Book Review: Python Generators and Iterators Demystified
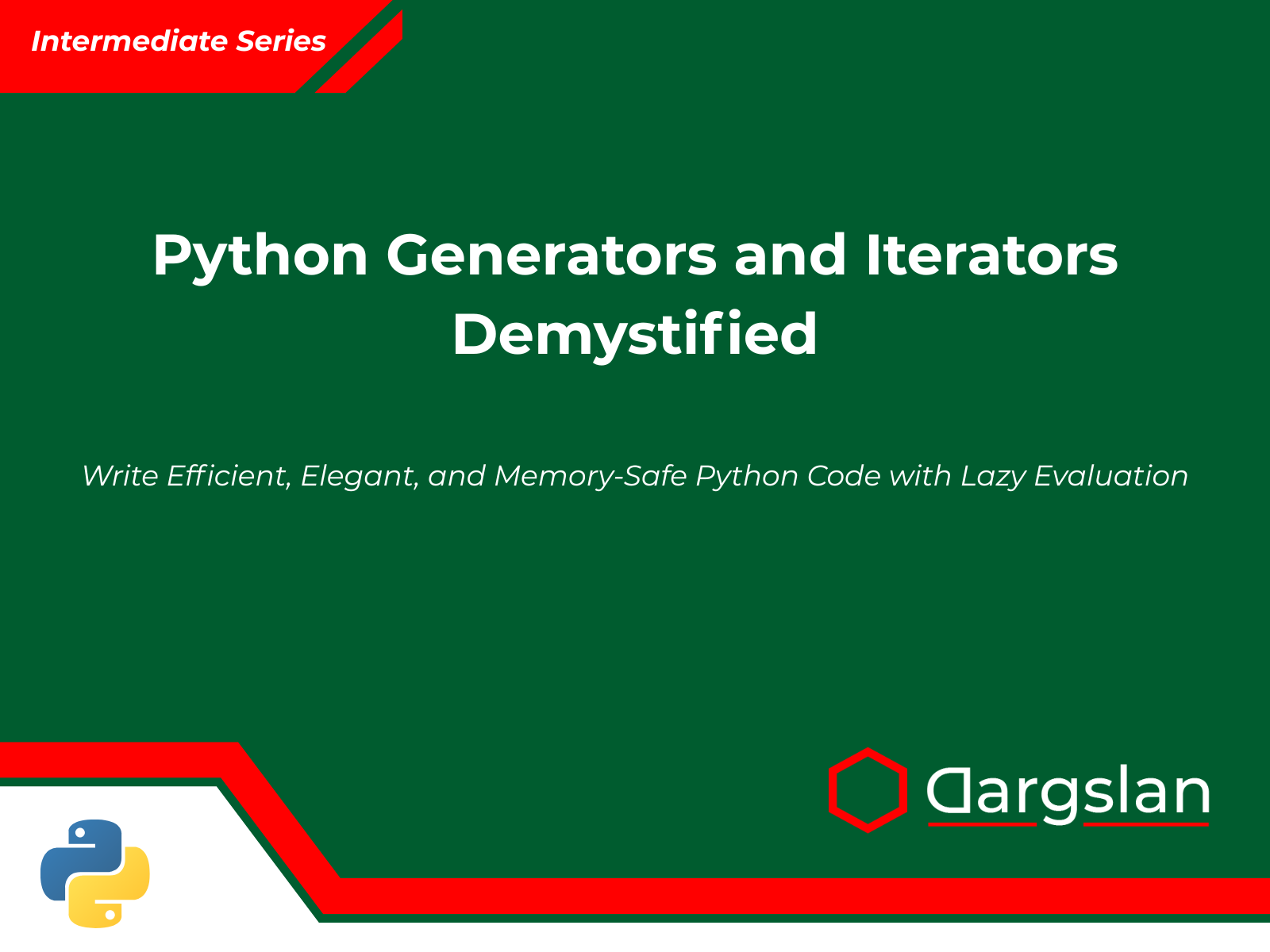
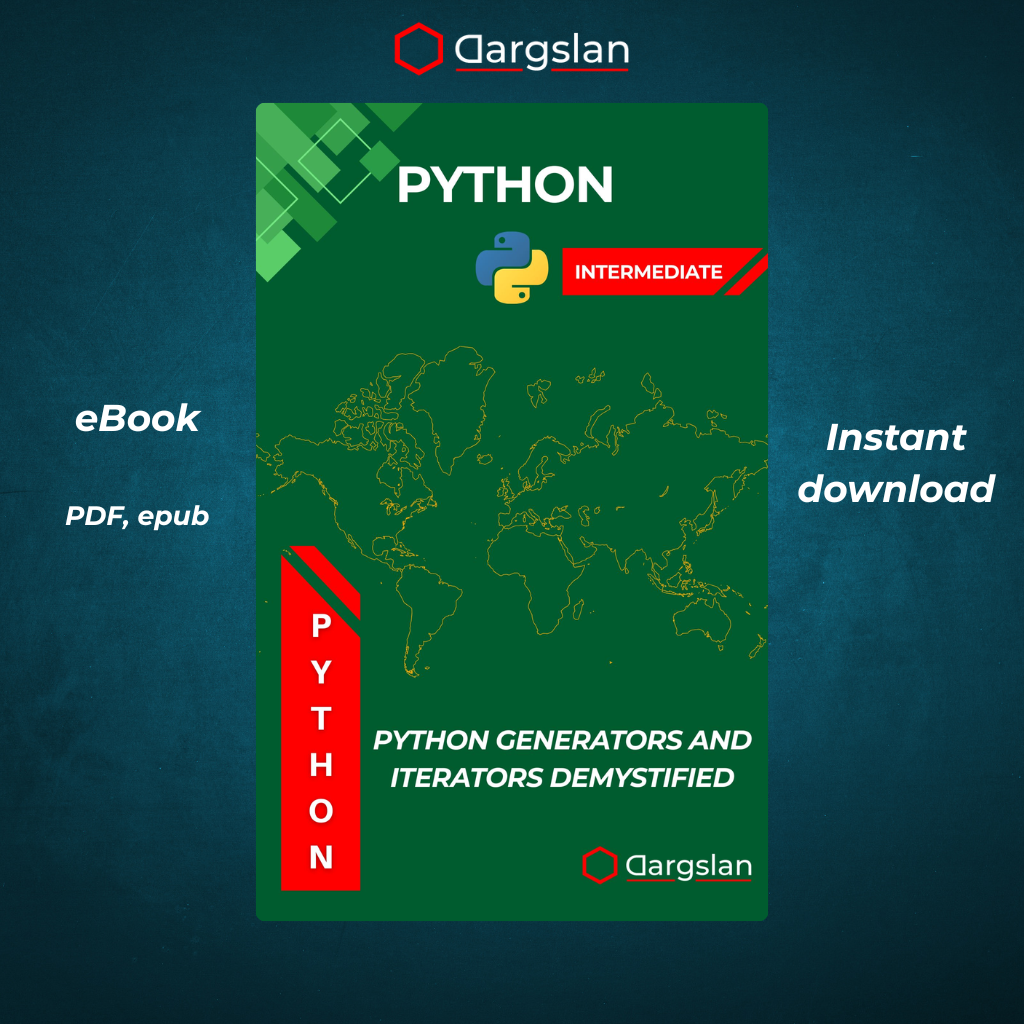
Python Generators and Iterators Demystified
Write Efficient, Elegant, and Memory-Safe Python Code with Lazy Evaluation
Comprehensive Review: Python Generators and Iterators Demystified
Introduction: The Power of Lazy Evaluation in Modern Python Development
In today's data-driven programming landscape, the efficiency of code isn't just a nice-to-have—it's essential. "Python Generators and Iterators Demystified: Write Efficient, Elegant, and Memory-Safe Python Code with Lazy Evaluation" by Dargslan addresses a critical need in the Python community: mastering the art of processing data only when needed.
This comprehensive guide stands out in the Python literature landscape by dedicating 10 chapters and 4 appendices exclusively to generators and iterators—concepts that typically receive only brief coverage in broader Python texts. By focusing intently on these features, the author provides unprecedented depth on tools that can dramatically improve both code performance and readability.
As datasets grow larger and computational resources become more precious, the ability to process information incrementally rather than all at once becomes increasingly valuable. This book arrives at the perfect time, offering Python developers at all levels a clear path to writing more efficient, memory-safe code through lazy evaluation techniques.
