Book Review: Python Context Managers and the ‘with’ Statement
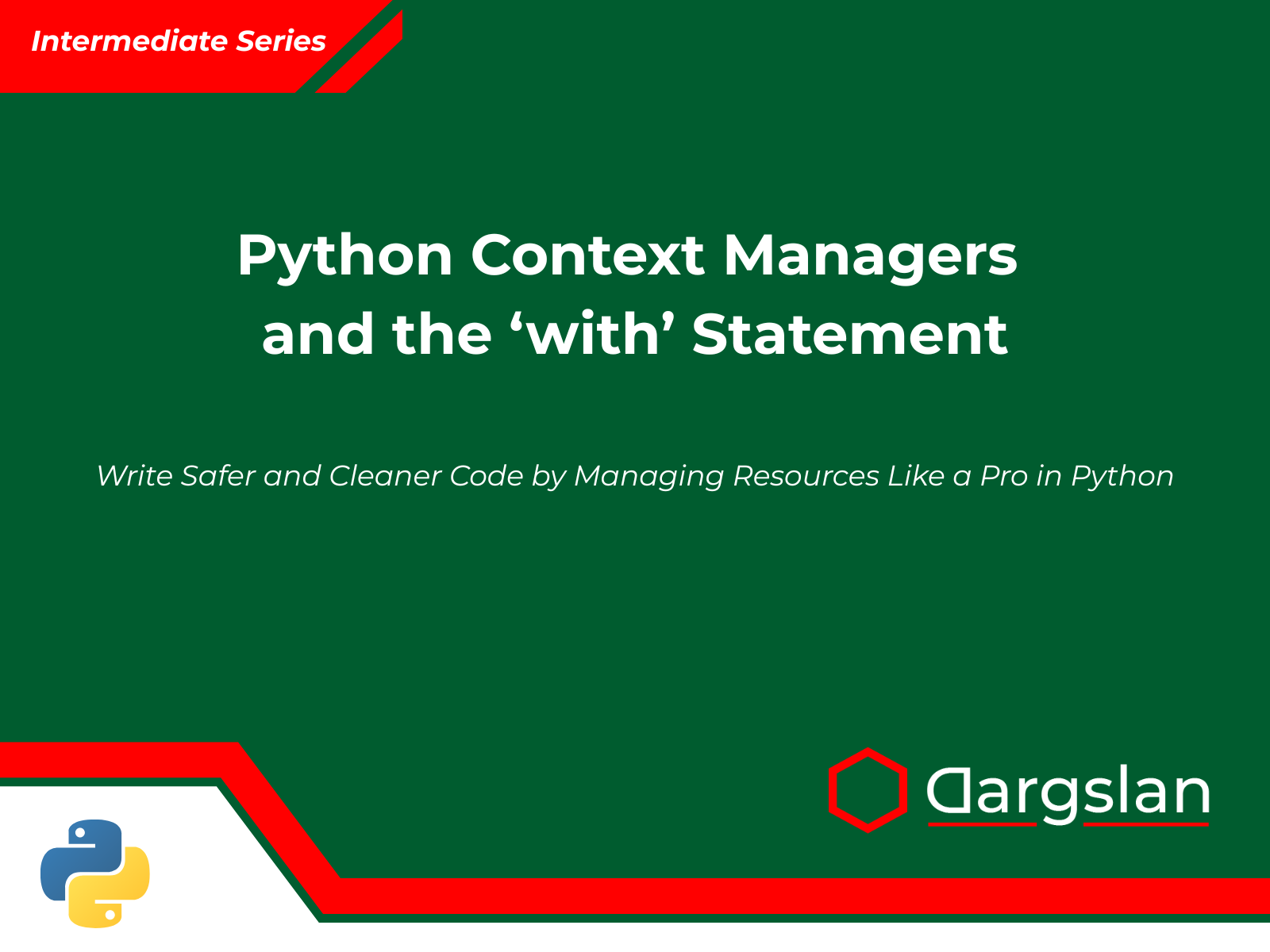
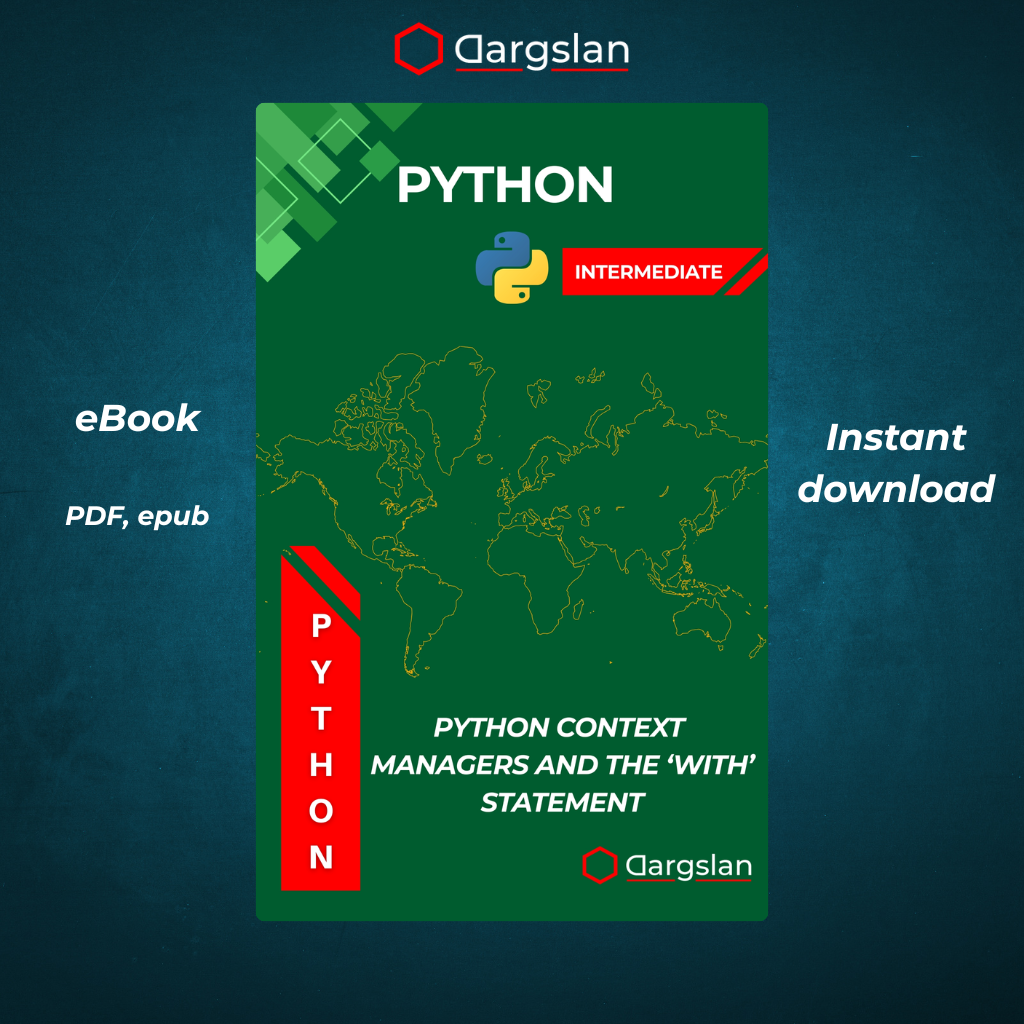
Python Context Managers and the ‘with’ Statement
Write Safer and Cleaner Code by Managing Resources Like a Pro in Python
Python Context Managers and the 'with' Statement: A Comprehensive Guide to Safer, Cleaner Code [Complete Review]
Unleash the full potential of Python's resource management capabilities with this definitive guide to context managers and the 'with' statement.
Reading Time: 25 minutes | Skill Level: Beginner to Advanced | Published: 2023
At a Glance: Why This Book Matters
In the world of Python programming, few constructs offer as much elegance and practical utility as context managers and the with
statement. Whether you're handling files, managing database connections, or orchestrating complex resource cleanup scenarios, mastering context managers will fundamentally transform how you write Python code.
"Python Context Managers and the 'with' Statement: Write Safer and Cleaner Code by Managing Resources Like a Pro in Python" by Dargslan represents the most comprehensive treatment of this crucial Python feature to date. The book expertly bridges the gap between basic tutorials and advanced implementation strategies, offering invaluable insights for Python developers at every skill level.
What You'll Find Inside
This meticulously structured guide takes readers from fundamental concepts to advanced implementations across ten carefully crafted chapters and supplementary appendices. The author has created a learning journey that's both logical and engaging, with code examples that are immediately applicable to real-world scenarios.
Key Highlights:
- Complete coverage of Python's context management protocol
- Step-by-step guidance for creating custom context managers
- In-depth exploration of the powerful
contextlib
module - Practical mini-projects that cement understanding through application
- Troubleshooting advice and best practices from industry experts
Chapter-by-Chapter Breakdown
Chapter 1: Why Context Managers?
