Book Review: Reactive Programming in JavaScript
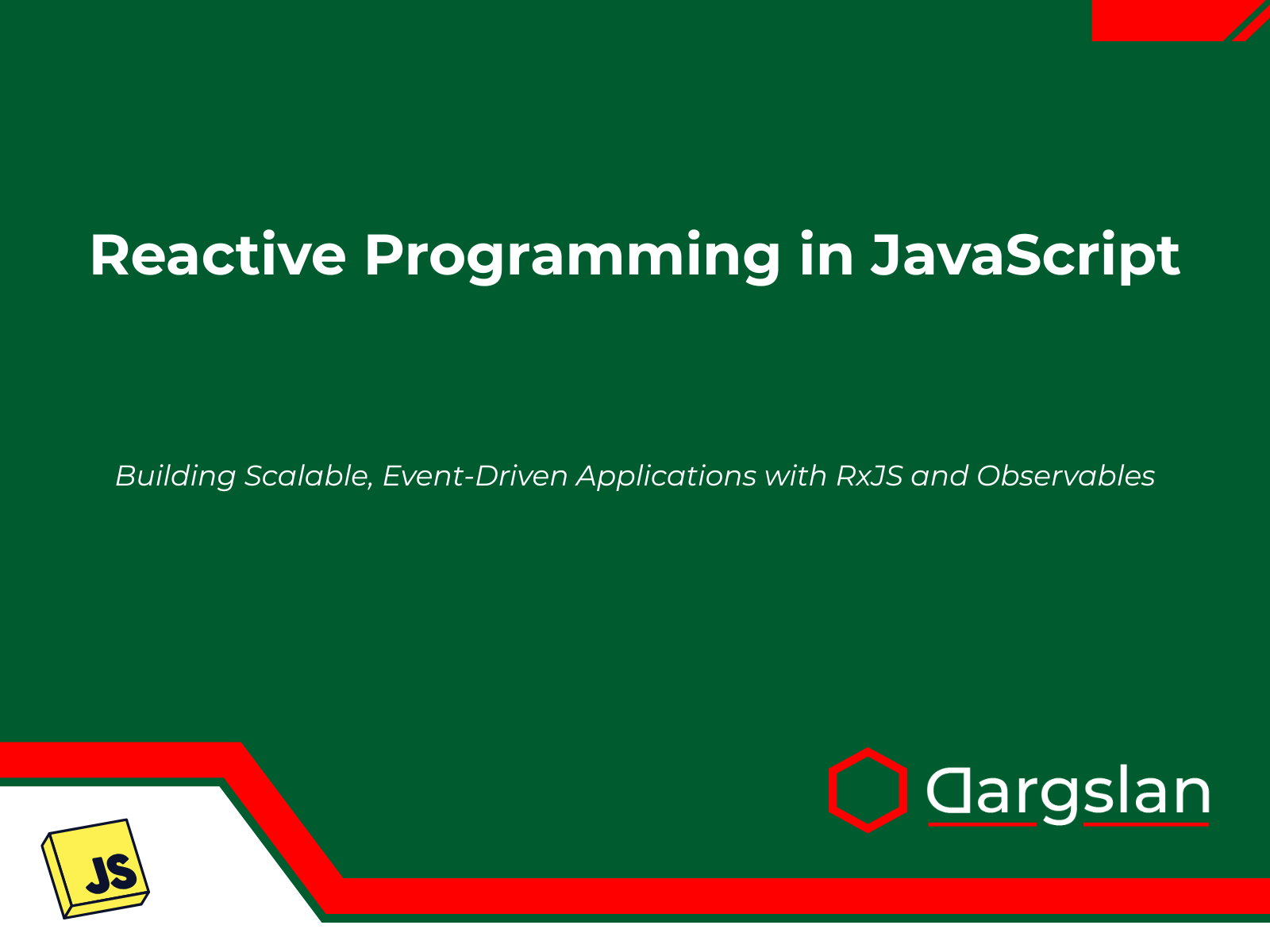
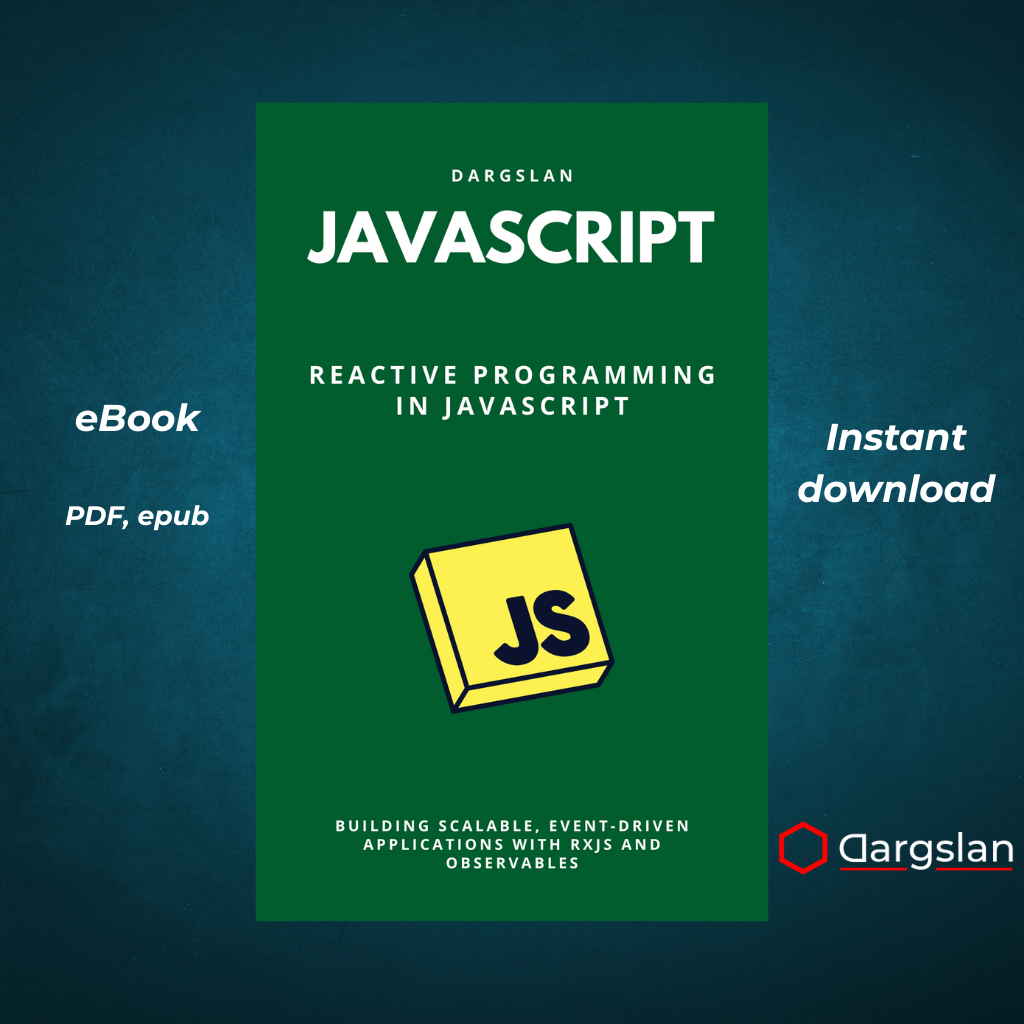
Reactive Programming in JavaScript
Building Scalable, Event-Driven Applications with RxJS and Observables
Reactive Programming in JavaScript: Building Scalable, Event-Driven Applications with RxJS and Observables
Comprehensive Book Preview
A detailed guide to mastering reactive programming principles, implementing RxJS, and building responsive web applications in JavaScript
Introduction: The Power of Reactive Programming in Modern JavaScript
In today's fast-paced digital landscape, web applications face unprecedented demands. Users expect real-time updates, seamless interactions, and lightning-fast responses. Traditional imperative programming approaches often struggle to meet these requirements efficiently, especially when handling complex asynchronous operations, event-driven architectures, and multiple data streams.
