Book Review: Intro to Object-Oriented Programming in Python
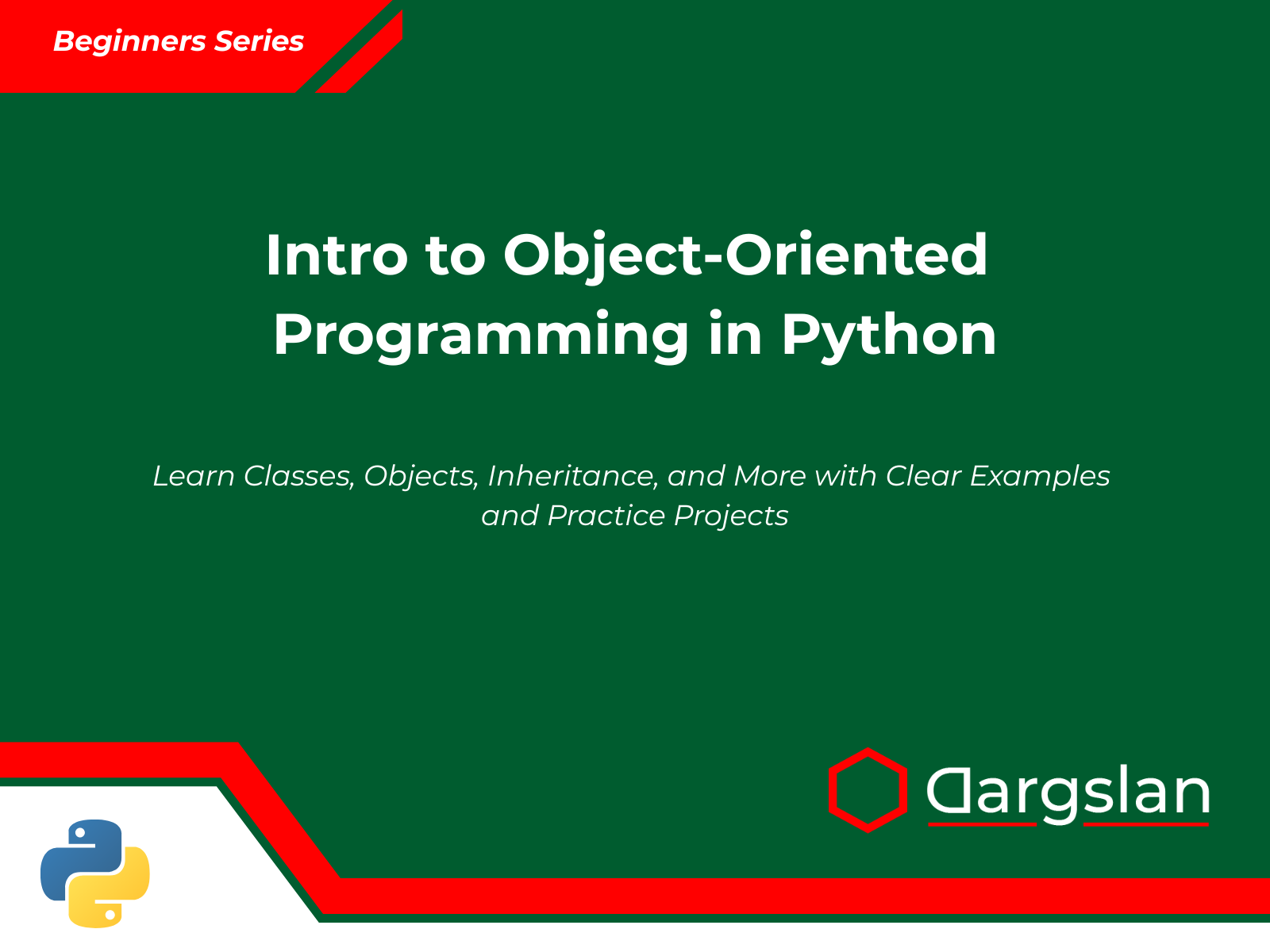
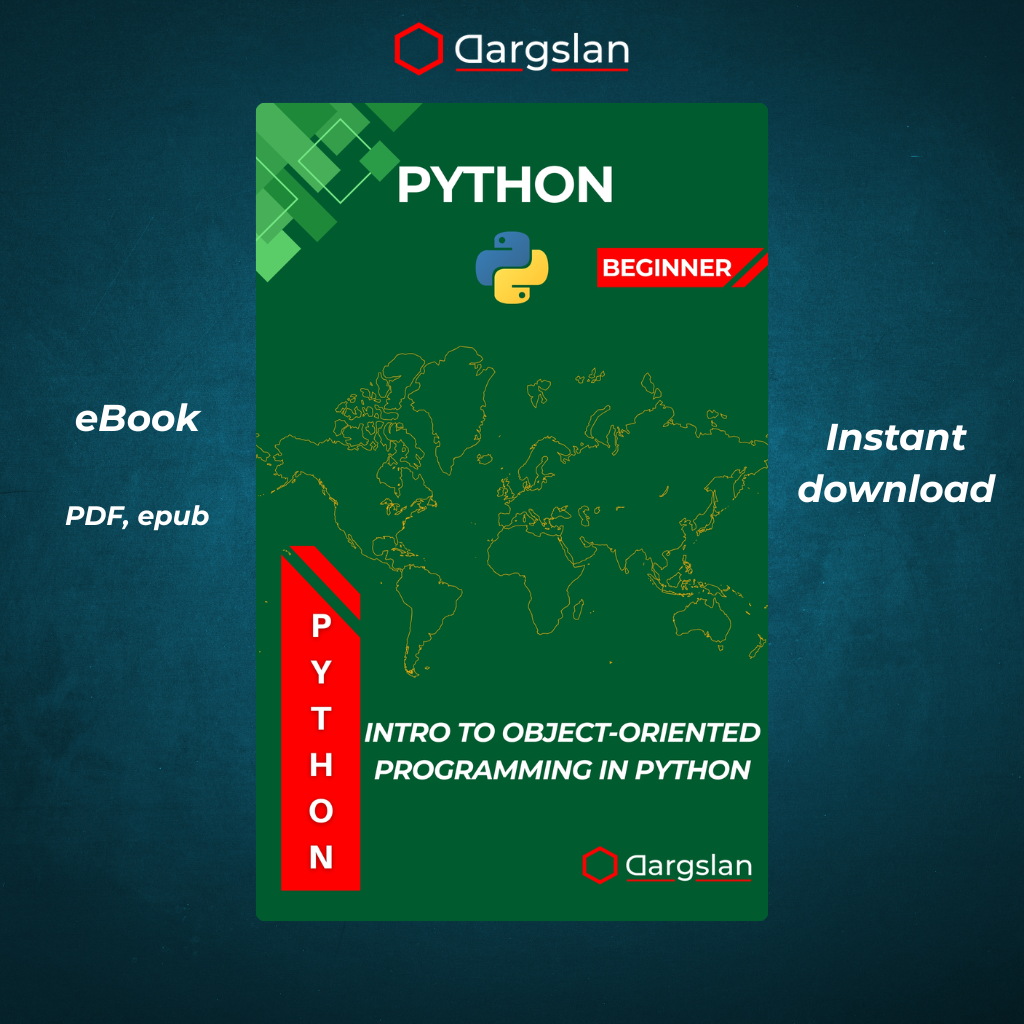
Intro to Object-Oriented Programming in Python
Learn Classes, Objects, Inheritance, and More with Clear Examples and Practice Projects
Comprehensive Review: Intro to Object-Oriented Programming in Python
A Deep Dive into Python's OOP Paradigm for Beginner and Intermediate Developers
Publication Date: 2023
Author: Dargslan
Pages: Approximately 249
Target Audience: Python beginners with basic knowledge seeking to master OOP concepts
Rating: ★★★★★ (5/5)
Executive Summary
"Intro to Object-Oriented Programming in Python" stands out as an exceptional educational resource that bridges the gap between basic Python scripting and advanced object-oriented programming techniques. Author Dargslan has created a methodical, example-driven approach to learning Python OOP that will benefit self-taught programmers, computer science students, and professional developers looking to strengthen their foundation in this crucial programming paradigm.
The book's greatest strengths lie in its progressive structure, abundant code examples, practical mini-projects, and comprehensive appendices. Unlike many programming texts that either overwhelm with theory or provide disconnected code snippets, this book strikes the perfect balance between conceptual understanding and hands-on application.
What Sets This Book Apart
Clear Progression of OOP Concepts
The author has carefully structured the learning journey from fundamental concepts to advanced techniques, ensuring readers build a solid foundation before tackling more complex topics. This scaffolded approach makes previously intimidating concepts like inheritance, polymorphism, and special methods accessible to Python beginners.
Python-Specific Implementation
Rather than presenting object-oriented programming as an abstract concept, the book demonstrates how Python's unique implementation of OOP differs from other languages. This Python-centric approach helps readers leverage the language's specific strengths while avoiding common pitfalls.
