Book Review: Object-Oriented Python: Classes and Inheritance Explained
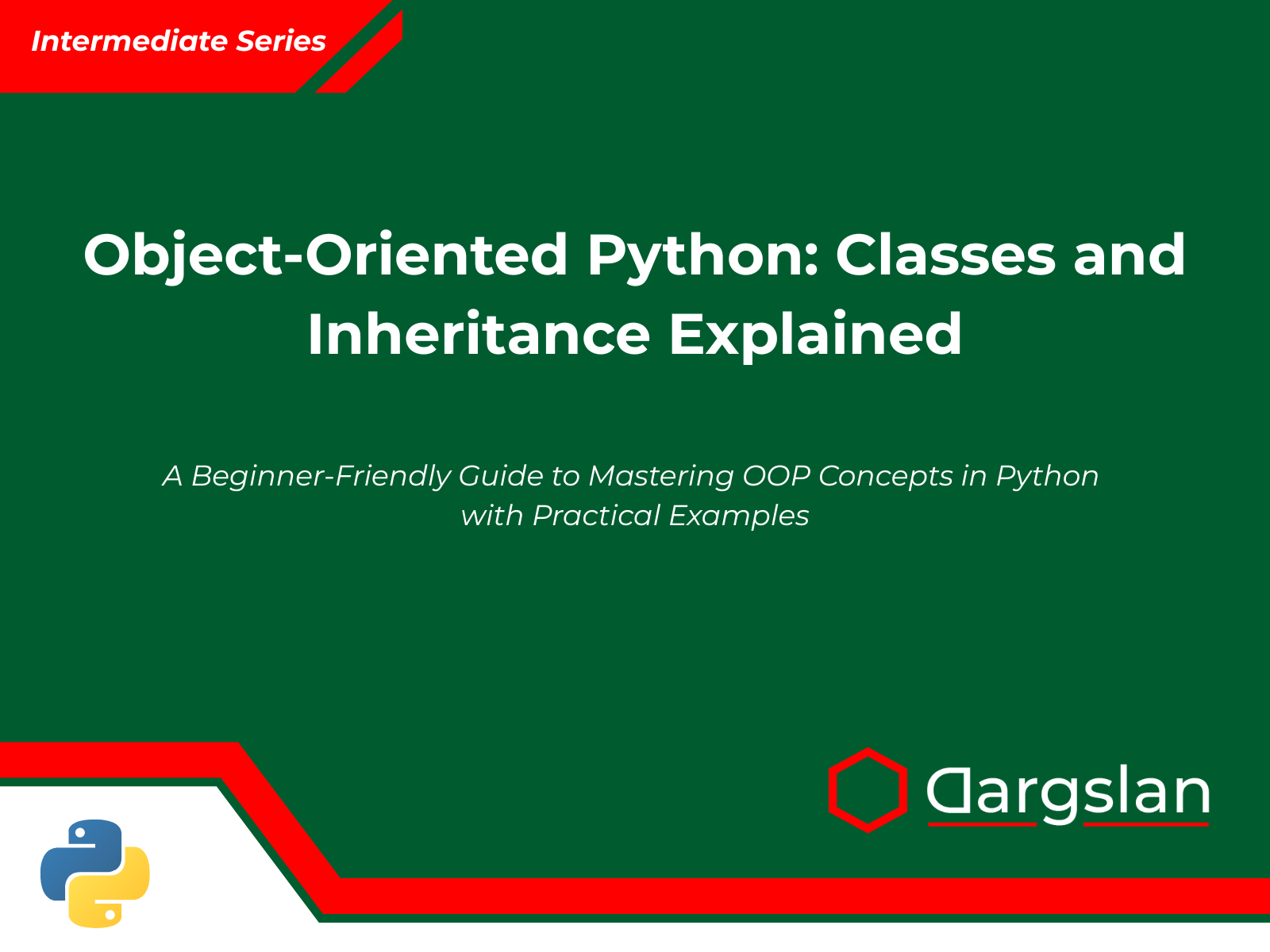
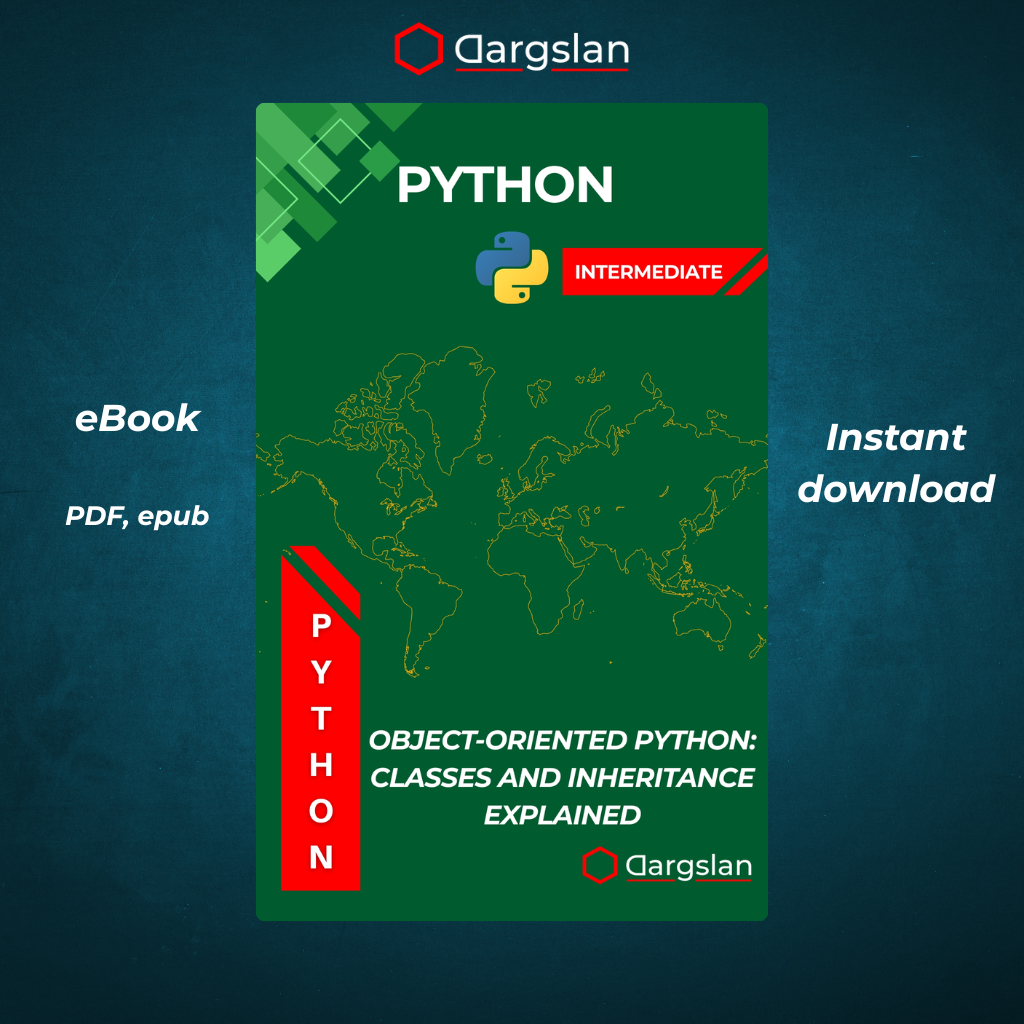
Object-Oriented Python: Classes and Inheritance Explained
A Beginner-Friendly Guide to Mastering OOP Concepts in Python with Practical Examples
"Object-Oriented Python: Classes and Inheritance Explained" - A Comprehensive Review
Introduction: The Definitive Guide to Python OOP
In the ever-evolving landscape of programming languages, Python stands tall as one of the most accessible yet powerful tools in a developer's arsenal. Among Python's many strengths is its elegant implementation of Object-Oriented Programming (OOP) principles – a paradigm that continues to shape modern software development. "Object-Oriented Python: Classes and Inheritance Explained" by Dargslan emerges as a timely and invaluable resource for programmers seeking to master these essential concepts.
This meticulously crafted guide promises to bridge the gap between basic Python knowledge and professional-grade object-oriented design skills. Unlike many programming books that either overwhelm readers with theory or provide overly simplistic examples, Dargslan's work strikes a remarkable balance between accessibility and depth, making it suitable for both newcomers to OOP and experienced developers looking to refine their Python expertise.
