Book Review: Getting Started with Python Lists and Tuples
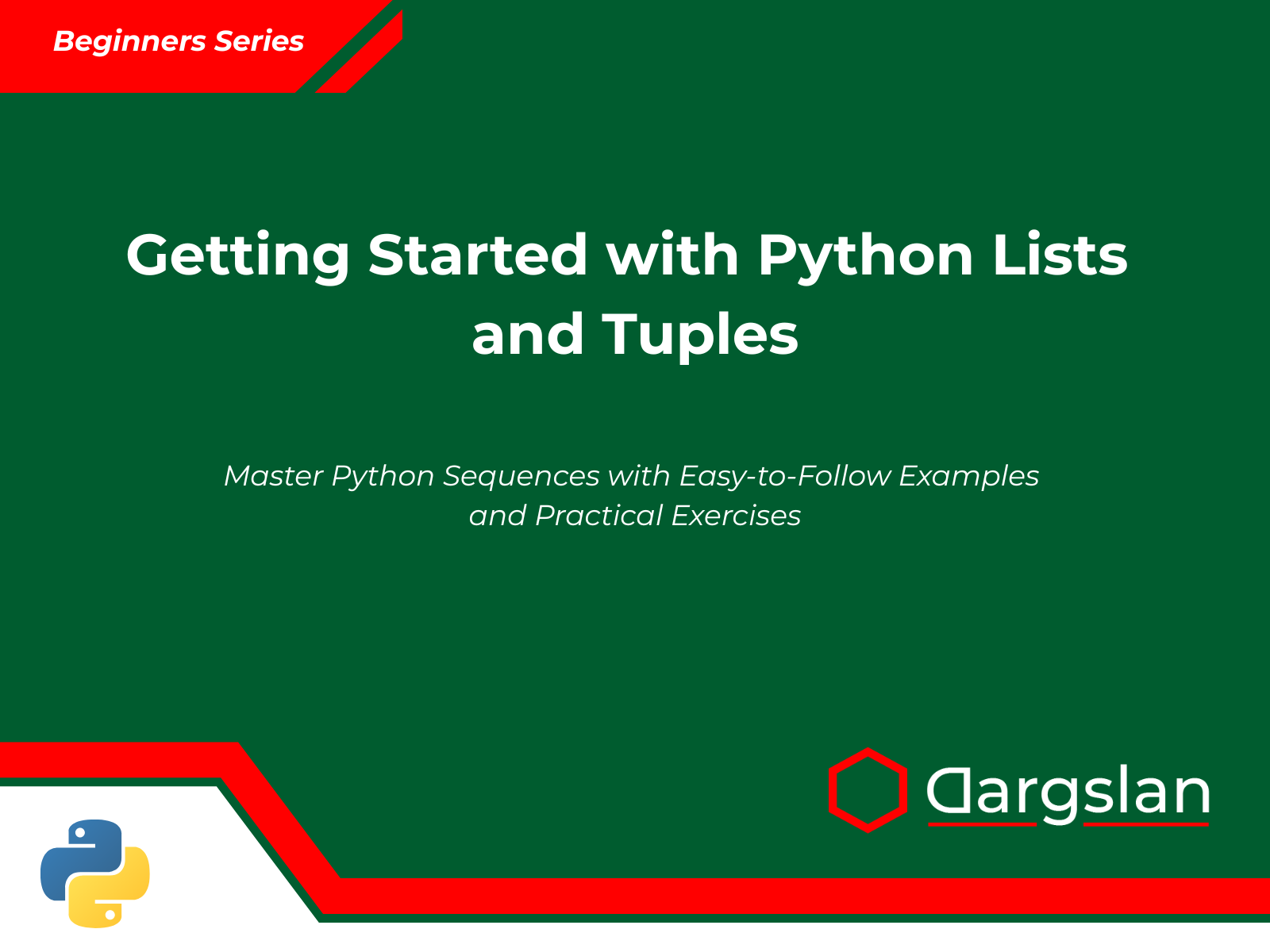
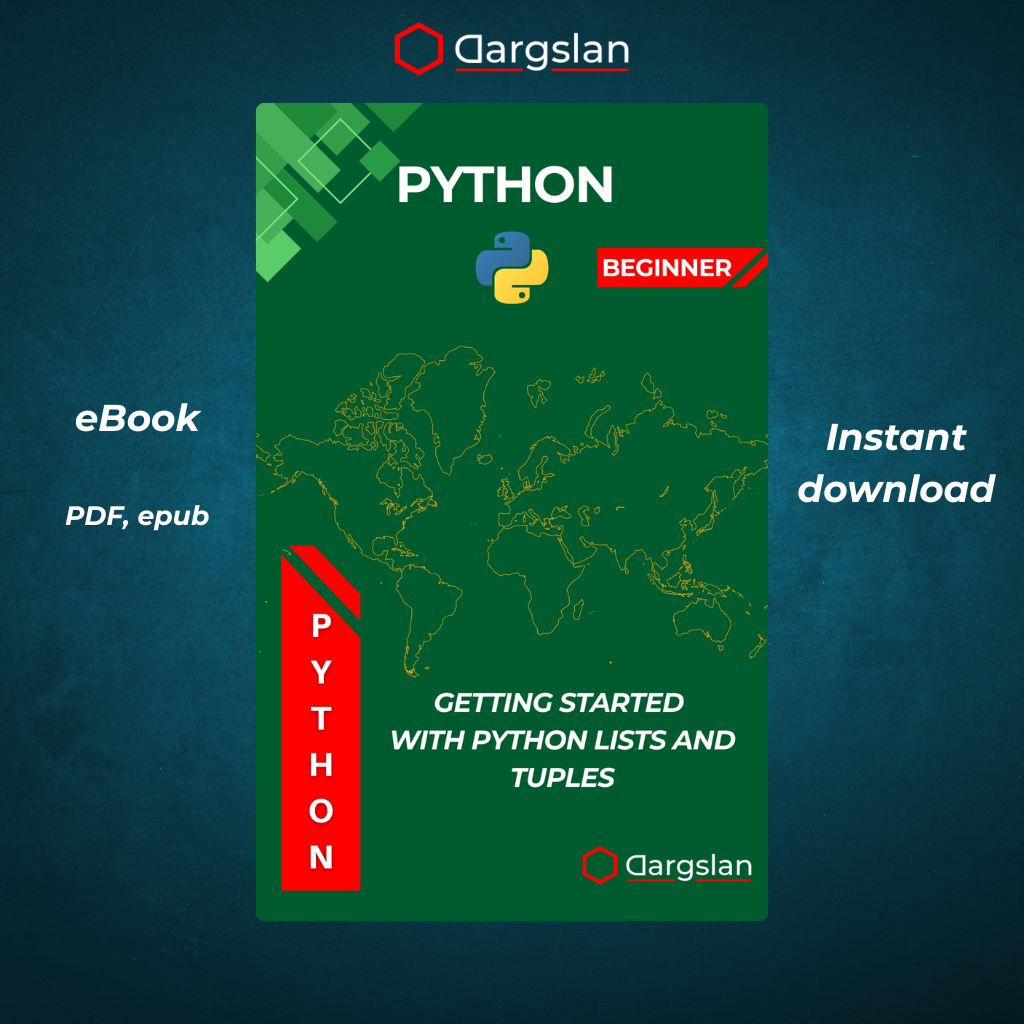
Getting Started with Python Lists and Tuples
Master Python Sequences with Easy-to-Follow Examples and Practical Exercises
Book Review: "Getting Started with Python Lists and Tuples"
A Comprehensive Guide to Essential Python Data Structures
"Getting Started with Python Lists and Tuples: Master Python Sequences with Easy-to-Follow Examples and Practical Exercises" by Dargslan offers a focused, in-depth exploration of two fundamental Python data structures that form the backbone of effective Python programming. This specialized resource fills an important gap in the Python learning ecosystem by providing comprehensive coverage of sequence types that most general Python books only touch upon briefly.
Why This Book Matters in the Python Learning Landscape
Python's popularity continues to grow exponentially across diverse domains including web development, data science, machine learning, and automation. At the core of nearly every Python application lie lists and tuples—versatile sequence types that store collections of items and enable countless programming patterns. Mastering these structures is essential for anyone serious about Python development, yet surprisingly few resources provide the depth of coverage these fundamental concepts deserve.
This book stands out by dedicating its entire focus to these critical data structures, allowing readers to develop a level of mastery that's difficult to achieve through more general Python texts or fragmented online tutorials. Rather than treating lists and tuples as just another topic in a broad curriculum, Dargslan explores their nuances, capabilities, and best practices in comprehensive detail.
