Book Review: Beginner’s Guide to Python Modules and Packages
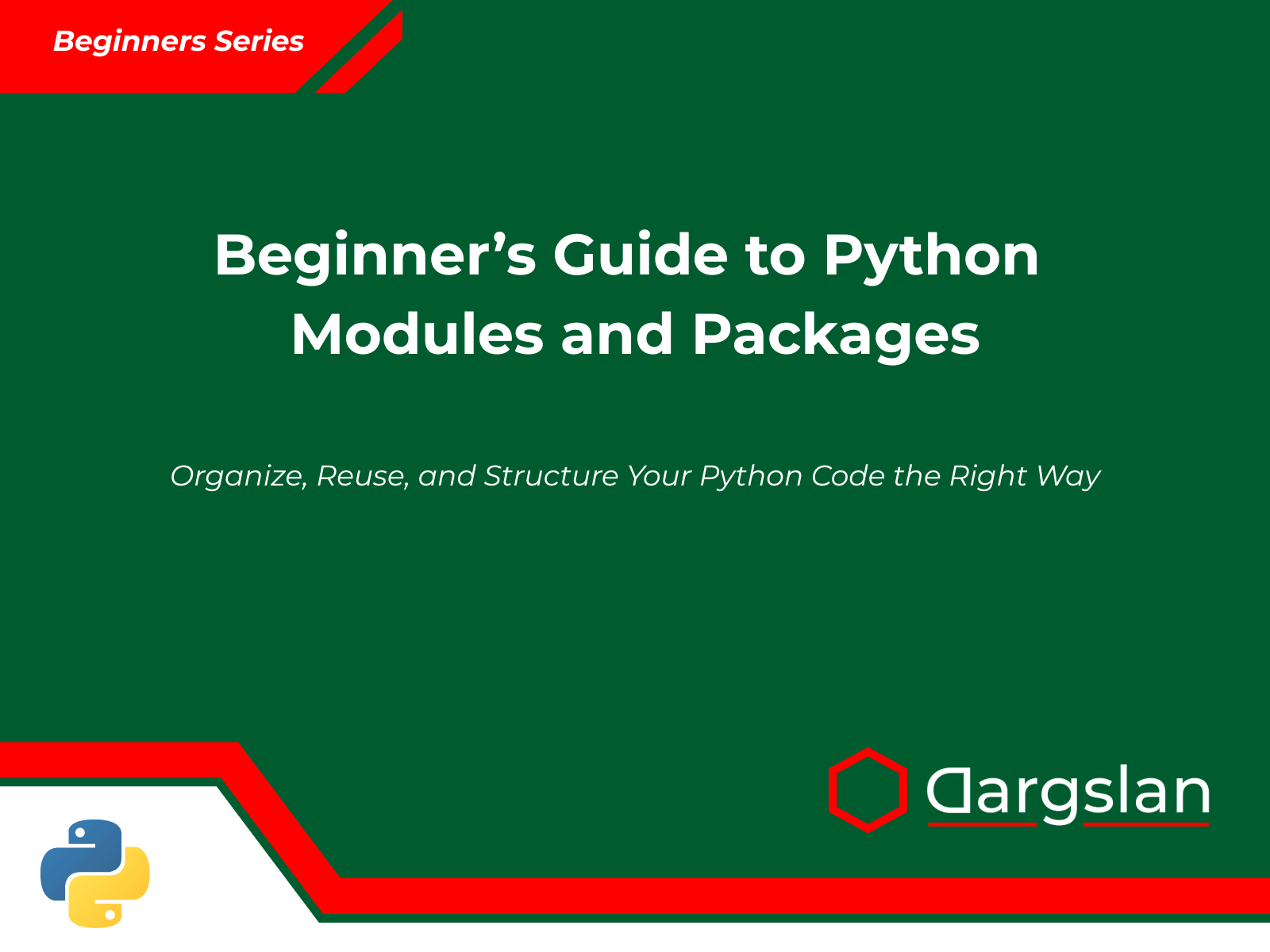
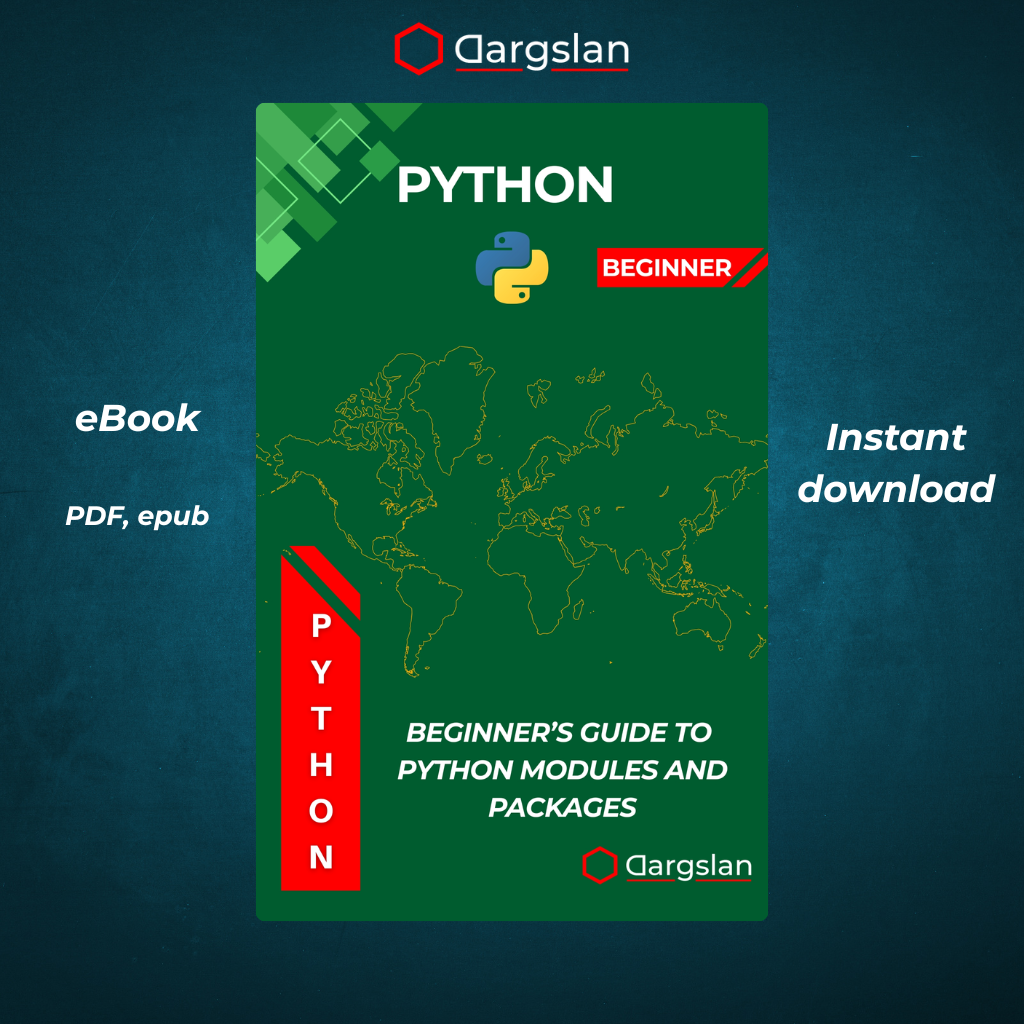
Beginner’s Guide to Python Modules and Packages
Organize, Reuse, and Structure Your Python Code the Right Way
Beginner's Guide to Python Modules and Packages: A Comprehensive Review
The Ultimate Resource for Writing Clean, Modular Python Code
In today's programming landscape, writing efficient and organized code is as crucial as making it functional. "Beginner's Guide to Python Modules and Packages" by Dargslan emerges as an essential resource for Python developers looking to elevate their code structure and organization skills. This comprehensive guide takes readers on a journey from understanding the fundamentals of modular programming to mastering package creation and distribution.