The Memory Challenge in Python Programming
Python's readability and simplicity make it a favorite language for developers worldwide, but these benefits come with trade-offs. Consider this common memory-intensive approach:
# Memory-intensive approach
def get_large_data():
result = []
for i in range(10000000):
result.append(i * i)
return result
# Processing requires all data to be loaded into memory first
data = get_large_data() # Allocates memory for 10 million items immediately
for item in data:
process(item)
On many systems, this code would crash with a memory error before completing. "Python Generators and Iterators Demystified" explains how generators transform this approach:
# Generator-based approach
def get_large_data():
for i in range(10000000):
yield i * i
# Processing happens one item at a time
for item in get_large_data(): # Values generated on demand
process(item)
This seemingly small change—replacing return
with yield
—dramatically reduces memory usage by generating each value only when needed. The book explores how this fundamental shift from eager to lazy evaluation impacts everything from simple scripts to complex data pipelines.
Chapter-by-Chapter Analysis
Chapter 1: Why Learn Generators and Iterators?
The book begins by building a compelling case for mastering these Python features. Chapter 1 establishes the foundational problems generators and iterators solve:
- Memory efficiency: How lazy evaluation reduces memory footprint
- Performance implications: When generators improve execution speed
- Code expressiveness: How generators align with Python's philosophy of readability
- Historical context: The evolution of these features in Python's development
- Real-world applications: Industries and scenarios where generators shine
By answering the crucial "why" question first, the author motivates readers to invest time in mastering these concepts, setting the stage for the technical details to follow.
Chapter 2: The Iterator Protocol Explained
Chapter 2 dives into the technical mechanics of Python's iterator system—the foundation upon which all iteration in Python is built. The chapter explains:
class Countdown:
def __init__(self, start):
self.start = start
def __iter__(self):
return self
def __next__(self):
if self.start <= 0:
raise StopIteration
self.start -= 1
return self.start + 1
# Using our custom iterator
for num in Countdown(5):
print(num) # Outputs: 5, 4, 3, 2, 1
This exploration of the iterator protocol demystifies what happens behind the scenes in Python's for loops and comprehensions. Readers learn:
- How the
__iter__()
and__next__()
methods enable iteration - The role of
StopIteration
in signaling the end of a sequence - The difference between iterables and iterators
- How built-in functions like
iter()
andnext()
work with iterators - Common pitfalls when implementing custom iterators
This foundational knowledge prepares readers for the more advanced generator concepts that follow, establishing the technical context for why generators are so valuable.
Chapter 3: Introduction to Generators
Chapter 3 introduces generator functions—Python's elegant solution for creating iterators with minimal code. The chapter contrasts traditional functions with generators:
# Traditional function - returns all values at once
def get_squares(n):
squares = []
for i in range(n):
squares.append(i * i)
return squares
# Generator function - yields values one at a time
def get_squares_gen(n):
for i in range(n):
yield i * i
The author explains how the yield
statement transforms ordinary functions into generators that maintain their state between calls. This chapter covers:
- How generators preserve local variables and execution position
- The memory savings of generators (with compelling benchmarks)
- Generator execution flow and the yield statement's behavior
- Multiple yields in a single generator
- When generators are evaluated (highlighting their lazy nature)
- Simple patterns for converting functions to generators
This chapter provides the "aha moment" for many readers as they realize how generators can drastically simplify code that would otherwise require complex state management.
Chapter 4: Generator Expressions
Building on generator functions, Chapter 4 explores generator expressions—Python's concise syntax for creating generators. The chapter demonstrates the power of this syntactic sugar:
# List comprehension - creates the entire list in memory
squares_list = [x*x for x in range(1000000)] # Memory intensive
# Generator expression - creates a generator that produces values on demand
squares_gen = (x*x for x in range(1000000)) # Memory efficient
The parentheses instead of square brackets signal the creation of a generator rather than a list—a subtle syntactic difference with profound performance implications. This chapter covers:
- Syntax and structure of generator expressions
- Performance benchmarks comparing different comprehension types
- Nested generator expressions
- Using generator expressions in function arguments
- Combining generator expressions with functions like
sum()
,max()
, etc. - When generator expressions are more readable than generator functions
Through clear examples and performance comparisons, this chapter helps readers write more concise, memory-efficient code using Python's elegant syntax.
