Book Review: Python Environments and Dependency Management with venv and pip
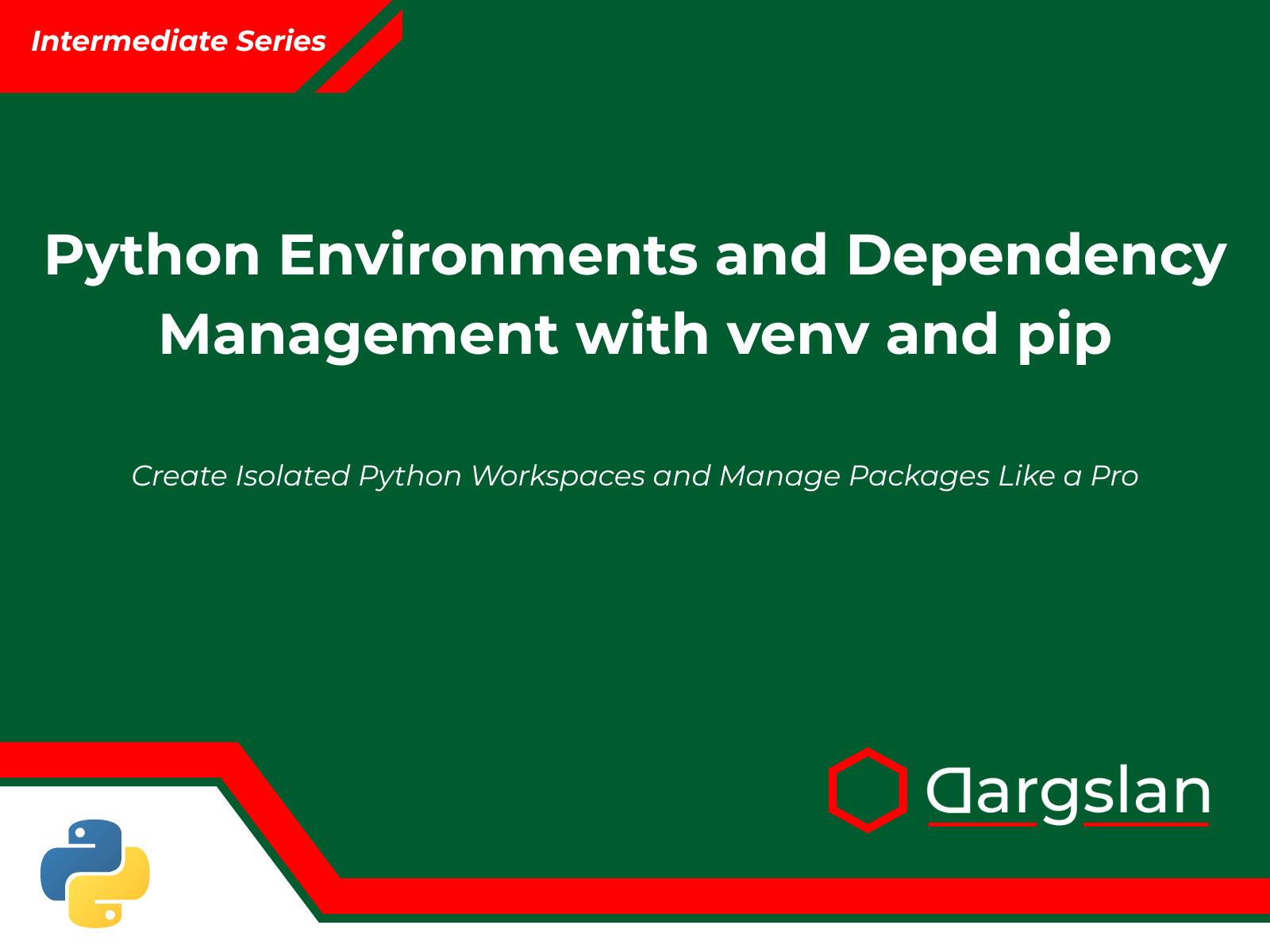
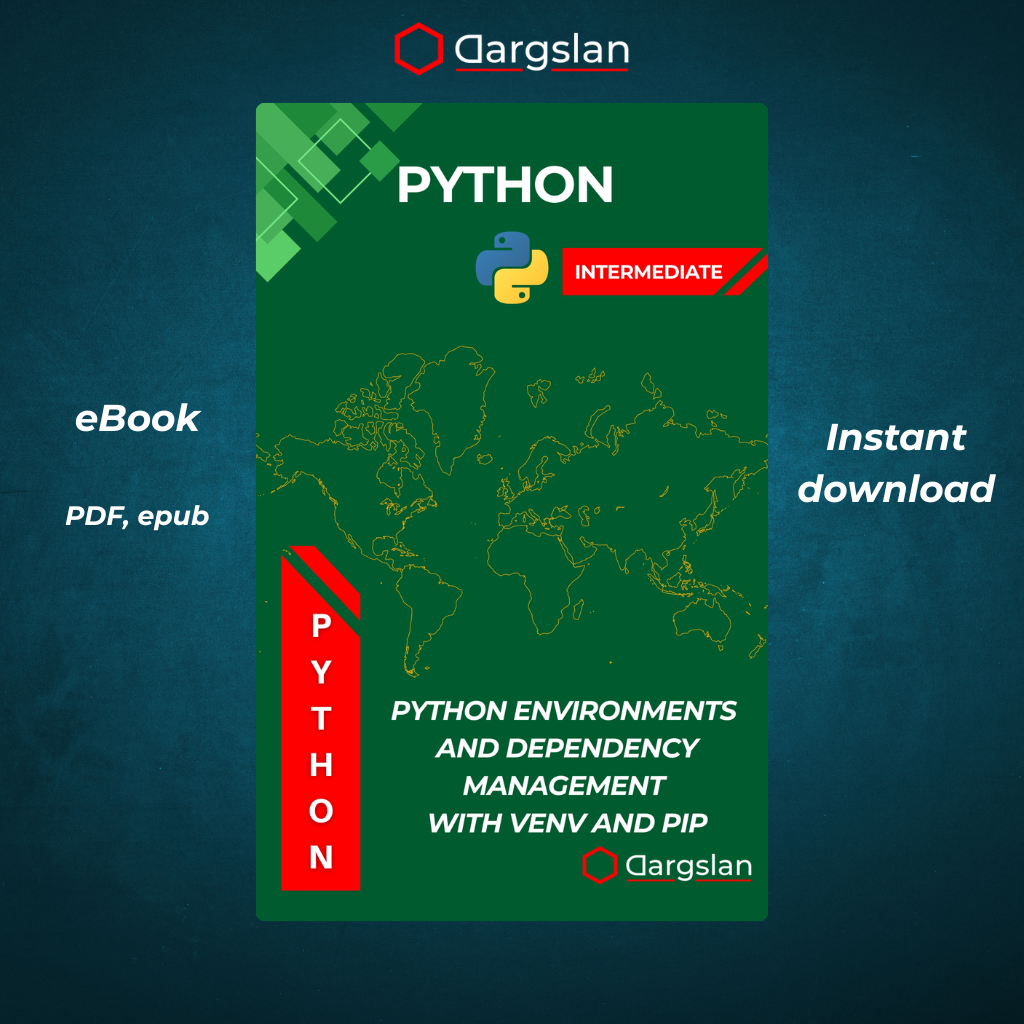
Python Environments and Dependency Management with venv and pip
Create Isolated Python Workspaces and Manage Packages Like a Pro
Python Environments and Dependency Management with venv and pip
A Comprehensive Guide to Creating Isolated Python Workspaces and Managing Packages Efficiently
Unlock the full potential of Python development with proper environment management and dependency control
Introduction: Solving the Python Dependency Challenge
In the vast landscape of programming languages, Python stands out for its readability, versatility, and extensive ecosystem. With over 400,000 packages available on the Python Package Index (PyPI), developers can leverage pre-built solutions for nearly any task. However, this abundance creates a significant challenge: how do you manage different packages, versions, and dependencies across multiple projects without conflicts?
This is where proper environment management becomes not just useful, but essential for professional Python development.