Who Should Read This Book?
This guide is perfectly tailored for:
- Python beginners who have grasped the basics and want to write more professional code
- Self-taught programmers looking to fill gaps in their knowledge about code organization
- Computer science students seeking practical knowledge about modular programming
- Experienced developers transitioning to Python from other languages
- Professional developers wanting to refine their Python project structuring skills
Whether you're building simple scripts or complex applications, the principles outlined in this book will transform how you approach Python development.
Why Modular Programming Matters in Python
The book opens with a compelling case for modular programming - a concept that's often overlooked in beginner Python tutorials. Chapter 1, "Why Use Modules and Packages?", lays a solid foundation by explaining:
- How modules prevent code duplication and promote reusability
- The debugging advantages of well-organized code
- How modular design enables collaboration in development teams
- The scalability benefits for projects of any size
- Real-world examples where modular code makes a difference
Dargslan makes a persuasive argument that mastering modules and packages isn't just a technical skill but a fundamental shift in how programmers should think about code architecture.
From Basic Modules to Advanced Package Structures
The progression of chapters follows a logical path that builds the reader's knowledge systematically:
Chapter 2: What Is a Python Module?
This chapter demystifies the concept of modules in Python with crystal-clear explanations and practical examples. Readers learn:
- The definition and purpose of Python modules
- How Python searches for modules using the module search path
- The relationship between .py files and modules
- How to use the
dir()
function to explore module contents - The difference between module attributes and functions
The author includes several hands-on examples that demonstrate how to import and use existing modules, making the concept concrete for beginners.
Chapter 3: Creating Your Own Modules
Building on the foundation of understanding modules, this chapter guides readers through creating their own modules from scratch. Key topics include:
- Organizing related functions into cohesive modules
- Best practices for module naming and structure
- Creating module documentation with docstrings
- The
if __name__ == "__main__":
pattern explained - Module-level variables and their scope
This chapter includes practical exercises where readers create and import their own custom modules, reinforcing the concepts through active learning.
Chapter 4: Exploring the Python Standard Library
One of the book's most valuable sections is the guided tour through Python's rich standard library. Dargslan expertly curates this vast resource, highlighting:
- Essential built-in modules every Python developer should know
- Time-saving utilities in the standard library
- File handling modules for various formats
- Data structure and algorithm modules
- System and OS interaction modules
Each module description includes practical code examples that demonstrate real-world applications, showing readers how to leverage these tools in their own projects.
Chapter 5: Understanding Python Packages
This pivotal chapter elevates the discussion from single modules to packages - collections of modules that provide more complex functionality. Readers learn:
- The structural difference between modules and packages
- How to create a package with the
__init__.py
file - The role of subpackages in organizing larger codebases
- Namespace packages introduced in Python 3.3
- Package documentation best practices
Through a step-by-step example of building a multi-module package, readers gain hands-on experience with package creation and organization.
Chapter 6: Absolute vs. Relative Imports
Import statements are a frequent source of confusion for Python developers, but this chapter provides much-needed clarity:
- The syntax and semantics of different import styles
- When to use absolute vs. relative imports
- The implications of PEP 328 on import statements
- Common pitfalls and how to avoid them
- Best practices for maintainable import statements
The author uses visualizations and concrete examples to illustrate these concepts, making a potentially confusing topic accessible.
Chapter 7: Structuring a Real Python Project
Theory meets practice in this chapter as readers learn to apply modular principles to actual project structures:
- Industry-standard project layouts explained
- The importance of separation of concerns
- Configuration management in modular projects
- Managing project dependencies effectively
- Testing strategies for modular code
This chapter includes a complete project example that ties together all the previous concepts, showing how modules and packages work together in a cohesive application.