Structure and Content: A Progressive Learning Journey
The book's ten chapters form a carefully designed learning progression that builds knowledge systematically from basic concepts to advanced applications:
Chapter 1: Introduction to Python Sequences
The journey begins with a solid theoretical foundation, introducing sequences as a core Python concept. This chapter establishes the essential characteristics of sequence types and explains how lists and tuples fit within Python's broader type system. Rather than diving immediately into syntax, the author takes time to explain why sequence types matter and how they reflect Python's design philosophy.
This approach helps readers understand not just how to use these data structures, but why they exist in the form they do. For beginners, this context provides crucial background knowledge. For experienced developers from other languages, it clarifies Python's unique approach to sequence handling.
Chapter 2: Creating and Accessing Lists
Chapter 2 transitions to practical implementation, focusing on list creation and element access. The coverage of list creation methods is comprehensive, including literal syntax, list comprehensions, and conversion from other types:
# Different ways to create lists
empty_list = []
simple_list = [1, 2, 3, 4, 5]
from_range = list(range(1, 6)) # [1, 2, 3, 4, 5]
comprehension = [x**2 for x in range(1, 6)] # [1, 4, 9, 16, 25]
The explanation of indexing and slicing is particularly strong, covering both basic and advanced usage with clear examples:
fruits = ["apple", "banana", "cherry", "date", "elderberry"]
# Basic indexing
first = fruits[0] # "apple"
last = fruits[-1] # "elderberry"
# Slicing
first_three = fruits[0:3] # ["apple", "banana", "cherry"]
every_other = fruits[::2] # ["apple", "cherry", "elderberry"]
reversed_list = fruits[::-1] # ["elderberry", "date", "cherry", "banana", "apple"]
What sets this chapter apart is the attention to practical applications and common patterns, showing readers not just the mechanics but the real-world utility of these operations.
Chapter 3: Modifying Lists
The third chapter explores list mutability—one of the key characteristics distinguishing lists from tuples. The coverage of modification methods is meticulous, clarifying subtle distinctions that often confuse beginners:
# Different ways to add elements
fruits = ["apple", "banana"]
fruits.append("cherry") # Adds single element: ["apple", "banana", "cherry"]
fruits.extend(["date", "fig"]) # Adds multiple elements: ["apple", "banana", "cherry", "date", "fig"]
fruits.insert(1, "apricot") # Inserts at specific position: ["apple", "apricot", "banana", "cherry", "date", "fig"]
# Different ways to remove elements
removed = fruits.pop() # Removes and returns last element: "fig"
fruits.remove("cherry") # Removes specific value: ["apple", "apricot", "banana", "date"]
del fruits[0] # Removes by index: ["apricot", "banana", "date"]
A particularly valuable section addresses the concept of reference versus copy, explaining how list assignments create references to the same underlying data—a common source of bugs for Python newcomers:
# List assignment creates a reference, not a copy
original = [1, 2, 3]
reference = original # Both variables point to the same list
reference.append(4)
print(original) # [1, 2, 3, 4] - original is also modified
# Creating actual copies
shallow_copy = original.copy() # or list(original) or original[:]
This chapter effectively combines syntactic knowledge with conceptual understanding, helping readers avoid common pitfalls while developing good programming practices.
Chapter 4: Working with List Methods
Chapter 4 provides an exhaustive tour of Python's built-in list methods. The coverage of sorting techniques is especially thorough, demonstrating not just basic sorts but also key-based sorting for complex scenarios:
# Basic sorting
numbers = [3, 1, 4, 1, 5, 9, 2]
numbers.sort() # [1, 1, 2, 3, 4, 5, 9]
# Reverse sorting
words = ["apple", "banana", "cherry"]
words.sort(reverse=True) # ["cherry", "banana", "apple"]
# Key-based sorting
names = ["Alice", "bob", "Charlie", "david"]
names.sort(key=str.lower) # Case-insensitive: ["Alice", "bob", "Charlie", "david"]
# Sorting complex objects
students = [
{"name": "Alice", "grade": 85},
{"name": "Bob", "grade": 92},
{"name": "Charlie", "grade": 78}
]
students.sort(key=lambda student: student["grade"], reverse=True)
The author effectively distinguishes between similar methods (like sort()
vs. sorted()
) and demonstrates how to chain methods for complex operations. This comprehensive treatment ensures readers develop not just basic competency but genuine fluency with list manipulation.