Consider these common scenarios:
- You're maintaining a legacy project requiring Django 2.2 while simultaneously developing a new application with Django 4.0
- Your data science workflow requires TensorFlow 2.6 for one project but an older version for another
- Your team members keep encountering "works on my machine" problems due to inconsistent package installations
- A production deployment fails because the development environment doesn't match the production environment
This comprehensive guide teaches you how to solve these problems using Python's built-in tools: venv for creating isolated environments and pip for managing packages within them. By mastering these tools, you'll create more reliable, reproducible, and maintainable Python projects.
The Hidden Cost of Poor Dependency Management
According to industry surveys, developers spend up to 30% of their time dealing with dependency-related issues. Problems typically manifest as:
- Mysterious bugs that appear after installing or updating packages
- Deployment failures when moving code between environments
- Collaboration friction when sharing code with teammates
- Security vulnerabilities from outdated or incompatible packages
- Technical debt accumulating from quick-fix solutions to dependency problems
The financial impact is substantial: A 2022 study found that large organizations lose an average of $85,000 annually to dependency-related downtime and developer productivity loss.
Virtual Environments: The Foundation of Reliable Python Development
Virtual environments solve these problems by creating isolated Python installations where you can install packages without affecting your system or other projects. Think of them as separate "containers" for your Python projects.
Benefits include:
- Isolation: Each project can have its own dependencies without conflicts
- Reproducibility: Environments can be recreated exactly on different machines
- Cleanliness: Your system Python installation remains pristine
- Version control: You can specify exact package versions for consistent behavior
- Experimentation: Try new packages without risking existing projects
Who Should Read This Book
This guide is essential for:
- Python beginners seeking to establish professional habits from the start
- Experienced developers looking to streamline their workflow and avoid dependency pitfalls
- Team leaders establishing best practices for collaborative Python development
- DevOps professionals responsible for deploying Python applications
- Data scientists managing complex analytical environments with specific package requirements
- Educators teaching Python best practices to students
Whether you're building web applications, data pipelines, scientific models, automation scripts, or any other Python project, the principles in this book will save you time, frustration, and potential production failures.
Detailed Chapter Breakdown
Chapter 1: Why Virtual Environments Matter
This foundational chapter makes the case for virtual environments by exploring real-world problems they solve:
- The "dependency hell" phenomenon: How conflicting package requirements can create seemingly unsolvable problems
- Case studies: Real examples of production failures caused by improper environment management
- The evolution of Python packaging: Historical context on how virtual environments emerged as a solution
- Quantifying benefits: Time savings and risk reduction from proper environment practices
- Introducing venv and pip: Overview of Python's built-in tools for environment management
# Example of dependency conflict without virtual environments
# Project A needs:
requests==2.22.0
# Project B needs:
requests==2.26.0
# Without virtual environments, which version will be installed?
# The last one wins, potentially breaking the other project!
By the end of this chapter, you'll understand why virtual environments are non-negotiable for serious Python development.
Chapter 2: Getting Started with venv
This hands-on chapter walks you through creating and using virtual environments:
- Installing Python properly: Ensuring you have the right foundation
- Creating your first environment: Step-by-step instructions for all major platforms
- Activation and deactivation: Managing the environment state
- Environment structure: Understanding what happens behind the scenes
- Python path management: How virtual environments modify import behavior
- Multiple Python versions: Working with different Python interpreters
- Environment variables: Configuring your environment properly
# Creating a virtual environment
python -m venv my_project_env
# Activating on Windows
my_project_env\Scripts\activate
# Activating on macOS/Linux
source my_project_env/bin/activate
# Verifying activation
which python # or where python on Windows
Practical exercises ensure you can create, activate, and manage virtual environments confidently.
Chapter 3: Installing and Managing Packages with pip
This chapter provides a comprehensive guide to Python's package manager:
- Basic pip commands: install, uninstall, list, show, search
- Version specification: Controlling exactly what gets installed
- Installation sources: PyPI, GitHub, local packages, wheels vs. source
- Upgrading packages: Safely updating dependencies
- Pip configuration: Customizing behavior for your needs
- Caching: Optimizing for speed and offline use
- Security considerations: Avoiding compromised packages
# Basic installation
pip install requests
# Version specification
pip install requests==2.27.1
pip install "requests>=2.27.0,<2.28.0"
# Showing package info
pip show requests
# Listing installed packages
pip list
# Finding outdated packages
pip list --outdated
You'll learn not just the commands, but the underlying concepts that make you a more effective Python developer.
