Book Review: Debugging Legacy PHP Applications
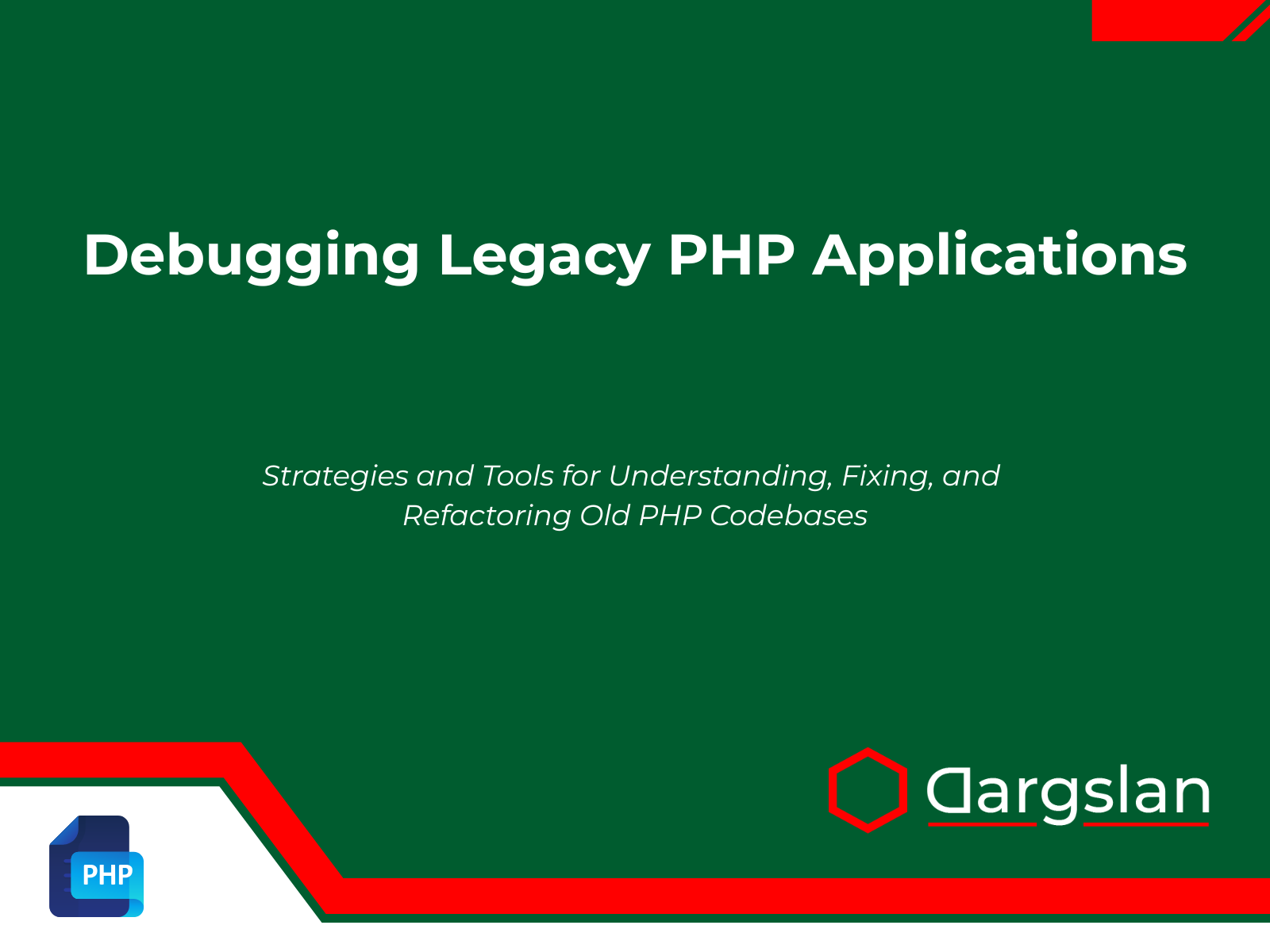
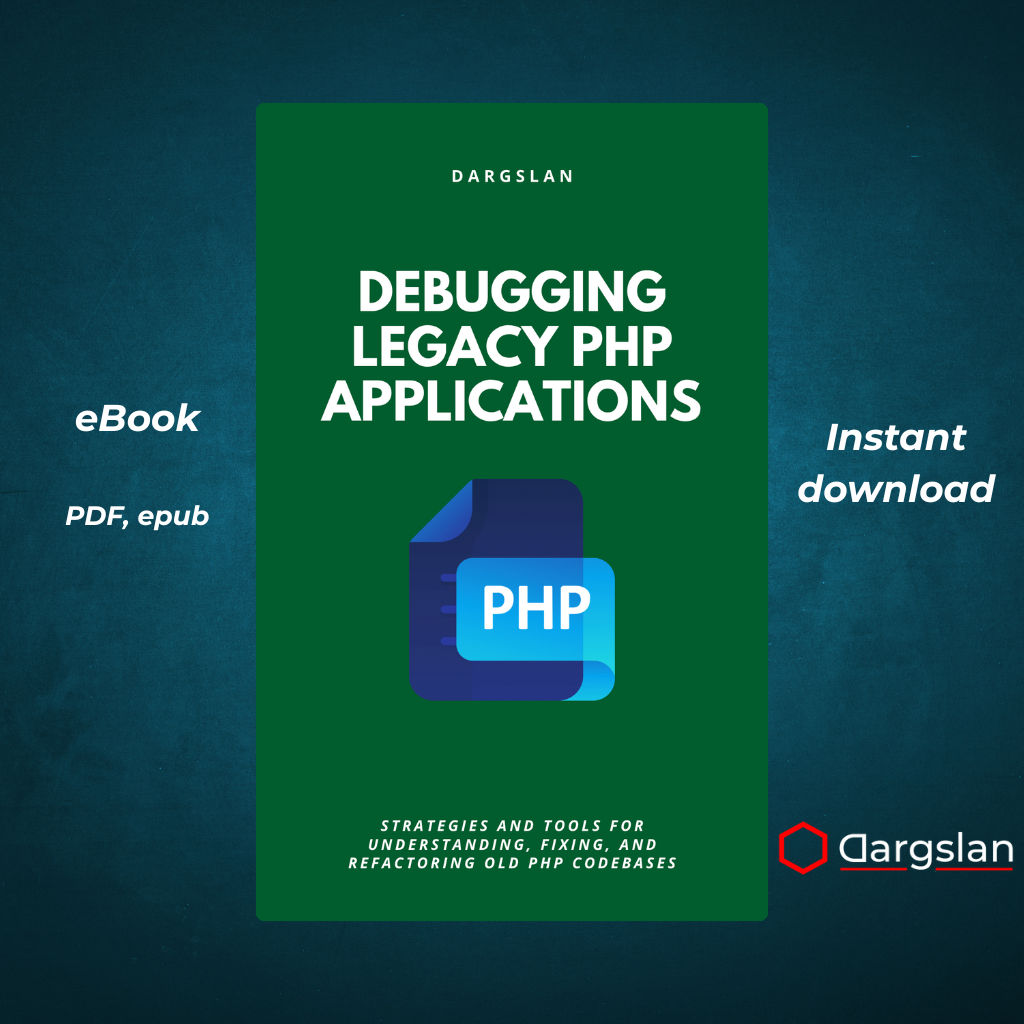
Debugging Legacy PHP Applications
Strategies and Tools for Understanding, Fixing, and Refactoring Old PHP Codebases
Debugging Legacy PHP Applications: A Comprehensive Guide
Introduction
In today's rapidly evolving web development landscape, PHP continues to power a significant portion of the internet. From small business websites to enterprise applications, PHP's extensive history has left us with countless legacy codebases that require ongoing maintenance, debugging, and modernization. "Debugging Legacy PHP Applications" addresses this critical need, offering developers a structured approach to understanding, improving, and extending older PHP systems.
Legacy code represents both a challenge and an opportunity. While outdated PHP applications may seem intimidating with their tangled logic and obsolete practices, they often contain years of refined business rules and institutional knowledge. This book provides the strategic framework and tactical tools needed to transform these aging codebases into maintainable, reliable systems without sacrificing their core functionality.
Who Should Read This Book
This comprehensive guide is ideal for:
- PHP developers tasked with maintaining inherited codebases
- Team leads managing projects with significant technical debt
- DevOps professionals supporting legacy PHP applications
- Software engineers looking to improve their debugging skills
- Web developers transitioning from modern frameworks to legacy systems
- Technical consultants who regularly encounter diverse PHP projects
Whether you're a seasoned PHP veteran or a developer who primarily works with modern frameworks but occasionally needs to dive into legacy code, this book offers valuable insights and practical techniques for navigating the complexities of older PHP applications.
Chapter Breakdowns
Chapter 1: Understanding the Legacy Code Challenge
Legacy PHP code presents unique challenges that differ significantly from greenfield development. This chapter establishes the foundation for effective legacy code management by:
- Defining what constitutes "legacy code" in the PHP ecosystem
- Examining the evolution of PHP from version 4 through PHP 8
- Addressing common emotional and psychological barriers to working with legacy code
- Introducing a structured approach to code archaeology
- Establishing realistic expectations and goals for legacy code improvement
Key Insight: Legacy code isn't inherently bad code—it's code that has survived and provided value over time. Understanding its historical context is crucial for effective maintenance.