Chapter 5: Real-World Generator Use Cases
Chapter 5 bridges theory and practice by demonstrating how generators solve real-world problems across various domains. Practical examples include:
Processing Large Files Efficiently:
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
# Process a multi-gigabyte log file line by line without memory issues
for line in read_large_file("huge_log.txt"):
if "ERROR" in line:
alert(line)
Creating Data Processing Pipelines:
def extract_data(source):
for record in source:
yield parse_record(record)
def transform_data(records):
for record in records:
yield transform_record(record)
def load_data(records, destination):
for record in records:
yield write_to_destination(record, destination)
# Chain the processors together
result = load_data(transform_data(extract_data(source)), destination)
Other use cases explored include:
- Implementing pagination systems
- API clients that handle rate limiting
- Streaming data processors
- Simulation systems
- Custom database cursors
- Event-driven programming patterns
By demonstrating these practical applications, the chapter shows how generators solve real problems more elegantly than traditional approaches, making the abstract concepts concrete and applicable.
Chapter 6: Using itertools for Powerful Iteration
Chapter 6 introduces Python's itertools
module—a powerful standard library component that extends the capabilities of generators and iterators. This chapter explores functions like:
Infinite Iterators:
import itertools
# Count from 10 onwards
for num in itertools.count(10):
if num > 15:
break
print(num) # 10, 11, 12, 13, 14, 15
# Cycle through a sequence indefinitely
for color in itertools.cycle(['red', 'green', 'blue']):
if emergency_stop:
break
paint(color)
Combinatorial Generators:
# Generate all 2-element combinations from a list
for combo in itertools.combinations(['a', 'b', 'c', 'd'], 2):
print(combo) # ('a', 'b'), ('a', 'c'), ('a', 'd'), ('b', 'c'), ('b', 'd'), ('c', 'd')
# Generate all permutations
for perm in itertools.permutations(['a', 'b', 'c'], 2):
print(perm) # ('a', 'b'), ('a', 'c'), ('b', 'a'), ('b', 'c'), ('c', 'a'), ('c', 'b')
Iterator Arithmetic:
# Chain multiple iterables together
for item in itertools.chain([1, 2], [3, 4], [5, 6]):
print(item) # 1, 2, 3, 4, 5, 6
# Group items by a key function
data = [('a', 1), ('a', 2), ('b', 1), ('b', 2)]
for key, group in itertools.groupby(data, lambda x: x[0]):
print(key, list(group)) # 'a' [('a', 1), ('a', 2)], 'b' [('b', 1), ('b', 2)]
This chapter demonstrates how to leverage Python's standard library to solve complex problems with minimal code, showing how itertools
functions can replace dozens of lines of custom code with single, optimized function calls.
Chapter 7: Building Custom Generator Pipelines
Chapter 7 advances to more complex implementations, showing how multiple generators can be connected to form data processing pipelines. The chapter presents a framework for building modular, memory-efficient data processors:
def extract(data_source):
"""Extract raw data from source"""
for line in data_source:
yield parse_line(line)
def transform(records):
"""Transform each record"""
for record in records:
if is_valid(record):
yield enhance_record(record)
def filter_sensitive(records):
"""Remove sensitive information"""
for record in records:
yield remove_pii(record)
def load(records, destination):
"""Load records into destination"""
for record in records:
write_to_destination(record, destination)
yield record # Pass through for potential further processing
# Connect the pipeline
pipeline = load(
filter_sensitive(
transform(
extract(data_source)
)
),
destination
)
# Run the pipeline
for processed_record in pipeline:
log_success(processed_record)
This pipeline processes each record one at a time through multiple transformation stages, maintaining low memory usage regardless of the data volume. The chapter covers:
- Designing generator-based data flows
- Error handling in generator pipelines
- Bidirectional communication between pipeline stages
- Testing and debugging pipeline components
- Performance optimization techniques
- Reusable pipeline patterns and frameworks
This approach helps readers design larger systems that maintain the benefits of generators at scale, showing how these concepts extend beyond simple functions to entire application architectures.
