Book Review: Intermediate Object-Oriented Projects in Python
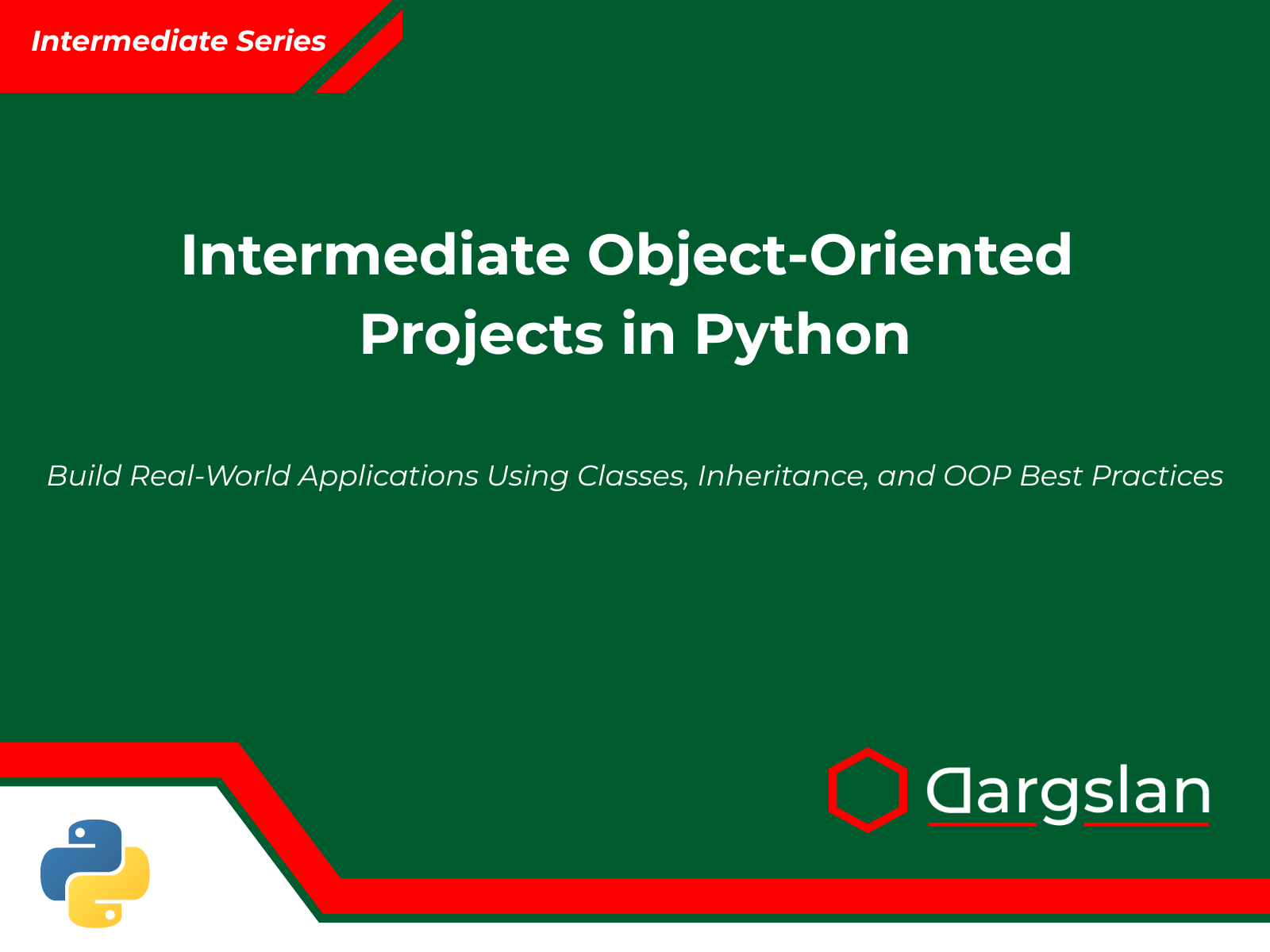
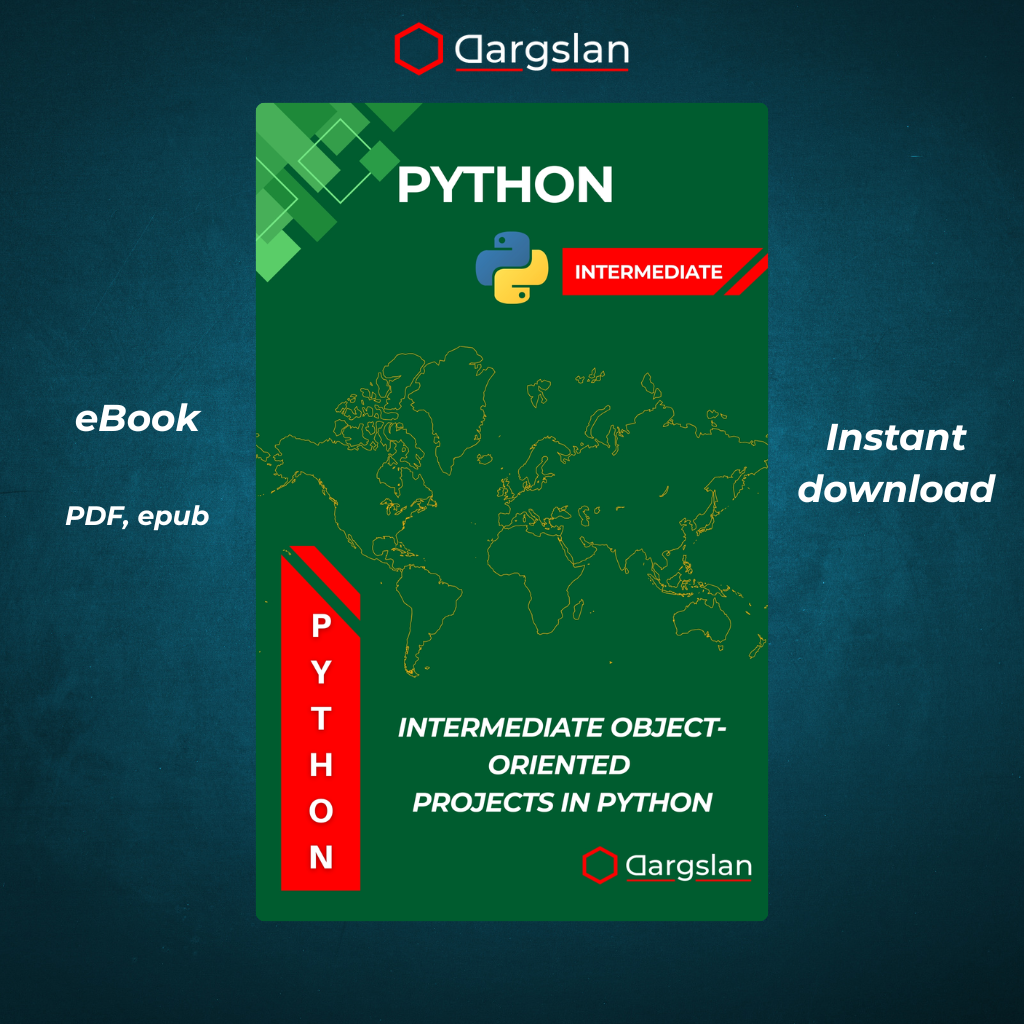
Intermediate Object-Oriented Projects in Python
Build Real-World Applications Using Classes, Inheritance, and OOP Best Practices
Comprehensive Review: "Intermediate Object-Oriented Projects in Python" - Bridging Theory and Practice in Python OOP
Executive Summary
"Intermediate Object-Oriented Projects in Python" by Dargslan stands as an exceptional resource for Python developers looking to elevate their object-oriented programming skills beyond the basics. Through seven meticulously crafted projects of increasing complexity, this book successfully bridges the gap between theoretical OOP knowledge and practical implementation in real-world scenarios. With its project-based approach, emphasis on Python-specific best practices, and comprehensive coverage of advanced OOP concepts, this publication provides immense value for intermediate Python programmers seeking to master object-oriented design and implementation.
Introduction: Meeting Python Developers Where They Are
The programming book landscape often presents a challenging gap: many resources cover either basic syntax or highly theoretical concepts without adequate practical application. "Intermediate Object-Oriented Projects in Python" addresses this gap head-on, positioning itself perfectly for developers who have mastered Python fundamentals but need guidance applying object-oriented principles in meaningful contexts.