Chapter 5: Introduction to Tuples
Chapter 5 shifts focus to tuples, Python's immutable sequence type. The explanation of tuple creation clarifies the sometimes confusing syntax, particularly for single-element tuples:
# Creating tuples
empty = ()
single = (42,) # Note the comma - critical for single-element tuples
multiple = (1, 2, 3)
no_parentheses = 4, 5, 6 # Parentheses are optional in many contexts
The coverage of tuple unpacking is excellent, showcasing this powerful Python feature that enables elegant, readable code:
# Basic unpacking
point = (10, 20)
x, y = point # x = 10, y = 20
# Multiple assignment
a, b, c = (1, 2, 3) # a = 1, b = 2, c = 3
# Swapping variables
a, b = b, a # Values are swapped
# Extended unpacking (Python 3.x)
first, *middle, last = (1, 2, 3, 4, 5) # first = 1, middle = [2, 3, 4], last = 5
The chapter effectively explains tuple immutability, emphasizing not just the constraints but the benefits this characteristic provides in terms of safety, hashability, and semantic clarity.
Chapter 6: Tuple Use Cases and Tricks
Building on the basics, Chapter 6 explores practical applications of tuples in real-world Python programming. The section on using tuples as dictionary keys is particularly informative:
# Using tuples as dictionary keys
locations = {
(40.7128, -74.0060): "New York City",
(34.0522, -118.2437): "Los Angeles",
(51.5074, -0.1278): "London"
}
# Looking up a location
print(locations[(40.7128, -74.0060)]) # "New York City"
The coverage of named tuples is excellent, showing how they bridge the gap between simple tuples and full-fledged classes:
from collections import namedtuple
# Creating a named tuple type
Person = namedtuple('Person', ['name', 'age', 'job'])
# Creating instances
alice = Person('Alice', 30, 'Engineer')
# Accessing by name or position
print(alice.name) # 'Alice'
print(alice[0]) # 'Alice'
# Unpacking still works
name, age, job = alice
These practical examples demonstrate how tuples enable elegant, efficient code solutions in various programming contexts.
Chapter 7: Lists vs Tuples in Practice
Chapter 7 offers comparative analysis to help readers make informed choices between lists and tuples. Instead of simplistic rules, the author presents nuanced guidance based on various considerations:
- Mutability requirements: Does your data need to change after creation?
- Performance characteristics: Which operations are most frequent in your use case?
- Memory usage: Are you working with large datasets where efficiency matters?
- Semantic intent: What does your choice communicate to other developers?
- Integration with other code: How will your choice interact with existing systems?
Practical examples demonstrate how these factors influence design decisions in real projects. This approach helps readers develop good judgment rather than memorizing rigid rules.
Chapter 8: Working with Nested Sequences
Chapter 8 tackles the complex topic of nested sequences—lists within lists, tuples within tuples, and mixed nesting. This advanced topic is presented with remarkable clarity through well-designed examples:
# Creating a 3x3 matrix
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
# Accessing elements
element = matrix[1][2] # 6 (row 1, column 2)
# Processing all elements
for row in matrix:
for element in row:
print(element, end=" ")
print() # New line after each row
# List comprehension with nested loops
flattened = [element for row in matrix for element in row]
The chapter addresses the challenges of copying nested structures, explaining shallow versus deep copying with concrete examples:
import copy
# Original nested list
original = [[1, 2], [3, 4]]
# Shallow copy - inner lists are still shared!
shallow = original.copy()
shallow[0][0] = 99
print(original[0][0]) # 99 - original is affected!
# Deep copy - complete independence
deep = copy.deepcopy(original)
deep[0][0] = 42
print(original[0][0]) # Still 99 - original is unaffected
Through clear diagrams and well-crafted examples, the author demystifies these complex structures, making them accessible to readers of all experience levels.
Chapter 9: Common Pitfalls and How to Avoid Them
Chapter 9 addresses frequent mistakes and misconceptions related to Python sequences. The section on mutable default arguments is particularly valuable, explaining a notorious Python gotcha:
# Dangerous: Using a mutable default argument
def add_item(item, items=[]): # This creates problems!
items.append(item)
return items
print(add_item("apple")) # ["apple"]
print(add_item("banana")) # ["apple", "banana"] - Surprise!
