Book Review: Python Logging and Debugging Techniques
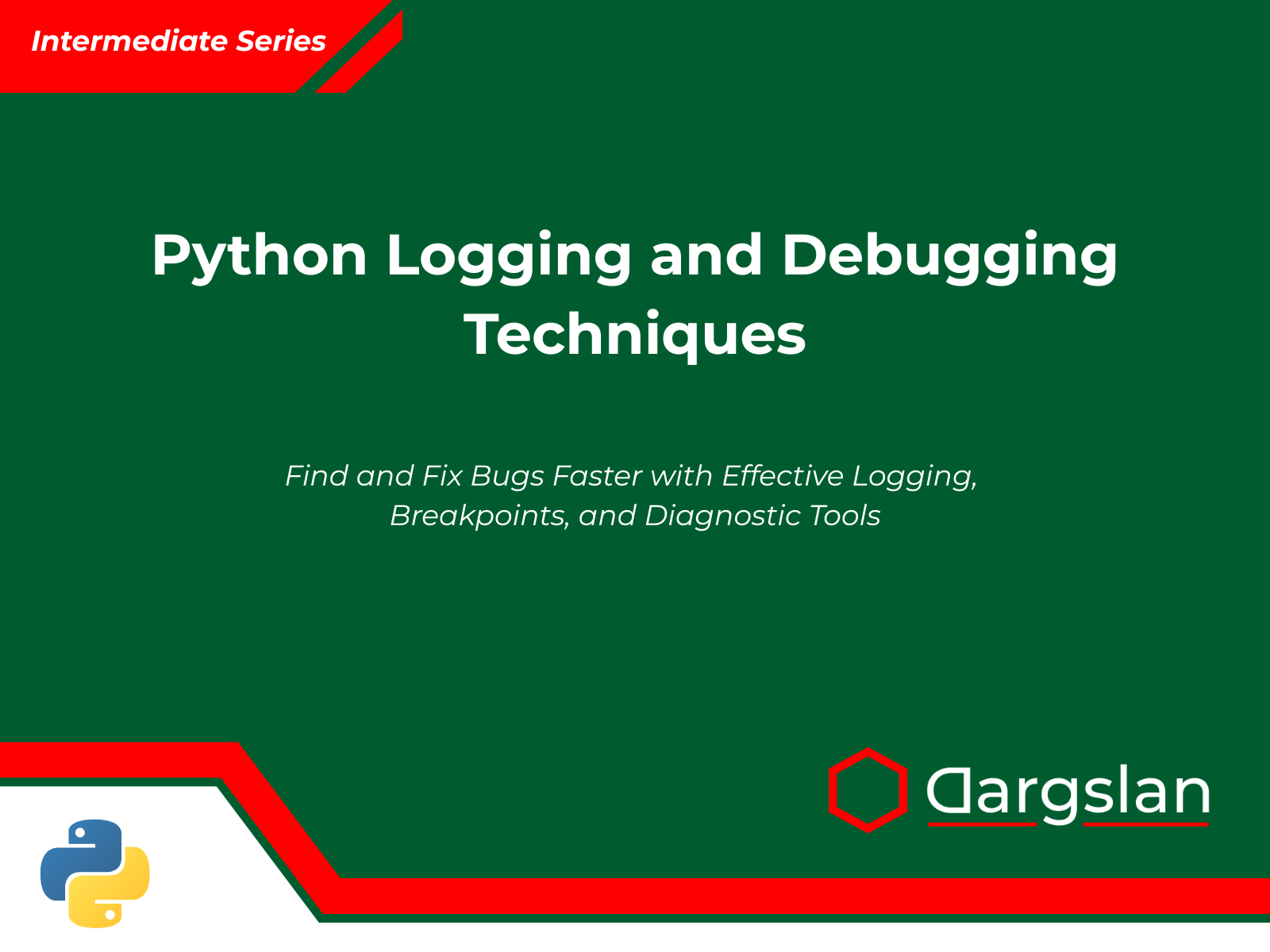
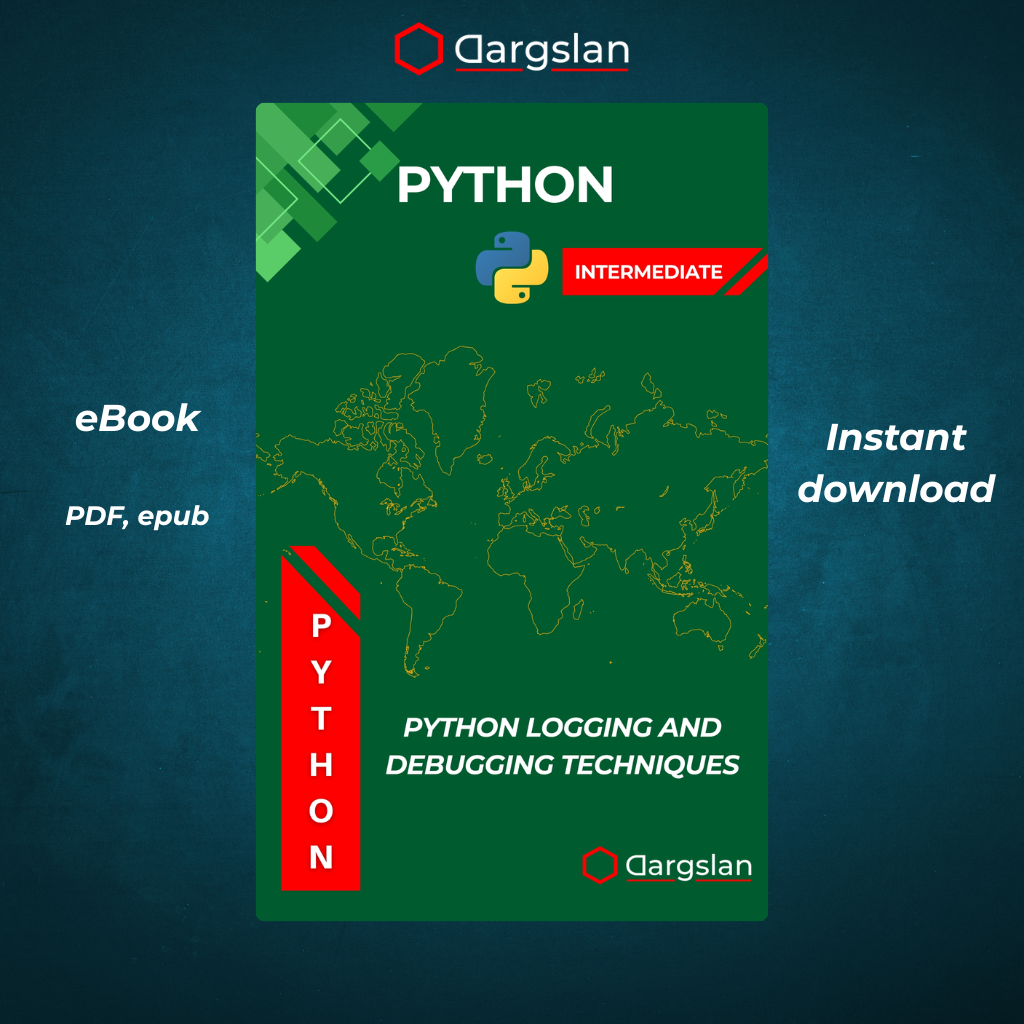
Python Logging and Debugging Techniques
Find and Fix Bugs Faster with Effective Logging, Breakpoints, and Diagnostic Tools
Python Logging and Debugging Techniques: A Comprehensive Book Review
Executive Summary
Python Logging and Debugging Techniques: Find and Fix Bugs Faster with Effective Logging, Breakpoints, and Diagnostic Tools is an essential resource for Python developers looking to master error detection, handling, and resolution. Written by Dargslan, this detailed guide takes readers from basic logging principles to advanced debugging strategies, providing practical solutions for real-world Python development challenges. With its systematic approach and comprehensive coverage, this book stands out as a valuable investment for programmers seeking to improve their Python troubleshooting skills.
Introduction to the Book
In today's complex software development landscape, efficient debugging and logging are no longer optional skills but fundamental requirements for professional Python developers. As applications grow in complexity, the ability to track program execution, identify errors, and diagnose issues becomes increasingly critical. This is where Python Logging and Debugging Techniques fills a significant gap in the programming literature.
This book systematically covers Python's built-in logging facilities and debugging tools, alongside third-party solutions that enhance the developer experience. What sets it apart is its balanced approach to theory and practice, providing both the conceptual understanding and hands-on techniques needed to implement effective logging and debugging strategies in Python projects of any size.
