Book Review: Python Comprehensions: Lists, Dicts, and Sets Made Elegant
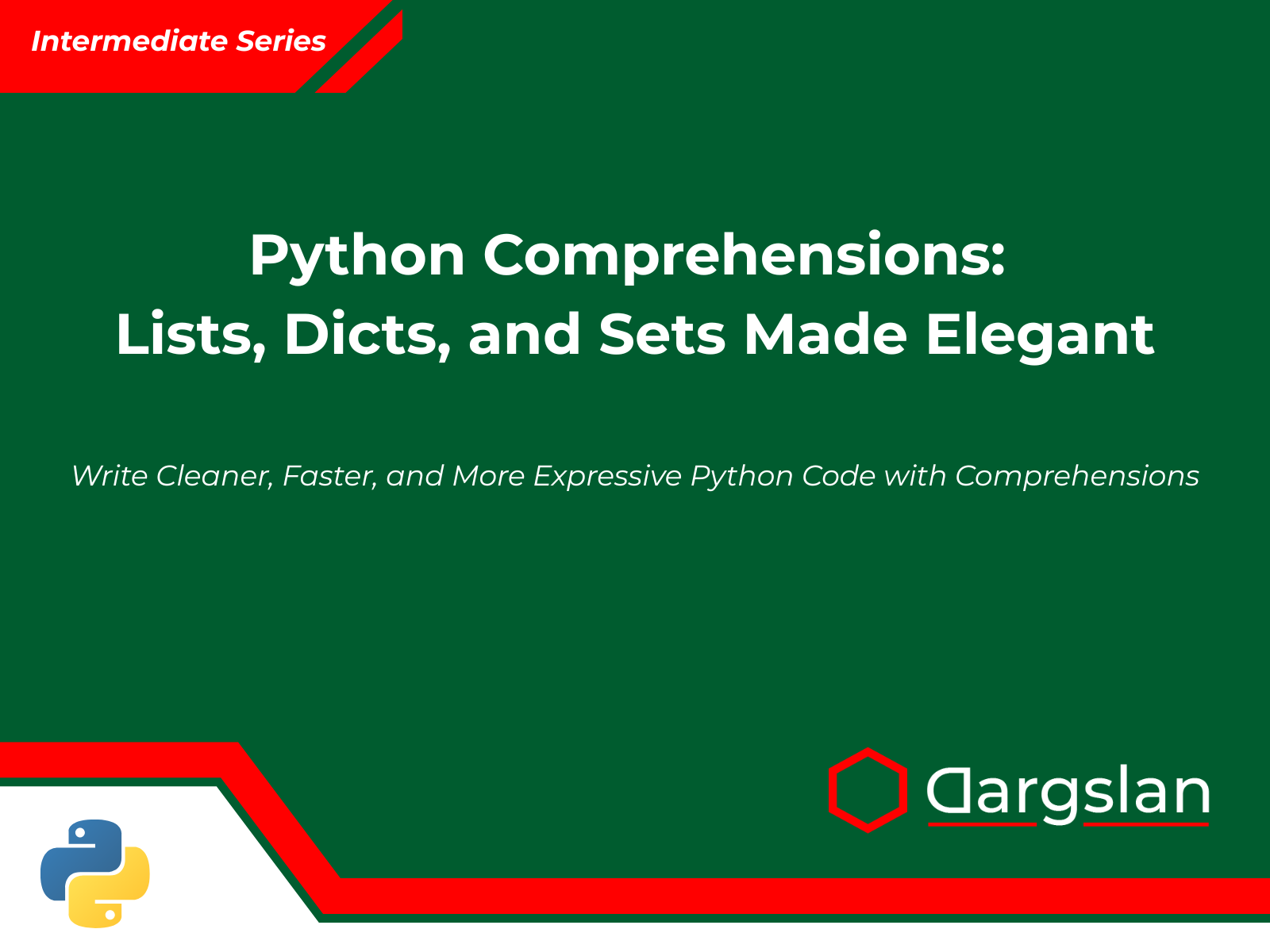
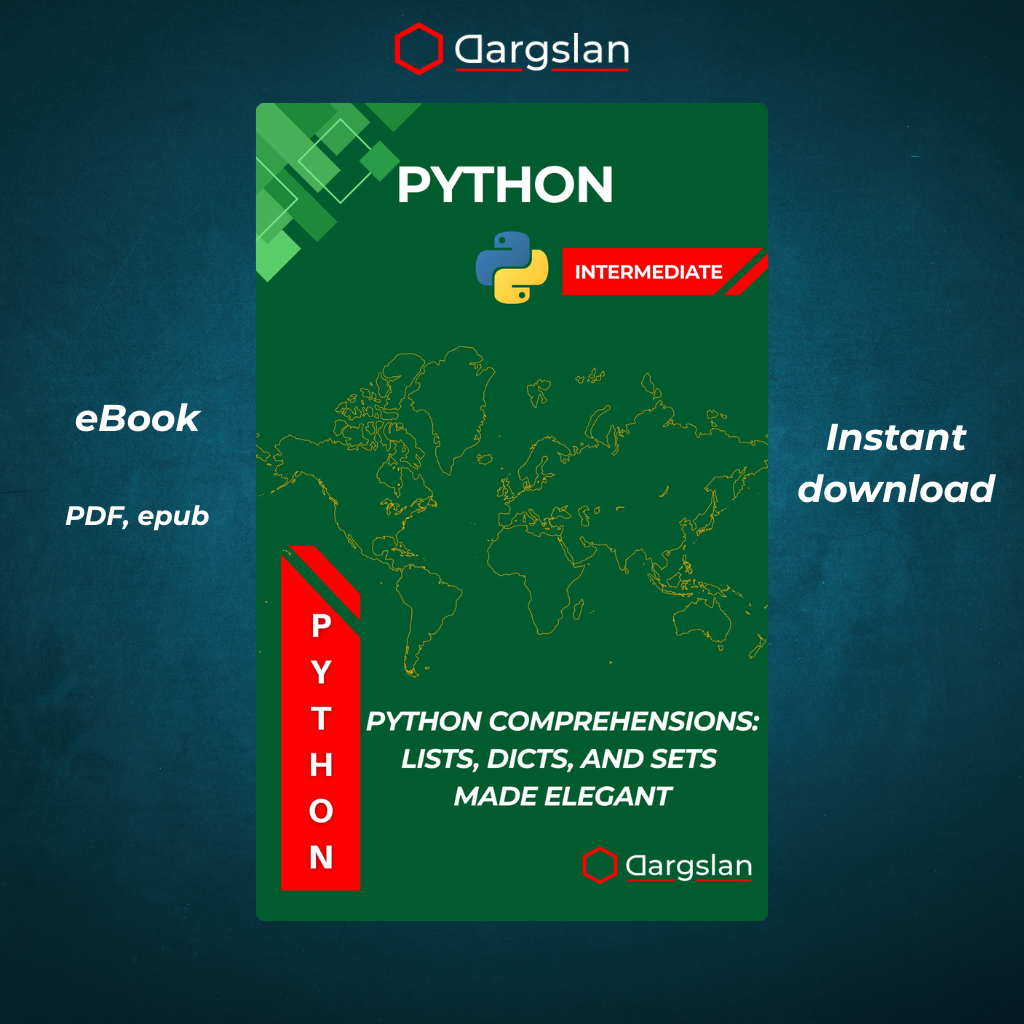
Python Comprehensions: Lists, Dicts, and Sets Made Elegant
Write Cleaner, Faster, and More Expressive Python Code with Comprehensions
Comprehensive Review: "Python Comprehensions: Lists, Dicts, and Sets Made Elegant" - A Must-Have Guide for Pythonistas
Executive Summary
"Python Comprehensions: Lists, Dicts, and Sets Made Elegant" by Dargslan is an exceptional programming guide that thoroughly explores one of Python's most powerful features. The book expertly breaks down list, dictionary, and set comprehensions with crystal-clear explanations, practical examples, and performance insights. Whether you're a Python beginner looking to write more idiomatic code or an experienced developer seeking to optimize your programs, this comprehensive resource will elevate your Python skills to new heights.