# Better approach
def add_item_fixed(item, items=None):
if items is None:
items = []
items.append(item)
return items
Other important topics include:
- Avoiding modification during iteration
- Understanding identity vs. equality (
is
vs.==
) - Preventing unintended side effects with mutable sequence elements
- Optimizing sequence operations for performance
This troubleshooting guide helps readers avoid common traps that might otherwise cause considerable frustration.
Chapter 10: Practice Projects and Challenges
The final chapter provides hands-on projects that integrate multiple concepts from throughout the book. These range from beginner-friendly exercises to challenging projects that push readers to apply their knowledge creatively.
The projects include:
- A task management application using lists to store and organize tasks
- A data analysis toolkit that processes nested data structures
- A simple game implementation using tuples for coordinates
- Performance optimization challenges that test efficiency understanding
Each project includes detailed requirements, starter code, and comprehensive solutions with explanations. This applied component transforms theoretical knowledge into practical skills, reinforcing learning through hands-on experience.
Supplementary Materials: Valuable References
The book's appendices provide excellent quick-reference materials:
- List and Tuple Methods Summary Table: A comprehensive reference of all methods with syntax, return values, and example usage
- Built-in Functions Useful with Sequences: Coverage of Python's built-in functions that work particularly well with sequence types
- Practice Exercises with Answers: Additional problems to reinforce learning
- Mini Quiz: Self-assessment questions to test understanding
These materials transform the book from a one-time read into an ongoing reference resource that remains valuable long after the initial learning process.
Pedagogical Strengths
The book's instructional approach incorporates several effective pedagogical strategies:
Progressive Skill Building
Concepts build systematically upon one another, creating a smooth learning curve that takes readers from basics to advanced applications without overwhelming jumps in complexity.
Practical Code Examples
Every concept is illustrated with clear, runnable code examples that demonstrate not just syntax but real-world application. These examples progress from simple illustrations to more complex, realistic scenarios.
Visual Learning Support
Well-designed diagrams and visual elements help clarify abstract concepts, particularly for topics like slicing, nested structures, and memory models that benefit from visual representation.
Active Learning Opportunities
Frequent exercises prompt readers to apply concepts immediately after learning them, reinforcing understanding through practice rather than passive reading.
Problem-Solving Focus
Rather than presenting Python features in isolation, the book consistently frames them as tools for solving specific programming problems, helping readers develop solution-oriented thinking.
Who Will Benefit from This Book
This book serves diverse audiences at different stages of their Python journey:
For Python Beginners (Beyond Absolute Basics)
Those who have grasped Python's basic syntax will find this book an excellent next step, providing deep understanding of fundamental data structures that form the foundation for further learning.
For Intermediate Python Developers
Programmers with some Python experience will discover nuances, best practices, and optimization techniques they may have missed, helping them write more elegant and efficient code.
For Developers from Other Languages
Experienced programmers adding Python to their toolkit will appreciate the focused approach that helps them quickly master Python's distinctive sequence handling without wading through introductory material.
For Computer Science Students
The book's detailed coverage of data structures complements theoretical computer science education with practical Python implementations and real-world usage patterns.
For Technical Interviewees
Those preparing for Python-related job interviews will find comprehensive coverage of sequence manipulation topics that frequently appear in technical assessments.
Comparison with Other Python Resources
When compared to other learning materials, this book offers distinctive advantages:
General Python Books
Unlike comprehensive Python texts that cover dozens of topics with limited depth, this book's focused approach allows for genuine mastery of critical data structures that underpin virtually all Python programming.
Online Tutorials
In contrast to fragmented online content that often lacks cohesion, this book presents a systematic learning path with consistent terminology, progressive skill building, and integrated examples.
Python Documentation
While Python's official documentation is comprehensive, it primarily serves as reference rather than instruction. This book bridges that gap, providing learning-focused guidance that builds practical skills.
Computer Science Textbooks
Unlike theoretical data structures texts, this book grounds concepts in practical Python implementation, showing not just how structures work conceptually but how to use them effectively in real code.
SEO-Relevant Aspects
For those specifically interested in Python data structures, this book addresses numerous key topics that developers frequently search for:
- Comprehensive coverage of Python list methods and operations
- Detailed explanation of list comprehensions and their applications
- Clear guidance on when to use lists vs. tuples
- In-depth coverage of tuple unpacking techniques
- Practical approaches to nested data structures
- Performance considerations for sequence operations
- Best practices for Python sequence manipulation
These focused topics make the book particularly valuable for readers seeking to strengthen specific Python skills that are in high demand across the industry.
