Book Review: How to Think in Python: Beginner’s Problem-Solving Guide
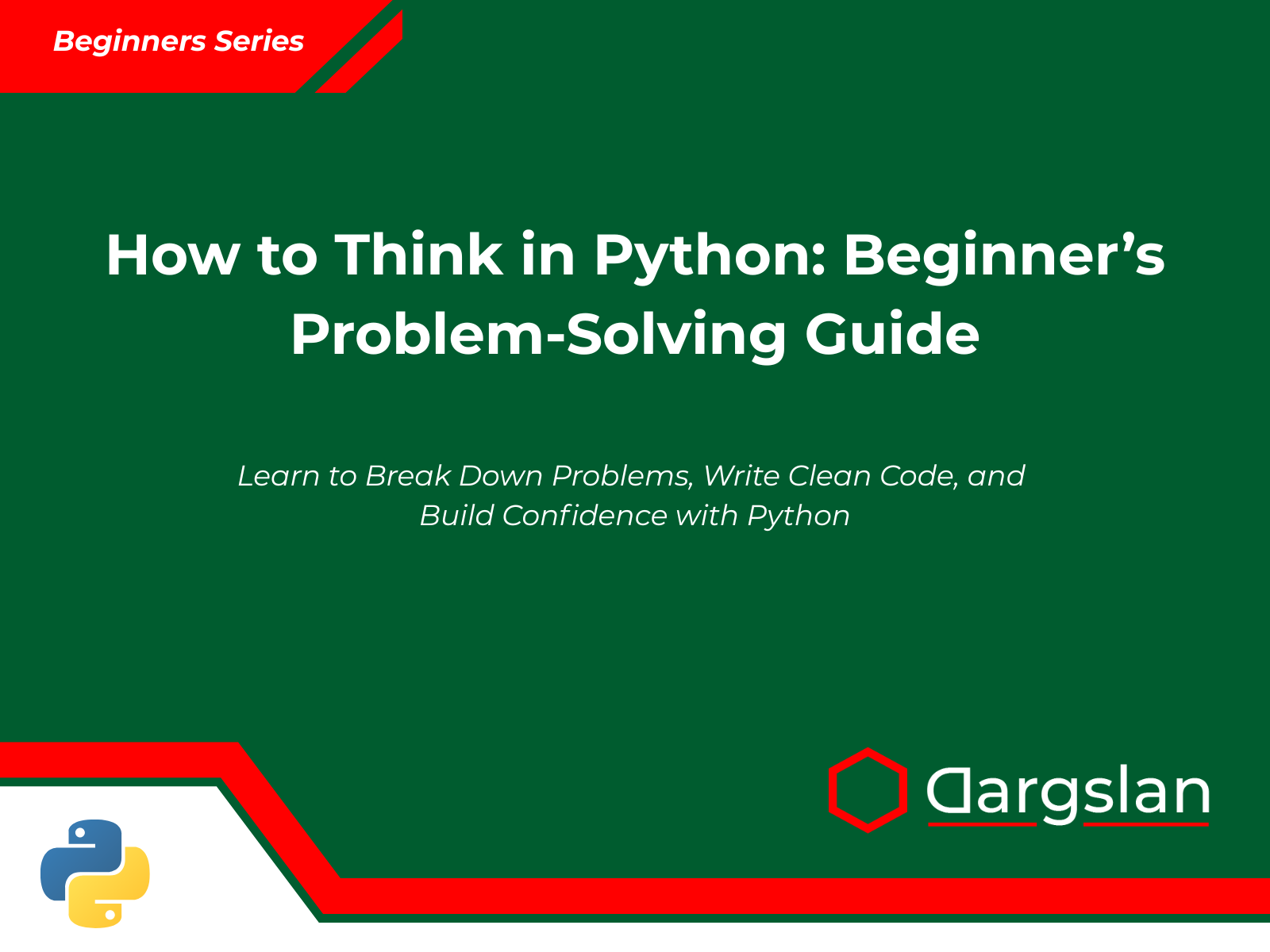
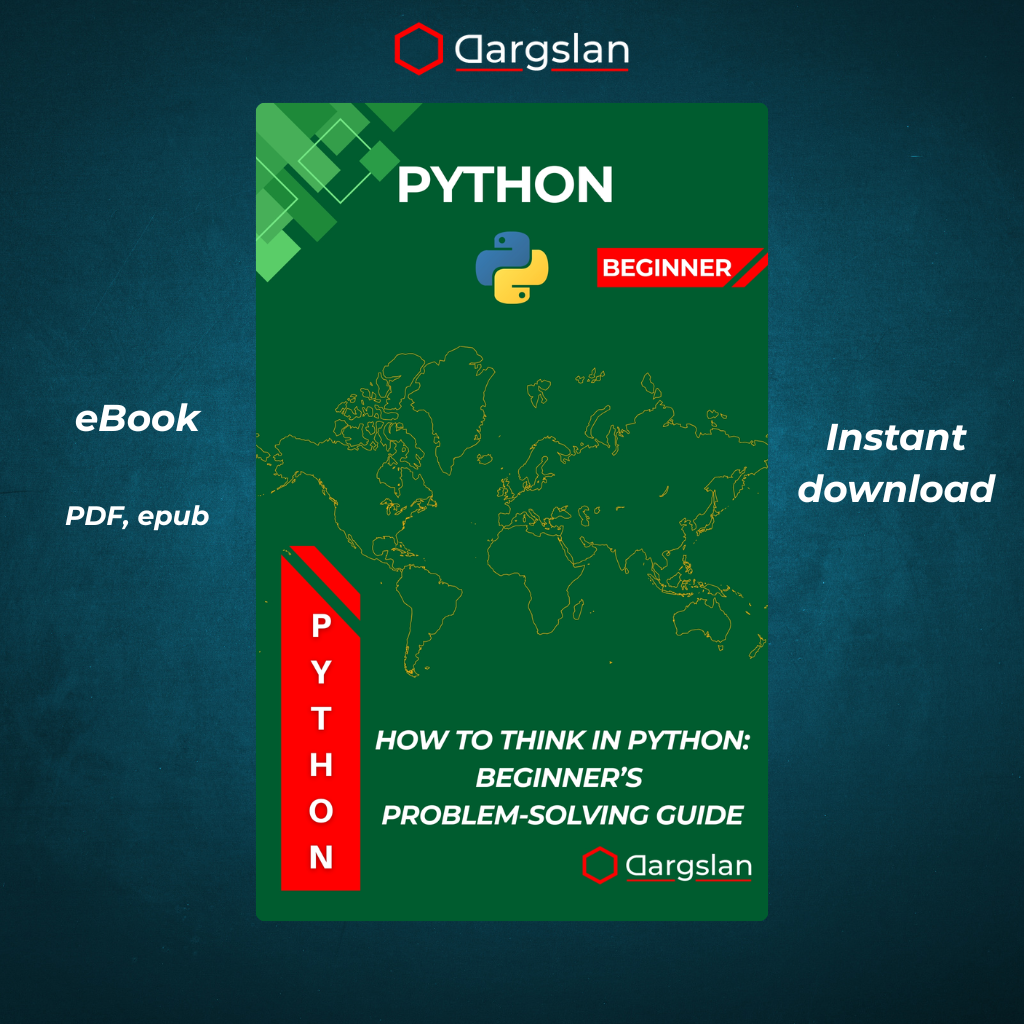
How to Think in Python: Beginner’s Problem-Solving Guide
Learn to Break Down Problems, Write Clean Code, and Build Confidence with Python
Comprehensive Review: "How to Think in Python: Beginner's Problem-Solving Guide"
[Meta Description: Discover how "How to Think in Python" transforms beginners into confident Python programmers through proven problem-solving techniques, practical exercises, and step-by-step guidance.]
Introduction: A Fresh Approach to Learning Python
In the ever-expanding universe of programming books, "How to Think in Python: Beginner's Problem-Solving Guide" by Dargslan stands out as a refreshing departure from conventional coding manuals. Rather than merely teaching syntax and commands, this book focuses on developing the critical thinking skills necessary to become a proficient Python programmer. This comprehensive guide bridges the gap between understanding Python's mechanics and applying that knowledge to solve real-world problems effectively.
As someone who has explored numerous Python resources, I can confidently say this book fills a crucial gap in the learning journey—the development of a "programmer's mindset" specifically tailored to Python's philosophy and capabilities.
Who This Book Is Perfect For
"How to Think in Python" caters specifically to:
- Complete beginners taking their first steps into programming
- Coding students struggling to move beyond basic syntax memorization
- Self-taught programmers looking to solidify their problem-solving approach
- Career changers seeking a structured path to Python proficiency
- Educators wanting a resource that emphasizes thinking processes alongside code
- Hobbyists aiming to build practical Python skills for personal projects
What sets this audience-friendly guide apart is its focus on developing mental models and thinking patterns rather than simply accumulating Python facts.
Key Strengths: Why This Book Succeeds Where Others Fall Short
1. Problem-Solving Focus
Unlike many programming books that present Python as a set of commands to memorize, "How to Think in Python" treats the language as a tool for solving problems. Each concept is introduced within the context of practical problem-solving, helping readers develop the analytical skills necessary for programming success.
