Book Review: Object-Oriented Python for Beginners: A Comprehensive Guide
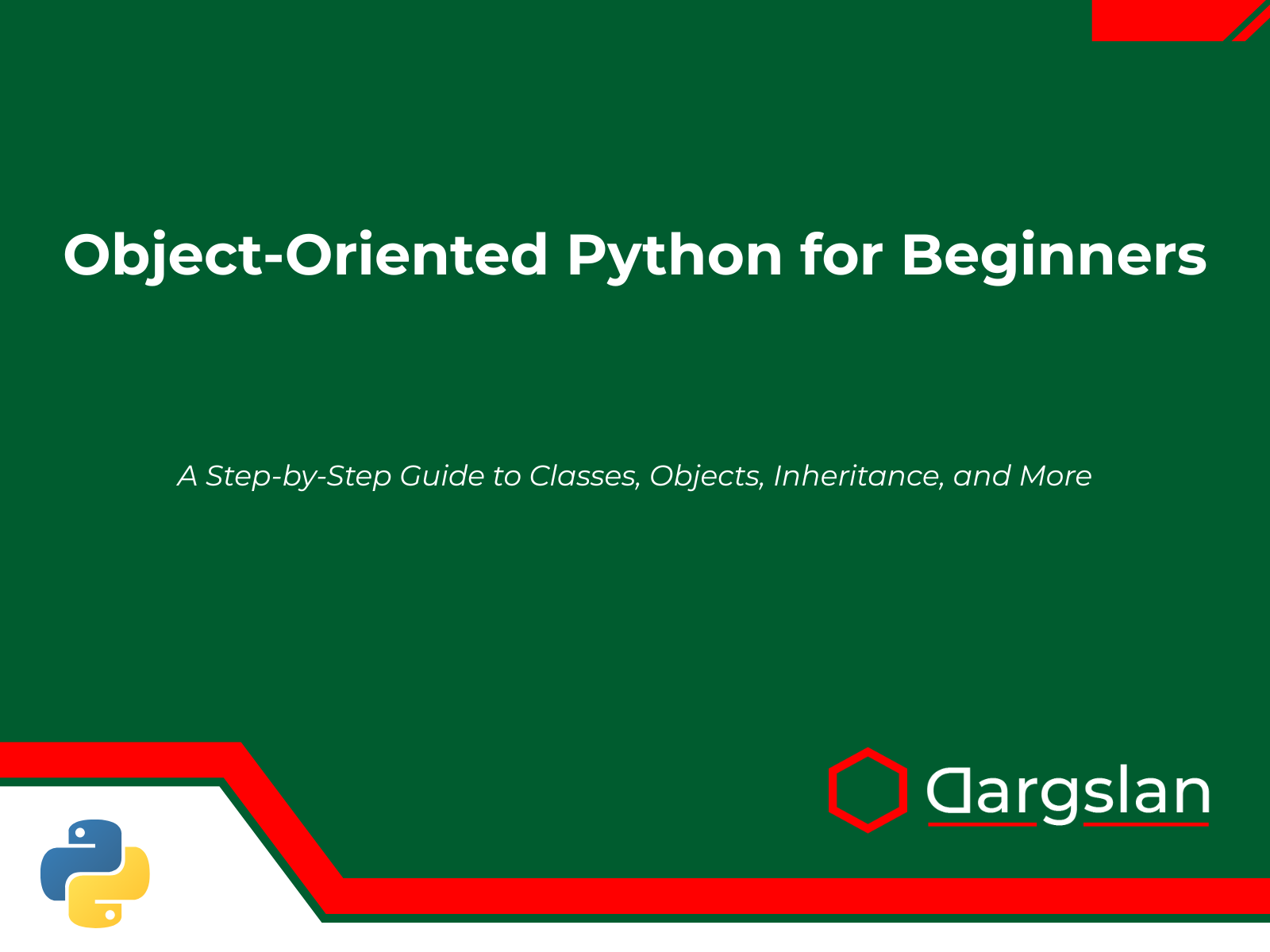
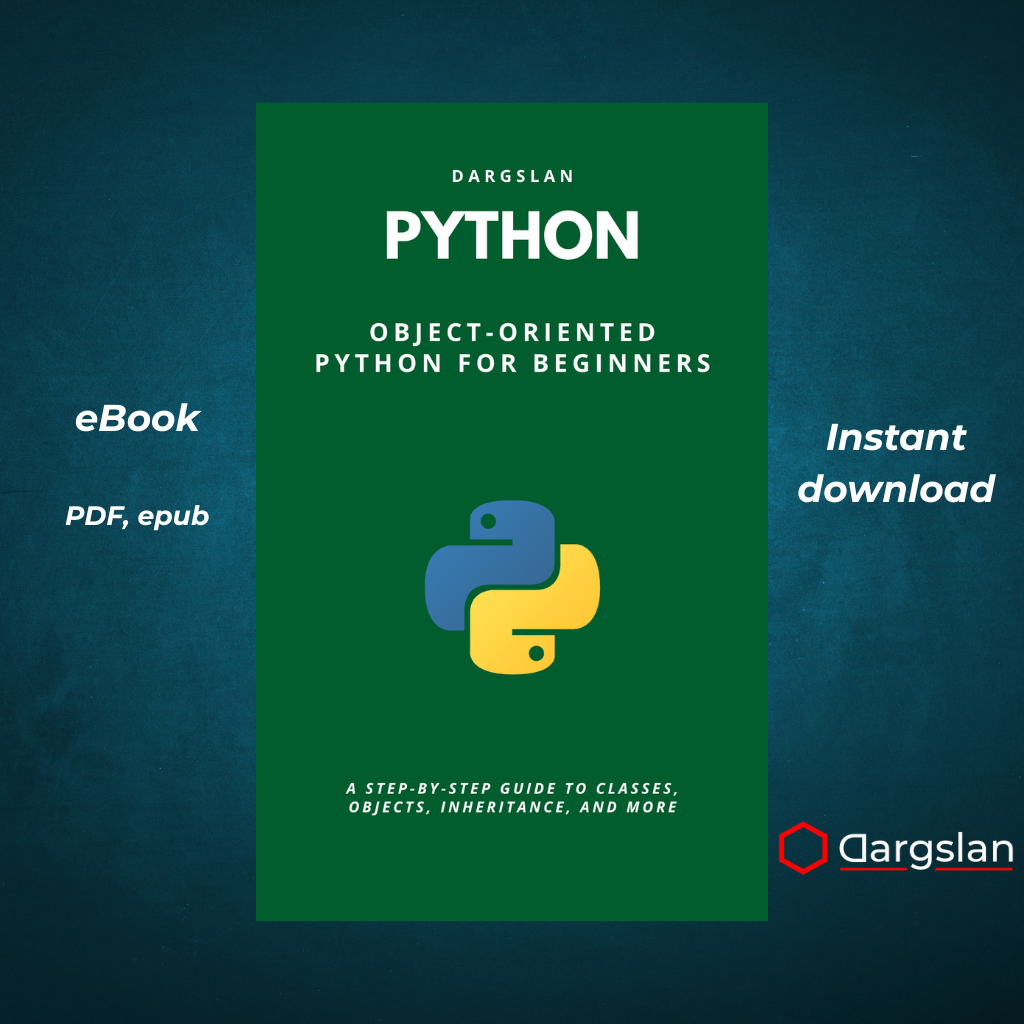
Object-Oriented Python for Beginners
A Step-by-Step Guide to Classes, Objects, Inheritance, and More
Welcome to the World of Object-Oriented Programming in Python
Are you ready to transform your Python coding skills and unlock powerful techniques used by professionals worldwide? "Object-Oriented Python for Beginners" is your complete roadmap to mastering one of programming's most important paradigms using Python's elegant implementation.