Real-World Applications
The knowledge presented in this book has immediate practical applications across various domains:
Web Development
Lists and tuples are essential for handling data in web frameworks like Django and Flask, from processing form submissions to organizing database query results.
Data Analysis
Before reaching for specialized libraries like NumPy or Pandas, strong fundamentals in Python's native data structures enable cleaner, more efficient data processing code.
Automation and Scripting
System automation tasks frequently involve collecting and processing items—skills that directly leverage the sequence handling techniques covered in this book.
API Integration
Working with JSON data from web APIs requires effective sequence manipulation to extract, transform, and organize information.
Game Development
Game state, coordinates, inventories, and other gaming elements are frequently represented using lists and tuples, making these skills directly applicable to game programming.
Conclusion: A Worthy Investment for Python Programmers
"Getting Started with Python Lists and Tuples" stands as an essential resource for anyone serious about Python programming. By focusing exclusively on critical sequence types rather than attempting to cover the entire language, the book achieves a depth of coverage that transforms fundamental understanding into practical mastery.
The progressive structure, practical orientation, and comprehensive examples make this book accessible to beginners while still valuable for experienced developers. The emphasis on best practices and common pitfalls helps readers avoid mistakes that might otherwise cause frustration and wasted time.
In a field often characterized by rapid change, mastery of fundamental data structures remains evergreen. This book delivers that mastery in an accessible, engaging, and thorough package that will serve readers throughout their Python programming journey.
Whether you're building web applications, analyzing data, automating tasks, or developing software, the skills presented in this book will enhance your programming capabilities and enable you to write cleaner, more efficient, and more Pythonic code. For anyone looking to elevate their Python skills, this focused guide represents an excellent investment of time and attention.
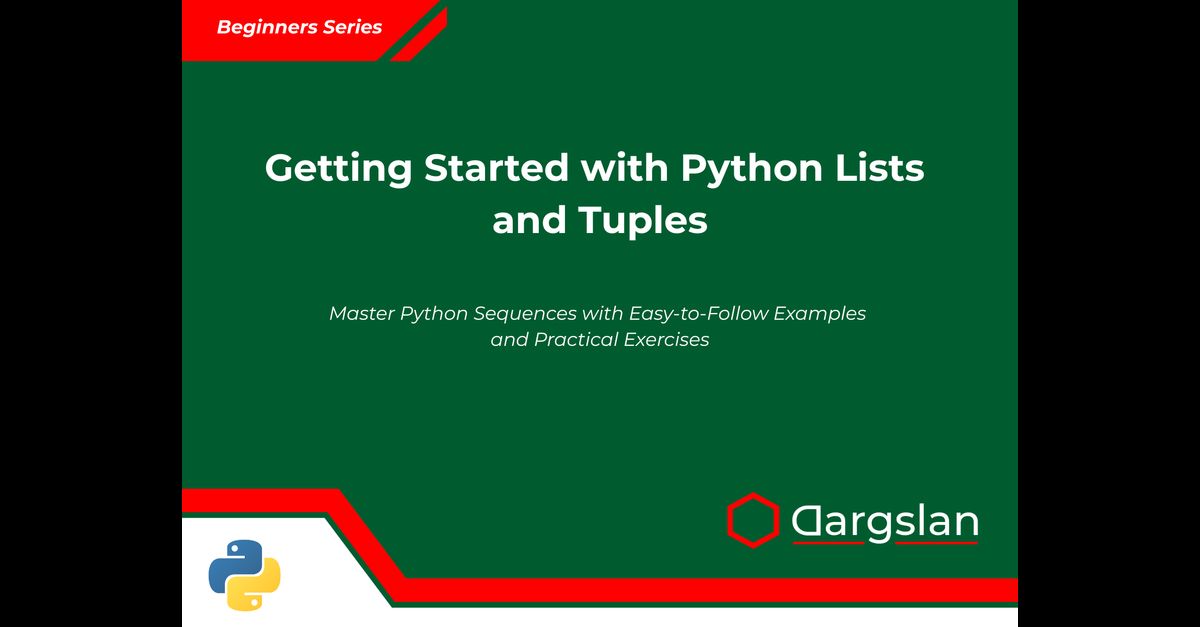
Getting Started with Python Lists and Tuples