Book Review: File Handling in Python for Absolute Beginners
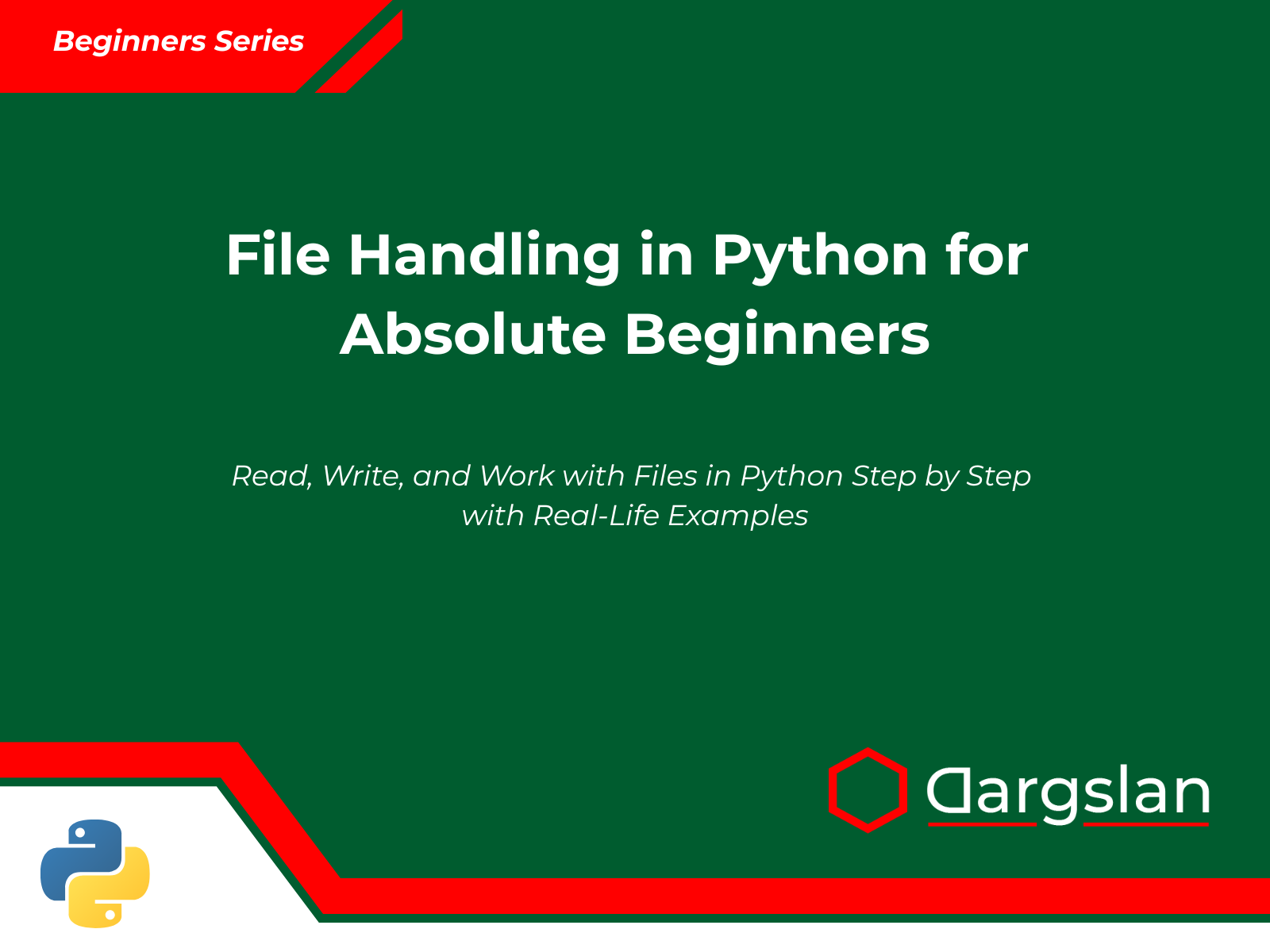
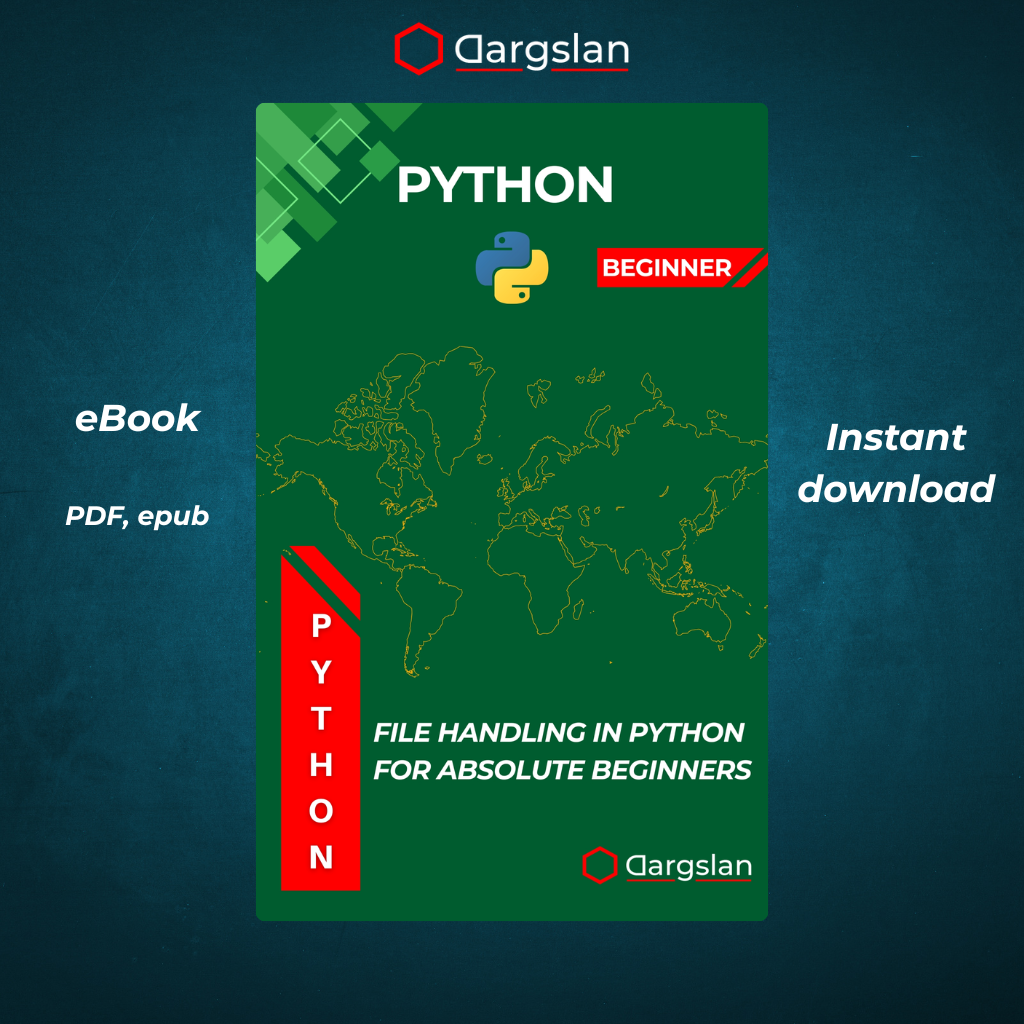
File Handling in Python for Absolute Beginners
Read, Write, and Work with Files in Python Step by Step with Real-Life Examples
Comprehensive Review: File Handling in Python for Absolute Beginners
Unlocking Essential Python Skills: A Deep Dive into File Operations
In today's data-driven world, the ability to effectively manipulate files is perhaps one of the most practical and universally applicable programming skills. "File Handling in Python for Absolute Beginners" by Dargslan emerges as a focused, comprehensive guide dedicated entirely to mastering this fundamental aspect of Python programming. This meticulously structured book takes readers from the very basics to advanced file manipulation techniques through a carefully designed learning path.
