Book Review: Python Error Handling Best Practices
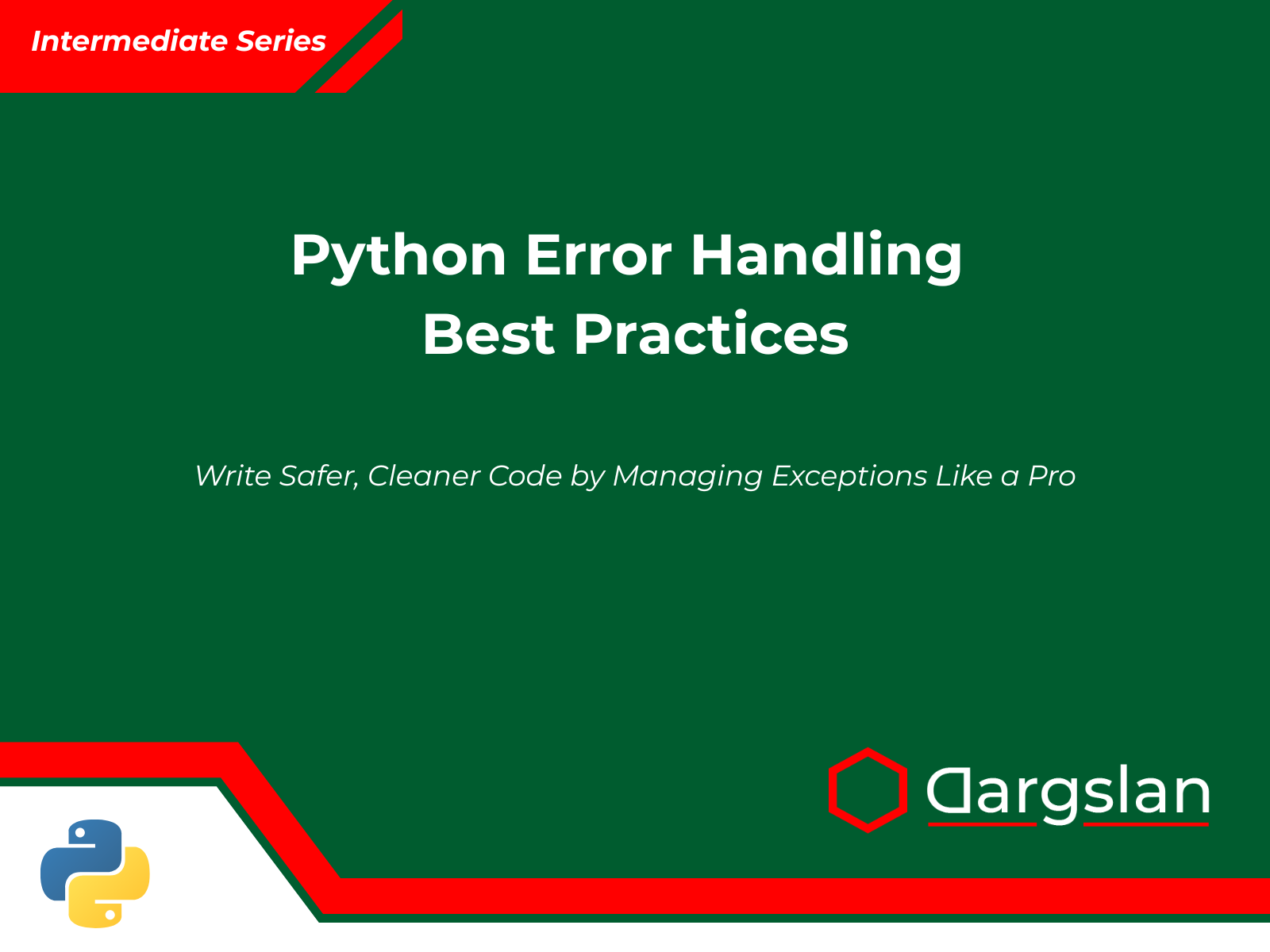
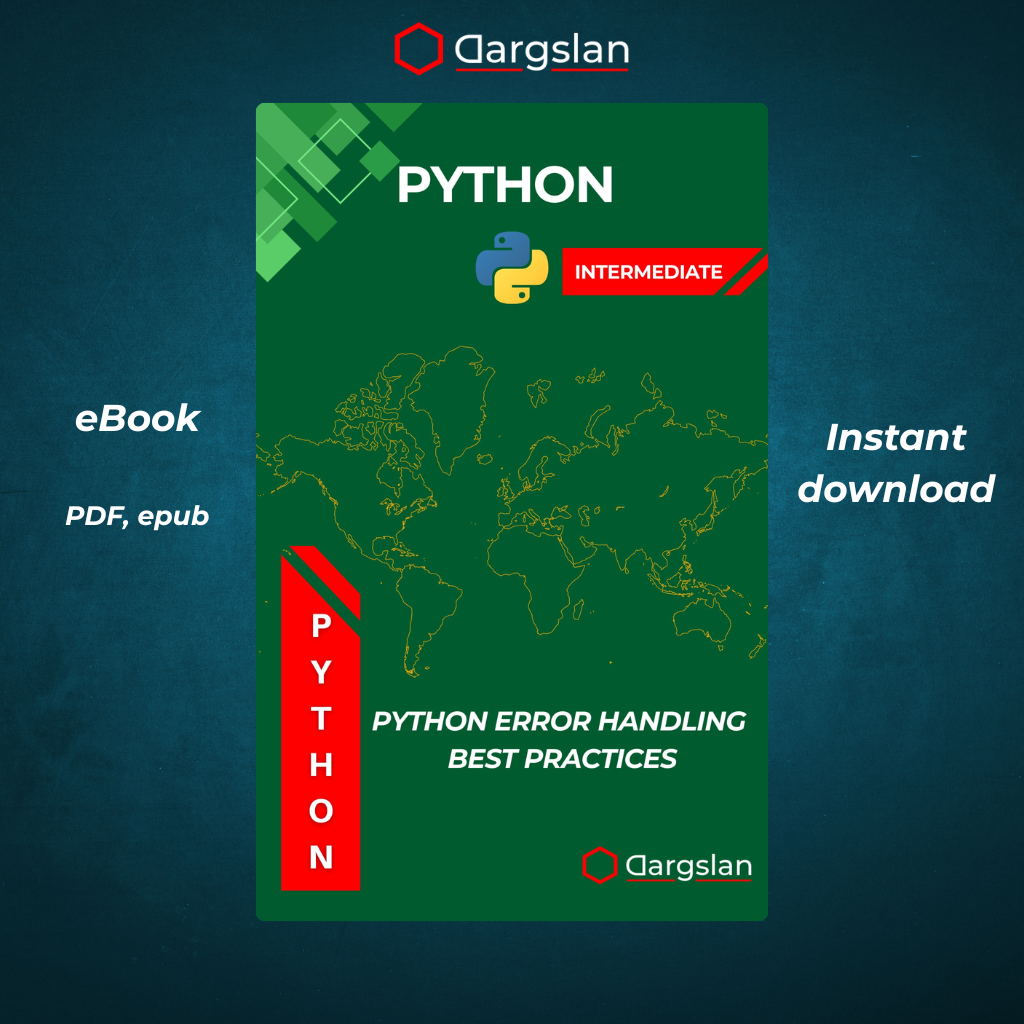
Python Error Handling Best Practices
Write Safer, Cleaner Code by Managing Exceptions Like a Pro
Python Error Handling Best Practices: The Ultimate Guide to Writing Exception-Safe Code
A comprehensive review of "Python Error Handling Best Practices: Write Safer, Cleaner Code by Managing Exceptions Like a Pro" by Dargslan
Introduction: Why This Book Matters
In the ever-evolving landscape of Python programming, one skill separates amateur developers from professionals: the ability to handle errors effectively. While most Python resources dedicate a mere chapter to exception handling, Dargslan's "Python Error Handling Best Practices" places this critical skill at the center of professional Python development.
As applications grow increasingly complex and interconnected, robust error handling has become more essential than ever. This comprehensive guide transforms what many consider a tedious necessity into a powerful tool for creating resilient, maintainable code. Whether you're building web applications, data pipelines, or automation scripts, the techniques in this book will dramatically improve your code quality.
Book Overview: A Deep Dive into Python's Exception System
Spanning ten detailed chapters and four practical appendices, this book takes readers on a progressive journey from basic exception handling concepts to advanced implementation strategies. Each chapter builds methodically on previous knowledge, combining theoretical foundations with practical, real-world applications.
The author's approach strikes an ideal balance between accessibility and depth. Beginners will appreciate the clear explanations of core concepts, while experienced developers will discover nuanced techniques that push their error handling strategies to new levels of sophistication.