This comprehensive guide takes you from foundational concepts to advanced techniques, providing clear explanations, practical examples, and hands-on projects at every step. Whether you're a complete newcomer to programming or looking to strengthen your existing Python knowledge, this book will elevate your skills to new heights.
Why Object-Oriented Programming Matters
Object-oriented programming (OOP) has become the dominant paradigm in software development for good reason. By organizing code around objects that combine data and functionality, OOP helps you:
- Write more maintainable code that's easier to update and expand
- Reduce duplication through powerful inheritance mechanisms
- Model real-world systems with intuitive, logical structures
- Create reusable components that save development time
- Build scalable applications from small scripts to enterprise systems
Python's implementation of OOP is particularly accessible, making it the perfect language for learning these critical concepts.
What You'll Learn
This comprehensive guide covers everything you need to know about object-oriented Python:
Core OOP Concepts
- Classes and Objects: Learn to create blueprints for your data and instantiate them as working objects
- Attributes and Methods: Understand how to store data and define behaviors within your classes
- Encapsulation: Master techniques for controlling access to data and hiding implementation details
- Inheritance: Discover how to build hierarchies of classes that share and extend functionality
- Polymorphism: Explore how different objects can respond uniquely to the same methods
Python-Specific OOP Features
- Special Methods: Harness Python's "magic methods" to integrate your objects with built-in operations
- Property Decorators: Create clean, controlled interfaces to your object's internal data
- Multiple Inheritance: Navigate Python's approach to inheriting from multiple parent classes
- Static and Class Methods: Understand when and how to use methods that work at the class level
- Abstract Base Classes: Design robust interfaces and class hierarchies
Advanced Techniques
- Duck Typing: Embrace Python's dynamic approach to object interfaces
- Composition vs. Inheritance: Learn when to use each approach for maximum flexibility
- Exception Handling: Design custom exception hierarchies for clean error management
- Context Managers: Implement the powerful
with
statement for your own classes - Operator Overloading: Define how your objects interact with Python's operators
Chapter-by-Chapter Breakdown
Chapter 1: Introduction to Object-Oriented Programming
Begin your journey by understanding why OOP exists and how it compares to other programming paradigms. You'll get a high-level overview of key concepts and see how Python's implementation makes OOP both powerful and accessible.
Chapter 2: Python Basics Refresher
Ensure you have a solid foundation with this quick review of essential Python concepts. We'll cover variables, data types, functions, and control structures—all the building blocks you'll need for OOP success.
Chapter 3: Defining and Using Classes
Dive into the core of OOP by learning to create and use your own classes. You'll write your first custom objects, understand instance creation, and see how methods and attributes work together.
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return f"{self.name} says Woof!"
# Creating and using an object
my_dog = Dog("Rex", "German Shepherd")
print(my_dog.bark()) # Output: Rex says Woof!
Chapter 4: Understanding self and Object Scope
Master one of Python's distinctive OOP features—the explicit self
parameter. You'll learn how Python manages instance references, variable scope within methods, and how objects maintain their state.
Chapter 5: Class Attributes and Static Methods
Move beyond instance-level programming to understand class-level attributes and methods. You'll learn when to use static methods, class methods, and how they differ from regular instance methods.
Chapter 6: Encapsulation and Data Hiding
Explore Python's approach to encapsulation—controlling access to an object's internal data. You'll learn about naming conventions, property decorators, and creating clean interfaces for your classes.
Chapter 7: Inheritance and Code Reuse
Unlock the power of inheritance to build hierarchies of related classes. You'll see how child classes can inherit, override, and extend parent functionality, promoting code reuse and logical organization.
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass # To be implemented by subclasses
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
Chapter 8: Polymorphism and Duck Typing
Understand how different objects can respond to the same method calls in their own ways. You'll explore Python's dynamic "duck typing" approach and see how it promotes flexible, adaptable code.
Chapter 9: Composition vs Inheritance
Dive into a fundamental design decision in OOP—when to use inheritance versus when to compose objects from other objects. You'll learn the principle "favor composition over inheritance" and when each approach shines.