Target Audience
The book caters to a diverse range of Python developers:
- Intermediate Python developers looking to advance beyond print-statement debugging
- Professional software engineers seeking to implement industry-standard logging practices
- Team leads and project managers who need to establish consistent debugging protocols
- DevOps professionals responsible for monitoring and maintaining Python applications
- Self-taught programmers aiming to fill knowledge gaps in their diagnostic skillset
While beginners with basic Python knowledge will find value in the introductory chapters, the book's greatest benefits are realized by developers who have already encountered the limitations of ad-hoc debugging approaches in larger projects.
Detailed Chapter Analysis
Chapter 1: Why Logging and Debugging Matter
The opening chapter establishes the critical foundation for the entire book by examining the fundamental importance of systematic logging and debugging in Python development. The author articulates how proper diagnostic practices contribute to:
- Reduced development cycles through faster bug identification
- Enhanced collaboration among team members
- Improved application stability and maintainability
- More effective post-deployment monitoring
- Simplified customer support and issue resolution
Through compelling real-world examples, the author demonstrates how inadequate logging often leads to extended debugging sessions and production failures that could have been avoided. This chapter effectively motivates readers to invest in mastering these essential skills before diving into technical details.
Chapter 2: Introduction to Python's logging Module
Chapter 2 provides a thorough exploration of Python's built-in logging module, covering:
- The five standard logging levels (DEBUG, INFO, WARNING, ERROR, CRITICAL) and when to use each
- Basic logger configuration and customization
- Log formatting options and best practices
- The Logger hierarchy and its inheritance model
- Output destinations including console, files, and network targets
Code examples illustrate practical implementation patterns, showing how to move beyond simple print statements to structured logging approaches. The author expertly explains how Python's logging architecture provides flexibility while maintaining performance, using clear diagrams to visualize the flow of log messages through the system.
Chapter 3: Configuring Advanced Logging
Building on the fundamentals, Chapter 3 delves into sophisticated logging configurations:
- Creating and managing multiple loggers
- Implementing custom log formatters and handlers
- Configuring rotating file handlers to manage log file growth
- Using filters to control which messages get logged
- Leveraging dictConfig and fileConfig for complex setups
- Performance considerations and optimization techniques
Particularly valuable is the section on configuration through external files (JSON, YAML, INI), which demonstrates how to separate logging configuration from application code—a best practice for maintainable systems. The author provides thoughtful comparisons between different configuration approaches, helping readers select the right strategy for their specific needs.
Chapter 4: Modular Logging for Larger Applications
Chapter 4 addresses the challenges specific to implementing effective logging in larger, multi-module Python applications:
- Organizing loggers in a hierarchical structure that mirrors code organization
- Sharing logging configurations across modules
- Propagation rules and when to disable propagation
- Context-specific logging with adapter patterns
- Integration with third-party libraries and frameworks
- Testing logging implementations effectively
The author uses a sample application that grows in complexity throughout the chapter, illustrating how logging strategies must evolve as projects scale. Practical advice on naming conventions and organization patterns helps readers avoid common pitfalls in larger codebases.
Chapter 5: Logging in Production Environments
This critical chapter focuses on the transition from development to production logging:
- Configuring logging for different environments (dev, staging, production)
- Implementing secure logging practices for sensitive information
- Performance considerations for high-volume applications
- Integration with centralized logging systems (ELK stack, Graylog, etc.)
- Structured logging with JSON for better searchability
- Implementing asynchronous logging for performance-critical applications
- Monitoring and alerting based on log events
The section on structured logging deserves special mention for its thorough explanation of how modern log aggregation tools benefit from consistent JSON-formatted logs. Sample configurations for popular services like Logstash and Fluentd provide immediate practical value for operations teams.
Chapter 6: Intro to Python Debugging Techniques
Chapter 6 shifts focus to debugging, covering fundamental techniques including:
- Strategic use of print statements as a debugging approach
- Using Python's built-in pdb debugger
- Understanding the Python call stack and tracebacks
- Debugging with assertions and custom exceptions
- Logging-based debugging strategies
- Post-mortem debugging techniques
The author provides a balanced view of different debugging approaches, acknowledging that even simple techniques like print debugging have their place alongside more sophisticated tools. Clear examples demonstrate how to progressively isolate issues using systematic debugging workflows.
Chapter 7: Using IDE Debuggers (VS Code, PyCharm)
This hands-on chapter explores the powerful debugging features in popular Python IDEs:
- Setting up debugging environments in VS Code and PyCharm
- Effective use of breakpoints, including conditional and temporary types
- Step execution controls (step into, over, out)
- Evaluating expressions during debugging sessions
- Working with watch variables and data visualization
- Remote debugging configurations
- Memory and performance profiling tools
The side-by-side comparisons between VS Code and PyCharm debugging capabilities help readers leverage the full potential of their preferred development environment. Screenshots and detailed instructions make even advanced features accessible to newcomers.