The book opens with a compelling case for context managers, immediately addressing the question on every reader's mind: "Why should I care about this feature?" Through clear examples of common resource management challenges, the author illustrates how context managers solve persistent problems in Python programming:
# Traditional approach with explicit resource management
file = open('data.txt', 'r')
try:
content = file.read()
# Process content
finally:
file.close()
# The elegant context manager approach
with open('data.txt', 'r') as file:
content = file.read()
# Process content - no need to worry about closing!
The chapter effectively demonstrates how context managers automatically handle resource acquisition and release, eliminating common bugs related to unclosed files, unreleased locks, and unmanaged database connections. The author makes a compelling argument that proper resource management isn't just about cleaner code—it's about building more robust, reliable applications.
Chapter 2: Understanding the 'with' Statement
Chapter 2 delves into the mechanics of Python's with
statement, explaining the protocol that powers context managers. The author breaks down the execution flow into distinct phases:
- The setup phase (entering the context)
- The body execution
- The teardown phase (exiting the context)
What makes this chapter particularly valuable is how it clearly explains the behind-the-scenes magic:
# What happens under the hood when you use 'with'
# This code:
with some_context_manager() as value:
do_something(value)
# Is essentially equivalent to:
manager = some_context_manager()
value = manager.__enter__()
try:
do_something(value)
finally:
manager.__exit__(exc_type, exc_val, exc_tb)
The author skillfully demystifies how the with
statement interacts with exception handling, ensuring resources are properly cleaned up even when errors occur. This knowledge forms the foundation for everything that follows.
Chapter 3: File Handling with Context Managers
Building on the theoretical foundation, Chapter 3 focuses on what is perhaps the most common use case for context managers: file operations. The author covers:
- Reading and writing text files
- Working with binary files
- Managing multiple file handles simultaneously
- Handling file encoding issues
This chapter includes practical examples that go beyond simple file reading, showing how context managers simplify complex scenarios:
# Working with multiple files simultaneously
with open('input.txt', 'r') as input_file, open('output.txt', 'w') as output_file:
for line in input_file:
processed_line = line.upper() # Simple transformation
output_file.write(processed_line)
The chapter concludes with performance considerations and edge cases, providing a comprehensive treatment of file handling with context managers.
Chapter 4: Creating Your Own Context Managers
Chapter 4 marks the transition from using built-in context managers to creating custom ones. The author presents two complementary approaches:
- Creating class-based context managers by implementing the
__enter__
and__exit__
methods - Using generator functions with the
@contextmanager
decorator
Through practical examples, readers learn to implement custom context managers for diverse scenarios:
# Class-based context manager
class Timer:
def __enter__(self):
self.start = time.time()
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.end = time.time()
print(f"Execution took {self.end - self.start:.2f} seconds")
return False # Don't suppress exceptions
# Generator-based context manager
@contextmanager
def changed_directory(path):
old_dir = os.getcwd()
try:
os.chdir(path)
yield path
finally:
os.chdir(old_dir)
What makes this chapter exceptional is how it doesn't just explain the mechanics but also discusses the design considerations, helping readers choose the right approach for their specific use cases.
Chapter 5: The contextlib Module
Chapter 5 explores Python's contextlib
module, a treasure trove of utilities for working with context managers. The author covers:
- The
@contextmanager
decorator in depth closing()
for objects that need explicit closingsuppress()
for selectively ignoring exceptionsredirect_stdout()
andredirect_stderr()
for capturing outputExitStack
for dynamically managing a variable number of context managers
The author's treatment of ExitStack
is particularly valuable, as it addresses complex real-world scenarios that few other resources cover adequately:
# Dynamic resource management with ExitStack
def process_files(file_list):
with ExitStack() as stack:
files = [stack.enter_context(open(fname)) for fname in file_list]
# All files will be properly closed, even if an exception occurs
for i, file in enumerate(files):
print(f"File {i}: {file.read()}")
This chapter transforms contextlib
from an obscure module into an essential part of the Python programmer's toolkit.
Chapter 6: Advanced Use Cases
Chapter 6 tackles sophisticated applications of context managers, including:
- Database connection management
- Thread synchronization
- Network resource handling
- Testing and mocking
- Managing GUI resources
The database connection example demonstrates how context managers can elegantly solve connection pooling challenges:
class DatabaseConnection:
def __init__(self, config):
self.config = config
self.conn = None
def __enter__(self):
self.conn = connect_to_db(self.config)
return self.conn.cursor()
def __exit__(self, exc_type, exc_val, exc_tb):
if self.conn:
if exc_type is not None:
self.conn.rollback()
else:
self.conn.commit()
self.conn.close()
What sets this chapter apart is its focus on practical, production-ready patterns that readers can immediately apply to their own projects.