Reactive Programming represents a paradigm shift that addresses these challenges head-on. By focusing on asynchronous data streams and the propagation of changes, reactive programming offers a powerful model for building applications that are more responsive, resilient, and scalable. At its core, it's about creating systems that react elegantly to events, user inputs, and changing data.
JavaScript, as the language of the web, is uniquely positioned to benefit from reactive programming techniques. Its event-driven nature and asynchronous capabilities make it an ideal environment for implementing reactive patterns. Combined with RxJS (Reactive Extensions for JavaScript), developers can leverage a comprehensive toolkit to create applications that handle complex data flows with elegance and precision.
Who This Book Is For
"Reactive Programming in JavaScript" is designed for:
- JavaScript developers looking to level up their asynchronous programming skills
- Front-end engineers building complex, interactive UIs that respond to multiple events
- Back-end Node.js developers managing concurrent operations and data streams
- Full-stack developers seeking a unified approach to handling reactivity across the stack
- Senior developers and architects designing scalable application architectures
- React, Angular, or Vue developers wanting to understand the reactive principles underlying modern frameworks
Whether you're already familiar with concepts like Promises and async/await or you're just beginning to explore JavaScript's asynchronous capabilities, this book will guide you through the reactive programming landscape with clear explanations and practical examples.
Chapter-by-Chapter Preview
Chapter 1: Introduction to Reactive Programming
The journey begins with a comprehensive introduction to reactive programming as a paradigm. This chapter explores:
- The core principles of reactive programming: responsive, resilient, elastic, and message-driven
- How reactive programming differs from imperative, functional, and object-oriented approaches
- The Observer pattern and its role as the foundation of reactive systems
- The ReactiveX project and its implementation across multiple languages
- Real-world scenarios where reactive programming shines
Key Takeaway: Understand why reactive programming has become essential for modern applications that deal with real-time data, user events, and complex asynchronous workflows.
// Traditional imperative approach
let button = document.querySelector('button');
let counter = 0;
let counterElement = document.querySelector('#counter');
button.addEventListener('click', () => {
counter++;
counterElement.textContent = counter;
});
// Versus reactive approach (preview)
const clicks$ = fromEvent(button, 'click');
const counter$ = clicks$.pipe(
scan(count => count + 1, 0)
);
counter$.subscribe(count => {
counterElement.textContent = count;
});
Chapter 2: Why Reactive JavaScript?
This chapter makes the case for adopting reactive programming specifically in JavaScript:
- JavaScript's event-driven nature aligns perfectly with reactive principles
- The evolution from callbacks to Promises to async/await to Observables
- The limitations of Promise-based code when dealing with multiple events or cancellation
- How reactive programming helps manage the complexity of modern web applications
- The JavaScript ecosystem's embrace of reactive patterns
Key Takeaway: Learn why JavaScript particularly benefits from reactive programming and how it addresses common pain points in asynchronous JavaScript development.
// Promise-based approach (one-time event)
fetchUserData()
.then(user => fetchUserOrders(user.id))
.then(orders => displayOrders(orders))
.catch(error => handleError(error));
// Observable-based approach (handles ongoing events)
const userData$ = fromFetch('/api/user').pipe(
switchMap(response => response.json())
);
const userOrders$ = userData$.pipe(
switchMap(user => fromFetch(`/api/orders/${user.id}`).pipe(
switchMap(response => response.json())
))
);
userOrders$.subscribe({
next: orders => displayOrders(orders),
error: error => handleError(error)
});
Chapter 3: JavaScript Foundations for Reactivity
Before diving deep into reactive libraries, this chapter ensures you have the necessary JavaScript fundamentals:
- Advanced asynchronous JavaScript: Promises, async/await, and their limitations
- Event handling fundamentals and the browser event loop
- Functions as first-class citizens and higher-order functions
- Closures and their importance in creating reactive pipelines
- JavaScript generators and iterators as precursors to reactive streams
Key Takeaway: Master the JavaScript fundamentals that form the building blocks of reactive programming, ensuring you have a solid foundation for the concepts ahead.