// Example of PHP 4 era code you might encounter
function get_user_data($user_id) {
global $db_connection;
$query = "SELECT * FROM users WHERE user_id = $user_id";
$result = mysql_query($query, $db_connection);
if ($result) {
return mysql_fetch_assoc($result);
} else {
return false;
}
}
Chapter 2: Preparing Your Environment for Debugging
Effective legacy code debugging requires the right tooling and environment setup. This chapter covers:
- Creating isolated development environments for legacy applications
- Setting up Docker containers for different PHP versions
- Configuring IDE debugging tools specifically for legacy code
- Essential debugging extensions and libraries
- Managing dependencies in older PHP applications
- Version control strategies for legacy projects
Practical Application: You'll learn how to configure PHP debugging tools like Xdebug to work with older codebases, even those designed before modern debugging practices were established.
Chapter 3: First Steps
Your initial approach to a legacy codebase can determine the success of your entire debugging effort. This chapter provides a systematic methodology for your first interaction with unfamiliar code:
- Performing an initial codebase assessment
- Creating a high-level architectural map
- Identifying critical paths and core functionality
- Establishing a debugging journal and documentation process
- Setting up baseline metrics to measure improvements
- Strategies for making your first safe changes
Case Study: Follow along as we document the first week of working with a real-world PHP 5.2 e-commerce application, illustrating how to gain an understanding of complex, undocumented systems.
Chapter 4: Identifying Common Anti-Patterns
Legacy PHP applications often contain recurring problematic patterns. This chapter helps you recognize and address:
- Global variable abuse and scope issues
- SQL injection vulnerabilities in database interactions
- Inconsistent error handling approaches
- Tight coupling between presentation and business logic
- Redundant and duplicated code sections
- Performance bottlenecks from inefficient algorithms
- Security vulnerabilities common in older PHP applications
Key Technique: You'll learn how to use static analysis tools like PHP_CodeSniffer and PHPStan to automatically identify potential issues, even in codebases that weren't designed with modern standards in mind.
Chapter 5: Using Xdebug Effectively
Xdebug is a powerful tool for PHP developers, but using it with legacy applications requires special consideration. This chapter provides:
- Configuring Xdebug for different PHP versions
- Step debugging techniques for complex legacy systems
- Stack trace analysis for tracking down elusive bugs
- Performance profiling to identify bottlenecks
- Memory usage analysis for resource-intensive applications
- Remote debugging strategies for production-like environments
Code Example:
// Setting up Xdebug in older PHP applications
// php.ini configuration for PHP 5.6 compatibility
xdebug.remote_enable = 1
xdebug.remote_autostart = 1
xdebug.remote_port = 9000
xdebug.remote_handler = dbgp
xdebug.remote_host = localhost
Chapter 6: Logging and Tracing Techniques
When direct debugging isn't feasible, logging becomes invaluable. This chapter explores:
- Implementing effective logging in legacy applications
- Creating audit trails for debugging complex user flows
- Log rotation and management in production environments
- Distributed tracing for multi-component systems
- Visualizing application flow through logs
- Retrofitting modern logging libraries into legacy code
Best Practice: Learn how to implement contextual logging that captures not just errors but the state of the application at critical points, making it easier to reproduce and fix issues.
Chapter 7: Isolating and Reproducing Bugs
One of the greatest challenges in legacy debugging is consistently reproducing reported issues. This chapter teaches:
- Techniques for isolating bug-triggering conditions
- Creating minimal reproducible test cases
- Working with incomplete or vague bug reports
- Identifying environmental factors affecting bug manifestation
- Techniques for debugging intermittent issues
- Data capture methods for hard-to-reproduce bugs
Real-world Scenario: Explore a methodical approach to tracking down a session handling bug that only appears under specific user interaction patterns, demonstrating the detective work often required in legacy debugging.
Chapter 8: Reverse Engineering Legacy Logic
When documentation is sparse or non-existent, reverse engineering becomes necessary. This chapter covers:
- Mapping implicit business rules from code behavior
- Extracting domain models from procedural code
- Visualizing complex workflows and dependencies
- Identifying core algorithms and their purpose
- Separating essential business logic from implementation details
- Techniques for incrementally building understanding
Visualization Example: Learn how to create dependency graphs that visualize relationships between different parts of a legacy system, revealing design patterns that may not be immediately obvious from the code alone.