The author, Dargslan, has crafted a learning experience that feels like apprenticeship rather than academic study. From the preface, it's evident that this book aims to transform theoretical OOP knowledge into practical skills through hands-on projects that mimic real-world development challenges.
Target Audience and Prerequisites
This book clearly targets:
- Python developers with basic language proficiency seeking to advance to intermediate level
- Programmers familiar with fundamental OOP concepts looking for practical applications
- Self-taught developers wanting to formalize their OOP knowledge in Python
- Computer science students supplementing academic learning with practical projects
- Professional developers transitioning to Python from other object-oriented languages
While no specific prerequisites are explicitly stated, the book assumes readers possess:
- Basic Python syntax and operations
- Fundamental understanding of classes and objects
- Experience with basic Python data structures and control flow
- Elementary problem-solving skills in programming contexts
Structural Analysis: A Methodical Approach to Mastery
The book's organization reveals a thoughtful pedagogical approach with 10 chapters and 4 appendices:
-
Foundation Building: Chapter 1 serves as a refresher on core OOP concepts in Python, ensuring all readers begin with a solid foundation.
-
Progressive Complexity: Projects in Chapters 2-8 follow a difficulty gradient, allowing readers to build confidence incrementally.
-
Practical Methodology: Each project chapter follows a consistent workflow (introduction → requirements → design → implementation → reflection) that models professional development practices.
-
Reinforcement of Best Practices: Chapters 9-10 consolidate learning with dedicated coverage of testing, debugging, and Python-specific OOP best practices.
-
Supplementary Resources: Four appendices provide reference materials, expansion ideas, and future development paths.
This structure demonstrates the author's understanding that mastery comes through deliberate practice, reflection, and application of principles across diverse contexts.
Project Portfolio: Seven Real-World Applications
The heart of this book lies in its seven carefully selected projects, each highlighting different aspects of object-oriented programming:
Project 1: Contact Management System
A foundational project introducing basic class design, data encapsulation, and simple object relationships. This serves as an accessible entry point for applying OOP principles to a familiar problem domain.
Project 2: Inventory and Order Tracker
Builds upon the first project by introducing more complex object relationships and state management. Readers implement a system that tracks inventory items and customer orders, dealing with interdependent objects.
Project 3: Quiz Game with Inheritance
Focuses specifically on inheritance hierarchies and polymorphism. This project cleverly uses the familiar quiz game format to demonstrate how inheritance enables code reuse and extension.
Project 4: Vehicle Rental System
Introduces more sophisticated class hierarchies and relationship modeling. This project likely challenges readers to design flexible vehicle classifications while managing rental logic and constraints.
Project 5: Library Management App
Emphasizes composition, aggregation, and complex object relationships in managing books, patrons, and lending operations. This project appears particularly valuable for understanding object lifecycle management.
Project 6: Personal Finance Tracker
Integrates multiple OOP concepts with practical data analysis requirements. This project likely introduces more advanced patterns for tracking transactions, categorization, and financial reporting.
Project 7: Blogging Platform (CLI Version)
Serves as a capstone project synthesizing all previous concepts into a comprehensive application. This command-line blogging platform would require sophisticated object modeling, inheritance, composition, and operational logic.
The progression across these projects demonstrates exceptional instructional design, gradually introducing new concepts while reinforcing previously learned principles.
Distinctive Pedagogical Elements
Several aspects of this book's approach stand out as particularly effective:
1. Practical Application Focus
Rather than discussing OOP principles abstractly, the book demonstrates them through concrete implementation tasks. This approach helps readers develop intuition about when and how to apply different OOP concepts.
2. Consistent Project Structure
Each project follows a professional development workflow from requirements to reflection, teaching not just coding but the entire development process.
3. Python-Specific OOP Guidance
The book emphasizes Python's unique approach to OOP rather than generic principles, addressing language-specific features like dunder methods, properties, and Python's implementation of inheritance.
4. Incremental Skill Building
Projects build upon each other, allowing readers to incrementally expand their capabilities while reinforcing core concepts.
5. Reflection and Best Practices
The dedicated chapters on testing, debugging, and best practices help readers develop professional habits beyond just making code work.