// Understanding generators as primitive streams
function* countGenerator() {
let i = 0;
while (true) {
yield i++;
}
}
const counter = countGenerator();
console.log(counter.next().value); // 0
console.log(counter.next().value); // 1
console.log(counter.next().value); // 2
// Closure in reactive context
function createMultiplier(factor) {
return function(stream$) {
return stream$.pipe(map(value => value * factor));
}
}
const double = createMultiplier(2);
const tripleStream$ = double(of(1, 2, 3));
Chapter 4: Getting Started with RxJS
This chapter introduces RxJS, the most popular reactive programming library for JavaScript:
- Setting up your development environment for RxJS
- Understanding the core RxJS classes: Observable, Observer, Subscription
- The RxJS operator model and method chaining with pipe()
- Marble diagrams and how to read them
- Creating your first RxJS applications
Key Takeaway: Get comfortable with the RxJS library, its core concepts, and how to set up your first reactive JavaScript project.
// Basic RxJS setup and usage
import { Observable } from 'rxjs';
const observable = new Observable(subscriber => {
subscriber.next('Hello');
subscriber.next('World');
setTimeout(() => {
subscriber.next('Async Value');
subscriber.complete();
}, 1000);
});
console.log('Subscription starting...');
observable.subscribe({
next: value => console.log(value),
error: err => console.error(err),
complete: () => console.log('Done!')
});
console.log('Subscription set up');
// Output:
// Subscription starting...
// Hello
// World
// Subscription set up
// Async Value (after 1 second)
// Done! (after 1 second)
Chapter 5: Creating and Subscribing to Observables
This chapter dives deeper into the various ways to create Observables and work with subscriptions:
- Observable creation methods: of(), from(), fromEvent(), interval(), timer()
- Custom Observables using the Observable constructor
- Understanding hot vs. cold Observables
- Subscription management and preventing memory leaks
- The importance of the unsubscribe() method
Key Takeaway: Master the various ways to create data streams and properly manage subscriptions to prevent memory leaks.
// Creating Observables from different sources
import { of, from, fromEvent, interval } from 'rxjs';
// From discrete values
of(1, 2, 3).subscribe(console.log);
// From an array
from([4, 5, 6]).subscribe(console.log);
// From a Promise
from(fetch('https://api.github.com/users/octocat')
.then(response => response.json()))
.subscribe(console.log);
// From DOM events
const clicks$ = fromEvent(document, 'click');
const subscription = clicks$.subscribe(event =>
console.log('Clicked at:', event.clientX, event.clientY)
);
// Cleanup when done
setTimeout(() => {
subscription.unsubscribe();
console.log('Unsubscribed from clicks');
}, 5000);
Chapter 6: Transforming Data with Operators
This chapter explores how to transform, filter, and manipulate data streams:
- Understanding operator categories: creation, transformation, filtering, combination
- Essential transformation operators: map, switchMap, mergeMap, concatMap
- Filtering streams with filter, take, takeUntil, distinct, distinctUntilChanged
- Time-based operations with debounceTime, throttleTime, delay
- Creating operator chains for complex data processing
Key Takeaway: Learn to manipulate data streams effectively, composing complex operations from simple building blocks.
import { fromEvent } from 'rxjs';
import {
map,
filter,
debounceTime,
distinctUntilChanged,
switchMap
} from 'rxjs/operators';
// Building a search feature with operators
const searchInput = document.querySelector('#search');
const searchResults = document.querySelector('#results');
fromEvent(searchInput, 'input').pipe(
map(event => event.target.value),
filter(text => text.length > 2),
debounceTime(300),
distinctUntilChanged(),
switchMap(searchTerm =>
fetch(`https://api.example.com/search?q=${searchTerm}`)
.then(response => response.json())
)
).subscribe({
next: results => {
searchResults.innerHTML = '';
results.forEach(item => {
const element = document.createElement('div');
element.textContent = item.title;
searchResults.appendChild(element);
});
},
error: err => {
searchResults.innerHTML = `Error: ${err.message}`;
}
});
Chapter 7: Error Handling and Retry Logic
This chapter focuses on building robust applications that gracefully handle failures:
- Error handling strategies in RxJS
- The catchError operator and error recovery
- Implementing retry logic with retry, retryWhen
- Exponential backoff strategies for API calls
- Notification patterns for communicating errors to users
Key Takeaway: Learn to create resilient applications that recover from failures and provide great user experiences even when things go wrong.