Chapter 9: Writing Tests for Untestable Code
Legacy PHP applications rarely come with comprehensive test suites. This chapter demonstrates:
- Strategies for adding tests to code never designed for testability
- Implementing characterization tests to document existing behavior
- Using test doubles to isolate components for testing
- Database testing approaches for legacy applications
- Gradually improving testability without rewriting everything
- Test-driven bug fixing for legacy code
Technical Approach: You'll learn how to use PHP testing frameworks like PHPUnit to create meaningful tests even for tightly coupled code that was never designed with testing in mind.
// Example of a characterization test for legacy code
public function testExistingUserAuthentication() {
// Capture the current behavior before making changes
$originalUser = array('username' => 'legacy_user', 'password' => 'old_hash_format');
$originalResult = legacy_authenticate_user($originalUser['username'], $originalUser['password']);
// Verify new implementation maintains the same behavior
$this->assertSame(
$originalResult,
$this->newAuthenticator->authenticate($originalUser['username'], $originalUser['password'])
);
}
Chapter 10: Safe Refactoring Strategies
Improving legacy code without breaking functionality requires careful refactoring. This chapter provides:
- Incremental refactoring techniques for high-risk code
- The strangler pattern for gradually replacing components
- Extracting methods and classes from procedural code
- Moving from global state to dependency injection
- Converting implicit to explicit dependencies
- Introducing modern design patterns without complete rewrites
Illustrated Process: Follow a step-by-step guide to refactoring a monolithic PHP script into a more maintainable object-oriented design while ensuring all existing functionality continues to work correctly.
Chapter 11: Working with Legacy Databases
Legacy PHP applications often include outdated database practices. This chapter addresses:
- Safely evolving database schemas in production environments
- Moving from raw SQL to parameterized queries
- Implementing data access layers for database abstraction
- Handling deprecated MySQL functions and extensions
- Performance optimization for legacy database interactions
- Data migration strategies for modernization efforts
Database Evolution Strategy: Learn how to incrementally improve database interactions while maintaining backward compatibility, using techniques like the repository pattern to encapsulate legacy database access.
Chapter 12: Documentation and Long-Term Maintenance
The final chapter focuses on sustaining your improvements over time:
- Creating effective documentation for legacy systems
- Building institutional knowledge around critical components
- Balancing maintenance with strategic improvements
- Training new team members on legacy codebases
- Planning for eventual system replacement or modernization
- Measuring and communicating the value of legacy code improvements
Maintenance Framework: Discover a structured approach to ongoing legacy application maintenance that balances immediate needs with long-term technical debt reduction.
Special Appendices
Appendix A: Tools for PHP Legacy Debugging
A comprehensive reference guide to tools specifically useful for legacy PHP debugging:
- Static analysis tools that support older PHP versions
- Compatibility layers for modern development tooling
- Environment virtualization and containerization tools
- Legacy-compatible debugging extensions
- Code transformation and migration utilities
- Performance analysis tools for older PHP applications
Appendix B: Deprecated PHP Features by Version
An invaluable reference chart showing:
- Deprecated functions, classes, and features by PHP version
- Recommended replacements for obsolete functionality
- Backward compatibility break points to be aware of
- Critical security changes between versions
- Performance implications of PHP version upgrades
Appendix C: Resources for Legacy PHP Projects
A curated collection of:
- Community forums specializing in legacy PHP support
- Documentation archives for older PHP versions
- Migration guides for common PHP frameworks
- Legacy code modernization case studies
- Training resources for legacy PHP maintenance
- Compatibility libraries and polyfills
Real-World Applications
Throughout "Debugging Legacy PHP Applications," you'll encounter practical examples based on real-world scenarios, including:
- E-commerce platforms built on custom PHP frameworks
- Content management systems developed before modern CMS solutions
- Enterprise resource planning (ERP) systems with decades of customizations
- Online community platforms running on PHP 5.x
- Payment processing systems with complex compliance requirements
- Data processing pipelines designed for earlier PHP versions
Each example illustrates common challenges and provides actionable solutions that you can apply to your own legacy projects.