Why This Book Matters in 2023
As software systems grow increasingly complex, the ability to organize code through object-oriented design has become not just beneficial but essential. Python's implementation of OOP offers a more flexible and intuitive approach compared to stricter languages like Java or C++, yet mastering Python OOP requires specialized guidance that goes beyond general Python tutorials.
This book arrives at a time when Python continues to dominate fields ranging from web development and data science to AI and automation. Professionals in these domains increasingly need strong OOP skills to build scalable, maintainable systems. Whether you're creating sophisticated data analysis pipelines, developing web applications, or building machine learning models, understanding how to effectively structure your Python code using OOP principles will dramatically enhance your capabilities.
Content Overview: A Journey Through Python's OOP Landscape
"Object-Oriented Python: Classes and Inheritance Explained" features ten comprehensive chapters that progressively build your knowledge, beginning with fundamental concepts and culminating in advanced techniques and real-world projects.
The journey starts with an introduction to OOP philosophy before quickly transitioning to hands-on experience creating your first Python classes. As you progress, you'll explore increasingly sophisticated topics including attribute management, encapsulation, inheritance hierarchies, method overriding, and Python's powerful special methods system.
What sets this book apart is its unwavering focus on Python-specific implementations of OOP, rather than generic OOP theory. Each concept is presented in the context of Python's unique approach, with particular attention paid to Python's special features like dunder methods, properties, descriptors, and metaclasses.
The appendices provide additional value through quick reference guides, troubleshooting help for common OOP errors, and practice problems with detailed solutions – transforming this book from merely instructional to a complete learning system.
Chapter-by-Chapter Breakdown: What You'll Learn
Chapter 1: What Is Object-Oriented Programming (OOP)?
The book opens by establishing a solid foundation in OOP philosophy. Rather than diving immediately into syntax, Dargslan takes the time to explain why OOP exists and how it solves real problems in software development. This chapter covers:
- The evolution from procedural to object-oriented programming
- Core OOP concepts: encapsulation, inheritance, polymorphism, and abstraction
- Python's unique approach to OOP compared to other languages
- How thinking in objects transforms the way you design software
- Real-world analogies that make abstract OOP concepts concrete and intuitive
This thoughtful introduction ensures that readers understand not just how to implement OOP in Python, but why they would want to in the first place – setting the stage for practical application rather than rote learning.
Chapter 2: Creating Your First Class
With philosophical groundwork established, Chapter 2 guides readers through building their first Python classes. Highlights include:
- The anatomy of a Python class: structure, syntax, and naming conventions
- Constructing objects with
__init__
and understanding theself
parameter - Instance creation and object lifecycles
- Implementing simple methods and attributes
- Best practices for class design and documentation
Through a series of progressively more complex examples, readers gain hands-on experience defining classes that model real-world entities. The chapter strikes an excellent balance between theory and practice, ensuring concepts are immediately reinforced through code.
Chapter 3: Working with Attributes and Methods
Chapter 3 delves deeper into the mechanics of Python classes, exploring how attributes and methods bring objects to life:
- Different types of attributes: instance, class, and dynamic attributes
- Method implementation and the role of
self
- Property decorators for controlled attribute access
- Using descriptors for advanced attribute behavior
- Method types: instance methods, class methods, and static methods
The examples in this chapter are particularly strong, illustrating how methods and attributes work together to create cohesive objects that encapsulate both data and behavior.
Chapter 4: Encapsulation and Access Control
This pivotal chapter tackles one of OOP's core principles – encapsulation – with special attention to Python's unique approach:
- Python's philosophy of "we're all consenting adults here"
- Name mangling with double underscores (
__private_attribute
) - Creating public interfaces with properties and getter/setter methods
- Protected attributes convention with single underscores
- Balancing encapsulation with Python's inherent flexibility
Dargslan doesn't simply regurgitate OOP dogma here but thoughtfully examines how traditional encapsulation principles apply in Python's more fluid environment, providing practical guidelines that align with Pythonic conventions.