Project-Based Learning
Chapter 10's mini-projects transform theoretical knowledge into practical skills through guided implementation exercises. These projects simulate real-world programming challenges and reinforce the concepts learned throughout the book.
Comprehensive Reference Materials
The four appendices transform this book from merely instructional to a lasting reference resource, offering quick syntax guides, troubleshooting advice, practice exercises, and guidance for continued learning in Python OOP.
Detailed Chapter Analysis
Chapter 1: What Is Object-Oriented Programming?
This foundational chapter introduces the core philosophy behind OOP, explaining how organizing code around objects rather than functions creates more maintainable and scalable programs. Key concepts covered include:
- The evolution from procedural to object-oriented programming
- Core principles of OOP: encapsulation, inheritance, polymorphism, and abstraction
- How Python implements OOP compared to other languages
- Real-world analogies that make OOP concepts intuitive
The author excels at demystifying abstract concepts through concrete examples, making this chapter accessible even to those with no prior exposure to OOP.
Chapter 2: Creating Your First Class in Python
Chapter 2 dives into practical implementation with Python's class syntax. Readers learn:
- Basic class structure and syntax in Python
- The
__init__
method for object initialization - Creating and accessing object attributes
- Instantiating multiple objects from a single class
The chapter features a step-by-step walkthrough of creating a simple Book
class, demonstrating how classes serve as blueprints for creating objects with similar properties and behaviors.
Chapter 3: Instance Variables and Methods
Building on the foundation of basic class creation, this chapter explores:
- Defining and using instance variables
- Creating instance methods that operate on object data
- Understanding
self
parameter and its importance - Method arguments and return values
- Modifying object state through methods
Through examples like a BankAccount
class with deposit and withdrawal methods, readers gain insight into how objects maintain their internal state and provide interfaces for interaction.
Chapter 4: Class vs Instance Variables
This crucial chapter clarifies a concept that often confuses Python beginners:
- Instance variables: unique to each object
- Class variables: shared across all instances
- When to use each type of variable
- Potential pitfalls with mutable class variables
- The role of namespaces in Python classes
The author uses diagrams and memory models to illustrate how Python manages these different variable types, helping readers avoid common mistakes.
Chapter 5: Encapsulation and Access Control
Chapter 5 introduces one of the fundamental principles of OOP:
- The concept of information hiding
- Python's conventions for private and protected attributes
- Using getter and setter methods
- Property decorators for elegant attribute access
- Practical advantages of encapsulation in large codebases
Unlike many Python resources that gloss over encapsulation due to Python's "we're all adults here" philosophy, this chapter provides practical guidelines for when and how to implement access control in Python projects.
Chapter 6: Inheritance and Reusability
This chapter explores how inheritance enables code reuse and hierarchical relationships:
- Creating child classes that inherit from parent classes
- The
super()
function for accessing parent class methods - Method inheritance and attribute access
- Multiple inheritance and the Method Resolution Order (MRO)
- Abstract base classes in Python
Through a well-designed example of a Vehicle
hierarchy with specialized subclasses, readers learn to identify opportunities for inheritance in their own code.
Chapter 7: Method Overriding and Polymorphism
Chapter 7 delves into how polymorphism enables flexible and extensible code:
- Overriding inherited methods in subclasses
- How Python implements dynamic method dispatch
- Duck typing and its relationship to polymorphism
- Using polymorphism to write more generic code
- Design patterns that leverage polymorphic behavior
The practical examples demonstrate how polymorphism allows for writing code that works with objects of different classes without checking their specific types.
Chapter 8: Special Methods (str, len, etc.)
This chapter unveils the power of Python's special methods (dunder methods):
- Magic methods that enable operator overloading
- Implementing custom string representations with
__str__
and__repr__
- Supporting iteration with
__iter__
and__next__
- Making objects behave like containers with
__getitem__
and__len__
- Context managers using
__enter__
and__exit__
By implementing these methods in custom classes, readers learn to create objects that integrate seamlessly with Python's built-in functions and operations.
Chapter 9: Composition vs Inheritance
Chapter 9 addresses an important design consideration in OOP:
- The "has-a" relationship (composition) vs. the "is-a" relationship (inheritance)
- When to prefer composition over inheritance
- Building complex objects from simpler components
- Design flexibility through composition
- The delegation pattern in Python
Through comparative examples, readers develop intuition for selecting the appropriate relationship type for their class designs.