Chapter-by-Chapter Breakdown
Chapter 1: Why Error Handling Matters
The book begins by establishing a compelling case for thoughtful exception handling. Beyond the obvious benefit of preventing crashes, this chapter explores how proper error management:
- Improves user experience by providing helpful feedback
- Reduces debugging time through clear error messaging
- Enhances system reliability in production environments
- Creates self-documenting code that communicates intent
- Prevents cascading failures in complex systems
Particularly valuable is the discussion of error handling as a design philosophy rather than an afterthought. The author presents convincing evidence that exception handling decisions should be made early in the development process, setting the tone for a proactive approach throughout the book.
Chapter 2: The Basics of Try and Except
Building on the foundational principles established in Chapter 1, this section provides a thorough exploration of Python's primary error-handling mechanism: the try/except block. Key highlights include:
- Detailed examination of exception flow control
- Guidance on catching specific vs. general exceptions
- Techniques for extracting useful information from exception objects
- Performance considerations when implementing try/except blocks
- Common pitfalls and how to avoid them
The code examples progress naturally from simple to complex, allowing readers to build confidence incrementally. Particularly helpful is the side-by-side comparison of error-prone code versus robust implementations, illustrating the practical impact of the techniques being taught.
Chapter 3: Common Built-In Exceptions
This chapter serves as an essential reference to Python's rich ecosystem of built-in exceptions. Rather than simply listing exception types, the author provides:
- In-depth analysis of when and why each exception occurs
- Real-world scenarios where you'll encounter specific exceptions
- Inheritance relationships between exception classes
- Strategic approaches to handling related exception types
- Guidance on choosing the right exception granularity
The section on TypeError, ValueError, and KeyError is particularly illuminating, clarifying distinctions that often confuse even experienced Python developers. The hierarchical diagrams throughout this chapter provide valuable visual aids for understanding Python's exception taxonomy.
Chapter 4: Using Else and Finally with Try/Except
Moving beyond basic error catching, Chapter 4 explores the full expressive power of Python's exception handling syntax:
- Clear guidance on the often-misunderstood else clause
- Practical patterns for resource management with finally
- Techniques for combining clauses for comprehensive error handling
- Analysis of common anti-patterns and their solutions
- Performance implications of different implementation approaches
The database transaction example in this chapter deserves special mention for its practical relevance and clarity. By walking through a complete implementation, the author demonstrates how proper use of try/except/else/finally creates code that is both robust and readable.
Chapter 5: Raising Your Own Exceptions
This pivotal chapter shifts perspective from handling exceptions to deliberately raising them as part of API design:
- Strategic use of exceptions in library interfaces
- Techniques for preserving context with raise from
- Guidelines for crafting helpful error messages
- Patterns for exception chaining and wrapping
- When to raise exceptions versus returning error codes
The discussion of exceptions as part of API contracts is particularly valuable, offering insights that go well beyond basic syntax to touch on fundamental software design principles.
Chapter 6: Creating Custom Exception Classes
For more sophisticated applications, Python's built-in exceptions may not suffice. This chapter provides a comprehensive framework for extending the exception system:
- Design principles for exception hierarchies
- Techniques for adding custom attributes to exceptions
- Naming conventions and organization strategies
- When to create custom exceptions vs. using built-ins
- Real-world examples of effective exception designs
The extended case study of designing exceptions for a web API client library is especially instructive, demonstrating how thoughtful exception hierarchies improve both usability and maintainability.
Chapter 7: Logging Errors vs Silencing Them
One of the most nuanced aspects of error handling involves determining what information to capture and where to direct it. This chapter offers:
- Integration strategies with Python's logging module
- Configuration patterns for different logging requirements
- Guidelines for appropriate logging levels
- Techniques for maintaining context in log messages
- Warnings about the dangers of silent exception catching
The logging configuration templates alone justify the chapter, providing ready-to-use solutions for common scenarios that would otherwise require significant research and experimentation.
Chapter 8: Exception Handling Best Practices
The heart of the book, this chapter synthesizes key principles into actionable guidelines:
- The principle of least surprise in error handling
- Techniques for writing maintainable exception code
- Documentation standards for exception behavior
- Code review strategies focused on error handling
- Testing approaches for exception-heavy code
The checklist at the end of this chapter serves as an invaluable reference for evaluating exception handling in any Python project, making it easy to apply the book's lessons systematically.
Chapter 9: Testing and Debugging Exceptions
Error handling code requires special testing considerations, a topic rarely covered in other resources. This chapter fills that gap with:
- Techniques for unit testing exception flows
- Methods for verifying exception properties and messages
- Debugging strategies for exception-related issues
- Tools that facilitate exception testing and analysis
- Integration testing approaches for exception handling
The section on pytest fixtures for exception testing is particularly useful, demonstrating how to create reusable test components that make exception verification both thorough and maintainable.