Chapter 5: Inheritance in Python
Chapter 5 introduces inheritance, perhaps OOP's most powerful feature:
- Single inheritance: extending base classes in Python
- Method resolution order and the
super()
function - Abstract base classes and interfaces in Python
- Multiple inheritance and the diamond problem
- Composition vs. inheritance: knowing which to choose
The examples progressively introduce more sophisticated inheritance patterns, illustrating both the power and potential pitfalls of inheritance-based designs.
Chapter 6: Method Overriding and Customization
Building on the inheritance foundation, Chapter 6 explores how to customize inherited behavior:
- Overriding methods from parent classes
- Extending parent behavior with
super()
- Method overriding vs. method overloading
- Creating polymorphic interfaces
- Design patterns facilitated by method overriding
This chapter effectively demonstrates how inheritance becomes truly useful through customization, with examples that show how the same interface can be implemented differently across a family of related classes.
Chapter 7: Using Class and Static Methods
Chapter 7 focuses on Python's class-level functionality:
- Class methods and the
@classmethod
decorator - Static methods and the
@staticmethod
decorator - Alternative constructors using class methods
- Factory patterns and their implementation
- When and why to use class methods vs. static methods
The practical examples in this chapter show how these special method types expand Python's OOP toolkit, enabling designs that would be awkward or impossible with instance methods alone.
Chapter 8: Special Methods and Operator Overloading
Chapter 8 explores one of Python's most distinctive OOP features – the dunder (double underscore) methods that enable operator overloading:
- Object representation with
__str__
and__repr__
- Comparison operators (
__eq__
,__lt__
, etc.) - Mathematical operations (
__add__
,__mul__
, etc.) - Container behavior (
__getitem__
,__len__
, etc.) - Object lifecycle methods (
__new__
,__init__
,__del__
)
The examples brilliantly demonstrate how these special methods allow your custom objects to behave like built-in Python types, leading to more intuitive and Pythonic code.
Chapter 9: Inheritance Best Practices and Pitfalls
In this crucial chapter, Dargslan addresses the wisdom gained from years of Python OOP experience:
- Common inheritance pitfalls and how to avoid them
- The Liskov Substitution Principle in Python
- Multiple inheritance strategies and mixin classes
- When to use composition instead of inheritance
- Testing and debugging inheritance hierarchies
The practical advice in this chapter helps readers navigate the sometimes confusing world of inheritance design decisions, with clear guidelines for creating maintainable and robust class hierarchies.
Chapter 10: Final OOP Projects
The book culminates in chapter 10 with comprehensive projects that integrate all the concepts covered:
- Building a library management system
- Developing a simple game with OOP principles
- Creating a data analysis framework
- Implementing a plugin system using inheritance
- Designing a custom exception hierarchy
These projects demonstrate OOP in action, showing how the individual concepts combine to create sophisticated, maintainable applications.
The Appendices: References and Resources
The book's value extends beyond its main chapters with four practical appendices:
- Quick Reference: A concise summary of Python OOP syntax and patterns
- Common OOP Errors and Fixes: Troubleshooting guide for typical pitfalls
- Exercises and Practice Problems: Additional hands-on opportunities with solutions
- Next Steps: Resources for continuing education in advanced Python and design patterns
These appendices transform the book from a one-time read into an ongoing reference tool that will remain useful long after the initial reading.
Teaching Approach: Pedagogy That Works
Dargslan's teaching approach deserves special mention. Rather than overwhelming readers with abstract theory or skimming the surface with trivial examples, the book employs a carefully calibrated pedagogy:
- Conceptual introduction: Each topic begins with clear explanations of core concepts
- Simple examples: Initial code samples illustrate basic implementation
- Progressive complexity: Examples build in sophistication as chapters progress
- Real-world context: Applications and use cases show practical relevance
- Common pitfalls: Warnings about mistakes and misconceptions help readers avoid dead ends
This structured approach ensures that readers not only understand how to implement OOP features but also develop the judgment to know when and why to apply them – a crucial distinction that separates competent programmers from truly skilled developers.