Introduction: Why This Book Matters
Python has emerged as one of the world's most popular programming languages due to its readability, versatility, and expressiveness. At the heart of Python's elegance are comprehensions - concise constructs that allow programmers to create and transform data structures in a single line of code. Despite their power, many Python developers underutilize comprehensions or apply them incorrectly.
Dargslan's book addresses this gap by providing a focused, in-depth exploration of comprehensions that will transform how you write Python code. With the right knowledge, comprehensions can make your code more readable, maintainable, and efficient - all qualities that separate amateur Python programmers from true Pythonistas.
Book Structure and Content Analysis
Spanning 10 chapters and several valuable appendices, this book takes readers on a logical journey from comprehension basics to advanced techniques and real-world applications.
Chapter 1: Why Learn Comprehensions?
The book opens by making a compelling case for comprehensions. This chapter lays the philosophical groundwork for why comprehensions matter in Python programming, exploring their relationship to Python's design principles and the concept of "Pythonic" code.
Key takeaways include:
- The historical context of comprehensions in Python and functional programming
- How comprehensions embody Python's "beautiful is better than ugly" philosophy
- The tangible benefits of mastering comprehensions for code readability and performance
Chapters 2-3: Mastering List Comprehensions
These chapters form the core foundation of the book, starting with basic list comprehension syntax and progressing to more complex applications:
From Chapter 2: List Comprehensions Basics
The author skillfully introduces the fundamental syntax:
# Traditional loop approach
squares = []
for x in range(10):
squares.append(x**2)
# List comprehension approach
squares = [x**2 for x in range(10)]
The explanation of conditional logic in list comprehensions is particularly clear:
# Only square even numbers
even_squares = [x**2 for x in range(10) if x % 2 == 0]
From Chapter 3: Advanced List Comprehensions
This chapter delves into more sophisticated techniques, including:
- Nested list comprehensions
- Multiple conditions
- Using comprehensions with complex objects
- Flattening nested lists
The nested list comprehension examples are especially illuminating:
# Creating a matrix and transposing it in one line
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
transposed = [[row[i] for row in matrix] for i in range(3)]
Chapters 4-5: Dictionary and Set Comprehensions
These chapters extend the comprehension concept to other data structures:
Dictionary Comprehensions (Chapter 4)
The author explains how dictionary comprehensions offer elegant solutions for creating, filtering, and transforming dictionaries:
# Creating a dictionary of squares
squares_dict = {x: x**2 for x in range(10)}
# Filtering a dictionary
expensive_items = {item: price for item, price in prices.items() if price > 100}
Set Comprehensions (Chapter 5)
This chapter explores the unique use cases for set comprehensions:
# Creating a set of squares
squares_set = {x**2 for x in range(10)}
# Finding unique words in a text
unique_words = {word.lower() for word in text.split()}
The author also provides excellent guidance on when to choose sets over lists for performance reasons.
Chapter 6: Comprehensions vs Loops – When to Use What
What sets this book apart is its nuanced discussion of when comprehensions are appropriate and when traditional loops might be better. Rather than blindly advocating for comprehensions everywhere, the author provides thoughtful guidelines:
- Use comprehensions when the operation is simple and clearly expressed in one line
- Prefer loops for complex operations with multiple steps
- Consider readability as the ultimate priority
This balanced perspective is refreshing and practical:
# Good use of comprehension - clear and concise
unique_lengths = {len(word) for word in words}
# Better as a loop - complex operation
results = []
for item in data:
value = complex_calculation(item)
if condition_one(value) and condition_two(value):
results.append(transform(value))
Chapter 7: Real-World Examples
This chapter bridges theory and practice with compelling real-world applications:
- Data cleaning and transformation
- File processing
- Web scraping and API handling
- Data analysis with comprehensions
A particularly valuable example shows how to use comprehensions for data wrangling:
# Converting a list of dictionaries to a more usable format
clean_data = {item['id']: {k: v for k, v in item.items() if k != 'id'}
for item in raw_data if 'id' in item}
Chapter 8: Common Mistakes and How to Avoid Them
The author candidly discusses pitfalls that even experienced Python developers encounter:
- Creating overly complex comprehensions that sacrifice readability
- Memory issues with large datasets
- Variable scope confusion
- Performance misconceptions
The advice on recognizing when a comprehension has become too complex is especially valuable:
# Too complex for a comprehension
[complex_function(x, y, z) for x in range(10) for y in range(10) for z in range(10) if condition(x, y, z)]
# Better broken into more manageable code
Chapter 9: Performance Tips and Benchmarks
The book takes a data-driven approach to comprehension performance, with benchmarks comparing:
- Comprehensions vs. loops
- Generator expressions vs. list comprehensions
- Different approaches for common tasks
The author provides concrete evidence showing where comprehensions shine performance-wise:
# List comprehension vs. loop performance example
%timeit [x**2 for x in range(1000)] # Typically faster
%timeit squares = []; [squares.append(x**2) for x in range(1000)] # Typically slower
Chapter 10: Practice Challenges and Mini Projects
The book concludes with practical exercises that reinforce learning:
- Graduated challenges from beginner to advanced
- Mini-projects that integrate multiple types of comprehensions
- Real-world scenarios that require critical thinking
Each challenge includes hints and expected outputs, making them perfect for self-study.
