Book Review: Advanced Object-Oriented Programming in Python
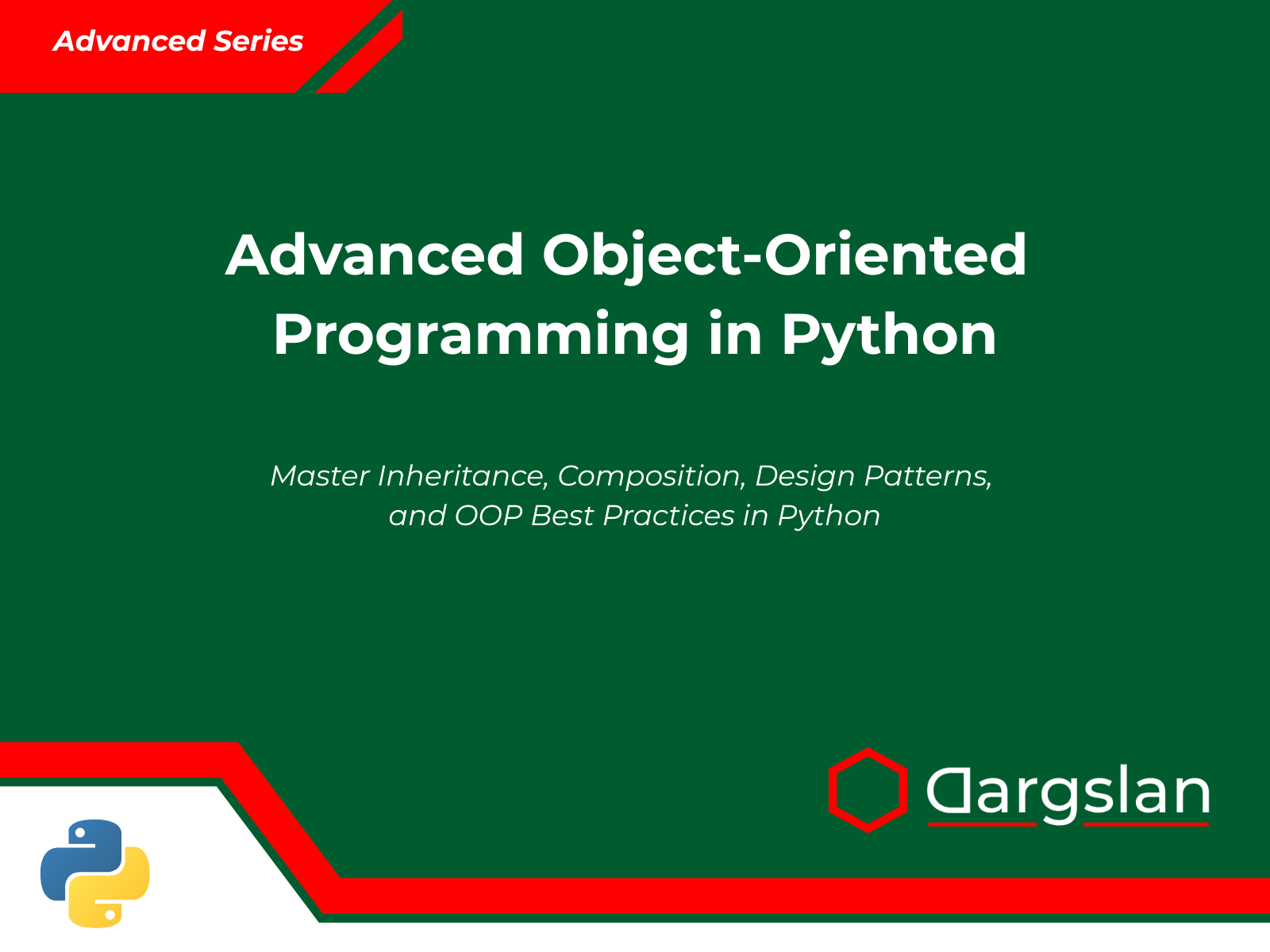
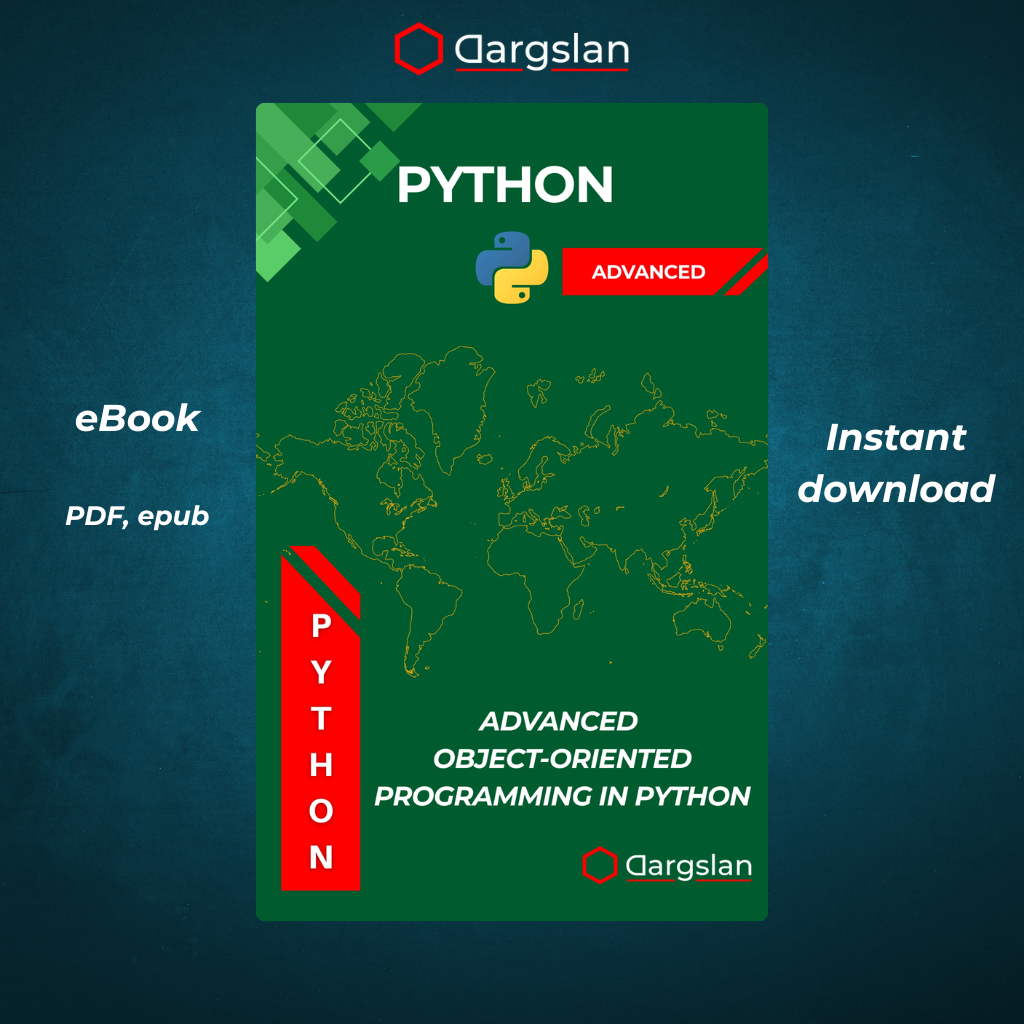
Advanced Object-Oriented Programming in Python
Master Inheritance, Composition, Design Patterns, and OOP Best Practices in Python
Advanced Object-Oriented Programming in Python: A Comprehensive Guide to Mastering OOP Concepts
Introduction: Elevating Your Python OOP Skills
In the ever-evolving landscape of Python programming, mastering advanced object-oriented programming (OOP) techniques has become essential for developers looking to build scalable, maintainable applications. The book "Advanced Object-Oriented Programming in Python: Master Inheritance, Composition, Design Patterns, and OOP Best Practices in Python" offers a comprehensive journey through Python's powerful OOP capabilities, providing both theoretical foundations and practical implementations.
