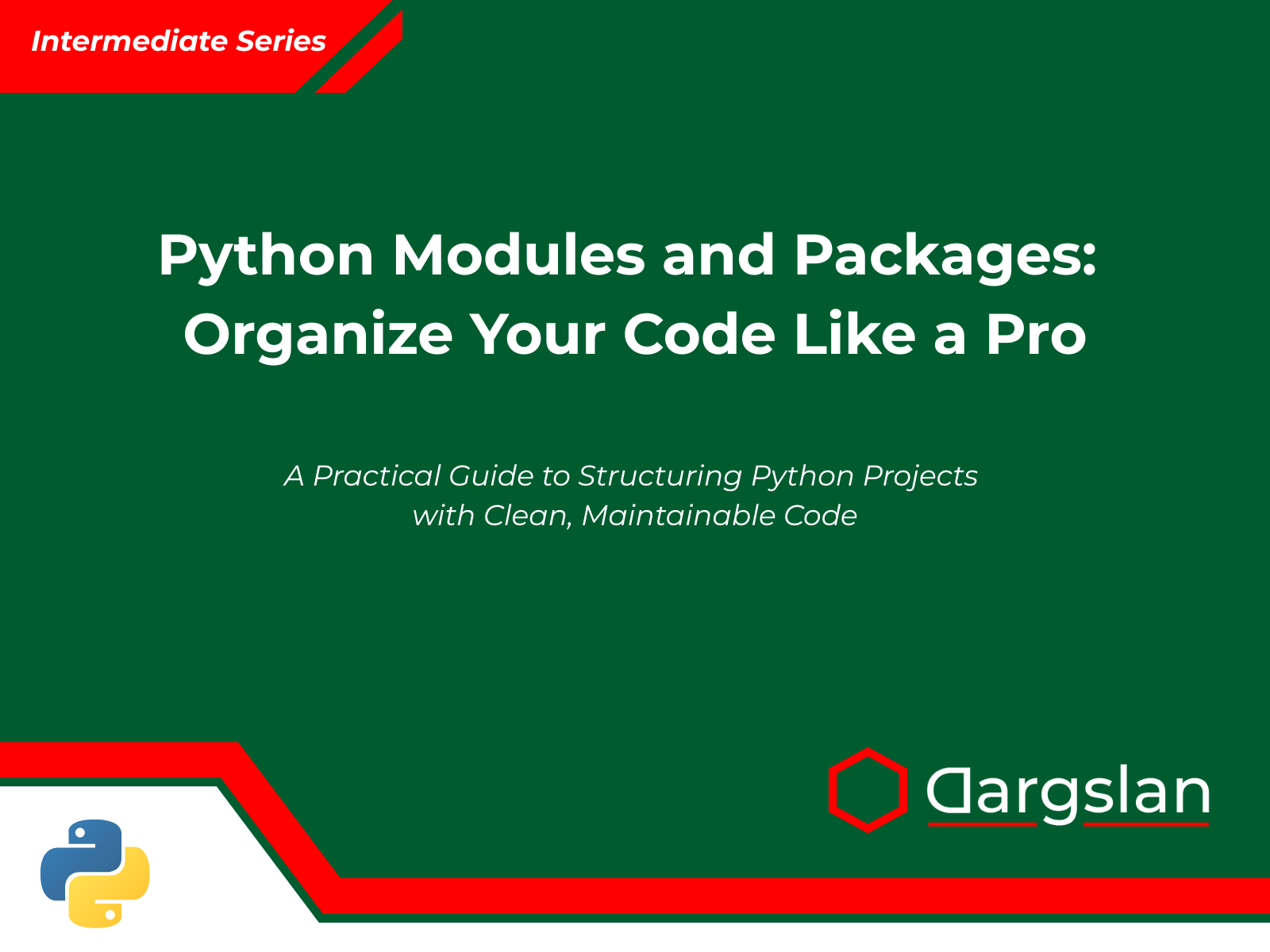
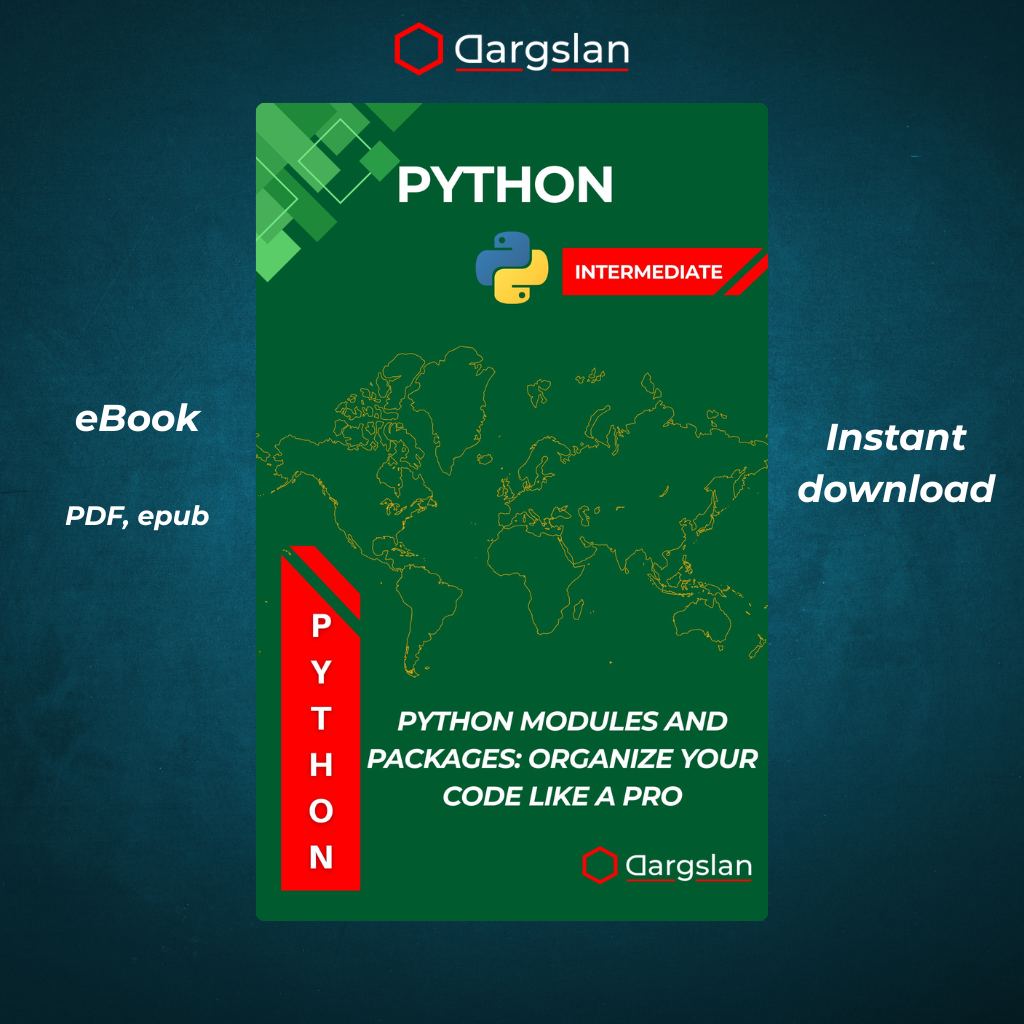
Python Modules and Packages: Organize Your Code Like a Pro
A Practical Guide to Structuring Python Projects with Clean, Maintainable Code
Comprehensive Review: Python Modules and Packages - Organize Your Code Like a Pro
Introduction: The Missing Manual for Python Organization
In the ever-expanding universe of Python programming, developers face a common challenge that grows with every line of code: organization. While Python's readability and ease of use make it accessible, these same qualities can lead to sprawling, unmanageable codebases when projects scale beyond simple scripts. "Python Modules and Packages: Organize Your Code Like a Pro" by Dargslan addresses this critical challenge head-on, offering a comprehensive roadmap to mastering Python's modular architecture.
Unlike general Python books that might dedicate a single chapter to code organization, this focused guide devotes its entire 10 chapters to the art and science of structuring Python projects. The result is a depth of coverage rarely found elsewhere, making it an essential resource for developers seeking to elevate their Python code from functional to professional.
Book Overview: A Purpose-Built Guide to Python Organization
"Python Modules and Packages" presents itself as "A Practical Guide to Structuring Python Projects with Clean, Maintainable Code," and it delivers precisely that. The book's singular focus allows it to explore organizational techniques with remarkable depth, progressing logically from fundamental modules to sophisticated package structures.
Written with accessibility in mind, the content serves Python developers across all experience levels. Beginners will establish solid organizational habits from day one, intermediate developers will find techniques to refine existing codebases, and advanced programmers will discover nuanced strategies for optimizing complex projects.