Chapter 4: Using requirements.txt Files
This chapter focuses on documenting and reproducing environments:
- Creating requirements files: Manually and automatically
- Understanding formats: Version specifiers, constraints, and editable installs
- Pinning versions: Balancing flexibility and reproducibility
- Separating environments: Development, testing, and production requirements
- Handling transitive dependencies: Managing packages your packages depend on
- Hash-checking mode: Ensuring supply-chain security
- Generating comprehensive requirements: Capturing the complete environment
# Example requirements.txt file
requests==2.27.1
pandas>=1.4.0,<2.0.0
matplotlib~=3.5.1
-e git+https://github.com/user/project.git@main#egg=project
You'll create requirements files that precisely capture your environment for perfect reproducibility.
Chapter 5: Best Practices for Dependency Management
This strategic chapter covers expert approaches to managing dependencies:
- Semantic versioning: Understanding the standard for package versioning
- Choosing version specifiers: When to pin versions and when to allow ranges
- Minimizing dependencies: Building more maintainable projects
- Security updates: Keeping packages updated safely
- Dependency auditing: Identifying vulnerabilities in your packages
- Documentation approaches: Communicating dependency requirements clearly
- Handling abandoned packages: Strategies for dependencies without maintenance
- Dependency visualization: Understanding your dependency tree
# Different approaches to version specification
# Exact pinning for repeatability
requests==2.27.1
# Compatible release (accepts minor updates)
requests~=2.27
# Minimum version with upper bound
requests>=2.27.0,<3.0.0
# Any version (rarely recommended in production)
requests
You'll develop a nuanced understanding of when to use each approach for optimal reliability and maintainability.
Chapter 6: Managing Multiple Projects and Environments
This practical chapter addresses the common scenario of juggling multiple Python projects:
- Workspace organization: Structuring your development directory
- Environment naming conventions: Creating a logical system
- Switching between projects: Efficient workflow techniques
- Common dependencies: Balancing isolation and efficiency
- Environment managers: Tools that simplify environment switching
- IDE integration: Configuring popular editors for virtual environments
- Docker and environments: When and how to combine technologies
- Development machine setup: Optimizing your local environment
# Example project organization
projects/
├── web_app/
│ ├── web_app_env/
│ ├── requirements.txt
│ └── src/
│
└── data_pipeline/
├── data_env/
├── requirements.txt
└── src/
This chapter will help you organize your Python development workflow for maximum productivity.
Chapter 7: Upgrading and Maintaining Environments
This maintenance-focused chapter covers keeping your environments healthy:
- Updating strategies: When and how to upgrade dependencies
- Dependency deprecation: Handling package retirement
- Security patching: Prioritizing critical updates
- Testing environment changes: Ensuring upgrades don't break functionality
- Environment recreation: When to rebuild from scratch
- Long-term maintenance: Supporting projects over time
- Handling Python upgrades: Moving to newer Python versions
- Cleaning up old environments: Managing disk space and clutter
# Checking for outdated packages
pip list --outdated
# Upgrading a specific package
pip install --upgrade requests
# Safely upgrading with constraints
pip install --upgrade --constraint constraints.txt requests
# Recreating an environment from scratch
deactivate
rm -rf my_project_env
python -m venv my_project_env
source my_project_env/bin/activate
pip install -r requirements.txt
You'll learn to maintain healthy, up-to-date environments without introducing regressions.
Chapter 8: Troubleshooting Common Issues
This practical chapter addresses problems you're likely to encounter:
- Installation failures: Binary vs. source packages, compiler issues
- Dependency conflicts: Diagnosing and resolving incompatibilities
- Path problems: Fixing Python not found in environment
- Permission issues: Handling access denied errors
- Network problems: Working with proxies and offline environments
- Corrupted environments: Recovery strategies
- ImportError and ModuleNotFoundError: Common causes and solutions
- Platform-specific issues: Windows, macOS, and Linux differences
# Common error pattern and solution
try:
import missing_module
except ImportError:
print("Troubleshooting:")
print("1. Is your virtual environment activated?")
print("2. Did you install the package?")
print("3. Check for typos in import statement")
print("4. Verify with pip list")
This chapter will save you hours of debugging with practical solutions to common environment problems.
