Book Review: Learn Python Loops the Easy Way
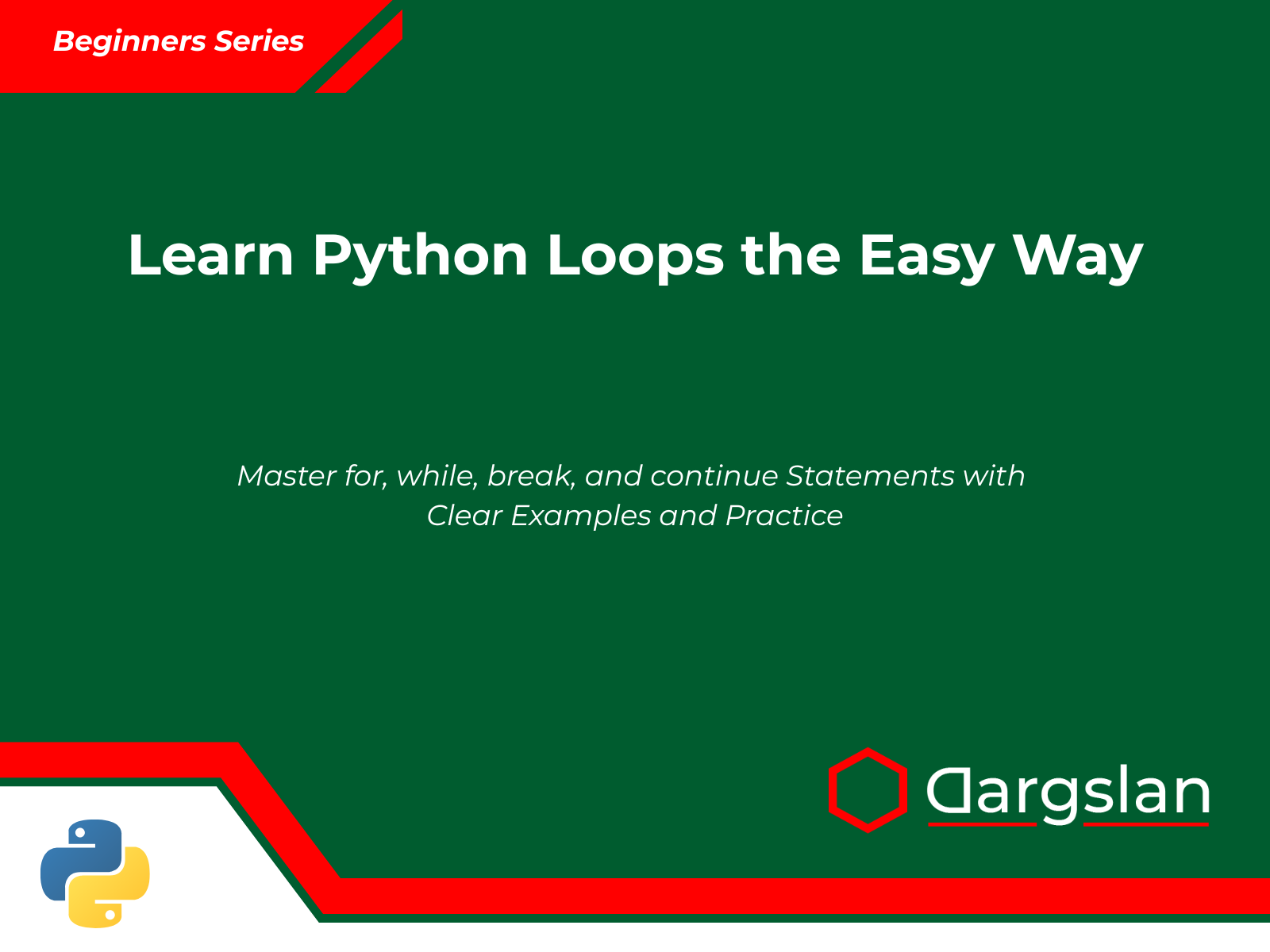
Learn Python Loops the Easy Way: The Ultimate Guide for Beginners and Intermediate Programmers
Book Review and Comprehensive Summary
Learn Python Loops the Easy Way is an exceptional educational resource that demystifies one of programming's most fundamental concepts. This well-structured guide takes readers from loop basics to advanced applications through clear explanations, practical examples, and hands-on projects.