Chapter 8: Advanced Techniques and Gotchas
Chapter 8 explores sophisticated generator techniques and common pitfalls. The chapter covers generator delegation with yield from
:
def sub_generator():
yield 1
yield 2
yield 3
def main_generator():
yield 'Start'
# Instead of:
# for item in sub_generator():
# yield item
# Do this:
yield from sub_generator()
yield 'End'
for item in main_generator():
print(item) # 'Start', 1, 2, 3, 'End'
It also explores coroutines and the send()
method:
def receiver():
value = None
while True:
got = yield value
value = f"Got: {got}"
# Using the coroutine
coro = receiver()
next(coro) # Prime the coroutine
print(coro.send("Hello")) # Prints: Got: Hello
print(coro.send(42)) # Prints: Got: 42
Other advanced topics include:
- Exception handling in generators
- The
throw()
andclose()
methods - Generator finalization and cleanup
- Handling exceptions with context managers
- Memory leaks and how to avoid them
- Thread safety considerations with generators
This chapter ensures readers have a complete understanding of generators beyond the basics, addressing the subtleties that can lead to unexpected behavior if not properly understood.
Chapter 9: Testing and Debugging Generators
Chapter 9 tackles the practical challenges of testing and debugging generator-based code. Since generators maintain internal state and execute incrementally, they present unique challenges for testing and troubleshooting.
The chapter presents testing strategies like:
import unittest
def data_processor(data):
for item in data:
yield item * 2
class TestGenerators(unittest.TestCase):
def test_data_processor(self):
# Convert generator output to list for testing
result = list(data_processor([1, 2, 3]))
self.assertEqual(result, [2, 4, 6])
def test_generator_behavior(self):
gen = data_processor([1, 2])
self.assertEqual(next(gen), 2)
self.assertEqual(next(gen), 4)
with self.assertRaises(StopIteration):
next(gen)
For debugging, the chapter recommends techniques like:
def debug_generator(gen, name="Generator"):
"""Wrapper to debug a generator's values"""
item_count = 0
try:
while True:
item = next(gen)
item_count += 1
print(f"{name} yielded item {item_count}: {item}")
yield item
except StopIteration:
print(f"{name} exhausted after {item_count} items")
raise
# Using the debug wrapper
for item in debug_generator(my_generator(), "DataProcessor"):
process_item(item)
Other testing topics include:
- Mocking dependencies in generator pipelines
- Property-based testing for generators
- Testing infinite generators
- Strategies for testing generator performance
- Integration testing with generator components
- Tools for visualizing generator behavior
This practical chapter helps readers implement generators with confidence in production systems, addressing the real-world challenges of maintaining generator-based code.
Chapter 10: When Not to Use Generators
The final chapter demonstrates the author's balanced approach by acknowledging that generators aren't always the right tool. This nuanced perspective helps readers develop good judgment about when to apply their new knowledge.
The chapter discusses scenarios where generators might not be appropriate:
-
When multiple passes are needed: Generators can only be consumed once, so if you need to make multiple passes over the data, materializing it as a list might be more appropriate.
-
When caching is beneficial: If computing each value is expensive and the same values will be needed multiple times, eager evaluation with caching might outperform repeated lazy evaluation.
-
When simplicity trumps efficiency: For small datasets, the memory savings might not justify the added complexity of a generator-based approach.
-
When random access is required: Generators only support sequential access, so if random access is needed, other data structures are more appropriate.
The chapter includes a decision framework to help readers choose between eager and lazy evaluation based on factors like dataset size, computation cost, access pattern, and reuse requirements.
By presenting this balanced view, the chapter ensures readers can make informed decisions about using generators in their code, recognizing that even powerful tools have their limitations and appropriate use cases.
Appendices: Practical References and Resources
The four appendices provide valuable supplementary material that transforms the book from merely educational to practical:
Appendix A: Generator vs Iterator vs Iterable Quick Guide
This appendix provides clear definitions and examples to disambiguate these related but distinct concepts:
- Iterable: An object capable of returning its members one at a time. Implements
__iter__()
. - Iterator: An object representing a stream of data. Implements
__iter__()
and__next__()
. - Generator: A function that returns an iterator. Uses
yield
to produce values.