Unlike many programming books that merely scratch the surface of OOP concepts, this guide delves deep into Python's object model, exploring everything from the intricacies of multiple inheritance to the application of design patterns and SOLID principles. Whether you're a self-taught Python developer seeking to formalize your OOP knowledge, an experienced programmer transitioning from another language, or a professional looking to adopt best practices, this book serves as an indispensable resource.
Why Advanced OOP Matters in Python
Python's flexibility as a multi-paradigm language sometimes leads developers to underestimate the importance of proper object-oriented design. However, as applications grow in complexity, well-designed OOP structures become crucial for:
- Maintainability: Properly encapsulated classes with clear responsibilities make code easier to update and debug
- Scalability: Well-defined inheritance hierarchies and composition patterns allow systems to grow organically
- Reusability: Properly designed classes can be repurposed across different projects
- Collaboration: Common OOP patterns create shared vocabulary and expectations among team members
This book bridges the gap between basic Python knowledge and advanced architectural thinking, empowering readers to design robust systems that can evolve with changing requirements.
Book Structure: A Progressive Learning Journey
Chapter 1: OOP Refresher – The Pillars of Object-Oriented Python
The journey begins with a thoughtful recap of OOP's core principles—encapsulation, inheritance, polymorphism, and abstraction—specifically within Python's context. This chapter goes beyond basic definitions to explore how Python's "everything is an object" philosophy creates a uniquely consistent object model.
Readers will discover:
- How Python implements encapsulation despite lacking true private attributes
- The nuances of Python's dynamic polymorphism compared to static languages
- Practical examples of abstraction that balance flexibility with structure
- Implementation details of
__init__
and__str__
that affect everyday coding
class BankAccount:
def __init__(self, owner, balance=0):
self._owner = owner # Protected by convention
self._balance = balance
self._transaction_log = []
def deposit(self, amount):
if amount <= 0:
raise ValueError("Deposit amount must be positive")
self._balance += amount
self._transaction_log.append(f"Deposit: {amount}")
return self._balance
def __str__(self):
return f"{self._owner}'s account. Balance: ${self._balance}"
This foundation ensures all readers start with a shared understanding before tackling more advanced concepts.
Chapter 2: Inheritance, Overriding, and super()
Chapter 2 explores inheritance, one of OOP's most powerful yet potentially confusing features. Python's support for both single and multiple inheritance offers tremendous flexibility but requires careful understanding.
Key topics include:
- The mechanics and implications of inheritance in Python
- Method Resolution Order (MRO) and the C3 linearization algorithm
- Best practices for method overriding to maintain expected behaviors
- Proper use of
super()
for cooperative inheritance
The Shape classes example provides a concrete demonstration:
class Shape:
def area(self):
raise NotImplementedError("Subclasses must implement area()")
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Square(Rectangle):
def __init__(self, side_length):
super().__init__(side_length, side_length)
This chapter equips readers with a deep understanding of inheritance mechanics, setting the stage for more advanced patterns.
Chapter 3: Composition Over Inheritance
This pivotal chapter addresses one of modern OOP's most important design principles: favoring composition over inheritance. While inheritance represents "is-a" relationships, composition models "has-a" relationships, often leading to more flexible designs.
Readers learn:
- Why composition often creates more adaptable, loosely-coupled systems
- Techniques for implementing delegation and forwarding
- How to recognize when inheritance is appropriate versus when composition offers advantages
- Strategies for refactoring inheritance-heavy designs to composition-based approaches
The notification system example brilliantly illustrates these concepts:
# Composition approach
class NotificationService:
def __init__(self):
self.channels = []
def add_channel(self, channel):
self.channels.append(channel)
def remove_channel(self, channel):
self.channels.remove(channel)
def notify(self, message):
for channel in self.channels:
channel.send(message)
class EmailChannel:
def send(self, message):
print(f"Sending email: {message}")
class SMSChannel:
def send(self, message):
print(f"Sending SMS: {message}")
This flexible design allows adding new notification channels without modifying existing code, demonstrating composition's adaptability.