What Makes This Python Loops Book Stand Out
Unlike many programming books that overwhelm readers with complex jargon, "Learn Python Loops the Easy Way" lives up to its title by breaking down concepts into digestible segments. Author Dargslan presents loops not just as syntax to memorize, but as powerful problem-solving tools that form the backbone of efficient programming.
The book's strength lies in its progressive approach, starting with fundamental concepts before building toward more complex applications. This scaffolded learning strategy ensures readers develop a solid foundation before tackling advanced topics.
Who Will Benefit From This Book
This guide serves multiple audiences effectively:
- Complete beginners will appreciate the clear explanations and step-by-step approach
- Self-taught programmers can fill knowledge gaps about loop concepts and best practices
- Computer science students will find it a valuable supplement to formal education
- Professional developers transitioning to Python will quickly grasp Python's loop implementation
- Teachers and trainers can utilize the structured content and examples in their instruction
Chapter-by-Chapter Breakdown
Chapter 1: Why Loops Matter in Programming
The book begins by establishing the crucial role loops play in programming. Rather than diving straight into syntax, the author takes time to explain why loops are essential for efficient coding. This foundation helps readers understand the purpose behind the patterns they'll learn.
Key topics include:
- The importance of automation in programming
- How loops reduce code redundancy
- Real-world scenarios where loops solve problems
- The computational thinking behind repetitive tasks
Chapter 2: Introduction to the for Loop
Chapter 2 introduces Python's for loop, arguably the most commonly used loop structure. The author explains:
- The syntax and components of for loops
- How for loops work with the range() function
- Iterating through sequences like lists, strings, and tuples
- When to choose a for loop over other approaches
# Example from the book showing a basic for loop
for number in range(1, 6):
print(f"Count: {number}")
This chapter lays the groundwork for the practical applications explored later in the book.
Chapter 3: Working with while Loops
Chapter 3 shifts focus to while loops, explaining their conditional nature and appropriate use cases. The author covers:
- The while loop structure and syntax
- Condition-based looping versus count-based looping
- Avoiding infinite loops (with practical examples of what not to do)
- Converting between for and while loops
# Example from the book demonstrating a while loop
counter = 1
while counter <= 5:
print(f"Count: {counter}")
counter += 1
The chapter includes particularly helpful comparisons between for and while loops, helping readers understand when each is most appropriate.
Chapter 4: The break Statement
Chapter 4 explores how to exit loops prematurely using the break statement. The author covers:
- The syntax and functionality of break
- Common scenarios for using break
- How break affects loop execution flow
- The difference between breaking from single versus nested loops
# Example showing break statement usage
for number in range(1, 100):
if number % 7 == 0 and number % 4 == 0:
print(f"Found it! {number}")
break
This chapter effectively demonstrates how break can make code more efficient by avoiding unnecessary iterations.
Chapter 5: The continue Statement
Building on the previous chapter, Chapter 5 introduces the continue statement for skipping iterations. Topics include:
- How continue differs from break
- Use cases for skipping certain loop iterations
- Patterns involving continue for data filtering
- Common mistakes when using continue
# Example demonstrating continue usage
for number in range(1, 11):
if number % 2 == 0:
continue
print(f"Odd number: {number}")
The author provides insightful examples showing how continue can simplify certain algorithms and data processing tasks.
Chapter 6: Looping Over Different Data Types
Chapter 6 expands the reader's perspective by exploring how loops interact with various Python data structures:
- Looping through lists, tuples, and sets
- Dictionary iteration (keys, values, and items)
- String manipulation using loops
- Working with specialized iterables
# Example showing dictionary iteration
student_grades = {"Alice": 92, "Bob": 85, "Charlie": 78}
for name, grade in student_grades.items():
print(f"{name} received {grade}%")
This chapter is particularly valuable for understanding Python's "iterable" concept, which underlies much of the language's elegance.
Chapter 7: Nested Loops Explained Simply
Chapter 7 tackles the often-challenging concept of nested loops with remarkable clarity:
- The mechanics of loops within loops
- Tracking multiple loop variables
- Performance considerations with nested loops
- Practical applications like coordinate systems and matrices
# Example of nested loops generating a multiplication table
for i in range(1, 6):
for j in range(1, 6):
print(f"{i} × {j} = {i*j}", end="\t")
print() # New line after each row
The author's approach to explaining nested loops through visual models and real-world analogies makes this potentially confusing topic accessible.