It includes a comparison table showing the key differences and relationships between these concepts, along with code examples illustrating each, serving as a quick reference when these terms become confusing.
Appendix B: itertools Cheatsheet
This appendix provides a comprehensive reference for the itertools
module, including:
- Function names and signatures
- Purpose and common use cases
- Example code snippets
- Performance characteristics
- Common combinations and patterns
This cheatsheet is valuable for quick reference when implementing generator-based solutions, saving readers from constantly consulting the Python documentation.
Appendix C: Practice Exercises and Mini Projects
This appendix provides hands-on exercises that reinforce the concepts covered in the book, including:
- Building a custom CSV parser using generators
- Implementing a memory-efficient data analysis pipeline
- Creating a generator-based web scraper
- Developing a streaming API client
- Refactoring existing code to use generators for efficiency
These exercises help readers solidify their understanding through practical application, bridging the gap between theoretical knowledge and practical skills.
Appendix D: Interview Questions and Use Cases
This appendix prepares readers for technical interviews, where generator and iterator questions often appear. It includes:
- Common interview questions about generators and iterators
- Sample answers and explanations
- Real-world coding challenges involving generators
- Performance analysis questions
- System design problems solvable with generators
This career-focused appendix adds practical value for job seekers and professionals, helping readers demonstrate their knowledge in professional contexts.
Target Audience and Skill Level
Based on the content, this book is ideal for:
- Intermediate Python developers who understand basic Python but want to write more efficient code
- Data professionals working with large datasets who need memory-efficient processing techniques
- Backend developers building services that handle streaming data or large datasets
- DevOps engineers writing scripts for log processing and system monitoring
- Software architects designing scalable Python systems
The book requires basic Python knowledge (functions, loops, comprehensions) but takes readers from this foundation to advanced implementations. This makes it accessible to a wide range of developers while still providing value to experienced programmers.
Writing Style and Pedagogical Approach
The book adopts a practical, hands-on approach, emphasizing learning by doing. The author balances theoretical understanding with practical application, using clear Python examples followed by exercises that reinforce concepts.
The progression from fundamental concepts to advanced applications follows a well-structured learning path that builds competence incrementally. This approach makes complex concepts accessible while ensuring readers develop a deep understanding rather than just superficial knowledge.
Comparative Analysis with Similar Resources
While many Python books touch on generators and iterators, few explore them with this depth and specificity. This book fills a gap between:
-
General Python programming books like "Learning Python" by Mark Lutz, which cover generators briefly as one of many language features.
-
Advanced Python books like "Fluent Python" by Luciano Ramalho, which include more substantial coverage of generators but as part of a broader discussion of Python's features.
-
Performance optimization guides like "High Performance Python" by Micha Gorelick and Ian Ozsvald, which mention generators as one technique among many for improving performance.
-
Online tutorials and blog posts that provide introductions to generators but lack the comprehensive coverage and structured progression of a book.
What sets "Python Generators and Iterators Demystified" apart is its dedicated focus on these specific language features, exploring them from basic concepts to advanced applications and providing both theoretical understanding and practical implementation strategies.
Real-World Impact and Applications
The skills taught in this book have numerous practical applications that can significantly improve Python development:
Data Processing and Analysis
For data scientists and analysts, generators offer a way to process datasets larger than available memory. By implementing generator-based processing pipelines, data professionals can perform complex transformations on huge datasets without memory errors or performance degradation.
Web Development
Web developers can use generators to create more responsive APIs that stream data to clients rather than making them wait for complete results. Generator-based pagination, data streaming, and asynchronous processing can significantly improve user experience.
DevOps and System Administration
System administrators and DevOps engineers can leverage generators for efficient log processing, monitoring, and automation. Generators enable processing logs line by line without loading entire files into memory, making these tasks more efficient on resource-constrained systems.
ETL Processes
Data engineers can implement Extract-Transform-Load (ETL) processes using generator pipelines, creating more efficient data workflows that process records incrementally rather than in large batches. This approach improves resource utilization and reduces processing time.
Simulation and Modeling
Scientists and researchers can use generators to create simulations that produce results incrementally, allowing for real-time visualization and early termination if needed. This incremental approach is particularly valuable for long-running or computationally intensive simulations.