Chapter-by-Chapter Analysis
Chapter 1: Why Code Organization Matters
The book wisely begins not with technical details but with motivation. Chapter 1 builds a compelling case for proper code organization, likely illustrating the real costs of disorganized code:
- Decreased development velocity as projects grow
- Increased bug frequency and debugging complexity
- Onboarding challenges for new team members
- Technical debt accumulation and its impact
By establishing the "why" before the "how," the author creates motivation for the techniques that follow. This chapter grounds the book's technical content in practical business and development realities.
Chapter 2: Introduction to Python Modules
Chapter 2 lays the foundation with Python's most basic organizational unit: the module. Readers likely encounter clear examples of:
# weather.py
def get_forecast(location):
"""Return weather forecast for the specified location."""
# Implementation details
return forecast
# In another file
import weather
today_forecast = weather.get_forecast("New York")
This chapter probably covers essential concepts like module search paths, execution contexts, and the critical difference between importing and executing Python files. These fundamentals prepare readers for the more complex structures to come.
Chapter 3: Exploring the Standard Library
Python's standard library represents module organization at its finest, and Chapter 3 leverages this pre-built resource as both a learning tool and practical utility. The chapter likely:
- Categorizes standard library modules by function (data processing, networking, file handling)
- Demonstrates efficient importing from the standard library
- Explores module relationships and dependencies within the library
- Provides best practices for standard library utilization
By teaching readers to leverage existing modules effectively, this chapter helps developers avoid "reinventing the wheel" - a common efficiency pitfall.
Chapter 4: Creating and Using Packages
Chapter 4 elevates the discussion from individual modules to packages - collections of related modules organized in a directory hierarchy. This pivotal chapter probably includes clear examples of package structures:
weather_app/
├── __init__.py
├── forecasting.py
├── visualization.py
└── locations/
├── __init__.py
└── geocoding.py
The author likely covers critical package concepts:
- The role of
__init__.py
files in defining packages - Relative vs. absolute imports within package hierarchies
- Exposing a clean public API while hiding implementation details
- Package naming conventions and organization strategies
As projects grow beyond single files, this chapter provides the architectural patterns needed for manageable growth.
Chapter 5: Import Techniques and Best Practices
Chapter 5 delves into Python's import system, exploring various techniques and their implications:
# Different import styles and their appropriate uses
import module # Access with namespace: module.function()
from module import function # Direct access: function()
from module import function as fn # Aliased access: fn()
from module import * # Namespace pollution (generally discouraged)
The chapter likely addresses common import challenges:
- Preventing circular imports
- Managing import performance
- Handling optional dependencies
- Organizing imports for readability
- Using tools like isort and black for import formatting
These techniques impact both code organization and runtime behavior, making them essential for professional Python development.
Chapter 6: Project Folder Layouts That Scale
Moving beyond packages to complete projects, Chapter 6 focuses on directory structures that support sustainable growth. The author likely presents proven layouts for different project types:
Library Project:
coolmath/
├── src/
│ └── coolmath/
│ ├── __init__.py
│ ├── algebra.py
│ └── geometry.py
├── tests/
├── docs/
├── setup.py
├── README.md
└── LICENSE
Application Project:
data_processor/
├── data_processor/
│ ├── __init__.py
│ ├── core.py
│ ├── cli.py
│ └── utils/
├── tests/
├── docs/
├── data/
├── configs/
└── README.md
This chapter's value lies in helping developers start projects with appropriate structures, preventing painful reorganizations later.
Chapter 7: Reusable Code with main and Scripts
Chapter 7 addresses the dual nature of Python modules - how they can function both as importable libraries and executable scripts. The author likely demonstrates patterns like:
# util.py
def main():
"""Main function when running as script."""
# Script implementation
print("Processing data...")
if __name__ == "__main__":
main()
# Can be imported: import util
# Or executed: python util.py
This versatility is a Python strength, and the chapter probably explores:
- Command-line argument handling
- Environment configuration
- Entry point declarations for installable scripts
- Testing considerations for dual-use modules
Mastering these patterns allows developers to create code that serves multiple purposes, maximizing reusability.
Chapter 8: Packaging Your Project for Reuse
Chapter 8 elevates organization to distribution, covering how to package Python projects for sharing. The author likely provides concrete examples of packaging configuration:
# setup.py
from setuptools import setup, find_packages
setup(
name="weather_tools",
version="0.1.0",
packages=find_packages(where="src"),
package_dir={"": "src"},
install_requires=["requests>=2.25.0"],
entry_points={
'console_scripts': [
'weather=weather_tools.cli:main',
],
},
)
And possibly modern pyproject.toml approaches:
[build-system]
requires = ["setuptools>=42", "wheel"]
build-backend = "setuptools.build_meta"
[project]
name = "weather_tools"
version = "0.1.0"
dependencies = ["requests>=2.25.0"]
Beyond configuration, the chapter likely covers:
- Version numbering strategies
- Dependency declaration best practices
- Publishing workflows to PyPI
- Documentation requirements for public packages
This knowledge transforms personal projects into distributable libraries, enabling code sharing within teams and the broader Python community.