Chapter 8: Looping with Conditions
Chapter 8 explores the intersection of conditionals and loops:
- Combining if/else structures with loops
- Early termination versus complete iteration
- Flag variables and their role in loop control
- Pattern matching within sequences
# Example showing conditional processing in a loop
numbers = [10, 5, 8, 3, 12]
has_even = False
for num in numbers:
if num % 2 == 0:
has_even = True
print(f"Found even number: {num}")
This chapter demonstrates how to implement more sophisticated algorithms by combining loops with conditional logic.
Chapter 9: Common Loop Patterns
Chapter 9 provides a valuable collection of loop patterns found throughout programming:
- Accumulation patterns (sum, product, string building)
- Search patterns (linear search, find all, find first)
- Transformation patterns (mapping operations)
- Filtering patterns
- Nested data traversal
# Example of an accumulation pattern
total = 0
for number in range(1, 101):
total += number
print(f"Sum of numbers 1 to 100: {total}")
By identifying these patterns, the author helps readers recognize solutions they can adapt to their own coding challenges.
Chapter 10: Loop Practice Projects
The final chapter transitions from theory to application with hands-on projects:
- A number guessing game using while loops
- Data analysis scripts utilizing for loops
- A simple text parser demonstrating nested loops
- A menu-driven program showing loop control flow
Each project includes:
- A problem statement
- Expected output
- Step-by-step implementation
- Code explanation
- Enhancement challenges
This project-based approach consolidates learning and shows how the individual concepts combine in practical scenarios.
Valuable Appendices
The book includes four appendices that serve as ongoing references:
Appendix A: Loop Syntax Quick Reference
A comprehensive cheat sheet covering:
- All loop syntax variations
- Common iteration patterns
- Iteration protocol explanations
- Time complexity considerations
Appendix B: Beginner Mistakes with Loops
This troubleshooting guide addresses:
- Infinite loop scenarios and fixes
- Off-by-one errors
- Modifying collections during iteration
- Performance pitfalls
Appendix C: Practice Problems with Solutions
Additional exercises covering:
- Basic loop exercises
- Intermediate challenges
- Advanced problems
- Real-world scenarios
Appendix D: How to Debug Loops with print() and IDE Tools
Practical debugging techniques including:
- Strategic print statements
- Using breakpoints effectively
- Visualizing loop execution
- Identifying logical errors
Teaching Methodology and Approach
What distinguishes this book is its multifaceted teaching approach:
-
Visual Learning: The book incorporates flow diagrams and visualizations to illustrate loop execution.
-
Incremental Complexity: Concepts build progressively, with each chapter assuming mastery of previous material.
-
Consistent Examples: Related examples across chapters help readers see connections between concepts.
-
Practical Context: Real-world applications demonstrate why each loop technique matters.
-
Error Awareness: The author highlights common mistakes and misconceptions throughout.
Code Quality and Standards
The Python code examples follow modern best practices:
- PEP 8 compliant formatting
- Clear variable naming
- Appropriate comments
- Pythonic approaches to common tasks
This attention to quality ensures readers develop good habits from the beginning.
Why Loops Are Central to Programming Success
The book effectively makes the case that mastering loops is essential for programming proficiency. The author explains how loops:
- Enable data processing at scale
- Reduce code duplication
- Provide mechanisms for implementing algorithms
- Form the basis for more advanced programming techniques
By emphasizing these points throughout, the book keeps readers motivated to master these fundamental concepts.
Comparing Python Loops to Other Languages
The author occasionally compares Python's loop implementation to other languages like JavaScript, Java, and C++, helping readers who may have experience in other programming environments make connections to their existing knowledge.
These comparisons highlight Python's clean syntax and the "Pythonic" way of handling iterations, such as the elegant for-in loop that abstracts away many details programmers must manage manually in other languages.
Digital Resources and Interactive Elements
The book mentions companion resources available online, including:
- Downloadable code examples
- Interactive quizzes
- Additional exercises
- Video walkthroughs of key concepts
These supplementary materials extend the learning experience beyond the printed page.
Perfect for Self-Paced Learning
One of the book's greatest strengths is its suitability for self-study. Each chapter includes:
- Clear learning objectives
- Knowledge check questions
- Summary points
- Review exercises
This structure helps independent learners track their progress and ensure comprehension before moving forward.
Beyond Basic Loops: Advanced Concepts
While focused on core loop concepts, the book doesn't shy away from introducing more advanced ideas:
- Generator expressions and their relationship to loops
- The iteration protocol in Python
- Efficient alternatives to loops for specific operations
- How loops interact with Python's memory model
These advanced topics are presented as extensions rather than requirements, making them accessible without overwhelming beginners.
