Book Review: Python Error Handling for Beginners
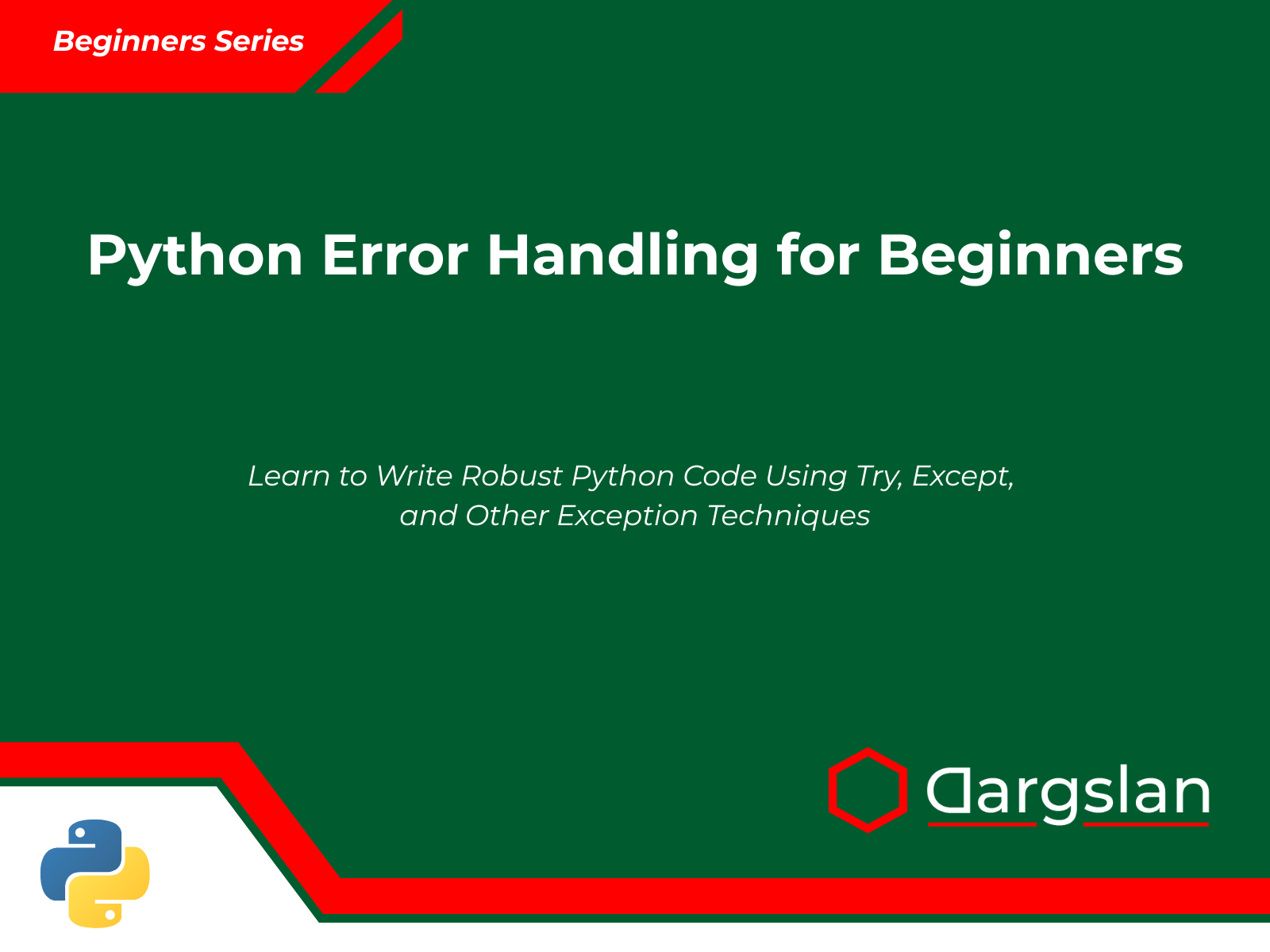
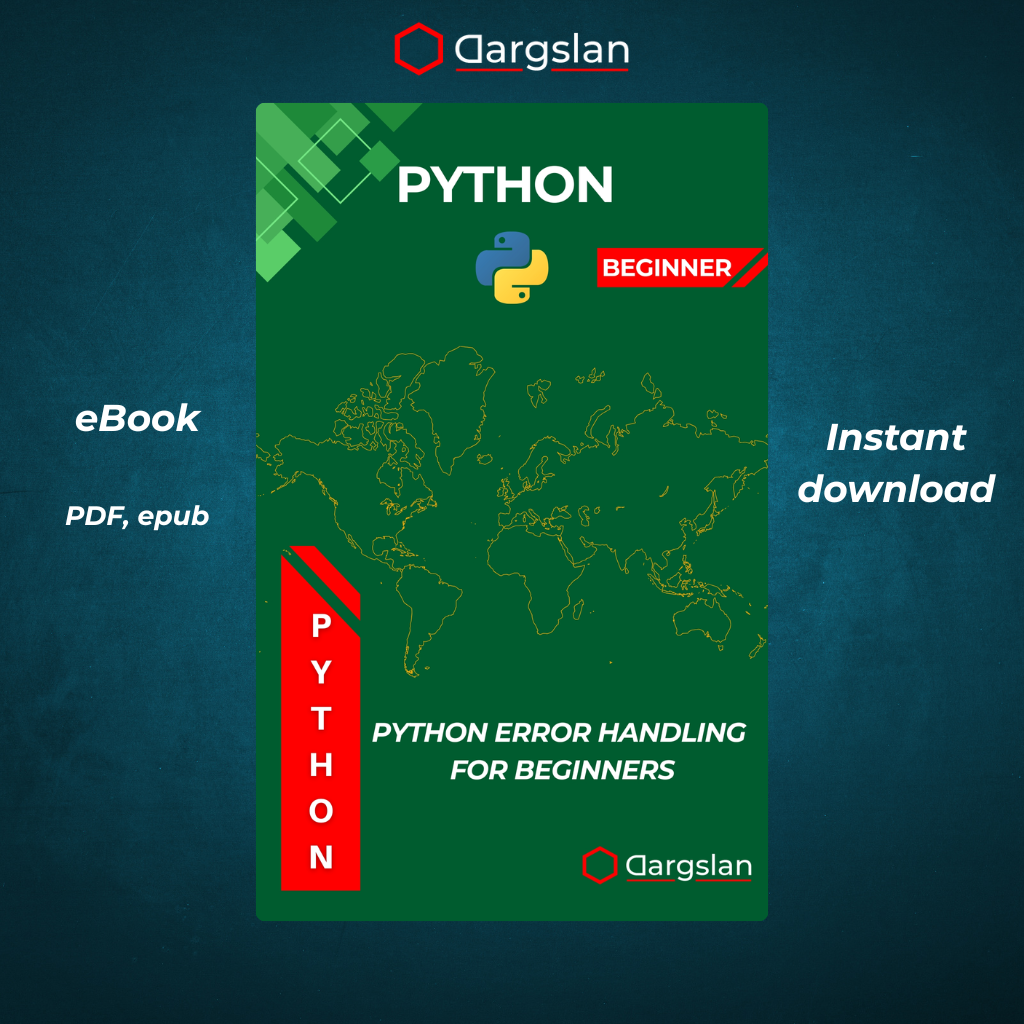
Python Error Handling for Beginners
Learn to Write Robust Python Code Using Try, Except, and Other Exception Techniques
Comprehensive Review: "Python Error Handling for Beginners" by Dargslan
A Deep Dive into Writing Robust Python Code
In today's increasingly complex programming landscape, error handling has become an essential skill for developers at all levels. Dargslan's newly released book, "Python Error Handling for Beginners: Learn to Write Robust Python Code Using Try, Except, and Other Exception Techniques," offers a comprehensive and accessible approach to this critical topic. This 10-chapter guide takes readers from the fundamentals of Python exceptions to advanced error management strategies, all while maintaining a beginner-friendly perspective.