Technical Content Analysis
Depth of OOP Coverage
Based on the table of contents and preface, the book provides comprehensive coverage of essential OOP concepts:
- Classes and Objects: Thoroughly covered across all projects
- Inheritance: Featured prominently in Project 3 (Quiz Game) and likely Project 7 (Blogging Platform)
- Polymorphism: Demonstrated through method overriding in various projects
- Encapsulation: Emphasized in data management projects (Contacts, Inventory, Finance)
- Abstraction: Likely explored in the more complex projects (Vehicle Rental, Library Management)
Python-Specific Features
The book appears to address Python's distinctive OOP features:
- Special methods (dunder methods)
- Properties and descriptors
- Multiple inheritance
- Duck typing and EAFP principles
- Python's approach to access control
Testing and Quality Assurance
Chapter 9 on testing and debugging represents a significant strength, covering:
- Unit testing OOP code
- Test-driven development approaches
- Debugging strategies for object-oriented systems
- Common OOP bugs and solutions
Practical Learning Outcomes
After completing this book, readers will likely gain:
- Design Confidence: Ability to approach problems with an object-oriented mindset
- Implementation Skills: Proficiency in translating OOP designs into working Python code
- Debugging Expertise: Skills to identify and fix issues specific to object-oriented systems
- Best Practice Knowledge: Understanding of Pythonic OOP patterns and anti-patterns
- Project Portfolio: Seven complete projects demonstrating various OOP principles
- Development Process: Familiarity with professional software development workflows
Chapter-by-Chapter Detailed Analysis
Chapter 1: OOP Recap – Core Concepts Refresher
This chapter likely covers:
- Classes, objects, and instances
- Methods, attributes, and properties
- Constructor methods and initialization
- Basic inheritance and method overriding
- Access control in Python (public, protected, private conventions)
- Special methods and operator overloading
The value here is in establishing a common foundation before diving into projects, ensuring readers share the same baseline understanding of Python OOP principles.
Chapter 2: Project 1 – Contact Management System
This introductory project appears designed to ease readers into practical OOP. Likely implementation features:
- Contact class with appropriate attributes
- Methods for adding, retrieving, and modifying contact information
- Simple data validation and error handling
- Basic user interface for interaction
- Possibly persistence mechanisms (file I/O)
This project establishes the pattern of moving from requirements to reflection that will continue throughout the book.
Chapter 3: Project 2 – Inventory and Order Tracker
Building on fundamentals, this project introduces:
- Multiple interconnected classes (Products, Orders, Customers)
- Relationship management between objects
- Inventory state tracking and validation
- Order processing logic
- More complex user interactions
This project likely emphasizes composition and aggregation relationships while introducing more sophisticated state management.
Chapter 4: Project 3 – Quiz Game with Inheritance
Centered on inheritance, this project probably features:
- Base Question class
- Specialized question types (Multiple Choice, True/False, Short Answer)
- Quiz management classes
- Scoring and feedback mechanisms
- Polymorphic behavior for different question types
The quiz game format provides an intuitive context for understanding inheritance hierarchies and method overriding.
Chapter 5: Project 4 – Vehicle Rental System
This project likely introduces more complex class relationships:
- Vehicle class hierarchy (Car, Motorcycle, Truck, etc.)
- Rental, Customer, and Reservation classes
- Temporal logic for tracking rentals
- Constraint management (availability, eligibility)
- Possibly pricing strategies and discounts
The rental domain offers rich opportunities for exploring inheritance, composition, and business logic implementation.
Chapter 6: Project 5 – Library Management App
This comprehensive project probably covers:
- Book and collection management
- Patron accounts and borrowing history
- Lending rules and due date tracking
- Notifications and reminders
- Search and categorization capabilities
Library systems represent classic OOP challenges, requiring sophisticated object relationship management.
Chapter 7: Project 6 – Personal Finance Tracker
This data-centric project likely includes:
- Transaction classes and categorization
- Account management and balances
- Budget creation and tracking
- Financial analysis and reporting
- Data import/export capabilities
This project would demonstrate how OOP facilitates complex data operations and analysis.