Language Accessibility
The author's writing style deserves special mention for its clarity and accessibility. Technical concepts are explained using everyday analogies, and jargon is introduced gradually with clear definitions. This approach makes the material approachable for readers from diverse backgrounds.
Final Assessment
"Learn Python Loops the Easy Way" delivers on its promise to make loops accessible and comprehensible. The book's structured approach, practical examples, and focus on understanding rather than memorization create an effective learning experience.
The comprehensive coverage—from basic syntax to complex patterns—ensures readers develop both the knowledge and confidence to implement loops in their own projects. The inclusion of common pitfalls and debugging techniques prepares readers for the challenges they'll face in real-world programming scenarios.
Whether you're a complete beginner or looking to solidify your understanding of Python's loop constructs, this book provides a clear path to mastery. By the final page, readers will not only know how to write loops but will understand when and why to apply different loop structures to solve programming challenges efficiently.
Who Should Read This Book
- Python beginners wanting to strengthen their foundational skills
- Self-taught programmers filling knowledge gaps
- Students supplementing their formal computer science education
- Experienced programmers transitioning to Python
- Anyone who has struggled with loops concepts in the past
Who Might Need Something Different
- Very advanced Python developers seeking specialized optimization techniques
- Readers looking for a general Python language reference
- Those seeking extensive coverage of algorithmic theory
The Verdict
"Learn Python Loops the Easy Way" earns a strong recommendation for its clear explanations, practical approach, and comprehensive coverage of loop concepts. It transforms what can be a challenging topic into an accessible and even enjoyable learning journey.
Through thoughtful organization, relevant examples, and hands-on practice, the book provides readers with both the understanding and confidence to implement loops effectively in their Python projects. For anyone looking to master this essential programming concept, this book represents an excellent investment in their programming education.
Whether you're writing your first Python script or looking to optimize existing code, understanding loops is essential. "Learn Python Loops the Easy Way" provides the foundation you need to write more efficient, elegant, and powerful code through mastery of Python's loop structures and patterns.
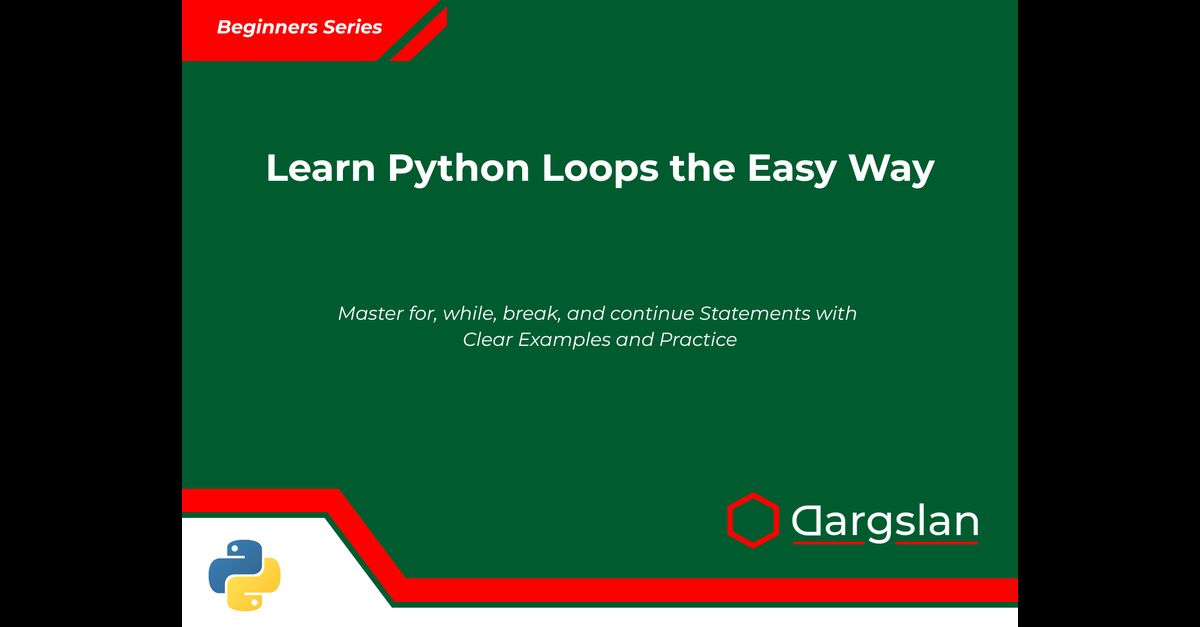