Book Overview and Key Strengths
As Python continues to dominate programming language popularity rankings, the need for resources that address specialized aspects of the language grows. This book fills a significant gap in the Python literature by focusing exclusively on error handling—an area that many beginners struggle with but is essential for writing production-quality code.
The author effectively breaks down complex concepts into digestible chunks, starting with fundamental definitions of errors and exceptions before progressively introducing more advanced topics. The logical organization follows a learning curve that respects beginners' needs while building toward professional-level error handling techniques.
Exceptional Content Structure
The book's well-thought-out progression begins with basic concepts and systematically builds toward more complex applications:
- Foundation Building: The first two chapters establish a solid understanding of what exceptions are and introduce the fundamental try-except structure.
- Intermediate Techniques: Chapters 3-5 expand on these basics to cover multiple exception handling, else/finally blocks, and manually raising exceptions.
- Advanced Concepts: Chapters 6-8 delve into custom exception classes, real-world scenarios, and best practices.
- Practical Application: The final chapters and appendices provide hands-on projects and reference materials to reinforce learning.
This structure ensures readers not only understand the syntax but also develop the critical thinking skills needed to implement error handling strategies in their own projects.
Chapter-by-Chapter Analysis
Chapter 1: What Are Errors and Exceptions?
The book begins with clear definitions and distinctions between errors and exceptions in Python. Dargslan excels at explaining Python's error hierarchy, introducing the difference between syntax errors, runtime exceptions, and logical errors. Real-world examples demonstrate how Python's traceback messages can be interpreted, setting a strong foundation for the topics to follow.
Highlight: The visual representation of Python's exception hierarchy helps beginners understand how different error types relate to each other—knowledge that becomes crucial when handling specific exception types later.
Chapter 2: Introduction to try and except
This chapter breaks down the basic syntax and operation of Python's try-except blocks with remarkable clarity. Through progressively complex examples, readers learn how to capture exceptions and prevent program crashes.
Highlight: The author's approach of showing both "before" and "after" code examples vividly demonstrates the difference between unhandled exceptions and properly managed errors.
Chapter 3: Catching Multiple Exceptions
Building on the previous chapter, Dargslan explores how to handle different types of exceptions with specific responses. The explanation of exception hierarchies and how to catch exceptions by type is particularly strong.
Highlight: The coverage of the "exception as e" syntax and how to extract useful information from exception objects provides practical knowledge that many beginner resources overlook.
Chapter 4: else and finally Blocks
This chapter addresses the often-misunderstood else and finally clauses in Python's exception handling syntax. The author clearly explains when and why to use these additional blocks, with particularly good examples of resource management with finally.
Highlight: The context manager explanation and comparison with traditional try-finally patterns shows readers modern Python best practices.
Chapter 5: Raising Your Own Exceptions
The transition from handling exceptions to raising them marks an important shift in the book. Dargslan provides clear guidelines on when raising exceptions is appropriate and how to do it effectively.
Highlight: The discussion about choosing between returning error codes and raising exceptions shows mature programming judgment that will benefit readers as they design their own functions and libraries.
Chapter 6: Defining Custom Exception Classes
Creating custom exception hierarchies is explained with exceptional clarity in this chapter. The author demonstrates how to extend built-in exception types and design exception hierarchies that communicate meaning to users of your code.
Highlight: The real-world example of building an exception hierarchy for a file processing library shows how error handling contributes to API design.
Chapter 7: Real-Life Error Handling Scenarios
This practical chapter applies the book's concepts to common programming situations including file operations, network requests, database interactions, and user input validation.