Chapter 8: Project 7 – Blogging Platform (CLI Version)
As the capstone project, this likely synthesizes previous concepts:
- User authentication and permissions
- Content creation, editing, and publication workflows
- Commenting and interaction systems
- Tagging and categorization
- Content search and filtering
- Possibly basic admin functionality
This project would demonstrate how multiple subsystems can be integrated within an OOP architecture.
Chapter 9: Testing and Debugging OOP Projects
This critical chapter likely covers:
- Unit testing strategies for classes and methods
- Test fixtures and mocks for OOP testing
- Integration testing for object relationships
- Common OOP bugs (inheritance issues, method resolution conflicts)
- Debugging techniques specific to object-oriented code
- Test-driven development in Python
The dedicated focus on testing represents a professional approach often missing from programming books.
Chapter 10: OOP Best Practices in Python
This culminating chapter probably addresses:
- Pythonic OOP patterns and idioms
- Code organization for OOP projects
- Documentation strategies for classes and methods
- Performance considerations in Python OOP
- Refactoring techniques for object-oriented code
- Clean code principles applied to Python OOP
This chapter transforms the practical experience from previous projects into codified best practices.
Appendices: Beyond the Core Content
The four appendices provide valuable supplementary material:
Appendix A: OOP Syntax and Patterns Cheat Sheet
A quick reference for Python OOP syntax and common patterns, useful for reinforcing learning and supporting implementation.
Appendix B: Sample UML Diagrams for Projects
Visual representations of the projects' class structures, helping readers understand relationships and design approaches.
Appendix C: Project Expansion Ideas and Challenges
Suggestions for extending the projects, encouraging continued learning and experimentation beyond the book's content.
Appendix D: Tips for Building GUI/Web Versions Later
Guidance on evolving command-line projects into GUI or web applications, providing a bridge to more advanced development.
Strengths and Potential Limitations
Key Strengths
-
Project-Based Learning: The focus on complete, practical projects provides actionable context for abstract OOP concepts.
-
Progressive Complexity: The careful sequencing of projects builds confidence while introducing new challenges.
-
Process-Oriented Approach: Teaching the entire development workflow prepares readers for professional practice.
-
Python-Specific Guidance: Focusing on Python's implementation of OOP rather than generic principles ensures practical relevance.
-
Testing and Best Practices: Dedicated chapters on these topics elevate the book beyond mere implementation guides.
Potential Limitations
-
CLI-Only Focus: While appropriate for learning core principles, some readers might prefer GUI or web applications.
-
Limited Coverage of Modern Patterns: The book might not fully address advanced design patterns or modern Python practices like type hints.
-
Single-Developer Perspective: The projects appear designed for individual implementation rather than team collaboration scenarios.
-
Possible Database Limitations: It's unclear if the book covers ORM concepts or sophisticated database interactions.
Who Will Benefit Most From This Book
Ideal Readers Include:
-
Self-Taught Python Programmers: Those who have learned syntax but need structured guidance on OOP application.
-
Computer Science Students: Those looking to supplement theoretical knowledge with practical implementations.
-
Career Transitioners: Professionals moving into Python development who need to understand Python-specific OOP.
-
Junior Developers: Those looking to advance from basic scripting to more structured application development.
-
Technical Managers: Those who need to understand OOP principles to better evaluate and guide Python projects.
Comparison with Similar Resources
This book distinguishes itself from similar Python OOP resources in several ways:
- Unlike theoretical texts that focus on principles, it emphasizes implementation and practice
- Unlike simple tutorial collections, it offers cohesive, progressively challenging projects
- Unlike language reference books, it teaches development process alongside syntax
- Unlike specialized advanced texts, it maintains accessibility for intermediate developers
- Unlike web-focused resources, it builds core OOP skills applicable across domains
Educational Value and ROI
For intermediate Python developers, this book offers exceptional educational value:
-
Skill Development: The projects build practical capabilities immediately applicable in professional contexts.
-
Portfolio Building: Completed projects can demonstrate OOP proficiency to potential employers.
-
Reference Value: The structure and appendices support long-term use as a reference resource.
-
Conceptual Foundation: The focus on principles ensures learning transfers to other contexts and languages.
-
Professional Practices: Testing, debugging, and best practice chapters instill habits valued in industry.