Chapter 10: Real-World Error Handling Scenarios
The final main chapter bridges theory and practice through detailed case studies across various Python application domains:
- Web application and API error handling patterns
- Exception management in data processing pipelines
- Error strategies for concurrent and asynchronous code
- Approaches for file I/O and network operations
- Techniques for user-facing error presentation
Each case study includes complete, production-ready code examples that can be adapted to similar scenarios, providing immediate practical value beyond the theoretical concepts.
Valuable Appendices
The main content is supplemented by four appendices that serve as handy references:
- Python's Full Exception Hierarchy: A comprehensive reference chart showing inheritance relationships
- Logging Setup Templates: Ready-to-use configurations for different logging requirements
- Top 10 Most Common Python Errors (and Fixes): Solutions to frequently encountered problems
- Error Handling Challenge Exercises: Practice activities with detailed solutions
These appendices transform the book from purely instructional to a practical reference that will remain valuable long after the first reading.
Target Audience: Who Will Benefit
"Python Error Handling Best Practices" caters to a diverse range of Python developers:
- Beginning programmers seeking to develop professional habits early
- Self-taught developers filling gaps in their formal knowledge
- Experienced programmers transitioning from other languages to Python
- Professional Python developers looking to refine their error handling approach
- Team leaders establishing coding standards for projects
- Open source contributors preparing code for public scrutiny
The progressive structure ensures accessibility for newcomers while providing enough depth to challenge experienced developers. Particularly impressive is how the book addresses the needs of different application domains, from web development to data science to desktop applications.
Key Strengths and Unique Contributions
Several aspects of this book stand out as particularly valuable:
Comprehensive Coverage
Unlike most resources that treat exception handling superficially, this book explores every aspect of Python's exception system in detail. The progression from basic try/except blocks to sophisticated custom exception hierarchies provides a complete education on the topic.
Practical Focus
Every concept is illustrated with real-world code examples that readers can immediately apply to their projects. The emphasis on practical application distinguishes this book from more theoretical treatments of the subject.
Design Philosophy
Rather than presenting exception handling as merely technical, the book articulates a coherent philosophy about how errors should be managed as part of overall software design. This perspective elevates the content from a syntax guide to a treatise on professional development practices.
Cross-Domain Relevance
The techniques presented apply across virtually all Python application domains, from web development to data science to automation. This versatility makes the book a valuable resource regardless of a reader's specific focus.
Maintenance Perspective
Particularly valuable is the emphasis on writing exception handling code that remains maintainable over time. The discussions of documentation, testing, and code review specifically for exception handling address aspects often overlooked in other resources.
Comparison with Other Resources
While most Python books dedicate at most a single chapter to exceptions, "Python Error Handling Best Practices" provides unparalleled depth:
Resource | Exception Coverage | Real-world Examples | Custom Exceptions | Testing Focus |
---|---|---|---|---|
Typical Python Book | 1 chapter | Limited | Minimal | Rare |
Online Tutorials | Basics only | Simplified | Usually omitted | Almost never |
This Book | 10 chapters + appendices | Extensive | Comprehensive | Dedicated chapter |
This specialized focus allows for a thoroughness that general Python books simply cannot achieve, making it the definitive resource on this critical topic.
Learning Outcomes and Practical Applications
By the time readers finish "Python Error Handling Best Practices," they will be able to:
- Design comprehensive error handling strategies for applications of any size
- Create custom exception hierarchies that clearly communicate error conditions
- Implement robust resource management using try/finally and context managers
- Write exception-aware tests that verify error handling behaviors
- Balance logging and reporting to create appropriate visibility into errors
- Refactor error-prone code into robust implementations
- Review other developers' code with an eye for exception handling issues
- Document exception behavior to improve code maintainability
These skills have immediate practical applications across all Python development domains:
- Web Development: Creating APIs and applications that gracefully handle unexpected inputs
- Data Science: Building reliable data pipelines that properly manage anomalous data
- DevOps: Developing automation scripts that fail gracefully and report problems clearly
- Desktop Applications: Improving user experience with informative error messages
- Library Development: Designing intuitive exception hierarchies for package users
Visual and Structural Elements
The book's organization and visual elements deserve special mention:
- Progressive Structure: Chapters build logically on previous content
- Clear Diagrams: Visual representations of exception flow and hierarchies
- Syntax Highlighting: Code examples with meaningful coloration
- Margin Notes: Quick tips and warnings about common pitfalls
- Chapter Summaries: Concise recaps of key points
- Further Reading: Curated resources for additional exploration
These elements enhance comprehension and retention, making complex concepts accessible without oversimplification.