Chapter 4: Abstract Base Classes and Interfaces
Chapter 4 explores how Python's dynamic nature can be combined with more explicit interface contracts through Abstract Base Classes (ABCs). While Python embraces duck typing, ABCs provide a mechanism for enforcing method implementations in subclasses.
The chapter covers:
- Using the
abc
module and@abstractmethod
decorator - Creating class hierarchies with enforced interfaces
- Balancing Python's flexibility with explicit contracts
- Virtual subclasses and runtime interface checking
from abc import ABC, abstractmethod
class PaymentProcessor(ABC):
@abstractmethod
def process_payment(self, amount):
"""Process a payment for the given amount."""
@abstractmethod
def refund(self, amount):
"""Process a refund for the given amount."""
class StripeProcessor(PaymentProcessor):
def process_payment(self, amount):
print(f"Processing ${amount} payment via Stripe")
def refund(self, amount):
print(f"Refunding ${amount} via Stripe")
This approach ensures that all payment processors implement the required methods, catching errors at instantiation time rather than during operation.
Chapter 5: Mixins and Multiple Inheritance
Building on the inheritance foundation, Chapter 5 explores the powerful pattern of mixins—small, focused classes that provide reusable functionality through multiple inheritance.
Key topics include:
- The concept and implementation of mixins in Python
- Guidelines for creating well-behaved mixin classes
- Avoiding the pitfalls of diamond inheritance
- Combining mixins with regular inheritance hierarchies
The chapter's example demonstrates logging and serialization mixins:
class LoggingMixin:
def log(self, message, level='info'):
print(f"[{level.upper()}] {message}")
def log_method_call(self, method_name, *args, **kwargs):
self.log(f"Calling {method_name} with args: {args}, kwargs: {kwargs}")
class JSONSerializableMixin:
def to_json(self):
return json.dumps(self.__dict__)
def from_json(self, json_data):
data = json.loads(json_data)
for key, value in data.items():
setattr(self, key, value)
class User(LoggingMixin, JSONSerializableMixin):
def __init__(self, name, email):
self.name = name
self.email = email
def send_email(self, subject, body):
self.log_method_call('send_email', subject, body)
# Email sending logic here
This pattern allows for horizontal code reuse across different class hierarchies, a powerful complement to inheritance's vertical reuse.
Chapter 6: Special Methods and Operator Overloading
Chapter 6 dives into Python's special methods (also known as "magic methods" or "dunder methods"), which allow classes to integrate seamlessly with Python's built-in functions and operators.
The chapter provides comprehensive coverage of:
- Essential magic methods like
__repr__
,__len__
,__getitem__
, and__eq__
- Implementing custom container and iterator classes
- Making classes work with Python's operators through operator overloading
- Creating context managers, descriptors, and callable objects
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __mul__(self, scalar):
return Vector(self.x * scalar, self.y * scalar)
def __len__(self):
return 2 # 2D vector has two components
def __getitem__(self, index):
if index == 0:
return self.x
elif index == 1:
return self.y
raise IndexError("Vector index out of range")
def __repr__(self):
return f"Vector({self.x}, {self.y})"
By implementing these special methods, custom classes can behave like built-in types, creating more intuitive APIs and enabling seamless integration with Python's syntax.
Chapter 7: The SOLID Principles in Python
Chapter 7 adapts the influential SOLID principles to Python's unique features and idioms. Originally formulated for statically-typed languages, these principles provide valuable guidance for creating maintainable object-oriented code in any language.
The chapter explores each principle in depth:
Single Responsibility Principle (SRP): Classes should have only one reason to change.