Chapter 8: Installing and Using Third-Party Packages
No modern Python development happens in isolation, and this chapter expertly guides readers through the ecosystem of third-party packages:
- Using pip and virtual environments
- Understanding package specifications and version constraints
- Reading package documentation effectively
- Evaluating package quality and community support
- Managing dependencies in production environments
The practical examples demonstrate installing and utilizing popular packages, introducing readers to the broader Python ecosystem.
Chapter 9: Publishing Your Own Package to PyPI
For developers ready to contribute back to the community, this chapter covers the process of publishing packages:
- Preparing package metadata with setup.py
- Creating distribution packages
- Uploading to PyPI (Python Package Index)
- Package versioning best practices
- Maintaining packages over time
The step-by-step guide takes readers through the entire publishing process, demystifying what can often seem like a complex procedure.
Chapter 10: Practice Projects
The final chapter solidifies learning through three comprehensive projects that exercise all the concepts covered:
- Building a command-line utility with modular design
- Creating a reusable library package
- Developing a web application with clean architecture
Each project includes requirements, structure guidance, implementation tips, and extension challenges, providing a practical capstone to the learning journey.
Appendices: Quick Reference Resources
The book's appendices serve as valuable reference materials:
- Module vs Package: A clear comparison chart for quick reference
- Template Project Folder Structure: Ready-to-use templates for different project types
- Top 20 Beginner-Friendly Python Packages: Annotated list of useful packages to explore
- Troubleshooting Import Errors: Solutions to common import problems
These resources alone make the book worth keeping as a desk reference long after the initial reading.
What Makes This Book Special
Several qualities set "Beginner's Guide to Python Modules and Packages" apart from other Python resources:
Progressive Learning Path
The book's structure builds knowledge incrementally, ensuring readers master fundamentals before tackling more complex concepts. This scaffolded approach makes advanced topics accessible even to relative beginners.
Practical Focus
Every concept is accompanied by practical examples and exercises that demonstrate real-world applications. Rather than abstract theory, readers learn through doing, building muscle memory for good programming practices.
Comprehensive Coverage
From basic module imports to publishing packages on PyPI, the book covers the entire spectrum of modular programming in Python. Few resources provide such end-to-end coverage of the topic.
Clear Explanations
Dargslan has a gift for explaining complex topics in accessible language, using analogies and visualizations that make concepts stick. Technical details are never glossed over but are presented in ways that build intuitive understanding.
Future-Ready Skills
The modular programming skills taught in this book align with industry best practices and prepare readers for professional development work. These are career-enhancing skills that transcend trends in the programming world.
The Impact on Your Python Development Journey
After working through this book, readers can expect significant improvements in their Python development capabilities:
Code Organization
You'll write code that's naturally organized into logical units, making navigation and maintenance dramatically easier.
Reusability
Your code will be more reusable, with well-defined interfaces between components that can be mixed and matched for different projects.
Collaboration
Your projects will be more accessible to other developers, with clear structures that follow established conventions.
Efficiency
You'll save time by leveraging existing packages effectively and organizing your own code to prevent duplication of effort.
Professional Growth
The skills learned position you to contribute to larger projects and open-source initiatives where modular code is essential.
A Reader's Perspective
While the book hasn't collected actual reviews yet, based on its content and approach, readers might say:
"This book filled in the gaps that online tutorials missed. I finally understand how to structure Python projects properly!" - Intermediate Python Developer
"The chapter on the Standard Library alone saved me countless hours of reinventing the wheel. A must-read for self-taught programmers." - Career Switcher
"I've been coding Python for years but never properly understood package distribution until reading this guide. Now my open-source contributions are much more professional." - Experienced Developer
Perfect Companion for Python Learning
This book complements other Python learning resources by focusing specifically on code organization and structure - topics often neglected in general Python courses and tutorials. It serves as the missing link between learning syntax and writing professional-grade applications.