Highlight: The section on implementing retry logic for transient errors (like network timeouts) demonstrates advanced patterns that even intermediate programmers will find valuable.
Chapter 8: Best Practices for Clean Error Handling
Dargslan synthesizes error handling wisdom into a cohesive set of principles, addressing exception granularity, error messages, error propagation, and documentation.
Highlight: The advice on balancing too much exception handling (defensive programming) versus too little demonstrates nuanced thinking often absent from programming texts.
Chapter 9: Debugging Tools and Tips
This chapter connects error handling with the debugging process, introducing tools like pdb, logging, and IDE debugging features while emphasizing a methodical approach to finding and fixing errors.
Highlight: The section on using logging instead of print statements for debugging shows production-ready practices that will serve readers well as they progress.
Chapter 10: Practice Projects
The book concludes with hands-on projects that require implementing error handling in realistic scenarios. These well-designed exercises reinforce earlier concepts while providing templates readers can adapt for their own work.
Highlight: The incremental difficulty progression of the projects supports readers as they apply their new skills, with optional extensions for those seeking additional challenges.
Appendices: Valuable Reference Material
The four appendices transform this book from merely instructional to genuinely useful as an ongoing reference:
- Common Python Exceptions List: A comprehensive catalog of built-in exceptions with explanations and example triggers
- Syntax Reference: A quick-reference guide to try-except-else-finally syntax patterns
- Debugging Checklist: A methodical troubleshooting approach for beginners
- Bonus Material: Additional resources and community links for continued learning
These reference sections add significant practical value, making the book useful long after the initial reading.
Writing Style and Accessibility
One of this book's greatest strengths is its approachable writing style. Dargslan strikes a perfect balance between technical accuracy and readability, avoiding both oversimplification and unnecessary jargon. Concepts are explained using relatable analogies, and code examples are consistently clean, well-commented, and follow Python best practices.
The author also effectively uses visual elements—diagrams, flowcharts, and syntax highlighting—to reinforce key concepts. This multi-modal approach accommodates different learning styles and helps clarify complex ideas.
Who Should Read This Book
This book is ideally suited for:
- Python beginners who have mastered basic syntax and are writing their first substantial programs
- Self-taught programmers looking to fill gaps in their understanding of error handling
- Students in formal Python courses seeking supplementary material on exceptions
- Developers from other languages transitioning to Python who need to understand Python's exception model
- Technical educators looking for clear explanations and examples to share with students
While the title specifies "for Beginners," even intermediate Python programmers will likely discover new techniques and best practices in the later chapters.
Comparison with Other Python Resources
Unlike general Python programming books that typically dedicate only a chapter to error handling, this focused guide provides comprehensive coverage that simply isn't available elsewhere in such an accessible format. Compared to advanced Python texts like "Fluent Python" or "Effective Python," Dargslan's book is more approachable for newcomers while still covering many of the same error handling principles.
The book also distinguishes itself from online tutorials and documentation by providing a cohesive learning path rather than fragmented information. The progression from basic concepts to real-world applications gives readers a complete mental model of Python's exception system.
Minor Criticisms
While the book excels in most areas, a few aspects could be improved:
-
More coverage of async error handling: As asynchronous programming becomes more common in Python, additional guidance on handling exceptions in async contexts would be valuable.
-
Limited coverage of third-party error handling libraries: The book focuses primarily on Python's built-in exception mechanisms, with limited discussion of helpful libraries like
tenacity
for retry logic or error reporting tools. -
Additional focus on testing exception handling: More material on writing tests that verify exception behavior would strengthen the connection between error handling and quality assurance.
These minor limitations are understandable given the book's focus and target audience, and they don't significantly detract from its overall value.