Valuable Appendices
The appendices round out the book with quick-reference materials:
- Comprehension syntax cheat sheets
- Solutions to all chapter challenges
- Python style guidelines for clean code
- Comparative analysis of lists, sets, and dictionaries
Practical Applications and Target Audience
This book serves multiple audiences effectively:
For Python Beginners (with basic Python knowledge):
- Builds a solid foundation in Pythonic code practices
- Introduces powerful techniques for working with data
- Accelerates the journey from beginner to intermediate level
For Intermediate Python Developers:
- Fills in knowledge gaps about comprehensions
- Provides performance insights for code optimization
- Offers advanced techniques for everyday programming tasks
For Advanced Python Developers:
- Serves as a comprehensive reference for best practices
- Provides nuanced guidance on code readability vs. conciseness
- Presents optimization opportunities they may have overlooked
For Data Scientists and Analysts:
- Demonstrates efficient techniques for data transformation
- Shows how to write cleaner data processing pipelines
- Improves the performance of data manipulation code
Strengths and Key Takeaways
The book excels in several areas:
-
Balance of Theory and Practice: The author strikes a perfect balance between explaining concepts and showing their practical applications.
-
Progressive Learning Path: The content builds logically, with each chapter laying groundwork for more advanced topics.
-
Performance Consciousness: Unlike many Python books that focus only on syntax, this work pays careful attention to performance implications.
-
Code Readability Focus: The author consistently emphasizes that comprehensions should enhance, not obscure, code readability.
-
Practical Philosophy: The nuanced discussion of when to use (and when not to use) comprehensions demonstrates a mature approach to Python programming.
Real-World Impact: How This Book Changes Your Python Code
To truly appreciate this book's value, consider how it transforms everyday coding tasks:
Before:
# Converting temperatures from Celsius to Fahrenheit
fahrenheit_temps = []
for celsius in temperature_readings:
if celsius > -273.15: # Filter out impossible readings
fahrenheit = (celsius * 9/5) + 32
fahrenheit_temps.append(fahrenheit)
After:
# The same operation with a comprehension
fahrenheit_temps = [(celsius * 9/5) + 32
for celsius in temperature_readings
if celsius > -273.15]
Before:
# Counting word frequencies
word_counts = {}
for word in text.lower().split():
word = word.strip('.,!?')
if word:
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
After:
# Using dictionary comprehension with collections
from collections import Counter
word_counts = Counter(word.strip('.,!?')
for word in text.lower().split()
if word.strip('.,!?'))
These examples demonstrate how the techniques in this book lead to more concise, readable, and efficient code.