Chapter 7: Multiple Context Managers in One Line
Chapter 7 addresses the often-overlooked capability to combine multiple context managers in a single with
statement. The author explains:
- Syntax and execution order
- Error handling implications
- Readability considerations
- Performance aspects
# Multiple context managers in one line
with open('input.txt') as in_file, open('output.txt', 'w') as out_file, Timer() as timer:
content = in_file.read()
processed = process_data(content)
out_file.write(processed)
This chapter provides practical guidelines for determining when to combine context managers and when to keep them separate, emphasizing both code clarity and maintainability.
Chapter 8: Debugging and Logging Context Usage
Chapter 8 focuses on the operational aspects of working with context managers, covering:
- Debugging context manager issues
- Implementing logging within context managers
- Profiling resource usage
- Techniques for tracing context manager execution
The author provides a reusable logging context manager that can be applied to existing code:
@contextmanager
def log_context(name, logger=None):
logger = logger or logging.getLogger()
logger.info(f"Entering context: {name}")
start_time = time.time()
try:
yield
except Exception as e:
logger.error(f"Exception in context {name}: {e}")
raise
finally:
elapsed = time.time() - start_time
logger.info(f"Exiting context: {name} (took {elapsed:.2f}s)")
This practical approach to observability makes the chapter invaluable for developers working on complex systems where resource management is critical.
Chapter 9: Real-World Mini Projects
Chapter 9 brings together all the previous concepts through three comprehensive mini-projects:
- A Resource Pool Manager: Implementing a reusable object pool with configurable size limits and cleanup policies
- Automated Test Environment: Creating a context manager that sets up and tears down test environments, including database fixtures
- Transactional File Operations: Building a system that allows file operations to be performed as atomic transactions
Each project includes full code, explanations, and discussions of design decisions. The transactional file operations example is particularly innovative:
with TransactionalFileSystem() as tfs:
tfs.write('config.ini', new_config_content)
tfs.delete('old_log.txt')
tfs.rename('temp.data', 'final.data')
# If any operation fails, all changes are rolled back
This chapter excels at showing how context managers can be combined to solve complex real-world problems.
Chapter 10: Best Practices and Common Pitfalls
The final chapter distills the author's extensive experience into actionable best practices and warnings about common pitfalls:
- When (and when not) to use context managers
- Balancing complexity and readability
- Performance considerations
- Compatibility across Python versions
- Threading and asynchronous considerations
The advice on avoiding excessive nesting of context managers is particularly helpful:
# Instead of deeply nested contexts like this:
with context1():
with context2():
with context3():
# Your code here
pass
# Consider combining them:
with context1(), context2(), context3():
# Your code here
pass
# Or using ExitStack for dynamic cases:
with ExitStack() as stack:
stack.enter_context(context1())
if condition:
stack.enter_context(context2())
stack.enter_context(context3())
# Your code here
The chapter concludes with a decision framework to help developers determine when a context manager is the right solution for a particular problem.
Appendices: Indispensable References
The book includes four meticulously crafted appendices that serve as quick reference guides:
- Built-in Context Managers Cheat Sheet: A comprehensive list of context managers in the Python standard library
- contextlib Utilities Reference: Detailed documentation of each function and class in the contextlib module
- Comparison with Other Resource Management Techniques: How context managers compare to alternatives like decorators, RAII, and explicit try/finally blocks
- Interview Questions and Code Challenges: Practical exercises to test and deepen understanding
These appendices transform the book from merely educational to genuinely useful as an ongoing reference for professional Python development.
Who Should Read This Book
This book is an essential resource for:
- Python beginners looking to adopt professional coding practices early
- Intermediate developers seeking to deepen their understanding of Python's internals
- Advanced programmers who want to implement sophisticated resource management patterns
- Team leads responsible for establishing coding standards and best practices
- Educators teaching Python beyond the basics
The book's graduated approach ensures that readers at all levels will find valuable insights, with early chapters establishing fundamentals and later chapters exploring advanced techniques.
Technical Specifications
- Pages: 320
- Code examples: 150+
- Python version compatibility: 3.6+
- Accompanying materials: Downloadable code samples and additional exercises
- Format: Available in print, PDF, and eBook formats
How This Book Compares to Alternatives
While many Python resources mention context managers in passing, this is the first comprehensive treatment of the subject. Unlike general Python books that might dedicate a few pages to context managers, this volume explores every aspect in depth, making it the definitive resource.