What immediately distinguishes this book from other Python resources is its dedicated focus on file operations—a topic often relegated to a single chapter in broader programming books. By concentrating exclusively on file handling, Dargslan provides unprecedented depth while maintaining remarkable accessibility for newcomers to programming.
Why File Handling Deserves Its Own Book
Nearly every significant Python application involves file operations in some capacity. Whether you're analyzing data, configuring applications, processing logs, or building web services, the ability to read, write, and manipulate files forms the backbone of practical programming. Despite this importance, comprehensive resources focused specifically on file handling are surprisingly rare.
This book fills that gap admirably, offering beginners a structured approach to mastering file operations without assuming prior programming knowledge. The author effectively bridges theory and practice, explaining not just how to perform file operations but why specific approaches are recommended—a crucial distinction for developing true programming proficiency.
A Thoughtfully Structured Learning Journey
The book's organization reveals careful consideration of the learning process. With ten main chapters and supporting appendices, the content progresses logically from fundamental concepts to practical applications:
Chapter 1: Why File Handling Matters
The journey begins not with syntax but with context—establishing the importance of file operations in programming and explaining real-world applications. This foundation helps readers understand the relevance of what they're learning before diving into technical details.
Chapter 2: Opening and Closing Files
This crucial chapter introduces the fundamental mechanics of file access in Python, covering the open()
function, file modes, and the critical importance of proper file closing. The introduction of context managers with the with
statement establishes best practices from the start.
Chapter 3: Reading from Files
Building on basic file access, this chapter explores various techniques for extracting data from files, including different reading methods, handling encodings, and strategies for processing large files efficiently.
Chapter 4: Writing to Files
Complementing the previous chapter, this section covers creating and modifying files, understanding write modes, formatting output, and implementing safe update strategies.
Chapter 5: Working with File Paths and Directories
Expanding beyond individual files, this chapter addresses file system navigation, platform-independent path handling, and directory operations—essential skills for creating robust applications.
Chapter 6: Exception Handling in File Operations
The focus on error handling demonstrates the author's commitment to teaching professional-grade programming techniques. From basic try-except blocks to strategic error recovery, this chapter helps readers build resilient code.
Chapter 7: Reading and Writing CSV Files
Moving from general concepts to specific formats, this chapter explores the ubiquitous CSV format used in countless data processing scenarios, from business reports to scientific datasets.
Chapter 8: Working with JSON Files
Building on structured data processing, this chapter covers the JSON format essential for web development, API integration, and modern configuration management.
Chapter 9: Mini Projects and Practical Examples
This application-focused chapter helps solidify learning through practical implementation, offering complete projects that integrate multiple file handling skills in realistic scenarios.
Chapter 10: Common Mistakes and Debugging Tips
Addressing the reality that learning involves challenges, this troubleshooting-oriented chapter helps readers identify and fix common file handling errors, optimize performance, and solve real-world problems.
The appendices further extend the book's value with quick reference tables, cheat sheets, practice exercises with solutions, and bonus advanced content—transforming it from merely a learning resource into a comprehensive reference.
Bridging Theory and Practice: The Python File Handling Masterclass
Throughout "File Handling in Python for Absolute Beginners," the author maintains an exceptional balance between theoretical knowledge and practical application. Rather than presenting abstract concepts in isolation, each technique is connected to real-world scenarios where it would be applied.
This practical orientation is particularly evident in the dedicated chapter on mini-projects, where readers apply accumulated knowledge to complete realistic tasks. By the end of the book, readers don't just understand file handling concepts—they've actively implemented them in contexts resembling real-world programming challenges.