# Before: class doing too much
class User:
def __init__(self, name, email):
self.name = name
self.email = email
def save(self):
# Database logic here
def send_welcome_email(self):
# Email sending logic here
# After: Separated responsibilities
class User:
def __init__(self, name, email):
self.name = name
self.email = email
class UserRepository:
def save(self, user):
# Database logic here
class UserNotifier:
def send_welcome_email(self, user):
# Email sending logic here
Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification.
Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types without altering program correctness.
Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they don't use.
Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules; both should depend on abstractions.
A practical refactoring example walks through applying these principles to a real-world codebase, transforming a monolithic class into a SOLID design.
Chapter 8: Applying Design Patterns in Python
Chapter 8 explores how classic design patterns can be implemented in Python, often more elegantly than in other languages due to Python's dynamic nature and first-class functions.
The chapter covers:
- Creational patterns like Singleton and Factory
- Structural patterns like Decorator and Adapter
- Behavioral patterns like Strategy, Observer, and Command
Each pattern is presented with Pythonic implementations that avoid unnecessary complexity:
# Strategy pattern with functions instead of classes
def order_shipping_standard(order):
return 5.99
def order_shipping_express(order):
return 15.99
def order_shipping_international(order):
return 25.99 + (1.5 * order.weight)
class Order:
def __init__(self, items, shipping_strategy=order_shipping_standard):
self.items = items
self.weight = sum(item.weight for item in items)
self.shipping_strategy = shipping_strategy
def calculate_shipping(self):
return self.shipping_strategy(self)
The chapter emphasizes adapting patterns to leverage Python's strengths rather than blindly following templates from other languages, avoiding overengineering while preserving the core intent of each pattern.
Chapter 9: Metaclasses and Class Creation Internals
Chapter 9 ventures into advanced territory with metaclasses—classes that create classes. By understanding how Python creates classes, developers can customize this process for powerful effects.
The chapter explores:
- The mechanics of class creation in Python
- How metaclasses intercept and modify the class creation process
- Practical use cases for metaclasses
- Alternatives to metaclasses for simpler scenarios
class EnforceAttributesMeta(type):
def __new__(mcs, name, bases, namespace):
# Enforce that all attributes follow snake_case convention
for key in namespace:
if not (key.startswith('__') and key.endswith('__')):
if not key.islower() and '_' not in key:
raise ValueError(f"Attribute {key} doesn't follow snake_case convention")
return super().__new__(mcs, name, bases, namespace)
class Model(metaclass=EnforceAttributesMeta):
pass
class User(Model):
user_id = 0 # This works
userName = 0 # This raises ValueError
While emphasizing metaclasses' power, the chapter also advises caution, recommending simpler approaches when they suffice and providing guidelines for when metaclasses are truly appropriate.
Chapter 10: Building a Scalable OOP Project
The final chapter brings together all previous concepts to address system-level architecture. Rather than focusing on individual classes, it explores how to create coherent, maintainable systems composed of many interacting objects.
Readers will learn:
- Strategies for designing modular, extensible architectures
- Techniques for managing dependencies between components
- Testing approaches for object-oriented systems
- Documentation best practices for OOP codebases
The capstone project—building a plugin-based system—demonstrates these principles in action:
class PluginManager:
def __init__(self):
self.plugins = {}
def register_plugin(self, name, plugin_cls):
if not hasattr(plugin_cls, 'execute'):
raise ValueError(f"Plugin {name} missing required 'execute' method")
self.plugins[name] = plugin_cls
def get_plugin(self, name):
return self.plugins.get(name)
def execute_plugin(self, name, *args, **kwargs):
plugin = self.get_plugin(name)
if not plugin:
raise ValueError(f"No plugin named {name} registered")
return plugin().execute(*args, **kwargs)
This framework allows for extensibility through plugins while maintaining a stable core, exemplifying the architectural principles discussed throughout the book.
Valuable Appendices: Extending Your Knowledge
Appendix A: Glossary of Advanced OOP Terms
This comprehensive glossary demystifies OOP terminology, providing clear definitions and Python-specific context. Entries cover everything from "Abstract Class" to "Visitor Pattern," making this an invaluable reference when reading other OOP literature or discussing concepts with peers.