import { from, of, timer } from 'rxjs';
import {
catchError,
retry,
retryWhen,
delayWhen,
tap,
mergeMap
} from 'rxjs/operators';
function fetchData(id) {
return from(fetch(`https://api.example.com/data/${id}`)
.then(response => {
if (!response.ok) throw new Error('Server error');
return response.json();
}));
}
fetchData('123').pipe(
// Simple retry - try 3 times
retry(3),
// Error handling
catchError(err => {
logError(err);
return of({ error: true, message: 'Could not fetch data' });
})
).subscribe(data => updateUI(data));
// Advanced retry with exponential backoff
fetchData('456').pipe(
retryWhen(errors =>
errors.pipe(
// Log the error
tap(err => console.log('Error occurred:', err.message)),
// Keep track of retry count
scan((count, _) => count + 1, 0),
// Implement exponential backoff
mergeMap(count => {
// Maximum 4 retries
if (count >= 4) {
return throwError(() => new Error('Maximum retries reached'));
}
const delay = Math.pow(2, count) * 1000;
console.log(`Retrying after ${delay}ms`);
return timer(delay);
})
)
),
catchError(err => {
displayErrorNotification(err.message);
return of({ error: true });
})
).subscribe(updateUI);
Chapter 8: Combining Multiple Streams
This chapter teaches you how to work with multiple data sources effectively:
- Combination operators: combineLatest, merge, concat, zip, race
- Flattening strategies and when to use each one
- Building data pipelines that respond to multiple inputs
- Coordinating complex asynchronous workflows
- Practical examples of stream composition
Key Takeaway: Master the art of combining multiple data streams to solve complex real-world problems with elegant reactive code.
import { combineLatest, merge, forkJoin } from 'rxjs';
import { map, withLatestFrom } from 'rxjs/operators';
// Real-time dashboard example
const userProfile$ = fetchUserProfile(userId);
const stockPrices$ = getStockPriceUpdates();
const notifications$ = getNotificationStream();
// Combine user data with latest stock prices
const userStocks$ = combineLatest([
userProfile$,
stockPrices$
]).pipe(
map(([user, prices]) => {
return user.stocksOwned.map(stock => ({
symbol: stock.symbol,
shares: stock.shares,
currentPrice: prices[stock.symbol],
totalValue: stock.shares * prices[stock.symbol]
}));
})
);
// Merge all user-related notifications
const userActivity$ = merge(
userStocks$.pipe(map(stocks => ({ type: 'portfolio-update', data: stocks }))),
notifications$.pipe(map(note => ({ type: 'notification', data: note })))
);
// Fetch multiple resources in parallel and combine when all complete
const pageData$ = forkJoin({
user: userProfile$,
preferences: userPreferences$,
recentActivity: userActivity$.pipe(take(10))
});
pageData$.subscribe({
next: ({ user, preferences, recentActivity }) => {
renderDashboard(user, preferences, recentActivity);
},
error: err => showErrorPage(err)
});
Chapter 9: State Management with Observables
This chapter explores how to manage application state using reactive principles:
- Creating a simple state store with BehaviorSubject
- Implementing the Redux pattern with RxJS
- Handling derived state and computed properties
- State synchronization across components
- Performance considerations when working with state
Key Takeaway: Discover how reactive programming can simplify state management in your applications, providing a consistent and predictable way to handle changing data.