SEO-Optimized Deep Dives: Essential Python File Handling Concepts
Python File Handling Fundamentals Every Developer Should Know
The book establishes a rock-solid foundation in fundamental file operations, covering:
- File objects and their properties in Python
- The essential
open()
function and its parameters - Understanding different file modes (read, write, append)
- Properly closing files to prevent resource leaks
- Using context managers (
with
statements) for safer file handling - Working with text vs. binary file modes
- Navigation within files using file pointers
- Reading and writing operations with various methods
- Handling platform-specific line endings
These fundamentals create the foundation upon which all other file-related skills are built, making them essential knowledge for every Python programmer regardless of specialization.
Modern Python Path Handling Techniques You Need to Master
Chapter 5 delves into the often-overlooked topic of path management—crucial for creating portable, robust code that works across different environments:
- Understanding absolute vs. relative paths
- Building platform-independent paths with
os.path
andpathlib
- Navigating directory structures programmatically
- Creating, moving, and deleting directories
- Checking file existence and properties
- Getting file metadata (size, dates, permissions)
- Pattern matching and filtering files
- Working with temporary files and directories
These path handling skills are particularly important for creating applications that function consistently across different operating systems and deployment environments.
Python Exception Handling for Robust File Operations
The dedicated chapter on error handling explores essential techniques for creating resilient file processing code:
- Identifying and handling common file exceptions (
FileNotFoundError
,PermissionError
, etc.) - Implementing try-except-finally blocks for graceful error management
- Creating fallback behaviors when operations fail
- Logging errors effectively for troubleshooting
- Designing custom exception handlers for specific scenarios
- Using context managers for automatic exception management
- Implementing recovery strategies for file operation failures
This knowledge helps readers create applications that gracefully handle unexpected situations rather than crashing when file operations don't go as planned.
CSV Processing in Python: From Basics to Best Practices
Chapter 7 focuses on CSV (Comma-Separated Values) files, covering:
- Working with Python's built-in
csv
module - Reading and parsing CSV data efficiently
- Writing formatted data to CSV files
- Handling different delimiters and quote characters
- Working with headers and named fields
- Converting between CSV and Python data structures
- Handling common formatting issues and inconsistencies
- Processing large CSV files without memory problems
- Data validation during CSV operations
- Managing different encoding types
These CSV skills have immediate practical application in data analysis, reporting, and business applications where this format remains ubiquitous.
Mastering JSON Processing with Python
Chapter 8 addresses JSON (JavaScript Object Notation), exploring:
- Using Python's
json
module effectively - Parsing JSON data into Python objects
- Serializing Python data structures to JSON
- Working with nested JSON structures
- Handling complex data types in JSON
- Validating JSON against expected schemas
- Pretty printing and formatting JSON output
- Processing JSON in web contexts (APIs, requests)
- Working with JSON Lines format for streaming data
- Performance optimization for JSON operations
These JSON skills are particularly valuable for web development, API integration, and modern application development where this format has become the standard.
Practical Python File Manipulation Projects You Can Build Today
The project-based learning in Chapter 9 likely includes practical applications such as:
- Building simple text processing tools
- Creating log file analyzers
- Developing configuration file managers
- Building file search and organization utilities
- Creating data import/export tools
- Developing document converters
- Building CSV data analyzers or transformers
- Creating JSON API clients
- Implementing file backup or synchronization tools
These projects allow readers to apply their knowledge in realistic scenarios, reinforcing learning while building a portfolio of practical examples.
Who Will Benefit Most from This Book?