2. Progressive Learning Path
The book's ten chapters form a carefully structured learning journey, beginning with fundamental programming concepts and gradually introducing more complex Python features. This scaffolded approach ensures readers build confidence with each new skill before progressing to more challenging material.
3. Practical Application Emphasis
Theory is consistently reinforced with practical exercises, ensuring readers don't just understand concepts but can apply them to solve diverse problems. The inclusion of mini-projects in Chapter 10 provides valuable hands-on experience in combining multiple Python skills.
4. Comprehensive Appendices
The four appendices serve as invaluable references, offering:
- A problem-solving template that readers can apply to any coding challenge
- Common pitfalls and solutions specifically for beginners
- 30 daily practice problems to reinforce learning
- A glossary of essential programming and thinking terminology
Chapter-by-Chapter Breakdown
Chapter 1: Programming Is Problem-Solving
This foundational chapter reframes programming as fundamentally a problem-solving discipline. Readers are introduced to the concept that code is merely the expression of solutions to problems, not the end goal itself. The chapter likely covers:
- The relationship between problems and programming solutions
- How programmers approach breaking down challenges
- The importance of analytical thinking in coding
- Why problem-solving skills transcend specific programming languages
This perspective shift is crucial for beginners who might otherwise focus too narrowly on syntax details while missing the bigger picture of what programming aims to accomplish.
Chapter 2: Python as a Thinking Tool
Building on the problem-solving foundation, this chapter positions Python specifically as an instrument for thought. The author explores:
- Python's philosophy and design principles
- How Python's readability supports clear thinking
- The relationship between Python's syntax and logical reasoning
- Advantages of Python for expressing algorithms and solutions
- Setting up a Python environment optimized for learning
This chapter helps readers understand not just what Python can do, but why its design makes it particularly well-suited for learning to think like a programmer.
Chapter 3: Step-by-Step Thinking – Inputs, Outputs, Process
Here, readers are introduced to a structured approach to problem decomposition. The chapter likely covers:
- Breaking problems into the input-process-output framework
- Identifying what information a program needs (inputs)
- Determining what results a program should produce (outputs)
- Mapping the transformations needed between input and output (process)
- Practical examples of this decomposition technique
This systematic approach gives beginners a repeatable method for tackling programming challenges without feeling overwhelmed.
Chapter 4: Working with Variables and Expressions
This chapter moves into the practical implementation of Python basics, focusing on:
- Understanding variables as containers for data
- Python's data types and their appropriate uses
- Creating meaningful expressions that manipulate data
- Best practices for variable naming and management
- Building expressions that solve concrete problems
Rather than simply listing Python's features, the chapter likely frames these concepts within practical problem contexts, helping readers understand when and why to use particular approaches.
Chapter 5: Decision-Making with if Statements
Conditional logic is introduced as a critical thinking tool. The chapter explores:
- How computers make decisions through boolean logic
- Structuring simple and complex conditional statements
- Creating effective comparison expressions
- Nested conditions and elif statements
- Common logical errors and how to avoid them
- Problem-solving patterns that rely on decision points
By framing conditionals as decision points in solution pathways, readers learn when and how to incorporate branching logic in their problem-solving approach.
Chapter 6: Repetition and Thinking in Loops
This chapter tackles one of the most powerful concepts in programming—iteration. Topics likely include:
- Recognizing problems that require repetitive operations
- Understanding loop structures (for and while loops)
- Loop control flow and common patterns
- Nested loops for complex iterations
- Avoiding infinite loops and other common pitfalls
- Problem-solving techniques using loops
The focus on "thinking in loops" helps readers identify opportunities to apply iteration to simplify solutions and handle repetitive tasks efficiently.
Chapter 7: Breaking Problems into Functions
Function-based decomposition is presented as a key strategy for managing complexity:
- The concept of modular thinking in programming
- Designing functions with single responsibilities
- Parameter passing and return values
- Scope and lifetime of variables
- Building complex solutions from simple functions
- Testing and debugging function-based code
This chapter likely emphasizes how functions enable more organized thinking about complex problems by breaking them into manageable pieces.
Chapter 8: Lists, Dictionaries, and Organizing Data
Data structures are presented as organizational tools for information:
- Choosing appropriate data structures for different problems
- Lists for sequential collections of items
- Dictionaries for key-value relationships
- Common operations and methods for data manipulation
- Nested data structures for complex information
- Problem-solving patterns using Python's data structures
Rather than simply cataloging these features, the chapter likely focuses on the thinking process behind choosing and using data structures effectively.