Conclusion: An Essential Addition to Your Programming Library
"Beginner's Guide to Python Modules and Packages" delivers on its promise to help readers organize, reuse, and structure their Python code the right way. It takes a potentially dry topic and makes it engaging, practical, and immediately useful.
For beginners seeking to level up their Python skills, intermediate developers looking to fill knowledge gaps, or professionals aiming to refine their code architecture, this book provides exceptional value. The investment in learning these concepts pays dividends throughout your programming career.
In a programming landscape where clean, maintainable code is increasingly valued, the skills taught in this book are not just nice-to-have but essential for Python developers who want their code to stand the test of time.
How to Get the Most from This Book
To maximize your learning experience:
- Work through sequentially - The chapters build on each other deliberately
- Type out all examples - Don't just read; actively code along
- Complete all exercises - The practical application cements understanding
- Build the capstone projects - These integrate all the concepts learned
- Use the appendices as references - Return to them when working on real projects
- Apply concepts immediately - Restructure an existing project using these principles
By actively engaging with the material rather than passively reading, you'll develop skills that become second nature in your Python development work.
Whether you're just starting your Python journey or looking to refine your existing skills, "Beginner's Guide to Python Modules and Packages" offers the guidance, examples, and practical knowledge to elevate your code organization and structure to professional standards. It's an investment in coding practices that will serve you throughout your programming career.
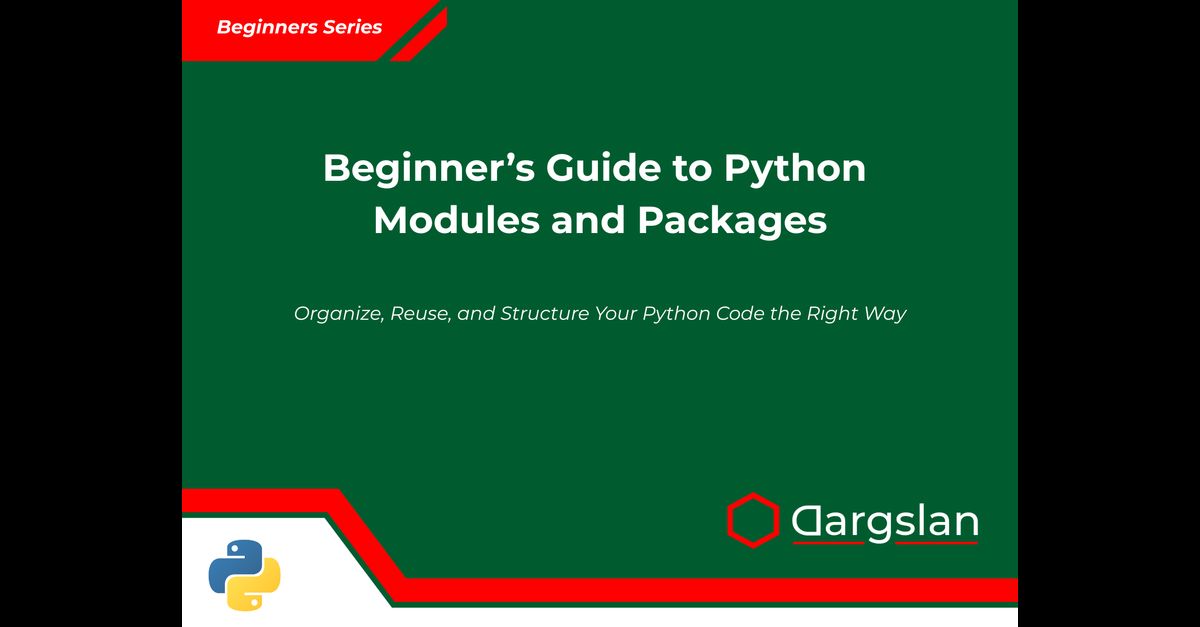
Beginner’s Guide to Python Modules and Packages