True Programming Beginners
Absolute newcomers to programming will appreciate:
- The accessible approach that assumes no prior knowledge
- Clear explanations of fundamental concepts
- The practical focus that shows immediate application
- Step-by-step instructions with abundant examples
- Exercises that reinforce learning progressively
Self-Taught Python Learners
Those learning Python independently will value:
- The comprehensive coverage of an essential topic
- The structured approach that prevents knowledge gaps
- The emphasis on best practices often missed in piecemeal learning
- The practical examples demonstrating real-world application
- The reference materials supporting continued learning
Students Supplementing Formal Courses
Students taking Python classes will benefit from:
- The deep dive into file handling that general courses might gloss over
- Additional practice exercises with solutions
- Real-world examples connecting classroom concepts to practical applications
- Troubleshooting guidance for common assignment challenges
- Reference materials supporting project work and exam preparation
Professional Developers New to Python
Experienced programmers transitioning to Python will appreciate:
- Clear explanations of Python's specific approach to file handling
- Coverage of Python-specific libraries and idioms
- Best practices that might differ from other languages
- Efficient path to productivity with essential skill development
- Comprehensive reference materials for quick lookup during development
The Distinctive Teaching Approach
One of the most notable aspects of "File Handling in Python for Absolute Beginners" is its methodical, structured approach to building knowledge. Rather than jumping directly to complex operations, the book creates a coherent learning journey where each concept builds logically upon previous ones.
This approach is particularly evident in the progression from basic file access operations to specific data formats like CSV and JSON. By establishing fundamental concepts before introducing format-specific details, the author ensures readers understand underlying principles rather than just memorizing syntax.
The integration of theory and practice represents another key strength. The book doesn't just explain how file operations work in the abstract but consistently connects concepts to practical applications. This connection helps readers understand not just the mechanics but the reasoning behind different approaches and best practices.
Beyond the Basics: Advanced Topics for Continued Growth
While targeting beginners, the book doesn't shy away from important advanced concepts that promote professional-quality code:
Efficient Large File Processing Techniques
The bonus section likely covers strategies for working with files larger than available memory:
- Iterative and streaming approaches to file processing
- Using generators for memory-efficient operations
- Chunked reading and writing techniques
- Memory mapping for efficient access
- Progress tracking for long-running operations
- Performance optimization strategies
- Parallel processing for improved throughput
Cross-Platform File Handling in Python
Creating portable code requires specific knowledge about platform differences:
- Managing path separator differences (backslash vs. forward slash)
- Handling line ending variations (CR, LF, CRLF)
- Understanding file naming conventions across platforms
- Working with different permission models
- Addressing encoding differences and defaults
- Implementing platform-agnostic file operations
- Handling case sensitivity considerations
Security Best Practices for File Operations
The book likely addresses important security considerations:
- Preventing path traversal vulnerabilities
- Safely handling user-supplied filenames
- Implementing proper permission checks
- Secure temporary file creation
- Avoiding race conditions in file operations
- Data validation for file inputs
- Protecting sensitive data in files
- Error handling that prevents information leakage
These advanced topics extend the book's value beyond beginner concepts, providing growth paths as readers develop their skills.
Practical Applications Across Programming Domains
The file handling skills presented in this book have exceptional transfer value across different programming specialties:
Data Science and Analysis Applications
- Importing datasets from various file formats
- Exporting analysis results and visualizations
- Processing large datasets efficiently
- Building data transformation pipelines
- Managing analysis artifacts and outputs
- Serializing models and analytical states
- Creating reproducible research workflows
Web Development Use Cases
- Processing uploaded files securely
- Generating downloadable content
- Managing static resources and assets
- Handling user-generated content
- Working with configuration and environment files
- Processing and generating API responses
- Implementing logging and diagnostics
System Administration and Automation
- Processing log files and system reports
- Managing configuration across systems
- Implementing backup and archiving solutions
- File synchronization and comparison
- System monitoring and reporting
- Batch processing system files
- Automating file organization and maintenance
Application Development Scenarios
- Storing application state and user preferences
- Building document-based applications
- Implementing file-based databases or stores
- Managing application resources efficiently
- Processing specialized document formats
- Creating import/export functionality
- Developing file-based interprocess communication
Conclusion: An Essential Resource for Python Learners
"File Handling in Python for Absolute Beginners" represents a valuable contribution to Python educational literature. By focusing exclusively on file handling, it provides unprecedented depth on an essential topic while maintaining accessibility for true beginners.