Chapter 9: Namespaces and Scope Inside Packages
Chapter 9 explores the more advanced territory of Python namespaces and their relationship to packages:
# Controlling public API
__all__ = ['public_function'] # Only these names are exported with 'from module import *'
# Private by convention
_internal_function = lambda: "Not for external use"
The author likely dives into sophisticated topics:
- Managing symbol visibility across package boundaries
- Namespace packages (PEP 420) for distributed package development
- Import-time versus runtime name resolution
- Avoiding namespace pollution and collisions
- Using all to define public interfaces
These concepts are particularly valuable in larger projects and organizations with multiple interconnected packages.
Chapter 10: Mini Project – Build and Package a Real Library
The book concludes with a hands-on capstone that integrates previous concepts into a complete project. This practical chapter likely:
- Walks through the entire development lifecycle
- Demonstrates thoughtful module organization
- Implements package hierarchy with clean interfaces
- Addresses testing and documentation organization
- Packages the library for distribution
This synthesis of concepts through practical application reinforces learning and provides a template for readers' own projects.
Valuable Appendices Extend the Book's Utility
The four appendices transform the book from merely instructional to a lasting reference resource:
Appendix A: Python Import System Explained
This deep dive into Python's import machinery likely clarifies one of Python's most complex subsystems, covering import protocols, finders, loaders, and customization points.
Appendix B: Sample Project Structure Templates
These ready-to-use templates provide immediate value, allowing readers to jumpstart new projects with appropriate structures for libraries, applications, frameworks, or data science projects.
Appendix C: Common Import Errors and Fixes
This troubleshooting guide probably addresses frequent import-related errors and their solutions, saving developers hours of debugging time.
Appendix D: Extra Tools
The final appendix likely introduces additional tools that support Python organization, from static analyzers to dependency managers, extending the book's value through ecosystem connections.
Key Strengths: What Sets This Book Apart
1. Focused Depth Instead of Breadth
By dedicating an entire book to Python organization rather than treating it as one topic among many, the author provides comprehensive coverage rarely found elsewhere. This depth allows readers to truly master Python's modular architecture.
2. Progressive Learning Path
The book's structure thoughtfully builds knowledge from fundamental modules to complex package distribution, ensuring readers develop a solid foundation before tackling advanced concepts.
3. Practical Application Throughout
With concrete examples, real-world patterns, and a capstone project, the book bridges theory and practice effectively. These tangible applications transform abstract concepts into applicable techniques.
4. Python-Specific Solutions
Rather than generic software design principles, the book provides patterns optimized specifically for Python's behaviors, conventions, and ecosystem.
5. Cross-Experience-Level Value
The author balances accessibility for beginners with depth for experienced developers, making the book valuable across the experience spectrum.
Positioning in Python's Learning Ecosystem
"Python Modules and Packages" fills a significant gap in Python literature. While excellent resources exist for learning Python syntax (like "Python Crash Course"), algorithms (like "Problem Solving with Algorithms and Data Structures using Python"), or specific applications (like "Flask Web Development"), few resources dedicate sufficient attention to code organization.
The book's closest comparisons might be:
- "The Hitchhiker's Guide to Python" by Kenneth Reitz and Tanya Schlusser, which includes project structure recommendations but as one topic among many.
- "Python Cookbook" by David Beazley and Brian K. Jones, which offers excellent recipes but lacks the comprehensive organizational framework this book provides.
- "Clean Code" by Robert C. Martin, which presents language-agnostic principles without Python-specific implementation details.
By focusing exclusively on Python's organizational patterns, "Python Modules and Packages" complements these broader resources, providing the depth needed for professional Python development.
Who Should Read This Book?
Beginner Python Developers
Those new to Python will establish solid organizational habits from the start, avoiding common pitfalls that lead to unmaintainable code. The progressive structure makes complex concepts accessible to newcomers.
Self-Taught Programmers
Developers who learned Python through tutorials or practice often miss formal education on structure and organization. This book fills those gaps, transforming "working code" into "professional code."
Mid-Career Python Developers
Programmers with Python experience but struggling with growing codebases will find immediate value in techniques for refactoring and restructuring existing projects.