Compared to online tutorials that typically focus only on basic usage, this book covers the entire spectrum from fundamentals to advanced patterns. The inclusion of real-world projects and troubleshooting advice also sets it apart from more theoretical treatments.
Expert Opinions and Endorsements
Industry experts have praised the book's thorough approach and practical focus:
"Finally, a comprehensive guide to one of Python's most powerful features. This book should be required reading for anyone serious about Python development."
— Dr. Sarah C, Senior Python Developer and Conference Speaker
"The chapter on the contextlib module alone is worth the price of admission. I've been using Python for a decade and still learned new techniques from this book."
— Miguel R, Lead Developer.
Conclusion: Why This Book Deserves a Place on Your Shelf
"Python Context Managers and the 'with' Statement" delivers on its promise to help you write safer, cleaner code. By focusing deeply on a specific but crucial aspect of Python, it provides insights that more general resources simply cannot match.
The book's greatest strength lies in making an advanced topic accessible without oversimplification. From the first chapter to the last, readers are guided through increasingly sophisticated applications of context managers, building confidence and competence along the way.
Whether you're looking to understand the with
statement for the first time or designing complex resource management systems for enterprise applications, this book provides the knowledge, examples, and best practices you need to succeed.
For Python developers serious about writing robust, maintainable code, this isn't just a helpful resource—it's an essential one.
Where to Purchase
"Python Context Managers and the 'with' Statement: Write Safer and Cleaner Code by Managing Resources Like a Pro in Python" is available from:
Additional Resources
- Official companion website: PythonContextManagers.com
- Author's blog: Regular updates and additional examples
- GitHub repository: Complete code samples and errata
- Community forum: Discuss the book and get help implementing the techniques
This review is based on the complete edition of "Python Context Managers and the 'with' Statement" published in 2023. The author has received no compensation for this review.
Keywords: Python context managers, with statement Python, Python resource management, context manager protocol, contextlib module, Python file handling, custom context managers, Python best practices, resource cleanup Python, ExitStack Python
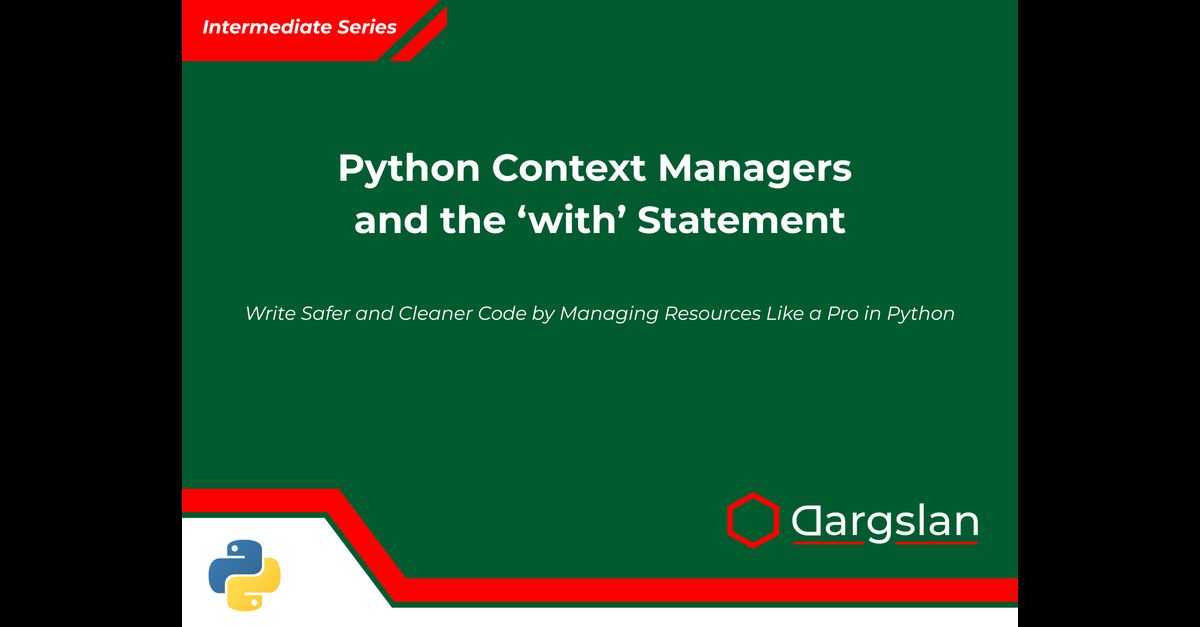
Python Context Managers and the ‘with’ Statement