Chapter 10: Special Methods and Operator Overloading
Discover Python's powerful special methods that allow your classes to integrate seamlessly with built-in operations. You'll implement custom behaviors for operations like addition, comparison, and string representation.
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Point(self.x + other.x, self.y + other.y)
def __str__(self):
return f"Point({self.x}, {self.y})"
p1 = Point(1, 2)
p2 = Point(3, 4)
p3 = p1 + p2 # Uses __add__ method
print(p3) # Uses __str__ method - Output: Point(4, 6)
Chapter 11: Abstract Classes and Interfaces
Learn to define class interfaces that enforce implementation in subclasses. You'll work with abstract base classes, understand interface design, and see how Python's approach differs from other languages.
Chapter 12: Exception Handling with Classes
Build robust error-handling mechanisms using custom exception classes. You'll design exception hierarchies, learn best practices for raising and catching exceptions, and integrate error handling into your object-oriented designs.
Chapter 13: A Mini Project
Apply everything you've learned in a comprehensive project—building a library management system. This hands-on chapter guides you through designing and implementing a complete object-oriented application from scratch.
Practical Learning with Real Examples
Throughout the book, you'll find numerous code examples that demonstrate concepts in action. Each example is carefully crafted to be:
- Clear and concise: Easy to understand even for beginners
- Practical and relevant: Showing realistic use cases, not contrived examples
- Progressive: Building on previous concepts to reinforce your learning
- Complete and runnable: Ready for you to try on your own computer
You'll build a variety of mini-applications that showcase OOP principles, including:
- A banking system with accounts, transactions, and interest calculations
- A shape hierarchy for calculating geometric properties
- A customizable deck of cards with shuffling and dealing capabilities
- A student grading system with multiple report formats
- And finally, a complete library management system in Chapter 13
Perfect for Self-Paced Learning
This book is designed for maximum learning effectiveness, whether you're:
- Working through it chapter by chapter in sequence
- Using it as a reference to understand specific OOP concepts
- Following along with the examples on your computer
- Adapting the techniques to your own projects
Each chapter includes:
- Clear learning objectives so you know what you'll accomplish
- Concept explanations with straightforward language and helpful analogies
- Practical code examples demonstrating each idea in action
- Common pitfalls and how to avoid them
- Review questions to test your understanding
- Challenge exercises to build your skills through practice
Essential References for Continued Learning
The book concludes with three valuable appendices:
Appendix A: Python OOP Keywords Cheat Sheet
A quick reference to all the keywords, decorators, and special methods related to Python's OOP implementation.
Appendix B: UML Basics for Python Developers
Learn to diagram your class structures using industry-standard Unified Modeling Language, adapted specifically for Python.
Appendix C: Further Learning Resources
Curated recommendations for books, courses, websites, and projects to continue your OOP journey.
Who This Book Is For
This guide is perfect for:
- Python beginners who have grasped basic syntax and want to level up
- Self-taught programmers looking to fill gaps in their OOP knowledge
- Students supplementing computer science coursework
- Developers from other languages learning Python's approach to OOP
- Professional developers wanting a refresher on OOP principles
Start Your Object-Oriented Python Journey Today
Object-oriented programming isn't just a technical skill—it's a powerful way of thinking about and structuring code that will transform your approach to software development. With "Object-Oriented Python for Beginners," you'll gain:
- Confidence in designing and implementing complex systems
- Efficiency in writing clean, reusable code
- Understanding of professional-grade software architecture
- Skills that are highly valued in the software industry
Don't just write scripts—design robust, scalable, maintainable software systems using the power of object-oriented Python. This comprehensive guide gives you everything you need to master OOP principles and apply them to real-world programming challenges.
Ready to elevate your Python skills? Turn the page and begin your journey into the world of object-oriented programming!