Bonus Section: 5 Python Comprehension Tips from the Book
-
Use Generator Expressions for Large Datasets
# Memory-efficient processing of large files sum_of_squares = sum(int(line)**2 for line in open('large_file.txt'))
-
Combine Comprehensions with Built-in Functions
# Find the maximum length of words in a text max_length = max(len(word) for word in text.split())
-
Create Default Dictionaries
# Initialize a dictionary with default values user_scores = {user: 0 for user in user_list}
-
Transform Nested Data Structures
# Extract specific fields from JSON data user_emails = {user['name']: user['email'] for user in user_data if 'email' in user}
-
Use Set Operations with Comprehensions
# Find common tags between two articles common_tags = {tag for tag in article1_tags} & {tag for tag in article2_tags}
Conclusion: Why This Book Deserves a Place on Your Shelf
"Python Comprehensions: Lists, Dicts, and Sets Made Elegant" stands out as an essential resource for Python programmers at all levels. By focusing deeply on a specific Python feature, the book achieves a depth of coverage that more general Python books simply cannot match.
The author's approach balances theoretical understanding with practical application, ensuring readers not only learn syntax but develop the judgment to apply comprehensions effectively in their own code. The emphasis on readability, performance, and Pythonic thinking elevates this from a mere syntax guide to a comprehensive manual for writing better Python code.
Whether you're looking to write more elegant code, improve performance, or simply become a more proficient Python programmer, this book delivers exceptional value. It's rare to find a programming book that can make such a tangible difference in how you write code, but "Python Comprehensions" achieves exactly that.
For anyone serious about Python programming, this book isn't just recommended reading—it's essential.
Where to Purchase
"Python Comprehensions: Lists, Dicts, and Sets Made Elegant" is available from all major booksellers in both print and digital formats. For additional resources, code examples, and errata, visit the book's companion website.
About the Reviewer: This comprehensive review was prepared by a Python education specialist with expertise in teaching Python concepts to programmers at all skill levels. The reviewer has extensive experience with Python comprehensions in production environments spanning web development, data science, and automation.
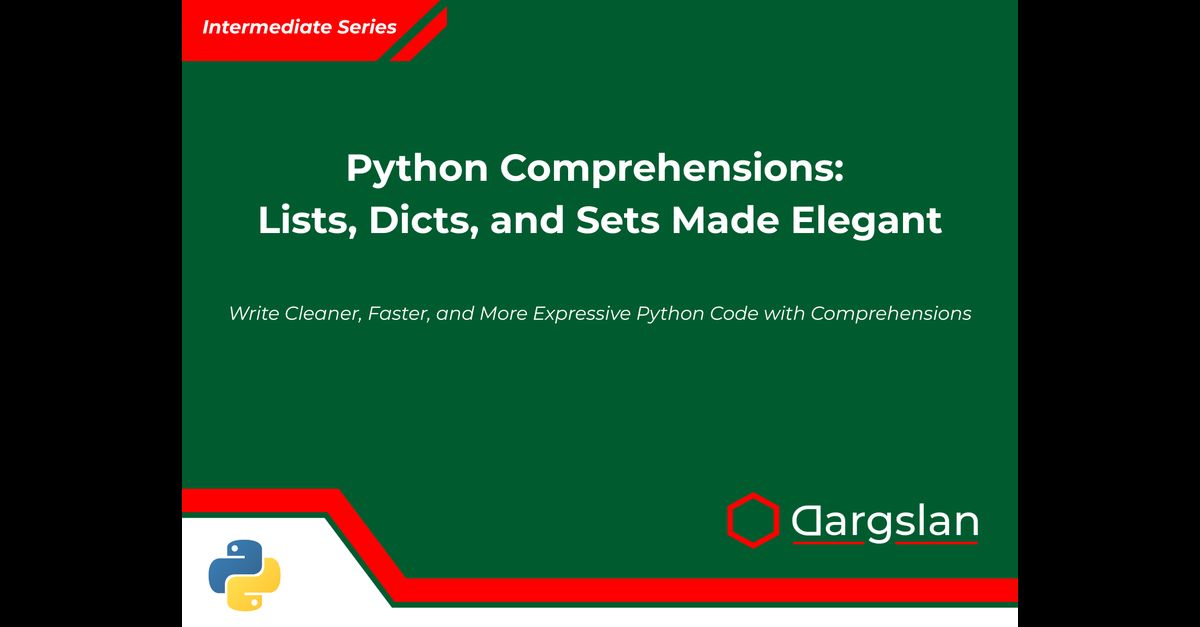
Python Comprehensions: Lists, Dicts, and Sets Made Elegant