The book's greatest strengths include:
-
Accessibility with depth: The structured approach makes complex concepts approachable without oversimplification.
-
Practical orientation: Real-world examples and projects connect theory to application, demonstrating immediate relevance.
-
Comprehensive coverage: From basic operations to specialized formats and advanced techniques, the book covers the full spectrum of file handling skills.
-
Best practices emphasis: Focus on proper techniques instills professional-grade habits from the beginning.
-
Long-term reference value: Quick reference materials and logical organization make the book valuable beyond initial learning.
For anyone looking to build practical Python skills, this book offers an accessible entry point to an essential programming domain. In a technology landscape where data processing, automation, and system integration are increasingly important, the file handling skills presented in this book represent some of the most practically valuable knowledge a Python programmer can acquire.
Whether you're just starting your programming journey or looking to strengthen a specific skill area, "File Handling in Python for Absolute Beginners" provides a solid foundation for mastering one of Python's most universally applicable capabilities. It's not just an instructional text but an investment in fundamental programming skills with immediate practical applications and long-term professional value.
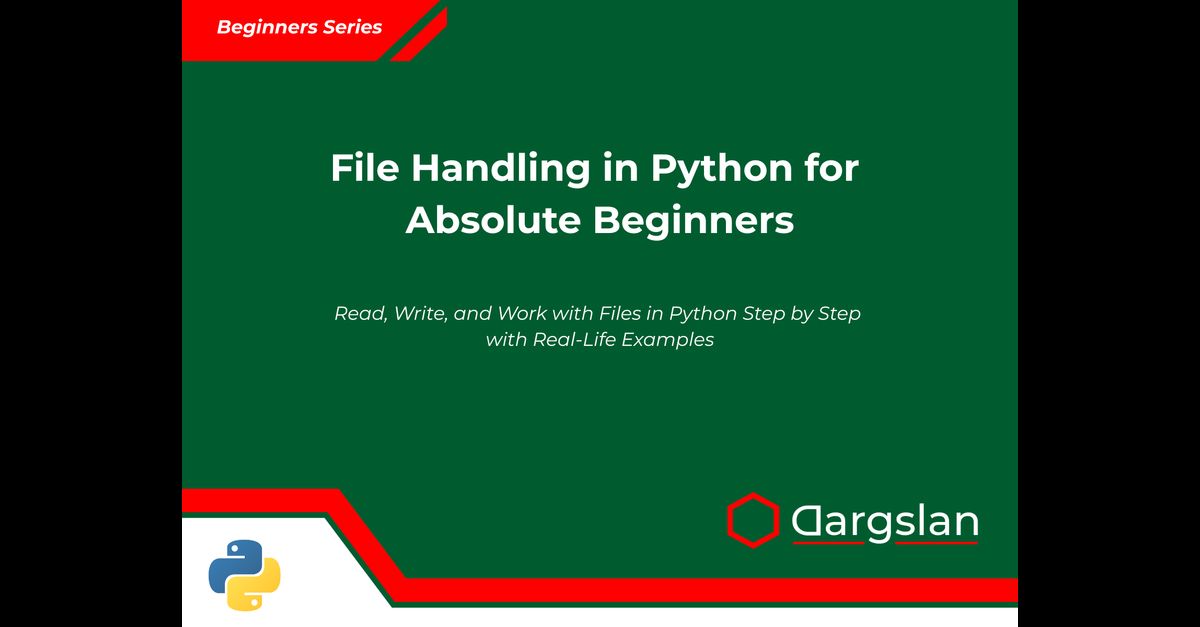
File Handling in Python for Absolute Beginners