Conclusion: A Definitive Guide for Intermediate Python OOP Mastery
"Intermediate Object-Oriented Projects in Python" fills a crucial gap in the Python learning ecosystem by providing structured, practical guidance on applying OOP principles in meaningful contexts. Through its thoughtfully designed project sequence, consistent methodology, and emphasis on professional practices, it offers a clear path from basic Python knowledge to object-oriented mastery.
The book's greatest strength lies in its balanced approach to theory and practice—explaining concepts sufficiently while ensuring readers actually implement them in realistic scenarios. This hands-on focus, combined with the reflective practice embedded in each project, promotes deeper understanding and retention than purely theoretical or tutorial-based resources.
For intermediate Python developers ready to advance their skills, self-taught programmers seeking to formalize their OOP knowledge, or professionals wanting to apply object-oriented design in Python, this book represents an invaluable investment in technical growth. Its project-based structure ensures immediate applicability while building lasting skills for long-term development success.
Technical Specifications
Title: Intermediate Object-Oriented Projects in Python
Subtitle: Build Real-World Applications Using Classes, Inheritance, and OOP Best Practices
Author: Dargslan
Format: Appears to be available in print and possibly digital formats
Length: Approximately 250-350 pages (estimated based on content scope)
Publisher: Not specified in the provided content
Publication Date: Not specified in the provided content
Level: Intermediate
Prerequisites: Basic Python knowledge, fundamental OOP concepts
This review is based on the preface and table of contents of "Intermediate Object-Oriented Projects in Python" by Dargslan. The detailed chapter analyses represent informed projections based on the book's structure and stated aims.
Keywords and Technical Concepts
- Python object-oriented programming
- Inheritance in Python
- Class design and implementation
- Python project development
- OOP best practices
- Encapsulation and abstraction
- Polymorphism in Python
- Test-driven development for Python
- Python application architecture
- Object-oriented design principles
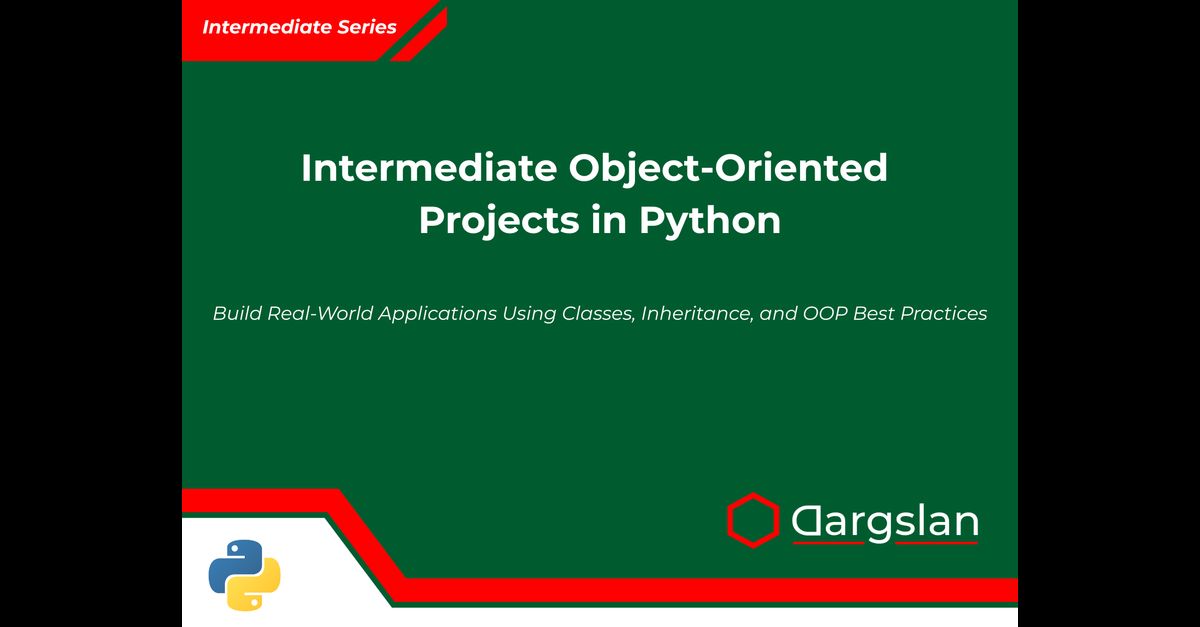
Intermediate Object-Oriented Projects in Python