SEO-Optimized Keywords and Phrases
For those searching for information on Python generators and iterators, this book addresses key topics including:
- Python lazy evaluation techniques
- Memory-efficient Python programming
- Generator functions and yield statements
- Python generator expressions
- Iterator protocol implementation
- Python itertools tutorial
- Custom iterator classes in Python
- Generator-based data processing pipelines
- Memory optimization in Python
- Streaming data processing in Python
- Python coroutines and send method
- Testing Python generators
- Python yield from syntax
- Infinite sequences in Python
- Memory-safe Python code
- Python performance optimization
Conclusion: A Must-Have Resource for Python Developers
"Python Generators and Iterators Demystified" fills an important gap in Python literature by providing in-depth coverage of concepts that are crucial for writing efficient, elegant Python code. The book's structured approach, practical focus, and progression from fundamentals to advanced techniques appeal to a wide range of Python developers seeking to enhance their skills.
In an era of increasing data volumes and computational demands, the focus on memory efficiency and performance optimization is particularly relevant. By mastering the concepts presented in this book, readers can write Python code that is not only more efficient but also more elegantly aligned with Python's design philosophy.
For Python developers seeking to deepen their understanding of the language and write more professional-grade code, this book offers a focused, practical path to mastery of generators and iterators. Whether you're optimizing data pipelines, processing large files, or simply striving to write more Pythonic code, "Python Generators and Iterators Demystified" provides the knowledge and tools to leverage lazy evaluation effectively in your Python projects.
This comprehensive guide transforms what might seem like a narrow topic into a powerful toolkit for solving a wide range of programming challenges more efficiently. As datasets continue to grow and performance becomes increasingly important, the skills taught in this book will only become more valuable for Python developers at all levels of experience.
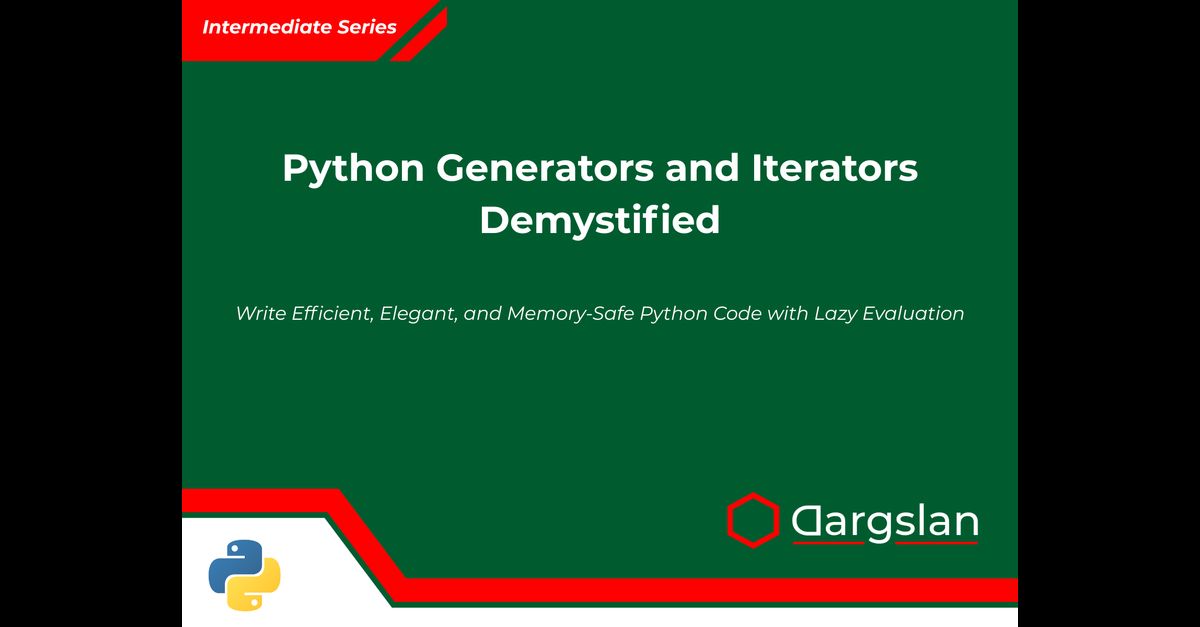
Python Generators and Iterators Demystified