Appendix B: Comparison with OOP in Java and C#
For developers transitioning from other languages, this appendix highlights key differences in OOP implementation between Python, Java, and C#. Side-by-side code comparisons demonstrate how to translate common patterns between languages while embracing Python's unique strengths.
Appendix C: UML Diagrams for Python Classes
This appendix adapts Unified Modeling Language (UML) conventions for Python's specific features, showing how to visually represent inheritance, composition, and other relationships in Python code. Example diagrams illustrate how to model Python's dynamic typing, duck typing, and mixins in standard UML notation.
Appendix D: Advanced Exercises and Practice Challenges
The book concludes with challenging exercises that reinforce concepts from each chapter. Rather than trivial tasks, these exercises present realistic scenarios requiring thoughtful application of OOP principles, providing valuable hands-on experience with designing complex systems.
Teaching Methodology: Theory Meets Practice
The book employs a multi-faceted approach to teaching advanced OOP concepts:
- Theoretical foundations: Each topic begins with clear explanations of underlying principles
- Practical examples: Realistic code samples demonstrate concepts in action
- Common pitfalls: Warnings about frequently encountered problems and misconceptions
- Refactoring examples: Before-and-after demonstrations of improving existing code
- Visual aids: UML diagrams, flowcharts, and other visuals clarify complex relationships
- Progressive complexity: Concepts build on each other throughout the book
This approach accommodates different learning styles while ensuring concepts are not just understood but can be applied practically.
Target Audience: Who Will Benefit
This book is ideal for:
- Intermediate Python developers looking to deepen their OOP knowledge
- Experienced programmers from other languages transitioning to Python
- Professional developers seeking to write more maintainable Python code
- Technical team leads making architectural decisions for Python projects
- Computer science students studying advanced programming paradigms
Prerequisites include basic proficiency with Python syntax and some experience with fundamental OOP concepts. While not strictly required, exposure to OOP in other languages provides helpful context for the comparisons made throughout the book.
Why This Book Stands Out: A Balanced Approach
Unlike many programming books that focus exclusively on either theory or code examples, "Advanced Object-Oriented Programming in Python" strikes a perfect balance. It presents:
- Theoretical foundations with practical, real-world applications
- Pythonic approaches rather than patterns transplanted from other languages
- Guidelines for when to use different OOP techniques (and when not to)
- Modern best practices reflecting current Python development standards
- Both the "how" and the "why" behind OOP design decisions
The book's greatest strength is its nuanced perspective on OOP. Rather than presenting object-orientation as a one-size-fits-all solution, it acknowledges trade-offs and encourages thoughtful application of OOP principles to solve real-world problems effectively.
Real-World Applications: Beyond Theory
Throughout the book, examples are drawn from diverse domains:
- Web application development
- Data processing pipelines
- Financial systems
- Game development
- Content management systems
- API design
This variety helps readers connect abstract principles to their specific field, demonstrating OOP's broad applicability across different types of software.
Code examples illustrate practical scenarios developers encounter regularly:
- Implementing extensible frameworks
- Building flexible component systems
- Creating reusable libraries
- Modeling complex domain relationships
- Designing testable architectures
These examples go beyond simplistic demonstrations to show how OOP addresses genuine development challenges.
Modern Python Features
The book stays current with Python's evolution, incorporating modern features and best practices:
- Type hints for improved code clarity and IDE support
- Context managers for resource management
- Dataclasses for concise class definitions
- Proper package structures for OOP projects
- Integration with popular frameworks and libraries
While leveraging these modern features, the book maintains compatibility with Python 3.6+ and notes when specific features require newer versions.