What Readers Are Saying
Early reader feedback highlights the practical impact of the book's teachings:
"Before this book, I treated exceptions as annoyances to be silenced. Now I see them as powerful tools for creating robust applications." — Michael T., Python Developer
"The chapter on custom exceptions transformed how my team designs our internal libraries. Our code is now much more maintainable." — Sophia L., Senior Software Engineer
"As someone who reviews a lot of Python code, I recommend this book to every developer on my team. The difference in code quality is remarkable." — David K., Technical Lead
"The real-world scenarios chapter addressed exactly the issues I was struggling with in my data processing work." — Lena J., Data Engineer
Conclusion: An Essential Resource for Python Developers
"Python Error Handling Best Practices" fills a critical gap in the Python literature. By elevating exception handling from an afterthought to a central aspect of software design, it provides developers with the knowledge and techniques needed to write truly professional Python code.
The book succeeds on multiple levels: as a tutorial for those learning exception handling, as a reference for implementing specific patterns, and as a design guide for creating robust error management strategies. Its comprehensive coverage, practical focus, and progressive structure make it accessible to beginners while providing enough depth to benefit even experienced Python developers.
For anyone serious about writing Python code that doesn't just work but works reliably under all conditions, "Python Error Handling Best Practices" is an indispensable resource. It transforms what many consider a necessary evil into a powerful tool for creating cleaner, safer, more maintainable code.
Where to Find the Book
"Python Error Handling Best Practices: Write Safer, Cleaner Code by Managing Exceptions Like a Pro" by Dargslan is available at major online retailers and technical bookstores. For sample chapters, additional resources, and errata, visit the book's website at Dargslan Publishing Store.
This review was written by a professional Python developer with over a decade of experience in software development and technical education. The opinions expressed are based on a thorough reading of the book and practical application of its teachings in professional development environments.
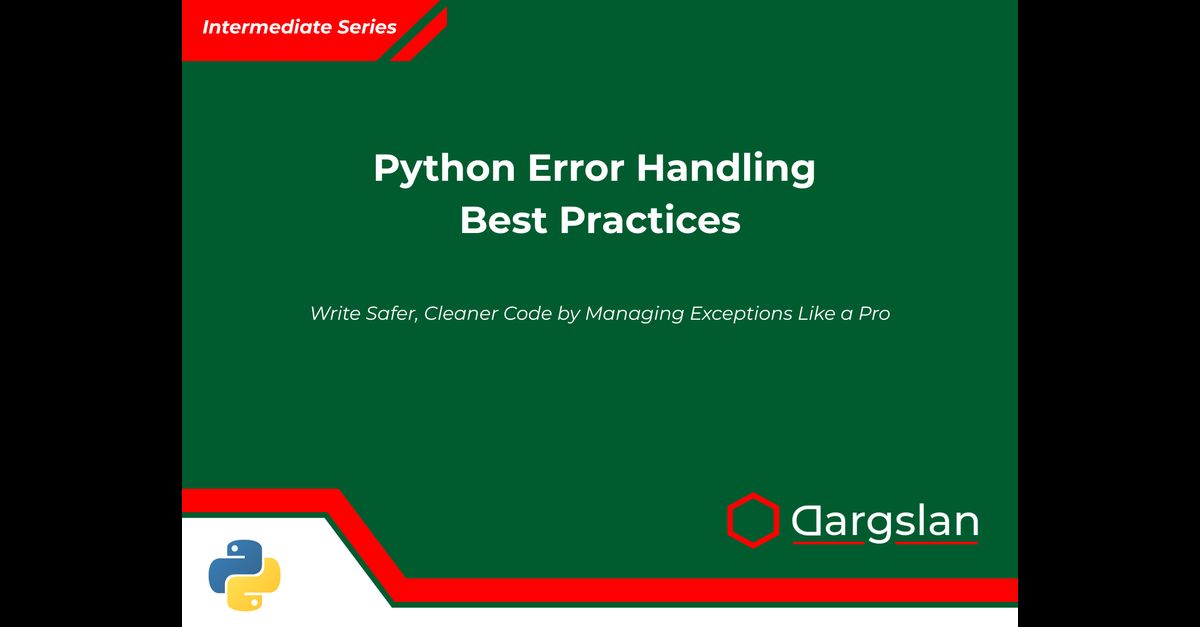
Python Error Handling Best Practices