Chapter 8: Logging vs Exceptions – How to Combine Them
Chapter 8 addresses a commonly misunderstood area—the relationship between logging and exception handling:
- Understanding when to log versus when to raise exceptions
- Techniques for logging exception details effectively
- Creating custom exception hierarchies that integrate with logging
- Context managers for cleaner exception handling and logging
- Balancing error recovery with diagnostic information capture
- Exception chaining and maintaining the full error context
The chapter includes thoughtful discussions about exception design philosophy in Python, presenting a nuanced view of how logging and exception handling complement each other rather than serving as alternatives.
Chapter 9: Real-World Debugging Scenarios
This practical chapter applies the book's concepts to complex debugging challenges:
- Diagnosing concurrency issues in multi-threaded applications
- Debugging network communication problems
- Finding memory leaks and performance bottlenecks
- Troubleshooting database interaction issues
- Resolving dependency conflicts in larger projects
- Debugging API integrations with third-party services
Each scenario follows a consistent problem-solving approach, from initial symptom identification through investigation and resolution. The author shows how combinations of logging and debugging techniques are typically required to solve real-world problems, rather than relying on a single approach.
Chapter 10: Best Practices and Diagnostic Mindset
The final chapter synthesizes the book's teachings into a cohesive diagnostic philosophy:
- Developing systematic troubleshooting workflows
- Building a debugging mindset that prioritizes evidence over assumptions
- Creating a logging strategy template for new projects
- Balancing diagnostic needs with code readability
- Conducting effective post-mortem analysis of production issues
- Continuous improvement of debugging skills through deliberate practice
This forward-looking chapter helps readers internalize not just specific techniques but the problem-solving approach that underlies effective debugging. The discussion of how to foster a diagnostic culture within development teams is particularly valuable for technical leaders.
Appendices: Practical Reference Materials
The four appendices provide quick-reference resources that enhance the book's practical value:
- logging Module Cheat Sheet: A comprehensive reference of logging module functions, classes, and parameters
- pdb Commands Reference: A complete guide to Python's built-in debugger commands with usage examples
- Sample Logging Configuration Files: Ready-to-use templates for common logging configurations
- Debugging Workflow Checklist: A step-by-step guide for methodical problem diagnosis
These appendices transform the book from merely educational to immediately useful in daily development work.
Writing Style and Presentation
Dargslan's writing style strikes an excellent balance between technical depth and accessibility. Complex concepts are explained clearly without oversimplification, and code examples are consistently practical rather than contrived. The progression from basic to advanced topics is logical, allowing readers to build their understanding incrementally.
The book features:
- Well-commented code samples that demonstrate best practices
- Diagrams illustrating conceptual relationships
- Highlighted "Pitfalls to Avoid" sections that prevent common mistakes
- "Pro Tip" callouts offering insider knowledge
- Chapter summaries that reinforce key learnings
The layout facilitates both cover-to-cover reading and reference use, with clear headings and consistent formatting that makes information easy to locate.
Technical Accuracy and Currency
The technical content reflects current Python best practices (as of Python 3.9+) while acknowledging backward compatibility concerns. The author addresses recent developments in the Python ecosystem, including:
- Updates to the logging module in recent Python versions
- Modern IDE debugging capabilities
- Integration with contemporary observability platforms
- Compatibility with asyncio and concurrent programming models
Where appropriate, the book notes differences between Python versions and provides guidance on maintaining code that works across version boundaries.
Comparative Analysis with Similar Resources
While several Python books touch on debugging and logging, few dedicate comprehensive attention to these specific skills. Compared to general Python programming books, Python Logging and Debugging Techniques offers:
- Much greater depth on logging configuration and best practices
- More systematic coverage of debugging workflows
- Practical guidance for real-world implementation scenarios
- Stronger focus on production-ready techniques
Compared to online tutorials and documentation, it provides:
- Cohesive progression from fundamentals to advanced concepts
- Integrated discussion of when and why to apply specific techniques
- Broader context for making architectural decisions
- Practical experience-based guidance on tool selection
Strengths and Potential Improvements
Key Strengths
- Comprehensive coverage of both logging and debugging in a single resource
- Practical orientation with immediately applicable techniques
- Balanced approach to simple and sophisticated debugging methods
- Strong focus on production readiness and scalability
- Well-structured progression from fundamentals to advanced topics
Areas for Potential Improvement
- More coverage of language-specific debugging techniques for Python libraries like NumPy and Pandas
- Additional examples for cloud-based deployment scenarios
- Expanded discussion of debugging Python in containerized environments
- More extensive coverage of automated testing as a debugging prevention strategy
Who Should Read This Book
This book is strongly recommended for:
- Python developers looking to level up their troubleshooting abilities
- Engineering teams establishing logging standards for Python projects
- System administrators responsible for maintaining Python applications
- Computer science students seeking practical debugging skills beyond theoretical knowledge
- Self-taught programmers wanting to fill gaps in their diagnostic approach
It is particularly valuable for those working on larger Python projects where ad-hoc debugging approaches become insufficient.