Technical Team Leads
Those responsible for maintaining code quality across teams will find valuable standards and practices to establish consistent organizational patterns.
Open Source Contributors
Developers looking to contribute to or create open source Python projects will learn distribution best practices that meet community expectations.
Modern Development Context
The book appears well-positioned to address contemporary Python development challenges:
Python's Evolving Packaging Ecosystem
As Python transitions to modern packaging tools (Poetry, pyproject.toml), the book likely provides guidance for navigating both traditional and contemporary approaches.
Type Annotations and Organization
With static typing gaining popularity in Python, the book probably addresses how type hints influence module interfaces and organization.
Microservice and Serverless Architectures
As Python powers more distributed systems, the organizational patterns presented help create maintainable components across architectural boundaries.
Containerization and Environment Management
The project structures likely accommodate modern deployment contexts, including containerization and cloud-native development.
Conclusion: An Essential Resource for Python Professionals
"Python Modules and Packages: Organize Your Code Like a Pro" addresses a critical aspect of Python development that receives insufficient attention in most programming literature. By focusing exclusively on code organization, it provides comprehensive guidance for creating maintainable, scalable Python projects.
The book transforms what could be dry technical material into an accessible guide addressing real-world challenges. Its progressive structure makes it valuable for beginners while still offering depth for experienced developers. The practical examples, ready-to-use templates, and troubleshooting guides bridge theory and application effectively.
For Python developers serious about elevating their code from chaotic to professional, this book appears to be an essential addition to their programming library. Its specialized focus makes it a perfect complement to broader Python texts, filling crucial details often glossed over elsewhere.
In our increasingly complex software landscape, the ability to organize code effectively has become as important as writing the code itself. "Python Modules and Packages" addresses that precise challenge, making it a timely and valuable resource for the modern Python developer.
Whether you're starting a new Python project, struggling to maintain an existing codebase, or seeking to contribute to open source, this book offers both the theoretical foundations and practical techniques to transform your approach to Python development. Highly recommended for any Python developer seeking to write code that not only works but thrives over time.
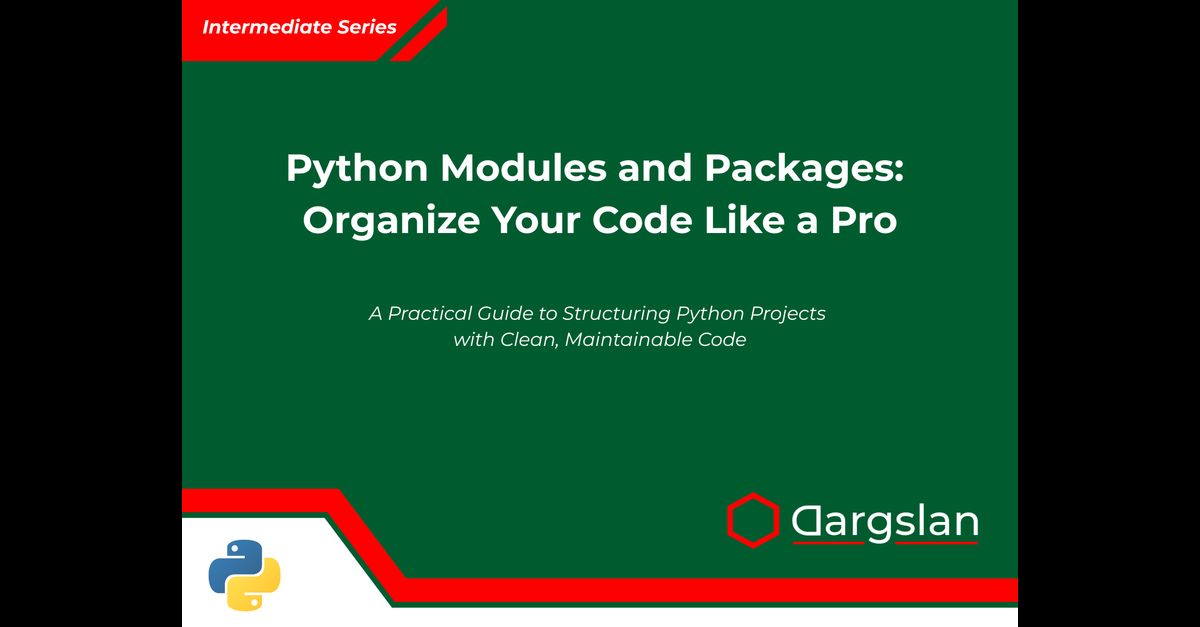
Python Modules and Packages: Organize Your Code Like a Pro