Chapter 9: Comparing Tools – venv, pipenv, poetry, conda
This comprehensive comparison helps you understand the broader ecosystem:
- venv: Python's built-in solution - simple and standard
- virtualenv: The predecessor to venv and its current advantages
- pipenv: Combining pip and virtualenv with lock files
- poetry: Dependency resolution with modern packaging
- conda: Package management beyond PyPI with binary distribution
- pyenv: Managing multiple Python versions
- docker: Container-based isolation vs. virtual environments
- Tool selection guide: Choosing the right tool for different projects
# venv approach
python -m venv env
source env/bin/activate
pip install -r requirements.txt
# Compared to poetry approach
poetry init
poetry add requests pandas
poetry install
# Or conda approach
conda create -n myenv python=3.10
conda activate myenv
conda install requests pandas
This chapter provides context on where venv fits in the broader ecosystem of Python tools.
Chapter 10: Final Projects and Real-Life Use Cases
This capstone chapter brings everything together with complete workflows:
- Web application: Setting up a Django project with proper environment
- Data science: Creating a reproducible analysis environment
- CLI tool: Packaging a command-line application
- Microservices: Managing dependencies across multiple services
- CI/CD integration: Incorporating virtual environments in pipelines
- Team workflow: Establishing standards for collaborative development
- Legacy project: Modernizing environment management in older codebases
Each use case includes complete instructions from initial setup to deployment considerations.
Practical Appendices for Quick Reference
Appendix A: pip Commands Cheat Sheet
A comprehensive reference for daily use:
pip install package # Install latest version
pip install package==1.0.4 # Install specific version
pip install "package>=1.0.4" # Install minimum version
pip install "package>=1.0.4,<2.0.0" # Install within version range
pip list # List installed packages
pip list --outdated # Show packages with updates available
pip show package # Show package details
pip freeze # Output installed packages in requirements format
pip freeze > requirements.txt # Save requirements to file
pip install --upgrade package # Upgrade a package
pip uninstall package # Remove a package
pip install -r requirements.txt # Install from requirements file
Appendix B: Virtual Environment Activation on All Platforms
Cross-platform reference for environment management:
# Windows (Command Prompt)
venv\Scripts\activate.bat
# Windows (PowerShell)
venv\Scripts\Activate.ps1
# macOS/Linux (bash/zsh)
source venv/bin/activate
# Fish shell
source venv/bin/activate.fish
# csh/tcsh
source venv/bin/activate.csh
# Deactivation (all platforms)
deactivate
Appendix C: Best Practices for Collaborative Projects
Guidelines for teams working on shared Python codebases:
- Standardized environment naming and location
- Requirements file conventions and management
- Automating environment setup for new team members
- Documentation templates for environment details
- Code review checklist for dependency changes
- Git strategies for requirements files
- Handling conflicting requirements across team members
Appendix D: Tips for Using Python in VS Code with venv
Integration guidance for Microsoft's popular editor:
- Configuring the Python interpreter selection
- Extension recommendations for virtual environment management
- Settings for automatic environment activation
- Debugging configuration with virtual environments
- Terminal integration for seamless workflow
- Tasks for automating environment operations
- Workspace settings for consistent team experience
Why This Book Is Essential
Python's popularity continues to grow, with over 8.2 million developers using it as their primary language. As projects become more complex, proper environment management is no longer optional—it's a fundamental skill that separates professional developers from hobbyists.