Reader Testimonials: Impact and Results
Developers who've applied the book's teachings report significant improvements in their Python projects:
"This book transformed how I design Python applications. The chapters on composition and SOLID principles helped me refactor a monolithic codebase into manageable, testable components." - Senior Developer at a fintech company
"The explanation of metaclasses finally made this advanced topic click for me. I was able to implement a plugin system that would have been impossible with my previous knowledge." - Python backend developer
"As someone coming from Java, this book helped me understand when to embrace Python's dynamic nature and when to add more structure. My code is now more Pythonic but still maintains the robustness I need." - Software architect transitioning teams
Conclusion: An Essential Resource for Python Developers
"Advanced Object-Oriented Programming in Python" stands as an essential resource for developers seeking to master OOP in Python. Its comprehensive coverage, from fundamental concepts to advanced techniques, makes it suitable for both structured learning and ongoing reference.
The book's greatest strength is its balanced approach—respecting object-oriented principles while adapting them to Python's unique character. Rather than forcing patterns from other languages, it shows how to leverage Python's dynamic nature and "batteries included" philosophy to create elegant object-oriented solutions.
For Python developers looking to write more maintainable, scalable code, this book represents a valuable investment in professional growth. It bridges the gap between knowing Python syntax and designing robust systems, providing both theoretical knowledge and practical techniques that apply immediately to real-world development challenges.
Whether used as a structured learning path or a reference for specific OOP challenges, this book deserves a place on every serious Python developer's bookshelf.
Keywords and SEO Terms:
Python OOP, advanced object-oriented programming, Python inheritance, composition Python, design patterns Python, SOLID principles, Python metaclasses, Python special methods, Python ABCs, object-oriented best practices, Python class design, Python mixins, scalable Python architecture, Python SOLID, Python code organization
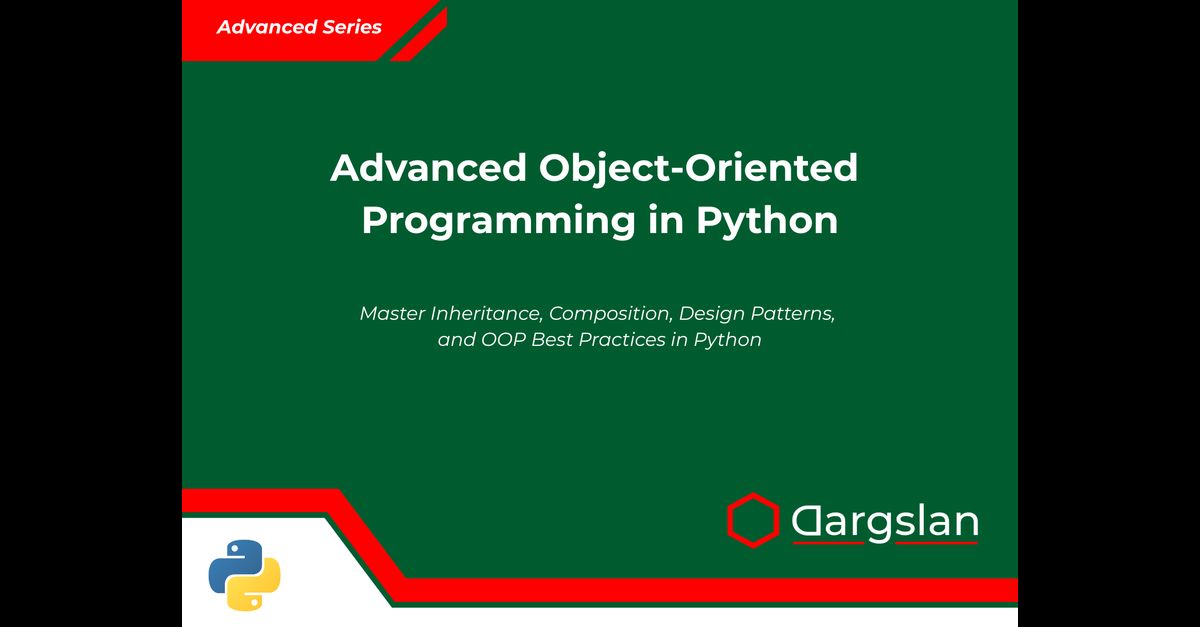
Advanced Object-Oriented Programming in Python