import { BehaviorSubject } from 'rxjs';
import { map, distinctUntilChanged } from 'rxjs/operators';
// Simple reactive state store
class Store {
constructor(initialState) {
this._state = new BehaviorSubject(initialState);
this.state$ = this._state.asObservable();
}
get value() {
return this._state.value;
}
updateState(partialState) {
this._state.next({
...this.value,
...partialState
});
}
select(selectorFn) {
return this.state$.pipe(
map(selectorFn),
distinctUntilChanged()
);
}
}
// Creating a todo application store
const todoStore = new Store({
todos: [],
filter: 'all',
loading: false
});
// Adding a todo
function addTodo(text) {
const newTodo = {
id: Date.now(),
text,
completed: false
};
todoStore.updateState({
todos: [...todoStore.value.todos, newTodo]
});
}
// Selecting specific parts of state
const pendingTodos$ = todoStore.select(state =>
state.todos.filter(todo => !todo.completed)
);
// UI updates automatically when state changes
pendingTodos$.subscribe(todos => {
renderPendingTodoCount(todos.length);
});
Chapter 10: Integrating RxJS in Real Projects
This chapter focuses on practical implementation in popular frameworks and libraries:
- Using RxJS with React: react-rxjs, rxjs-hooks
- Angular's deep integration with Observables
- Vue.js and RxJS: vuex-observable
- Node.js backend applications with RxJS
- Testing reactive code with marble testing
Key Takeaway: Learn how to effectively integrate reactive programming into your existing projects and frameworks, with practical patterns for common scenarios.
// React with RxJS example
import React, { useEffect, useState } from 'react';
import { interval } from 'rxjs';
import { map, takeUntil } from 'rxjs/operators';
function CounterComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
const counter$ = interval(1000).pipe(
map(i => i + 1)
);
const subscription = counter$.subscribe(value => {
setCount(value);
});
return () => subscription.unsubscribe();
}, []);
return <div>Counter: {count}</div>;
}
// Angular component example
@Component({
selector: 'weather-widget',
template: `
<div class="weather-card">
<div *ngIf="weather$ | async as weather; else loading">
<h3>{{weather.location}}</h3>
<p class="temperature">{{weather.temperature}}°C</p>
<p>{{weather.condition}}</p>
</div>
<ng-template #loading>Loading weather data...</ng-template>
</div>
`
})
export class WeatherWidgetComponent implements OnInit, OnDestroy {
private destroy$ = new Subject<void>();
weather$ = this.weatherService.getCurrentWeather().pipe(
takeUntil(this.destroy$)
);
constructor(private weatherService: WeatherService) {}
ngOnDestroy() {
this.destroy$.next();
this.destroy$.complete();
}
}
Chapter 11: Reactive Patterns and Anti-Patterns
This chapter examines proven patterns and common pitfalls in reactive programming:
- Best practices for Observable composition
- The "reactive loop" pattern for cyclical data flows
- Avoiding common mistakes: memory leaks, nested subscribes, and operator misuse
- Performance optimization techniques
- When reactive programming might not be the right solution
Key Takeaway: Develop an intuition for when and how to apply reactive programming effectively, while avoiding common pitfalls that lead to bugs and performance issues.
// Anti-pattern: Nested subscribes
searchTerms$.subscribe(term => {
fetchResults(term).subscribe(results => {
results.forEach(result => {
fetchDetails(result.id).subscribe(details => {
// Callback hell all over again!
displayResultWithDetails(result, details);
});
});
});
});
// Correct pattern: Proper operator chaining
searchTerms$.pipe(
debounceTime(300),
switchMap(term => fetchResults(term)),
mergeMap(results =>
from(results).pipe(
concatMap(result =>
fetchDetails(result.id).pipe(
map(details => ({ result, details }))
)
)
)
)
).subscribe(({ result, details }) => {
displayResultWithDetails(result, details);
});
// Anti-pattern: Not handling subscription cleanup
ngOnInit() {
this.userActivity$.subscribe(activity => {
this.updateUserInterface(activity);
});
}
// Correct pattern: Proper cleanup
ngOnInit() {
this.subscription = this.userActivity$.subscribe(activity => {
this.updateUserInterface(activity);
});
}
ngOnDestroy() {
this.subscription.unsubscribe();
}
Chapter 12: Real-World Project - Building a Reactive Weather Dashboard
The final chapter guides you through building a complete application using reactive principles:
- Designing a reactive architecture for a weather dashboard
- Implementing real-time updates from multiple data sources
- Managing complex UI state reactively
- Adding interactive features like search and favorites
- Optimizing performance for production
Key Takeaway: Consolidate everything you've learned by building a practical, real-world application that showcases the power of reactive programming.