Chapter 9: Debugging and Thinking Through Errors
Error handling is reframed as an integral part of the problem-solving process:
- Types of errors (syntax, runtime, logical)
- Strategies for systematic debugging
- Reading and interpreting error messages
- Using print statements and debugging tools
- Developing a methodical approach to finding bugs
- Learning from errors to improve future code
This practical chapter equips readers with the resilience and techniques needed when inevitable coding challenges arise.
Chapter 10: Mini Projects to Practice Thinking
The culminating chapter applies all previous concepts to complete projects:
- Integrating multiple Python concepts into coherent programs
- Practicing the full problem-solving workflow
- Building confidence through accomplishment
- Applying the systematic thinking approach to larger challenges
- Reinforcing key concepts through varied applications
These projects likely serve as a capstone experience, helping readers see how individual concepts combine to create useful programs.
The Invaluable Appendices
Appendix A: Problem-Solving Template (I/O → Steps → Code)
This practical template provides a repeatable framework for approaching any programming challenge:
- Identifying and clarifying inputs
- Defining expected outputs
- Breaking down the process into algorithmic steps
- Translating steps into Python code
- Testing and refining the solution
Having this structured approach gives beginners confidence when facing new problems.
Appendix B: Top 10 Mistakes Beginners Make (and How to Fix Them)
This troubleshooting guide addresses common pitfalls such as:
- Misunderstanding variable assignment
- Incorrect indentation
- Loop control errors
- String vs. numeric confusion
- Function scope issues
- List indexing problems
By highlighting these common issues, the appendix helps readers avoid frustrating errors and develop cleaner code more quickly.
Appendix C: 30 Daily Practice Problems
This collection of graduated challenges provides:
- Focused exercises targeting specific Python concepts
- Progression from simple to complex problems
- Opportunities to combine multiple skills
- Practical scenarios that reinforce thinking patterns
- Solutions for self-assessment
These curated problems ensure readers can consolidate their learning through consistent practice.
Appendix D: Glossary of Thinking Terms
This reference section defines essential terminology such as:
- Algorithm
- State
- Edge case
- Abstraction
- Decomposition
- Iteration
- Boolean logic
- Recursive thinking
Understanding these concepts builds a vocabulary for describing and approaching programming challenges.
What Makes This Book Unique
Several factors distinguish "How to Think in Python" from other programming books:
-
Thinking-First Approach: The book prioritizes developing mental models over memorizing syntax.
-
Problem-Oriented Learning: Each concept is introduced through practical problem scenarios.
-
Scaffolded Skill Building: Topics build progressively, with each chapter leveraging previous knowledge.
-
Structured Problem-Solving Framework: The book provides repeatable methodologies rather than isolated techniques.
-
Comprehensive Practice Resources: The appendices offer extensive opportunities for reinforcement and application.
-
Focus on Python's Problem-Solving Strengths: The book highlights how Python's design facilitates specific thinking approaches.
Real-World Applications and Benefits
Readers who master the principles in "How to Think in Python" will be equipped to:
- Automate repetitive tasks in their personal and professional lives
- Analyze data with confidence using Python's powerful libraries
- Build web applications with frameworks like Flask or Django
- Develop tools for specific workplace or educational needs
- Contribute to open-source projects with well-structured code
- Continue learning more advanced Python topics with a solid foundation
Moreover, the thinking skills developed through this book transfer to other programming languages and problem domains, making it an investment in broader computational thinking abilities.
Comparisons to Other Python Books
Unlike "Python Crash Course," which emphasizes project-based learning, "How to Think in Python" focuses more deeply on developing systematic thinking processes.
While "Automate the Boring Stuff with Python" excellently demonstrates practical applications, this book more explicitly teaches the mental framework behind solution development.
"Learn Python the Hard Way" emphasizes disciplined practice, whereas "How to Think in Python" balances practice with conceptual understanding of problem-solving approaches.
Ideal Learning Approach with This Book
To maximize learning with "How to Think in Python," readers should:
- Read actively, attempting to solve presented problems before reviewing solutions
- Practice regularly using the 30 daily problems in Appendix C
- Apply the problem-solving template from Appendix A to challenges outside the book
- Experiment with code by modifying examples and observing results
- Create personal projects that incorporate concepts from multiple chapters
- Review the glossary to internalize key thinking terminology
- Participate in Python communities to discuss approaches with other learners
This active learning strategy aligns with the book's emphasis on developing thinking skills rather than passive knowledge acquisition.