Why Legacy PHP Skills Matter
Mastering legacy PHP debugging provides significant career advantages:
- Job Security - Organizations value developers who can maintain critical legacy systems that others avoid
- Higher Compensation - Specialized legacy skills often command premium rates due to limited market availability
- Broader Perspective - Understanding evolution of programming practices enhances your overall development approach
- Problem-Solving Growth - Legacy debugging builds advanced diagnostic and analytical skills
- Business Impact - Improving legacy systems typically delivers direct business value
Modern Tools for Legacy Challenges
The book emphasizes how modern development practices can be adapted for legacy contexts:
- Implementing CI/CD pipelines for legacy PHP applications
- Using static analysis with reduced strictness for gradual improvement
- Applying containerization to standardize legacy development environments
- Integrating automated testing into previously untested codebases
- Implementing modern logging and monitoring in legacy systems
- Using feature flags for safer legacy code modifications
From the Experts
Throughout the book, you'll find insights from experienced developers who specialize in legacy PHP maintenance:
"The key to successful legacy code maintenance isn't rewriting everything—it's understanding the business value embedded in the existing code and preserving it while incrementally improving the implementation." — Maria Rodriguez, Lead Application Architect
"I've seen companies spend millions rewriting legacy applications only to lose critical functionality in the process. A methodical debugging and improvement approach almost always delivers better ROI." — James Chen, PHP Legacy Systems Consultant
Conclusion
"Debugging Legacy PHP Applications" transforms what many developers consider a dreaded task into a structured, manageable process. By combining analytical techniques, practical tools, and strategic thinking, this comprehensive guide empowers you to confidently tackle even the most challenging legacy PHP codebases.
Whether you're maintaining mission-critical applications, modernizing outdated systems, or simply trying to understand the inherited code you're now responsible for, this book provides the roadmap you need to succeed. Legacy code doesn't have to remain mysterious or intimidating—with the right approach, it becomes an opportunity to demonstrate your problem-solving abilities while delivering significant value to your organization.
Start your journey to legacy PHP mastery today, and transform perceived liabilities into valuable assets for your development career and your business.
About the Author
Dargslan brings over 15 years of experience in PHP development, specializing in the maintenance and modernization of legacy applications. Having worked with PHP systems spanning versions 4 through 8, they have developed practical methodologies for making legacy code more maintainable, secure, and aligned with modern development practices. Their approach emphasizes pragmatic, business-focused improvements over theoretical purity, helping organizations extract maximum value from their technology investments.
"Debugging Legacy PHP Applications: Strategies and Tools for Understanding, Fixing, and Refactoring Old PHP Codebases" provides the comprehensive guidance every PHP developer needs when faced with the challenges of legacy code maintenance.
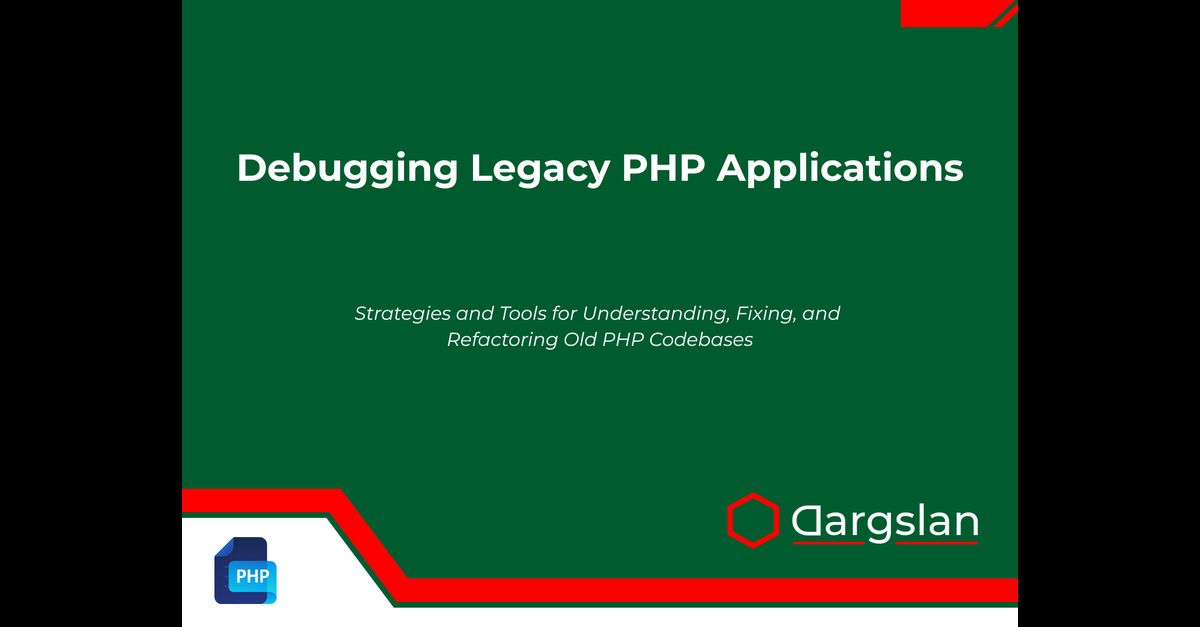
Debugging Legacy PHP Applications