// Excerpt from the Weather Dashboard project
class WeatherDashboard {
constructor() {
// Core observables
this.locationInput$ = fromEvent(this.locationInput, 'input').pipe(
map(e => e.target.value),
debounceTime(300),
distinctUntilChanged()
);
this.currentLocation$ = new BehaviorSubject(null);
this.userPreferences$ = this.preferencesService.getPreferences();
// Derived data streams
this.currentWeather$ = this.currentLocation$.pipe(
filter(location => !!location),
switchMap(location => this.weatherService.getCurrentWeather(location)),
catchError(err => {
this.showError('Could not fetch current weather');
return EMPTY;
}),
shareReplay(1)
);
this.forecast$ = this.currentLocation$.pipe(
filter(location => !!location),
switchMap(location =>
this.weatherService.getForecast(location, 5)
),
shareReplay(1)
);
// Combining streams for UI
this.dashboardData$ = combineLatest([
this.currentWeather$,
this.forecast$,
this.userPreferences$
]).pipe(
map(([current, forecast, preferences]) => {
return {
current: this.applyUserPreferences(current, preferences),
forecast: forecast.map(day =>
this.applyUserPreferences(day, preferences)
),
units: preferences.units
};
})
);
// Auto-refresh weather data every 10 minutes
this.refresh$ = interval(600000).pipe(
startWith(0),
withLatestFrom(this.currentLocation$),
filter(([_, location]) => !!location),
tap(([_, location]) => {
this.weatherService.invalidateCache(location);
this.currentLocation$.next(location);
})
);
}
init() {
// Setup main data subscription
this.dashboardData$.subscribe(data => {
this.renderDashboard(data);
});
// Setup location search
this.locationInput$.pipe(
switchMap(query =>
this.geocodingService.searchLocations(query)
)
).subscribe(suggestions => {
this.showLocationSuggestions(suggestions);
});
// Setup auto-refresh
this.refresh$.subscribe();
// Initialize with user's saved location or geolocate
this.initializeUserLocation();
}
}
The Reactive Programming Advantage: Key Takeaways
Throughout this book, you'll discover how reactive programming transforms your approach to JavaScript development:
1. Declarative over Imperative
Reactive programming allows you to describe what you want to happen, not how it should happen. This declarative approach makes your code more readable and maintainable, as it separates the what from the how.
2. Unified Asynchronous Model
Instead of juggling different asynchronous patterns (callbacks, promises, event listeners), reactive programming provides a consistent model for handling all types of asynchronous data. Everything becomes a stream, simplifying your mental model.
3. Superior Composition
Complex asynchronous workflows that would require nested callbacks or complex Promise chains can be expressed elegantly through observable composition, making your code more modular and easier to reason about.
4. Built-in Error Handling
Reactive programming provides robust error handling mechanisms that allow for graceful recovery from failures, improving application resilience and user experience.
5. Cancellation by Design
Unlike Promises, Observables support cancellation out of the box, allowing you to clean up resources and prevent unnecessary operations when they're no longer needed.
6. Backpressure Management
When dealing with high-frequency events or large data volumes, reactive programming offers strategies to handle backpressure, ensuring your application remains responsive even under load.
Learning Path: From Beginner to Reactive Expert
This book is structured to accommodate developers at different stages of their reactive programming journey:
For Beginners
If you're new to reactive programming, start with Chapters 1-5, which provide a solid foundation in the core concepts and basic RxJS usage. Practice with the examples and exercises before moving on.
For Intermediate Developers
If you're familiar with basic reactive concepts but want to deepen your knowledge, focus on Chapters 6-9, which cover advanced operators, error handling, stream combination, and state management.
For Advanced Developers
If you're looking to apply reactive programming in production environments, pay special attention to Chapters 10-12, which address integration with frameworks, patterns for large-scale applications, and real-world implementation strategies.