Who Would Benefit Most from This Book
While "How to Think in Python" is valuable for many readers, it's particularly beneficial for:
- Analytical thinkers who want to understand the "why" behind coding approaches
- Visual learners who appreciate seeing problems broken down systematically
- Students preparing for computer science courses or coding bootcamps
- Career changers building a solid foundation for technical roles
- Self-directed learners who prefer structured, methodical skill development
- Educators looking for material that emphasizes computational thinking
The book may be less ideal for readers seeking only quick recipes for specific programming tasks without understanding underlying principles.
Conclusion: A Valuable Investment in Python Proficiency
"How to Think in Python: Beginner's Problem-Solving Guide" represents a significant contribution to Python educational literature. By focusing on the thinking processes that underlie effective programming, it addresses the most challenging aspect of learning to code—developing the mental frameworks necessary to approach problems systematically.
The book's structured approach, comprehensive coverage, and emphasis on practical application make it an excellent resource for beginners serious about building lasting Python skills. Rather than producing programmers who can only replicate examples, this guide aims to develop independent problem-solvers who can confidently tackle new challenges.
For anyone looking to truly understand Python rather than simply use it, "How to Think in Python" provides the thinking tools and practical knowledge necessary for programming success. In a field where problem-solving abilities ultimately matter more than syntax knowledge, this focus on "how to think" represents the book's greatest strength and most enduring value.
Additional Resources for Python Learners
To complement "How to Think in Python," consider these additional learning resources:
- Python.org: The official Python documentation and tutorials
- Real Python: In-depth articles and tutorials on Python concepts
- Codecademy's Python Course: Interactive exercises to reinforce learning
- PyCharm Educational Edition: An IDE with built-in learning features
- Python Discord Community: A supportive forum for Python learners
- Project Euler: Mathematical problems that can be solved with Python
- LeetCode: Coding challenges to practice problem-solving skills
These resources can provide additional practice and community support as you apply the thinking approaches learned in the book.
This comprehensive review covers the structure, approach, and benefits of "How to Think in Python: Beginner's Problem-Solving Guide." If you're serious about developing not just Python skills but the problem-solving mindset needed for programming success, this book deserves a place in your learning library.
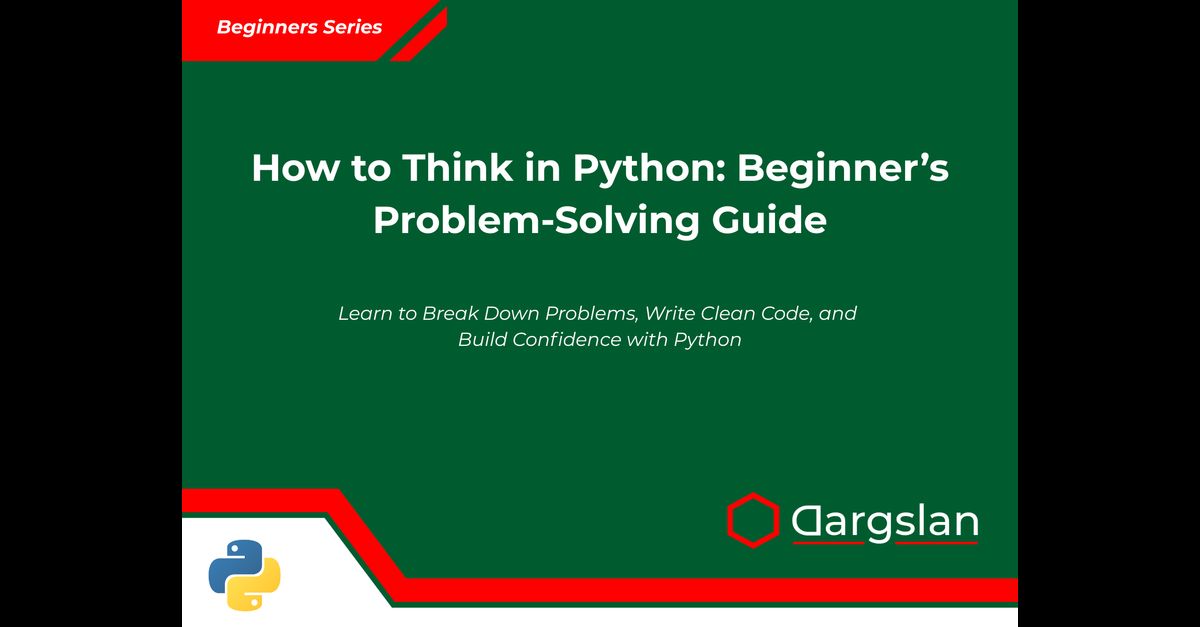
How to Think in Python: Beginner’s Problem-Solving Guide