Conclusion
Python Logging and Debugging Techniques stands out as an authoritative and practical guide to a critical aspect of Python development. Its systematic approach to error diagnosis, comprehensive coverage of Python-specific tools, and focus on real-world application make it an essential addition to any Python developer's library.
By investing time in mastering the techniques presented in this book, developers will save countless hours of frustrating debugging sessions and build more robust, maintainable Python applications. For its target audience, this book represents an excellent return on investment both in terms of immediate skill development and long-term productivity improvement.
About the Author
Dargslan brings significant expertise in Python development to this book, with particular focus on application reliability and troubleshooting. Their experience across various Python domains—from web development to data processing systems—informs the practical advice throughout the text, offering readers insights that only come from extensive hands-on experience.
Keywords and Technical Topics Covered
Python debugging, Python logging module, exception handling, pdb debugger, Python IDE debugging, VS Code Python, PyCharm debugging, log configuration, structured logging, Python error tracking, Python troubleshooting, breakpoints, watch variables, debug console, remote debugging, Python stack traces, logging best practices, production logging, log rotation, JSON logging, ELK stack integration, debugging workflow, Python performance debugging, memory leak detection, thread debugging, application monitoring.
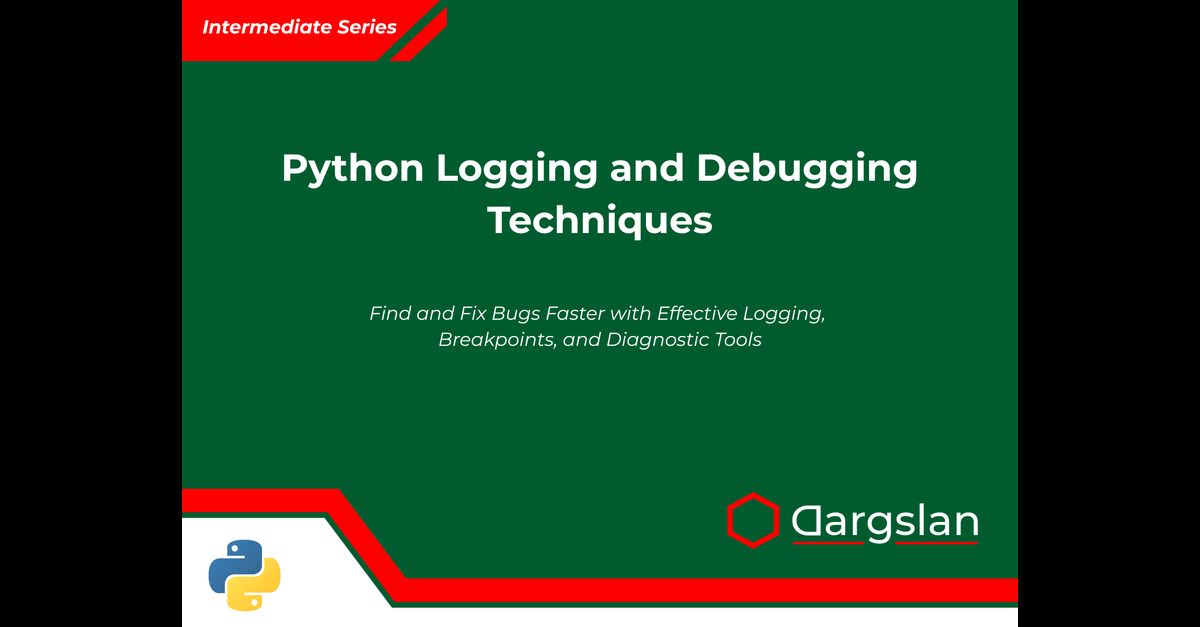
Python Logging and Debugging Techniques