Tools and Resources
Throughout the book, you'll find references to helpful tools and resources to enhance your reactive programming journey:
- RxJS Marbles: Interactive diagrams for visualizing operator behavior
- RxJS DevTools: Browser extensions for debugging observable streams
- RxViz: Visualization tool for reactive streams
- Recommended libraries: Compatible utility libraries that work well with RxJS
- Online playgrounds: Environments for experimenting with reactive code
Conclusion: Embracing the Reactive Future
As web applications continue to grow in complexity, reactive programming has emerged as a powerful paradigm for managing that complexity. By providing a consistent model for handling asynchronous operations, multiple events, and complex data flows, it enables developers to build more responsive, resilient, and maintainable applications.
"Reactive Programming in JavaScript" equips you with the knowledge and skills to harness this paradigm effectively. From understanding the fundamental concepts to implementing advanced patterns, this book is your comprehensive guide to mastering reactive programming and transforming your approach to JavaScript development.
Whether you're building a simple interactive website or a complex enterprise application, the principles and techniques you'll learn in this book will help you write better code and deliver superior user experiences. The reactive revolution is here—and now you have the tools to be part of it.
Are you ready to transform your JavaScript development approach? Turn the page and begin your reactive programming journey!
"Reactive Programming in JavaScript: Building Scalable, Event-Driven Applications with RxJS and Observables" is the definitive guide for JavaScript developers looking to master reactive programming techniques. With comprehensive coverage of RxJS, practical examples, and best practices, this book will elevate your ability to build responsive, resilient, and maintainable applications for today's demanding web environment.
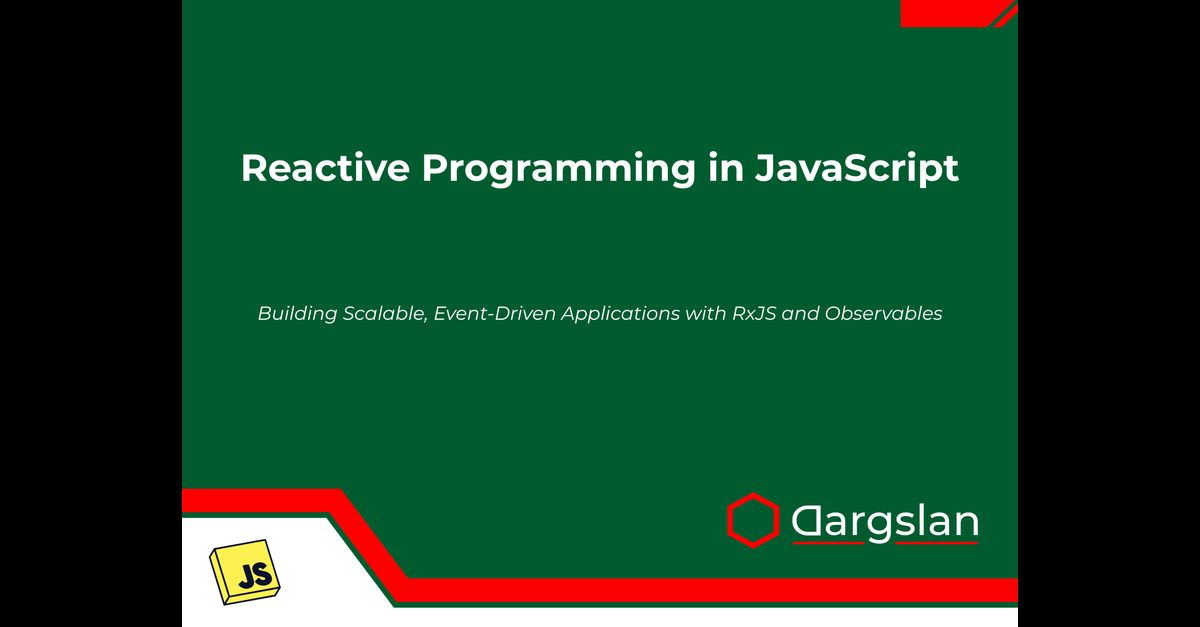