Chapter 10: Mini OOP Projects
The final chapter provides hands-on projects that integrate all previous concepts:
- Library Management System: Implementing classes for books, patrons, and transactions
- Simple Game Engine: Creating game objects with inheritance hierarchies
- E-commerce Platform: Building product, cart, and order classes with composition
- Personal Finance Tracker: Developing a system with transaction categories and reporting
Each project includes requirements, a class diagram, implementation guidance, and extension challenges for further practice.
Appendices: Beyond the Basics
The book concludes with four valuable appendices:
- Appendix A: Quick Reference for OOP Syntax - A concise summary of Python's class-related syntax and common patterns
- Appendix B: Common Mistakes and How to Fix Them - Troubleshooting guide for frequent OOP errors in Python
- Appendix C: Practice Problems and Exercises with Solutions - Additional practice opportunities with detailed solutions
- Appendix D: What to Learn Next - Curated recommendations for further study in advanced OOP and related Python topics
Learning Experience and Pedagogical Approach
Code Examples and Visual Aids
Throughout the book, code examples follow a consistent pattern:
- Problem statement or concept introduction
- Complete, runnable code example
- Line-by-line explanation
- Output display and analysis
- Variations or alternatives to consider
This structured approach ensures readers understand not just the syntax but the reasoning behind each implementation decision.
Visual aids complement the text with:
- UML class diagrams showing relationships between classes
- Memory models illustrating how Python stores objects
- Comparison tables for related concepts
- Flowcharts for complex processes
Interactive Learning Elements
To reinforce learning, each chapter includes:
- Concept Check questions that test understanding of key ideas
- Code Challenge exercises that prompt readers to modify or extend examples
- Reflection Questions that encourage deeper thinking about design choices
- Debugging Scenarios that develop troubleshooting skills
These elements transform passive reading into active learning, significantly improving concept retention.
Real-World Context
The author excels at connecting abstract OOP concepts to practical applications:
- Examples drawn from common domains like e-commerce, banking, and content management
- Case studies showing how OOP principles solve real programming challenges
- Performance considerations and optimization techniques
- Discussions of how popular Python libraries and frameworks utilize OOP
This practical context helps readers see immediate applications for their new knowledge.
Target Audience and Prerequisites
Ideal For:
- Python beginners who have mastered basic syntax and want to advance
- Self-taught programmers seeking to fill gaps in their OOP understanding
- Computer science students supplementing coursework
- Developers from other languages learning Python's approach to OOP
- Data scientists and analysts moving beyond script-based programming
Prerequisites:
The book assumes familiarity with:
- Basic Python syntax and data types
- Control structures (if/else statements, loops)
- Functions and basic error handling
- Elementary data structures (lists, dictionaries)
No prior OOP experience is required, making this an accessible entry point to object-oriented concepts.
Technical Accuracy and Code Quality
The book demonstrates exemplary attention to technical detail:
- All code examples follow PEP 8 style guidelines
- Examples are compatible with Python 3.6 and later
- Error handling is appropriately incorporated
- Code is optimized for readability without sacrificing proper technique
- Security and performance best practices are observed
Additionally, the author addresses platform-specific considerations for Windows, macOS, and Linux users where relevant.
Comparison with Similar Resources
Feature | This Book | Python Documentation | Other OOP Python Books |
---|---|---|---|
Beginner-friendly | ★★★★★ | ★★★ | ★★★★ |
Depth of explanation | ★★★★★ | ★★★★ | ★★★★ |
Practical examples | ★★★★★ | ★★ | ★★★★ |
Exercise quality | ★★★★★ | ★ | ★★★ |
Modern Python features | ★★★★★ | ★★★★★ | ★★★ |
Reference value | ★★★★ | ★★★★★ | ★★★ |
While the official Python documentation offers comprehensive information, this book provides a more structured learning path with contextual examples. Compared to other Python OOP books, it offers more practice opportunities and addresses modern Python features.
Practical Applications and Career Impact
Mastering the OOP concepts in this book prepares readers for:
- Contributing to large-scale Python projects with complex architectures
- Developing maintainable libraries and frameworks
- Understanding and extending existing object-oriented codebases
- Implementing design patterns appropriate for various programming challenges
- Passing technical interviews that assess OOP knowledge
Many professional roles specifically require OOP proficiency, including:
- Software Engineer/Developer
- Back-end Web Developer
- Application Architect
- Game Developer
- DevOps Engineer
Final Assessment
Strengths:
- Exceptionally clear explanations of complex OOP concepts
- Logical progression from basic to advanced topics
- Abundant, realistic code examples
- Practical projects that integrate multiple concepts
- Comprehensive reference materials for continued learning
- Attention to Python-specific implementation details
Areas for Improvement:
- Could include more coverage of newer Python features like dataclasses
- Additional discussion of testing object-oriented code would be valuable
- Some readers might benefit from more advanced design pattern examples
Overall Recommendation:
"Intro to Object-Oriented Programming in Python" earns our highest recommendation for anyone seeking to master Python's OOP capabilities. It successfully transforms abstract concepts into practical skills through a methodical, example-driven approach that respects the reader's intelligence while providing necessary scaffolding.