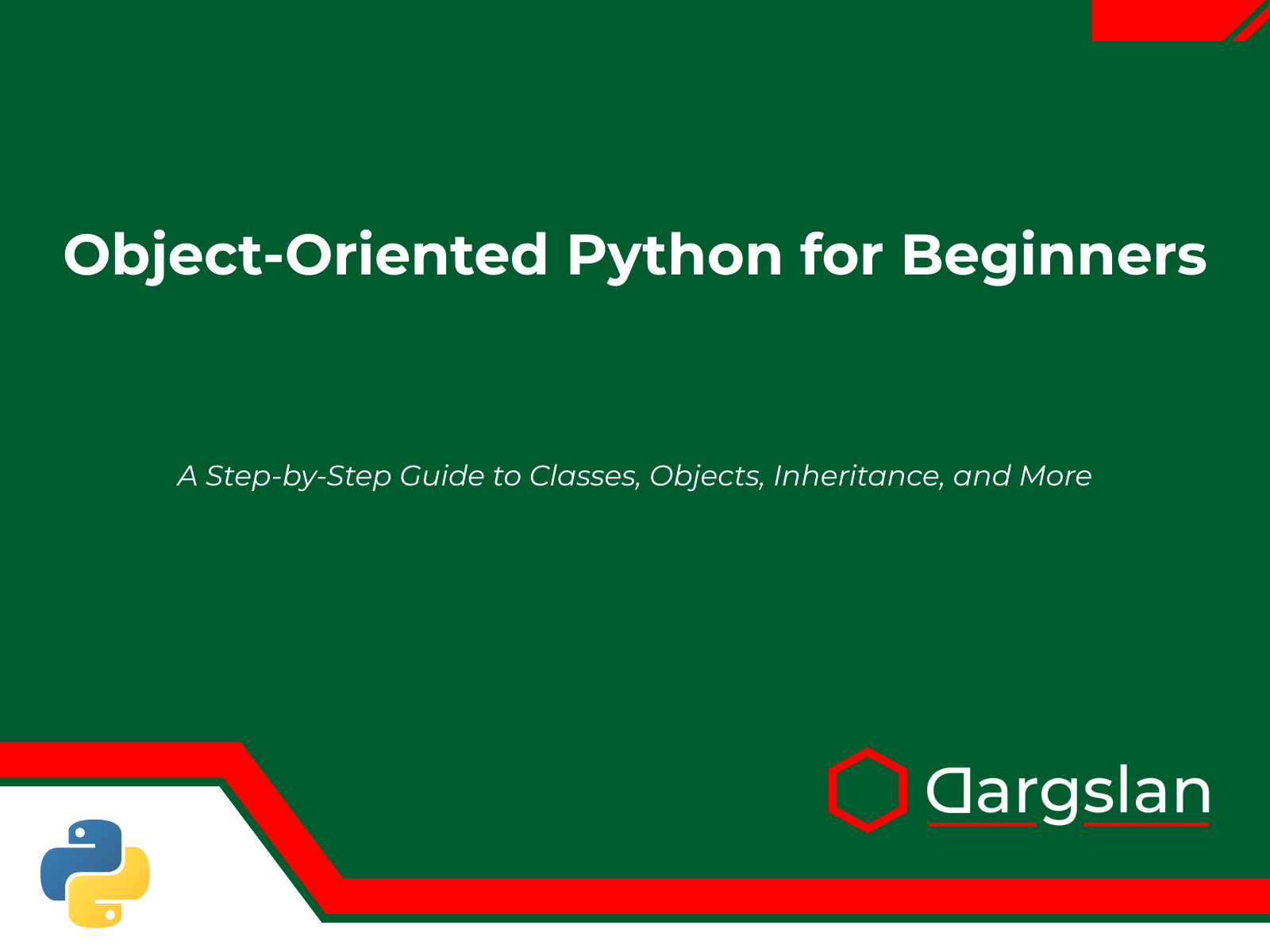
Object-Oriented Python for Beginners
A Step-by-Step Guide to Classes, Objects, Inheritance, and More