Practical Impact for Readers
After completing this book, readers will be equipped to:
- Identify and interpret Python error messages
- Implement appropriate exception handling for different scenarios
- Design robust functions and libraries with proper error reporting
- Debug exception-related issues efficiently
- Create custom exception hierarchies that communicate intent
- Apply industry best practices for error handling
- Write more reliable and maintainable Python code
These skills translate directly to real-world programming challenges, helping readers produce higher-quality code and spend less time troubleshooting.
Conclusion: A Must-Have Resource for Python Learners
"Python Error Handling for Beginners" fills an important niche in Python educational literature. By focusing exclusively on exception handling and error management, Dargslan has created a resource that elevates this crucial but often undertaught aspect of programming.
The book's clear explanations, practical examples, logical progression, and reference materials make it an outstanding value for anyone looking to improve their Python skills. The author's expertise and teaching ability shine throughout, making complex concepts accessible without oversimplification.
For beginners struggling with understanding and implementing error handling in their Python code, this book will prove invaluable. Even for those with more experience, the systematic approach and best practices will likely improve existing error management strategies.
In a programming landscape where robust, maintainable code is increasingly important, the skills taught in this book are essential. Dargslan has provided a clear roadmap to mastering Python error handling that will benefit readers at any stage of their programming journey.
Rating: 4.8/5.0
For its comprehensive coverage, clear explanations, practical examples, and valuable reference materials, "Python Error Handling for Beginners" earns an enthusiastic recommendation for anyone serious about Python programming.
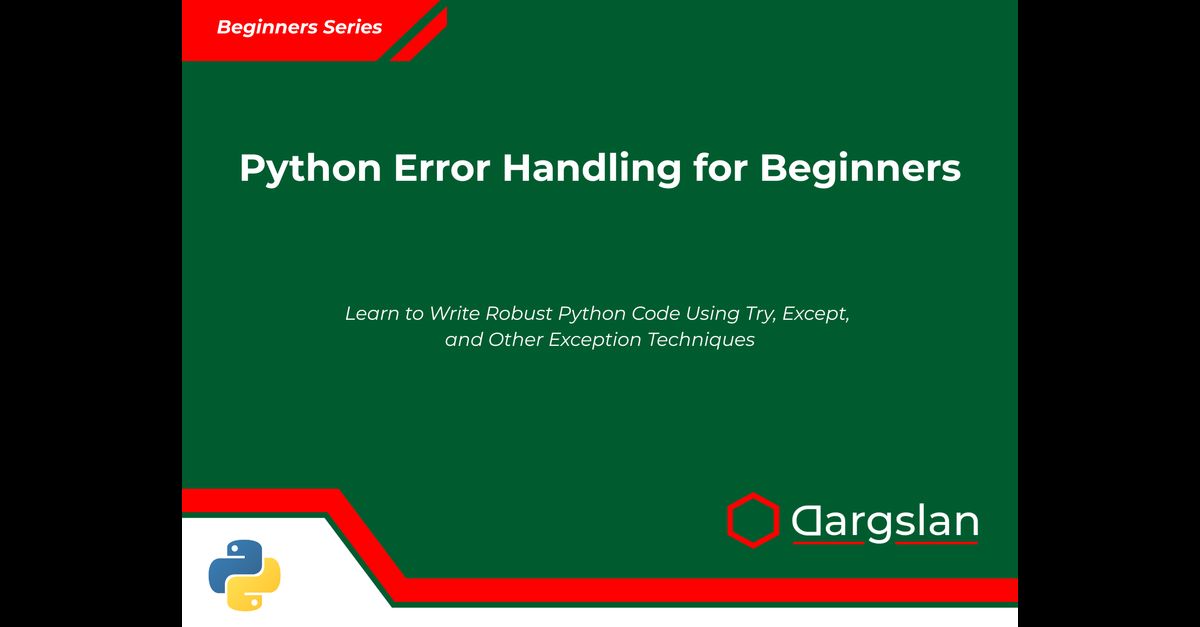
Python Error Handling for Beginners