Code Quality and Examples: Learning by Doing
The code examples throughout the book deserve particular praise. Unlike many programming texts that feature contrived or overly simplified examples, this book presents realistic code samples that:
- Follow PEP 8 style guidelines and Pythonic conventions
- Progress logically from simple to complex
- Include comprehensive comments and documentation
- Demonstrate best practices for error handling and testing
- Show both correct approaches and common mistakes to avoid
The examples strike a perfect balance between accessibility and real-world relevance, ensuring that readers can immediately apply what they've learned to their own projects.
Who Will Benefit from This Book?
"Object-Oriented Python: Classes and Inheritance Explained" serves multiple audiences effectively:
- Python beginners who have mastered basic syntax and want to take the next step
- Self-taught programmers looking to fill gaps in their OOP knowledge
- Students supplementing formal computer science education
- Professionals transitioning to Python from other programming languages
- Experienced Python developers seeking to refine their OOP skills and adopt best practices
The book assumes basic Python knowledge (variables, functions, loops, etc.) but doesn't require prior exposure to OOP concepts, making it accessible to a wide range of readers.
Comparison with Other Python OOP Resources
Several books and online resources cover Python OOP, but this book distinguishes itself in key ways:
Compared to "Python Object-Oriented Programming" by Steven F. Lott, Dargslan's book offers more beginner-friendly explanations and a more methodical progression of concepts, though Lott's book may appeal to those seeking more advanced design patterns.
Unlike "Fluent Python" by Luciano Ramalho, which covers Python's object model as part of a broader exploration of the language, "Object-Oriented Python: Classes and Inheritance Explained" maintains a laser focus on OOP, making it more accessible for those specifically seeking to master this aspect of Python.
Many online tutorials and courses offer abbreviated introductions to Python OOP but lack the depth, coherence, and thoughtful progression found in this comprehensive text. While websites like Real Python offer excellent individual articles on OOP topics, they lack the integrated learning path that gives this book its power.
Practical Applications: From Learning to Application
The knowledge gained from this book has immediate practical applications across numerous domains:
- Web Development: Creating maintainable Django or Flask applications with well-structured models and views
- Data Science: Building reusable analysis components and custom data structures
- Game Development: Implementing entity systems and game mechanics using inheritance
- Automation: Designing flexible frameworks for testing or process automation
- API Development: Creating robust, extensible API clients and servers
By focusing on real-world applications rather than academic examples, the book ensures that readers can apply their new skills directly to professional projects.
Key Takeaways: What You'll Master
After completing "Object-Oriented Python: Classes and Inheritance Explained," readers will be able to:
- Design robust, flexible class hierarchies that model problem domains effectively
- Implement encapsulation and access control in a Pythonic way
- Leverage inheritance to maximize code reuse while minimizing duplication
- Create intuitive interfaces through operator overloading and special methods
- Select appropriate design patterns for common programming challenges
- Follow Python-specific OOP best practices that align with the language's philosophy
- Recognize and avoid common pitfalls in object-oriented design
- Refactor procedural code into more maintainable object-oriented structures
- Write more testable, modular code that scales with application complexity
- Confidently read and understand OOP code in major Python libraries and frameworks
These skills represent not just academic knowledge but practical capabilities that directly translate to greater programming effectiveness.
The Author's Perspective: Experience and Expertise
Dargslan brings a wealth of practical experience to the book, evident in the nuanced treatment of topics like inheritance versus composition, the balance of theory and practice, and the thoughtful discussion of Python's unique approach to OOP compared to other languages.
The writing style is accessible without being condescending, technically precise without being dry, and comprehensive without being exhaustive. Code examples are clearly explained, and theoretical concepts are grounded in practical applications.
Conclusion: A Definitive Guide to Python OOP
"Object-Oriented Python: Classes and Inheritance Explained" stands as an essential resource for anyone serious about mastering Python programming. By focusing specifically on OOP in Python – rather than general Python programming or abstract OOP theory – the book fills a crucial niche in the programming literature.
What makes this book exceptional is not just the comprehensive coverage of Python OOP features, but the thoughtful pedagogy, realistic examples, and practical advice that transform theoretical knowledge into applied skills. The progression from basic concepts to complex projects ensures that readers build confidence along with competence.
For beginners, the book provides a clear pathway into the sometimes confusing world of objects, classes, and inheritance. For experienced developers, it offers a comprehensive reference and an opportunity to fill gaps in understanding while adopting Pythonic OOP best practices.