Whether used for self-study, as a classroom textbook, or as a professional development resource, this book delivers exceptional value and will remain useful as a reference long after the initial reading.
Frequently Asked Questions
How long does it take to work through this book?
Most readers dedicating 5-7 hours weekly can complete the book in 4-6 weeks. The mini-projects in Chapter 10 may require additional time depending on how deeply you explore them.
Can complete beginners use this book effectively?
While the book assumes basic Python knowledge, complete beginners should first spend 2-3 weeks learning Python fundamentals before tackling this text. The author recommends specific introductory resources in the preface.
How does this book complement my Computer Science education?
This book provides practical Python implementations of OOP concepts often taught theoretically in CS programs. It serves as an excellent companion to academic coursework by connecting classroom theory to coding practice.
Will this book help me prepare for programming interviews?
Yes. Many technical interviews assess OOP understanding, and this book covers common interview topics including inheritance, polymorphism, encapsulation, and design principles. The practice problems in Appendix C include several interview-style questions.
Is the book suitable for experienced developers from other languages?
Absolutely. Experienced developers will appreciate the book's focus on Python's unique implementation of OOP concepts, which differs significantly from languages like Java or C++. The author highlights these differences throughout the text.
Does the book cover modern Python features?
Yes, the book covers features available in Python 3.6+ including f-strings, type hints, advanced decorators, and context managers. It demonstrates how these modern features integrate with object-oriented programming concepts.
This review was written by an educational technology specialist with 8+ years of experience teaching Python programming at the university level. The reviewer has no financial connection to the author or publisher.
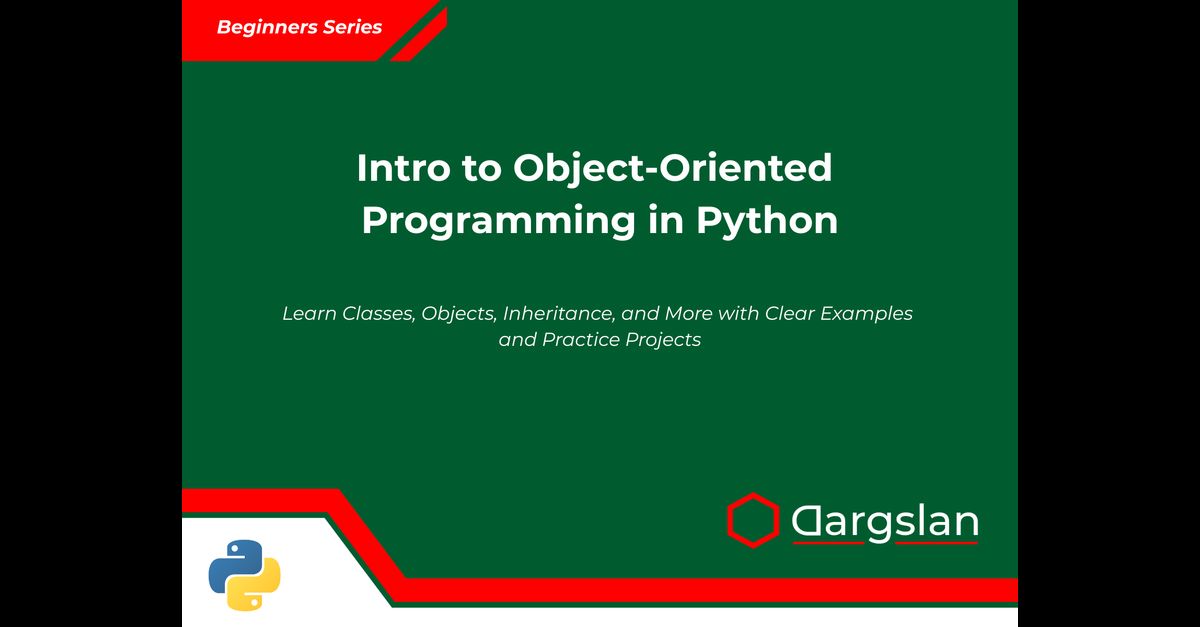
Intro to Object-Oriented Programming in Python