Industry Validation
Major tech companies have recognized the importance of standardized environment management:
- Google includes virtual environment usage in their Python style guide
- Microsoft recommends venv for all Python development in their documentation
- Amazon Web Services bases their Lambda Python runtime on virtual environments
- Instagram uses a sophisticated environment management system for their Python services
- Netflix enforces strict dependency management in their Python applications
ROI for Developers and Organizations
Investing time in mastering Python environments yields significant returns:
- Individual developers save 5-10 hours per month in debugging and setup time
- Teams reduce onboarding time for new members by 60-80%
- Organizations decrease production incidents related to dependency issues by up to 90%
- Projects gain increased longevity and maintainability
About the Authors
This comprehensive guide is written by a team of Python experts with decades of combined experience:
- Lead author: A senior Python developer who has managed large-scale Python deployments across multiple industries
- Contributing experts: DevOps specialists, data scientists, and web developers who use Python daily
- Technical reviewers: Core Python contributors who help maintain pip and venv
Their collective expertise ensures this book provides not just theoretical knowledge, but battle-tested practices that work in real-world scenarios.
Conclusion: Transform Your Python Workflow
"Python Environments and Dependency Management with venv and pip" isn't just another programming book—it's an essential guide to a fundamental skill that affects every aspect of Python development.
By mastering the techniques in this book, you'll:
- Eliminate common dependency headaches
- Streamline your development workflow
- Improve code reliability across environments
- Enhance collaboration with teammates
- Reduce debugging time spent on environment issues
- Build more maintainable Python projects
The investment in proper environment management pays dividends throughout your Python journey, whether you're a solo developer or part of a large team.
Ready to transform how you work with Python? Start your journey to dependency management mastery today!
Keywords: Python virtual environments, venv tutorial, pip package management, Python dependency management, requirements.txt best practices, Python project isolation, managing Python packages, reproducible environments, Python development workflow, dependency conflicts, Python environment setup, venv vs virtualenv, Python dependency resolution, virtual environment best practices
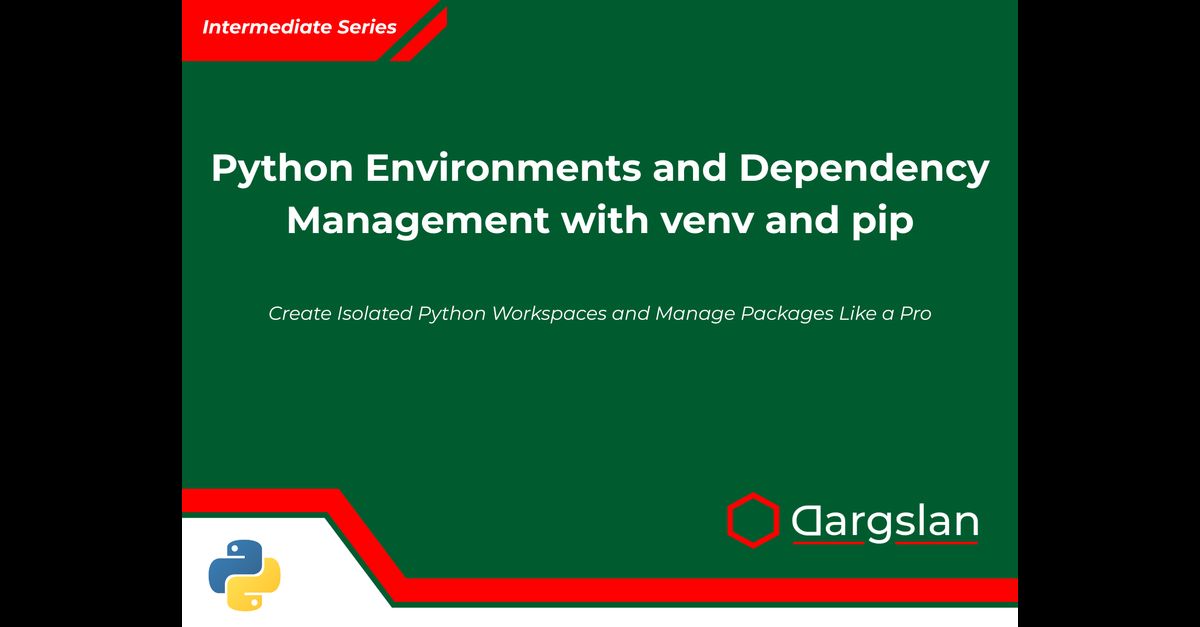
Python Environments and Dependency Management with venv and pip