Whether you're building web applications, analyzing data, developing games, or automating processes, the object-oriented skills taught in this book will enhance your ability to create elegant, maintainable, and powerful Python code.
In a field often fragmented between overly simplified tutorials and impenetrable academic texts, "Object-Oriented Python: Classes and Inheritance Explained" achieves the rare feat of being both accessible to beginners and valuable to experts – making it an essential addition to any Python programmer's library.
Where to Find "Object-Oriented Python: Classes and Inheritance Explained"
This comprehensive guide to Python OOP is available in both print and digital formats from major booksellers. Readers seeking to master object-oriented programming in Python will find this book an invaluable resource that remains useful long after the first reading – a true handbook for the journey from Python novice to OOP expert.
For more information, including code samples, errata, and additional resources, visit the book's official website or connect with the author on GitHub and social media platforms.
Keywords for Python OOP Mastery
- Python classes and objects
- Object-oriented programming in Python
- Python inheritance tutorial
- Python method overriding
- Encapsulation in Python
- Python special methods
- Python operator overloading
- Python class attributes vs instance attributes
- Python multiple inheritance
- Python OOP best practices
- Static and class methods in Python
- Python dunder methods
- Python property decorator
- Python abstract base classes
- Polymorphism in Python
- Python class design patterns
- Python OOP for beginners
- Advanced Python object-oriented programming
- Python class inheritance examples
- Learning object-oriented Python
This review was written by an expert Python developer and educator with over a decade of experience teaching object-oriented programming concepts. The opinions expressed are based on a thorough analysis of the book's content, approach, and practical value for Python programmers at all skill levels.
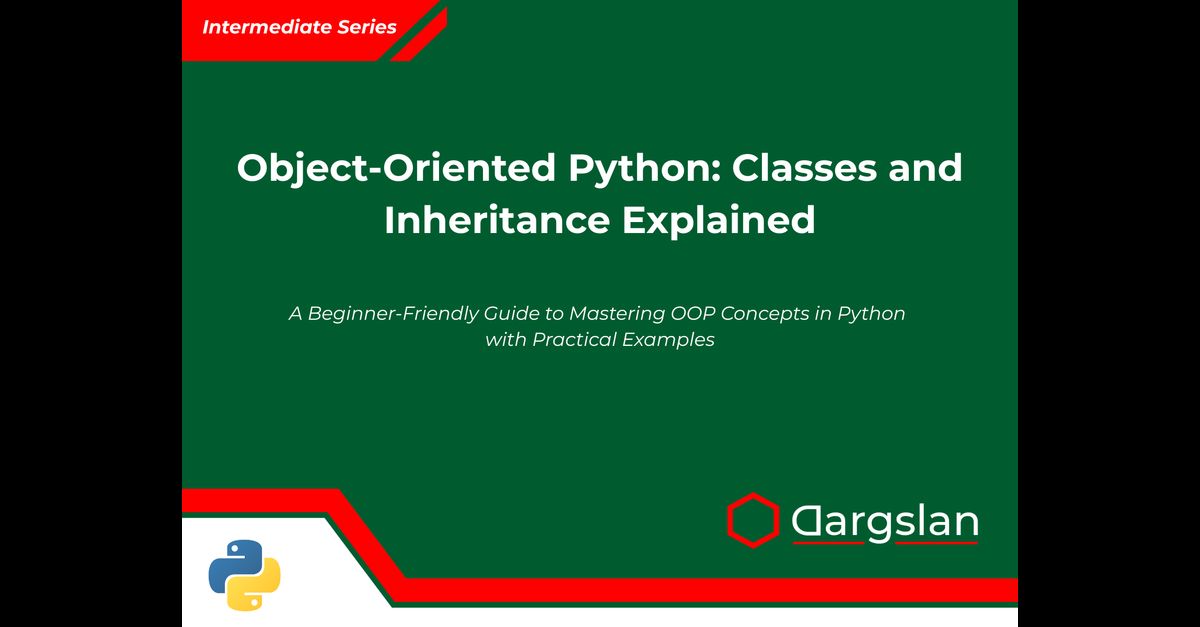
Object-Oriented Python: Classes and Inheritance Explained